KDECore
KInetSocketAddress Class Reference
An Inet (IPv4 or IPv6) socket address. More...
#include <ksockaddr.h>
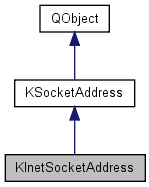
Public Member Functions | |
const sockaddr_in * | addressV4 () const |
const sockaddr_in6 * | addressV6 () const |
Q_UINT32 | flowinfo () const |
in_addr | hostV4 () const |
in6_addr | hostV6 () const |
KInetSocketAddress (const QString &addr, unsigned short port, int family=-1) | |
KInetSocketAddress (const in6_addr &addr, unsigned short port) | |
KInetSocketAddress (const in_addr &addr, unsigned short port) | |
KInetSocketAddress (const sockaddr_in6 *sin6, ksocklen_t len) | |
KInetSocketAddress (const sockaddr_in *sin, ksocklen_t len) | |
KInetSocketAddress (const KInetSocketAddress &) | |
KInetSocketAddress () | |
virtual QString | nodeName () const |
operator const sockaddr_in * () const | |
operator const sockaddr_in6 * () const | |
KInetSocketAddress & | operator= (const KInetSocketAddress &other) |
unsigned short | port () const |
virtual QString | pretty () const |
int | scopeId () const |
virtual QString | serviceName () const |
bool | setAddress (const QString &addr, unsigned short port, int family=-1) |
bool | setAddress (const in6_addr &addr, unsigned short port) |
bool | setAddress (const in_addr &addr, unsigned short port) |
bool | setAddress (const sockaddr_in6 *sin6, ksocklen_t len) |
bool | setAddress (const sockaddr_in *sin, ksocklen_t len) |
bool | setAddress (const KInetSocketAddress &ksa) |
bool | setFamily (int family) |
bool | setFlowinfo (Q_UINT32 flowinfo) |
bool | setHost (const QString &addr, int family=-1) |
bool | setHost (const in6_addr &addr) |
bool | setHost (const in_addr &addr) |
bool | setPort (unsigned short port) |
bool | setScopeId (int scopeid) |
virtual ksocklen_t | size () const |
virtual | ~KInetSocketAddress () |
Static Public Member Functions | |
static QString | addrToString (int family, const void *addr) |
static bool | areEqualInet (const KSocketAddress &s1, const KSocketAddress &s2, bool coreOnly) |
static bool | areEqualInet6 (const KSocketAddress &s1, const KSocketAddress &s2, bool coreOnly) |
static bool | stringToAddr (int family, const char *text, void *dest) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
An Inet (IPv4 or IPv6) socket address.This is an IPv4 or IPv6 address of the Internet
This class inherits most of the functionality from KSocketAddress, but is targeted specifically to Internet addresses
Definition at line 233 of file ksockaddr.h.
Constructor & Destructor Documentation
KInetSocketAddress::KInetSocketAddress | ( | ) |
KInetSocketAddress::KInetSocketAddress | ( | const KInetSocketAddress & | other | ) |
KInetSocketAddress::KInetSocketAddress | ( | const sockaddr_in * | sin, | |
ksocklen_t | len | |||
) |
Creates an IPv4 socket from raw sockaddr_in.
- Parameters:
-
sin a sockaddr_in structure to copy from len the socket address length
Definition at line 282 of file ksockaddr.cpp.
KInetSocketAddress::KInetSocketAddress | ( | const sockaddr_in6 * | sin6, | |
ksocklen_t | len | |||
) |
Creates an IPv6 socket from raw sockaddr_in6.
- Parameters:
-
sin6 a sockaddr_in6 structure to copy from len the socket address length
Definition at line 288 of file ksockaddr.cpp.
KInetSocketAddress::KInetSocketAddress | ( | const in_addr & | addr, | |
unsigned short | port | |||
) |
Creates a socket from information.
- Parameters:
-
addr a binary address port a port number
Definition at line 294 of file ksockaddr.cpp.
KInetSocketAddress::KInetSocketAddress | ( | const in6_addr & | addr, | |
unsigned short | port | |||
) |
Creates a socket from information.
- Parameters:
-
addr a binary address port a port number
Definition at line 300 of file ksockaddr.cpp.
KInetSocketAddress::KInetSocketAddress | ( | const QString & | addr, | |
unsigned short | port, | |||
int | family = -1 | |||
) |
Creates a socket from text representation.
- Parameters:
-
addr a text representation of the address port a port number family the family for this address. Use -1 to guess the family type
- See also:
- setAddress
Definition at line 306 of file ksockaddr.cpp.
KInetSocketAddress::~KInetSocketAddress | ( | ) | [virtual] |
Member Function Documentation
const sockaddr_in * KInetSocketAddress::addressV4 | ( | ) | const |
Returns the socket address.
This will be NULL if this is a non-convertible v6. This function will return an IPv4 socket if this IPv6 socket is a v4-mapped address. That is, if it's really an IPv4 address, but in v6 disguise.
- Returns:
- the sockaddr_in struct, can be 0.
Definition at line 513 of file ksockaddr.cpp.
const sockaddr_in6 * KInetSocketAddress::addressV6 | ( | ) | const |
Returns the socket address in IPv6.
- Returns:
- the sockaddr_in struct, can be 0 if IPv6 is unsupported.
Definition at line 532 of file ksockaddr.cpp.
QString KInetSocketAddress::addrToString | ( | int | family, | |
const void * | addr | |||
) | [static] |
Convert s the given raw address into text form.
This function returns QString::null if the address cannot be converted.
- Parameters:
-
family the family of the address addr the address, in raw form
- Returns:
- the converted address, or QString::null if not possible.
Definition at line 716 of file ksockaddr.cpp.
bool KInetSocketAddress::areEqualInet | ( | const KSocketAddress & | s1, | |
const KSocketAddress & | s2, | |||
bool | coreOnly | |||
) | [static] |
Compares two IPv4 addresses.
- Parameters:
-
s1 the first address to compare s2 the second address to compare coreOnly true if only core parts should be compared (only the address)
- Returns:
- true if the given addresses are equal.
Definition at line 629 of file ksockaddr.cpp.
bool KInetSocketAddress::areEqualInet6 | ( | const KSocketAddress & | s1, | |
const KSocketAddress & | s2, | |||
bool | coreOnly | |||
) | [static] |
Compares two IPv6 addresses.
- Parameters:
-
s1 the first address to compare s2 the second address to compare coreOnly true if only core parts should be compared (only the address)
- Returns:
- true if the given addresses are equal.
Definition at line 646 of file ksockaddr.cpp.
Q_UINT32 KInetSocketAddress::flowinfo | ( | ) | const |
Returns flowinfo for IPv6 socket.
- Returns:
- the flowinfo, 0 if unsupported
Definition at line 608 of file ksockaddr.cpp.
in_addr KInetSocketAddress::hostV4 | ( | ) | const |
Returns the host address.
Might be empty.
- Returns:
- the host address
Definition at line 541 of file ksockaddr.cpp.
in6_addr KInetSocketAddress::hostV6 | ( | ) | const |
Returns the host address.
WARNING: this function is not defined if there is no IPv6 support
- Returns:
- the host address
QString KInetSocketAddress::nodeName | ( | ) | const [virtual] |
Returns the text representation of the host address.
- Returns:
- a text representation of the host address
Reimplemented from KSocketAddress.
Definition at line 574 of file ksockaddr.cpp.
KInetSocketAddress::operator const sockaddr_in * | ( | ) | const [inline] |
Returns the socket address.
This will be NULL if this is a non-convertible v6.
- Returns:
- the sockaddr_in structure, can be 0 if v6.
- See also:
- addressV4()
Definition at line 514 of file ksockaddr.h.
KInetSocketAddress::operator const sockaddr_in6 * | ( | ) | const [inline] |
Returns the socket address.
- Returns:
- the sockaddr_in structure, can be 0 if v6 is unsupported.
- See also:
- addressV6()
Definition at line 522 of file ksockaddr.h.
KInetSocketAddress& KInetSocketAddress::operator= | ( | const KInetSocketAddress & | other | ) | [inline] |
unsigned short KInetSocketAddress::port | ( | ) | const |
QString KInetSocketAddress::pretty | ( | ) | const [virtual] |
Returns a pretty representation of this address.
- Returns:
- a pretty representation
Reimplemented from KSocketAddress.
Definition at line 559 of file ksockaddr.cpp.
int KInetSocketAddress::scopeId | ( | ) | const |
Returns the scope id for this IPv6 socket.
- Returns:
- the scope id
QString KInetSocketAddress::serviceName | ( | ) | const [virtual] |
Returns the text representation of the port number.
- Returns:
- the name of the service (a number)
Reimplemented from KSocketAddress.
Definition at line 593 of file ksockaddr.cpp.
Sets this socket to text address and port.
You can use the family
parameter to specify what kind of socket you want this to be. It could be AF_INET or AF_INET6 or -1.
If the value is -1 (default), this function will make an effort to discover what is the family. That isn't too hard, actually, and it works in all cases. But, if you want to be sure that your socket is of the type you want, use this parameter.
This function returns false if the socket address was not valid.
- Parameters:
-
addr the address port the port number family the address family, -1 for any
- Returns:
- true if successful, false otherwise
Definition at line 380 of file ksockaddr.cpp.
bool KInetSocketAddress::setAddress | ( | const in6_addr & | addr, | |
unsigned short | port | |||
) |
Sets this socket to raw address and port.
- Parameters:
-
addr the address port the port number
- Returns:
- true if successful, false otherwise
Definition at line 375 of file ksockaddr.cpp.
bool KInetSocketAddress::setAddress | ( | const in_addr & | addr, | |
unsigned short | port | |||
) |
Sets this socket to raw address and port.
- Parameters:
-
addr the address port the port number
- Returns:
- true if successful, false otherwise
Definition at line 370 of file ksockaddr.cpp.
bool KInetSocketAddress::setAddress | ( | const sockaddr_in6 * | sin6, | |
ksocklen_t | len | |||
) |
Sets this socket to given raw socket.
Note: this function does not clear the scope ID and flow info values
- Parameters:
-
sin6 the raw socket len the socket address length
- Returns:
- true if successful, false otherwise
Definition at line 342 of file ksockaddr.cpp.
bool KInetSocketAddress::setAddress | ( | const sockaddr_in * | sin, | |
ksocklen_t | len | |||
) |
Sets this socket to given raw socket.
- Parameters:
-
sin the raw socket len the socket address length
- Returns:
- true if successful, false otherwise
Definition at line 330 of file ksockaddr.cpp.
bool KInetSocketAddress::setAddress | ( | const KInetSocketAddress & | ksa | ) |
Sets this socket to given socket.
- Parameters:
-
ksa the other socket
- Returns:
- true if successful, false otherwise
Definition at line 319 of file ksockaddr.cpp.
bool KInetSocketAddress::setFamily | ( | int | family | ) |
Turns this into an IPv4 or IPv6 address.
- Parameters:
-
family the new address family
- Returns:
- false if this is v6 and information was lost. That doesn't mean the conversion was unsuccessful.
Definition at line 465 of file ksockaddr.cpp.
bool KInetSocketAddress::setFlowinfo | ( | Q_UINT32 | flowinfo | ) |
Sets flowinfo information for this socket address if this is IPv6.
- Parameters:
-
flowinfo flowinfo
- Returns:
- true if successful, false otherwise
Definition at line 488 of file ksockaddr.cpp.
Sets this socket's host address to given text representation.
- Parameters:
-
addr the address family the address family, -1 to guess the family
- Returns:
- true if successful, false otherwise
Definition at line 405 of file ksockaddr.cpp.
bool KInetSocketAddress::setHost | ( | const in6_addr & | addr | ) |
Sets this socket's host address to given raw address.
- Parameters:
-
addr the address
- Returns:
- true if successful, false otherwise
Definition at line 393 of file ksockaddr.cpp.
bool KInetSocketAddress::setHost | ( | const in_addr & | addr | ) |
Sets this socket's host address to given raw address.
- Parameters:
-
addr the address
- Returns:
- true if successful, false otherwise
Definition at line 385 of file ksockaddr.cpp.
bool KInetSocketAddress::setPort | ( | unsigned short | port | ) |
Sets this socket's port number to given port number.
- Parameters:
-
port the port number
- Returns:
- true if successful, false otherwise
Definition at line 454 of file ksockaddr.cpp.
bool KInetSocketAddress::setScopeId | ( | int | scopeid | ) |
Sets the scope id for this socket if this is IPv6.
- Parameters:
-
scopeid the scope id
- Returns:
- true if successful, false otherwise
Definition at line 500 of file ksockaddr.cpp.
ksocklen_t KInetSocketAddress::size | ( | ) | const [virtual] |
Returns the socket length.
Will be either sizeof(sockaddr_in) or sizeof(sockaddr_in6)
- Returns:
- the length of the socket
Reimplemented from KSocketAddress.
Definition at line 617 of file ksockaddr.cpp.
bool KInetSocketAddress::stringToAddr | ( | int | family, | |
const char * | text, | |||
void * | dest | |||
) | [static] |
Converts the address given in text form into raw form.
The size of the destination buffer dest
is supposed to be large enough to hold the address of the given family.
- Parameters:
-
family the family of the address text the text representation of the address dest the destination buffer of the address
- Returns:
- true if convertion was successful.
Definition at line 723 of file ksockaddr.cpp.
void KInetSocketAddress::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
The documentation for this class was generated from the following files: