KDECore
KSocketAddress Class Reference
A socket address. More...
#include <ksockaddr.h>
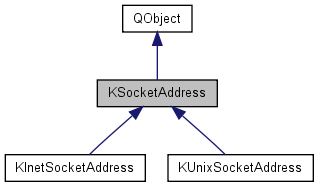
Public Member Functions | |
const sockaddr * | address () const |
int | family () const |
int | ianaFamily () const |
bool | isCoreEqual (const KSocketAddress *other) const |
bool | isCoreEqual (const KSocketAddress &other) const |
bool | isEqual (const KSocketAddress *other) const |
virtual bool | isEqual (const KSocketAddress &other) const |
virtual QString | nodeName () const |
operator const sockaddr * () const | |
bool | operator== (const KSocketAddress &other) const |
virtual QString | pretty () const |
virtual QString | serviceName () const |
virtual ksocklen_t | size () const |
virtual | ~KSocketAddress () |
Static Public Member Functions | |
static int | fromIanaFamily (int iana) |
static int | ianaFamily (int af) |
static KSocketAddress * | newAddress (const struct sockaddr *sa, ksocklen_t size) |
Protected Member Functions | |
KSocketAddress (const sockaddr *sa, ksocklen_t size) | |
KSocketAddress () | |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
sockaddr * | data |
ksocklen_t | datasize |
bool | owndata |
Detailed Description
A socket address.This class envelopes almost if not all socket addresses.
Definition at line 46 of file ksockaddr.h.
Constructor & Destructor Documentation
KSocketAddress::KSocketAddress | ( | ) | [inline, protected] |
KSocketAddress::KSocketAddress | ( | const sockaddr * | sa, | |
ksocklen_t | size | |||
) | [protected] |
Creates with given data.
Class KSocketAddress.
- Parameters:
-
sa a sockaddr structure size the size of sa
Definition at line 83 of file ksockaddr.cpp.
KSocketAddress::~KSocketAddress | ( | ) | [virtual] |
Member Function Documentation
const sockaddr* KSocketAddress::address | ( | ) | const [inline] |
Returns a sockaddr structure, for passing down to library functions.
- Returns:
- the sockaddr structure, can be 0
Reimplemented in KUnixSocketAddress.
Definition at line 78 of file ksockaddr.h.
int KSocketAddress::family | ( | ) | const |
Returns the family of this address.
- Returns:
- the family of this address, AF_UNSPEC if it's undefined
Definition at line 115 of file ksockaddr.cpp.
int KSocketAddress::fromIanaFamily | ( | int | iana | ) | [static] |
Returns the address family of the given IANA family number.
- Returns:
- the address family, AF_UNSPEC for unknown IANA family numbers
Definition at line 221 of file ksockaddr.cpp.
int KSocketAddress::ianaFamily | ( | int | af | ) | [static] |
Returns the IANA family number of the given address family.
Returns 0 if there is no corresponding IANA family number.
- Parameters:
-
af the address family, in AF_* constants
- Returns:
- the IANA family number of this address (1 for AF_INET. 2 for AF_INET6, otherwise 0)
Definition at line 206 of file ksockaddr.cpp.
int KSocketAddress::ianaFamily | ( | ) | const [inline] |
Returns the IANA family number of this address.
- Returns:
- the IANA family number of this address (1 for AF_INET. 2 for AF_INET6, otherwise 0)
Definition at line 107 of file ksockaddr.h.
bool KSocketAddress::isCoreEqual | ( | const KSocketAddress * | other | ) | const [inline] |
Some sockets may differ in such things as services or port numbers, like Internet sockets.
This function compares only the core part of that, if possible.
If not possible, like the default implementation, this returns the same as isEqual.
- Parameters:
-
other the other socket
- Returns:
- true if the code part is equal
Definition at line 148 of file ksockaddr.h.
bool KSocketAddress::isCoreEqual | ( | const KSocketAddress & | other | ) | const |
Some sockets may differ in such things as services or port numbers, like Internet sockets.
This function compares only the core part of that, if possible.
If not possible, like the default implementation, this returns the same as isEqual.
- Parameters:
-
other the other socket
- Returns:
- true if the code part is equal
Definition at line 179 of file ksockaddr.cpp.
bool KSocketAddress::isEqual | ( | const KSocketAddress * | other | ) | const [inline] |
Definition at line 116 of file ksockaddr.h.
bool KSocketAddress::isEqual | ( | const KSocketAddress & | other | ) | const [virtual] |
Returns true if this equals the other socket.
- Parameters:
-
other the other socket
- Returns:
- true if both sockets are equal
Definition at line 159 of file ksockaddr.cpp.
KSocketAddress * KSocketAddress::newAddress | ( | const struct sockaddr * | sa, | |
ksocklen_t | size | |||
) | [static] |
Creates a new KSocketAddress or descendant class from given raw socket address.
- Parameters:
-
sa new socket address size new socket address's length
- Returns:
- the new KSocketAddress, or 0 if the function failed
Definition at line 123 of file ksockaddr.cpp.
QString KSocketAddress::nodeName | ( | ) | const [virtual] |
Returns the node name of this socket, as KExtendedSocket::lookup expects as the first argument.
In the case of Internet sockets, this is the hostname. The default implementation returns QString::null.
- Returns:
- the node name, can be QString::null
Reimplemented in KInetSocketAddress.
Definition at line 196 of file ksockaddr.cpp.
KSocketAddress::operator const sockaddr * | ( | ) | const [inline] |
Returns a sockaddr structure, for passing down to library functions.
- Returns:
- the sockaddr structure, can be 0.
- See also:
- address()
Definition at line 93 of file ksockaddr.h.
bool KSocketAddress::operator== | ( | const KSocketAddress & | other | ) | const [inline] |
QString KSocketAddress::pretty | ( | ) | const [virtual] |
Returns a string representation of this socket.
- Returns:
- a pretty string representation
Reimplemented in KInetSocketAddress, and KUnixSocketAddress.
Definition at line 110 of file ksockaddr.cpp.
QString KSocketAddress::serviceName | ( | ) | const [virtual] |
Returns the service name for this socket, as KExtendedSocket::lookup expects as the service argument.
In the case of Internet sockets, this is the port number. The default implementation returns QString::null.
- Returns:
- the service name, can be QString::null
Reimplemented in KInetSocketAddress, and KUnixSocketAddress.
Definition at line 201 of file ksockaddr.cpp.
virtual ksocklen_t KSocketAddress::size | ( | ) | const [inline, virtual] |
Returns sockaddr structure size.
- Returns:
- the size of the sockaddr structre, 0 if there is none.
Reimplemented in KInetSocketAddress.
Definition at line 85 of file ksockaddr.h.
void KSocketAddress::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KInetSocketAddress, and KUnixSocketAddress.
Definition at line 884 of file ksockaddr.cpp.
Member Data Documentation
sockaddr* KSocketAddress::data [protected] |
Definition at line 170 of file ksockaddr.h.
ksocklen_t KSocketAddress::datasize [protected] |
Definition at line 171 of file ksockaddr.h.
bool KSocketAddress::owndata [protected] |
Definition at line 172 of file ksockaddr.h.
The documentation for this class was generated from the following files: