KDECore
KNetwork::KBufferedSocket Class Reference
Buffered stream sockets. More...
#include <kbufferedsocket.h>
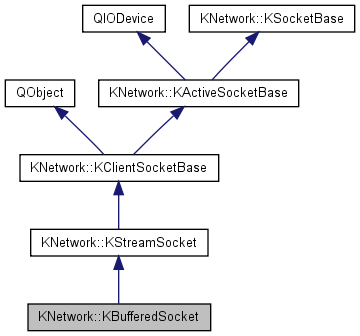
Signals | |
void | bytesWritten (int bytes) |
Public Member Functions | |
virtual Q_LONG | bytesAvailable () const |
virtual Q_ULONG | bytesToWrite () const |
bool | canReadLine () const |
virtual void | close () |
virtual void | closeNow () |
virtual void | enableRead (bool enable) |
virtual void | enableWrite (bool enable) |
KIOBufferBase * | inputBuffer () |
KBufferedSocket (const QString &node=QString::null, const QString &service=QString::null, QObject *parent=0L, const char *name=0L) | |
KIOBufferBase * | outputBuffer () |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen, KSocketAddress &from) |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen) |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen, KSocketAddress &from) |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen) |
QCString | readLine () |
void | reset () |
void | setInputBuffering (bool enable) |
void | setOutputBuffering (bool enable) |
virtual void | setSocketDevice (KSocketDevice *device) |
void | waitForConnect () |
virtual Q_LONG | waitForMore (int msecs, bool *timeout=0L) |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len, const KSocketAddress &to) |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len) |
virtual | ~KBufferedSocket () |
Protected Slots | |
virtual void | slotReadActivity () |
virtual void | slotWriteActivity () |
Protected Member Functions | |
virtual bool | setSocketOptions (int opts) |
virtual void | stateChanging (SocketState newState) |
Detailed Description
Buffered stream sockets.This class allows the user to create and operate buffered stream sockets such as those used in most Internet connections. This class is also the one that resembles the most to the old QSocket implementation.
Objects of this type operate only in non-blocking mode. A call to setBlocking(true) will result in an error.
- Note:
- Buffered sockets only make sense if you're using them from the main (event-loop) thread. This is actually a restriction imposed by Qt's QSocketNotifier. If you want to use a socket in an auxiliary thread, please use KStreamSocket.
Definition at line 58 of file kbufferedsocket.h.
Constructor & Destructor Documentation
KBufferedSocket::KBufferedSocket | ( | const QString & | node = QString::null , |
|
const QString & | service = QString::null , |
|||
QObject * | parent = 0L , |
|||
const char * | name = 0L | |||
) |
Default constructor.
- Parameters:
-
node destination host service destination service to connect to parent the parent object for this object name the internal name for this object
Definition at line 50 of file kbufferedsocket.cpp.
KBufferedSocket::~KBufferedSocket | ( | ) | [virtual] |
Member Function Documentation
Q_LONG KBufferedSocket::bytesAvailable | ( | ) | const [virtual] |
Make use of the buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 96 of file kbufferedsocket.cpp.
Q_ULONG KBufferedSocket::bytesToWrite | ( | ) | const [virtual] |
void KNetwork::KBufferedSocket::bytesWritten | ( | int | bytes | ) | [signal] |
This signal is emitted whenever data is written.
bool KBufferedSocket::canReadLine | ( | ) | const |
Returns true if a line can be read with readLine.
Definition at line 297 of file kbufferedsocket.cpp.
void KBufferedSocket::close | ( | ) | [virtual] |
Closes the socket for new data, but allow data that had been buffered for output with writeBlock to be still be written.
- See also:
- closeNow
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 82 of file kbufferedsocket.cpp.
void KBufferedSocket::closeNow | ( | ) | [virtual] |
Closes the socket and discards any output data that had been buffered with writeBlock but that had not yet been written.
- See also:
- close
Definition at line 290 of file kbufferedsocket.cpp.
void KBufferedSocket::enableRead | ( | bool | enable | ) | [virtual] |
Catch changes.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 197 of file kbufferedsocket.cpp.
void KBufferedSocket::enableWrite | ( | bool | enable | ) | [virtual] |
Catch changes.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 214 of file kbufferedsocket.cpp.
KIOBufferBase * KBufferedSocket::inputBuffer | ( | ) |
KIOBufferBase * KBufferedSocket::outputBuffer | ( | ) |
Q_LONG KBufferedSocket::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Peeks data from the socket.
The from
parameter is always set to peerAddress()
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 154 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [virtual] |
Peeks data from the socket.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 138 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::readBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Reads data from a socket.
The from
parameter is always set to peerAddress()
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 132 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::readBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [virtual] |
Reads data from the socket.
Make use of buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 116 of file kbufferedsocket.cpp.
QCString KBufferedSocket::readLine | ( | ) |
void KNetwork::KBufferedSocket::reset | ( | ) | [inline] |
- Deprecated:
- Closes the socket.
Reimplemented from QIODevice.
Definition at line 247 of file kbufferedsocket.h.
void KBufferedSocket::setInputBuffering | ( | bool | enable | ) |
void KBufferedSocket::setOutputBuffering | ( | bool | enable | ) |
void KBufferedSocket::setSocketDevice | ( | KSocketDevice * | device | ) | [virtual] |
Be sure to catch new devices.
Reimplemented from KNetwork::KSocketBase.
Definition at line 67 of file kbufferedsocket.cpp.
bool KBufferedSocket::setSocketOptions | ( | int | opts | ) | [protected, virtual] |
Buffered sockets can only operate in non-blocking mode.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 73 of file kbufferedsocket.cpp.
void KBufferedSocket::slotReadActivity | ( | ) | [protected, virtual, slot] |
Slot called when there's read activity.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 320 of file kbufferedsocket.cpp.
void KBufferedSocket::slotWriteActivity | ( | ) | [protected, virtual, slot] |
Slot called when there's write activity.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 367 of file kbufferedsocket.cpp.
void KBufferedSocket::stateChanging | ( | SocketState | newState | ) | [protected, virtual] |
Catch connection to clear the buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 226 of file kbufferedsocket.cpp.
void KBufferedSocket::waitForConnect | ( | ) |
Blocks until the connection is either established, or completely failed.
Definition at line 310 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::waitForMore | ( | int | msecs, | |
bool * | timeout = 0L | |||
) | [virtual] |
Make use of buffers.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 104 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::writeBlock | ( | const char * | data, | |
Q_ULONG | len, | |||
const KSocketAddress & | to | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Writes data to the socket.
The to
parameter is discarded.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 190 of file kbufferedsocket.cpp.
Q_LONG KBufferedSocket::writeBlock | ( | const char * | data, | |
Q_ULONG | len | |||
) | [virtual] |
Writes data to the socket.
Reimplemented from KNetwork::KClientSocketBase.
Definition at line 160 of file kbufferedsocket.cpp.
The documentation for this class was generated from the following files: