KDECore
KNetwork::KClientSocketBase Class Reference
Abstract client socket class. More...
#include <kclientsocketbase.h>
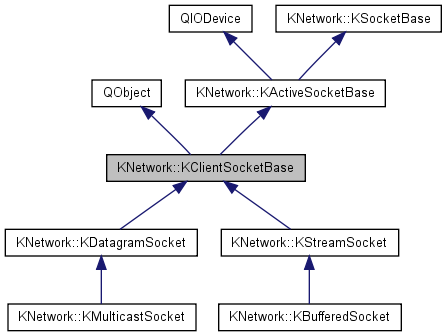
Public Types | |
enum | SocketState { Idle, HostLookup, HostFound, Bound, Connecting, Open, Closing, Unconnected = Bound, Connected = Open, Connection = Open } |
Signals | |
void | aboutToConnect (const KResolverEntry &remote, bool &skip) |
void | bound (const KResolverEntry &local) |
void | closed () |
void | connected (const KResolverEntry &remote) |
void | gotError (int code) |
void | hostFound () |
void | readyRead () |
void | readyWrite () |
void | stateChanged (int newstate) |
Public Member Functions | |
virtual bool | bind (const KResolverEntry &address) |
virtual bool | bind (const QString &node=QString::null, const QString &service=QString::null)=0 |
virtual Q_LONG | bytesAvailable () const |
virtual void | close () |
virtual bool | connect (const KResolverEntry &address) |
virtual bool | connect (const QString &node=QString::null, const QString &service=QString::null)=0 |
void | connectToHost (const QString &host, Q_UINT16 port) |
virtual bool | disconnect () |
bool | emitsReadyRead () const |
bool | emitsReadyWrite () const |
virtual void | enableRead (bool enable) |
virtual void | enableWrite (bool enable) |
virtual void | flush () |
KClientSocketBase (QObject *parent, const char *name) | |
virtual KSocketAddress | localAddress () const |
KResolver & | localResolver () const |
const KResolverResults & | localResults () const |
virtual bool | lookup () |
virtual bool | open (int) |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen, KSocketAddress &from) |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen) |
virtual KSocketAddress | peerAddress () const |
KResolver & | peerResolver () const |
const KResolverResults & | peerResults () const |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen, KSocketAddress &from) |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen) |
void | setFamily (int families) |
void | setResolutionEnabled (bool enable) |
SocketState | state () const |
virtual Q_LONG | waitForMore (int msecs, bool *timeout=0L) |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len, const KSocketAddress &to) |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len) |
virtual | ~KClientSocketBase () |
Protected Slots | |
virtual void | slotReadActivity () |
virtual void | slotWriteActivity () |
Protected Member Functions | |
void | copyError () |
virtual bool | setSocketOptions (int opts) |
void | setState (SocketState state) |
virtual void | stateChanging (SocketState newState) |
Detailed Description
Abstract client socket class.This class provides the base functionality for client sockets, such as, and especially, name resolution and signals.
- Note:
- This class is abstract. If you're looking for a normal, client socket class, see KStreamSocket and KBufferedSocket
Definition at line 49 of file kclientsocketbase.h.
Member Enumeration Documentation
Socket states.
These are the possible states for a KClientSocketBase:
- Idle: socket is not connected
- HostLookup: socket is doing host lookup prior to connecting
- HostFound: name lookup is complete
- Bound: the socket is locally bound
- Connecting: socket is attempting connection
- Open: socket is open
- Connected (=Open): socket is connected
- Connection (=Open): yet another name for a connected socket
- Closing: socket is shutting down
Whenever the socket state changes, the stateChanged(int) signal will be emitted.
- Enumerator:
-
Idle HostLookup HostFound Bound Connecting Open Closing Unconnected Connected Connection
Definition at line 71 of file kclientsocketbase.h.
Constructor & Destructor Documentation
KClientSocketBase::KClientSocketBase | ( | QObject * | parent, | |
const char * | name | |||
) |
Default constructor.
- Parameters:
-
parent the parent QObject object name the name of this object
Definition at line 50 of file kclientsocketbase.cpp.
KClientSocketBase::~KClientSocketBase | ( | ) | [virtual] |
Member Function Documentation
void KNetwork::KClientSocketBase::aboutToConnect | ( | const KResolverEntry & | remote, | |
bool & | skip | |||
) | [signal] |
This signal is emitted when the socket is about to connect to an address (but before doing so).
The skip
parameter can be used to make the loop skip this address. Its value is initially false: change it to true if you want to skip the current address (as given by remote
).
This function is also useful if one wants to reset the timeout.
- Parameters:
-
remote the address we're about to connect to skip set to true if you want to skip this address
- Note:
- if the connection is successful, the connected signal will be emitted.
bool KClientSocketBase::bind | ( | const KResolverEntry & | address | ) | [virtual] |
Reimplemented from KSocketBase.
Connect this socket to this specific address.
Unlike bind(const QString&, const QString&) above, this function really does bind the socket. No lookup is performed. The bound signal will be emitted.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
Definition at line 189 of file kclientsocketbase.cpp.
virtual bool KNetwork::KClientSocketBase::bind | ( | const QString & | node = QString::null , |
|
const QString & | service = QString::null | |||
) | [pure virtual] |
Binds this socket to the given nodename and service, or use the default ones if none are given.
Upon successful binding, the bound signal will be emitted. If an error is found, the gotError signal will be emitted.
- Note:
- Due to the internals of the name lookup and binding mechanism, some (if not most) implementations of this function do not actually bind the socket until the connection is requested (see connect). They only set the values for future reference.
- Parameters:
-
node the nodename service the service
Implemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
void KNetwork::KClientSocketBase::bound | ( | const KResolverEntry & | local | ) | [signal] |
This signal is emitted when the socket successfully binds to an address.
- Parameters:
-
local the local address we bound to
Q_LONG KClientSocketBase::bytesAvailable | ( | ) | const [virtual] |
Returns the number of bytes available on this socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 281 of file kclientsocketbase.cpp.
void KClientSocketBase::close | ( | ) | [virtual] |
Closes the socket.
Reimplemented from QIODevice.
The closing of the socket causes the emission of the signal closed.
Reimplemented from QIODevice.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 261 of file kclientsocketbase.cpp.
void KNetwork::KClientSocketBase::closed | ( | ) | [signal] |
This signal is emitted when the socket completes the closing/shut down process.
bool KClientSocketBase::connect | ( | const KResolverEntry & | address | ) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
Definition at line 210 of file kclientsocketbase.cpp.
virtual bool KNetwork::KClientSocketBase::connect | ( | const QString & | node = QString::null , |
|
const QString & | service = QString::null | |||
) | [pure virtual] |
Attempts to connect to the these hostname and service, or use the default ones if none are given.
If a connection attempt is already in progress, check on its state and set the error status (NoError or InProgress).
If the blocking mode for this object is on, this function will only return when all the resolved peer addresses have been tried or when a connection is established.
Upon successfully connecting, the connected signal will be emitted. If an error is found, the gotError signal will be emitted.
- Note for derived classes:
- Derived classes must implement this function. The implementation will set the parameters for the lookup (using the peer KResolver object) and call lookup to start it.
- The implementation should use the hostFound signal to be notified of the completion of the lookup process and then proceed to start the connection itself. Care should be taken regarding the value of blocking flag.
- Parameters:
-
node the nodename service the service
Implemented in KNetwork::KDatagramSocket, and KNetwork::KStreamSocket.
void KNetwork::KClientSocketBase::connected | ( | const KResolverEntry & | remote | ) | [signal] |
This socket is emitted when the socket successfully connects to a remote address.
- Parameters:
-
remote the remote address we did connect to
void KNetwork::KClientSocketBase::connectToHost | ( | const QString & | host, | |
Q_UINT16 | port | |||
) | [inline] |
- Deprecated:
- This is a convenience function provided to ease migrating from Qt 3.x's QSocket class.
Definition at line 260 of file kclientsocketbase.h.
void KClientSocketBase::copyError | ( | ) | [protected] |
Convenience function to set this object's error code to match that of the socket device.
Definition at line 472 of file kclientsocketbase.cpp.
bool KClientSocketBase::disconnect | ( | ) | [virtual] |
Disconnects the socket.
Note that not all socket types can disconnect.
Implements KNetwork::KActiveSocketBase.
Definition at line 244 of file kclientsocketbase.cpp.
bool KClientSocketBase::emitsReadyRead | ( | ) | const |
Returns true if the readyRead signal is set to be emitted.
Definition at line 383 of file kclientsocketbase.cpp.
bool KClientSocketBase::emitsReadyWrite | ( | ) | const |
Returns true if the readyWrite signal is set to be emitted.
Definition at line 398 of file kclientsocketbase.cpp.
void KClientSocketBase::enableRead | ( | bool | enable | ) | [virtual] |
Enables the emission of the readyRead signal.
By default, this signal is enabled.
- Parameters:
-
enable whether to enable the signal
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 388 of file kclientsocketbase.cpp.
void KClientSocketBase::enableWrite | ( | bool | enable | ) | [virtual] |
Enables the emission of the readyWrite signal.
By default, this signal is disabled.
- Parameters:
-
enable whether to enable the signal
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 403 of file kclientsocketbase.cpp.
virtual void KNetwork::KClientSocketBase::flush | ( | ) | [inline, virtual] |
This call is not supported on sockets.
Reimplemented from QIODevice.
Reimplemented from QIODevice.
Definition at line 288 of file kclientsocketbase.h.
void KNetwork::KClientSocketBase::gotError | ( | int | code | ) | [signal] |
This signal is emitted when this object finds an error.
The code
parameter contains the error code that can also be found by calling error.
void KNetwork::KClientSocketBase::hostFound | ( | ) | [signal] |
This signal is emitted when the lookup is successfully completed.
KSocketAddress KClientSocketBase::localAddress | ( | ) | const [virtual] |
Returns the local socket address.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Definition at line 373 of file kclientsocketbase.cpp.
KResolver & KClientSocketBase::localResolver | ( | ) | const |
Returns the internal KResolver object used for looking up the local host name and service.
This can be used to set extra options to the lookup process other than the default values, as well as obtaining the error codes in case of lookup failure.
Definition at line 101 of file kclientsocketbase.cpp.
const KResolverResults & KClientSocketBase::localResults | ( | ) | const |
Returns the internal list of resolved results for the local address.
Definition at line 106 of file kclientsocketbase.cpp.
bool KClientSocketBase::lookup | ( | ) | [virtual] |
Starts the lookup for peer and local hostnames as well as their services.
If the blocking mode for this object is on, this function will wait for the lookup results to be available (by calling the KResolver::wait method on the resolver objects).
When the lookup is done, the signal hostFound will be emitted (only once, even if we're doing a double lookup). If the lookup failed (for any of the two lookups) the gotError signal will be emitted with the appropriate error condition (see KSocketBase::SocketError).
This function returns true on success and false on error. Note that this is not the lookup result!
Definition at line 131 of file kclientsocketbase.cpp.
virtual bool KNetwork::KClientSocketBase::open | ( | int | ) | [inline, virtual] |
Opens the socket.
Reimplemented from QIODevice.
You should not call this function; instead, use connect
Reimplemented from QIODevice.
Definition at line 274 of file kclientsocketbase.h.
Q_LONG KClientSocketBase::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Peeks data from the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 337 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [virtual] |
Peeks data from the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 325 of file kclientsocketbase.cpp.
KSocketAddress KClientSocketBase::peerAddress | ( | ) | const [virtual] |
Returns the peer socket address.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Definition at line 378 of file kclientsocketbase.cpp.
KResolver & KClientSocketBase::peerResolver | ( | ) | const |
Returns the internal KResolver object used for looking up the peer host name and service.
This can be used to set extra options to the lookup process other than the default values, as well as obtaining the error codes in case of lookup failure.
Definition at line 91 of file kclientsocketbase.cpp.
const KResolverResults & KClientSocketBase::peerResults | ( | ) | const |
Returns the internal list of resolved results for the peer address.
Definition at line 96 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::readBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Reads data from a socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 313 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::readBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [virtual] |
Reads data from a socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 301 of file kclientsocketbase.cpp.
void KNetwork::KClientSocketBase::readyRead | ( | ) | [signal] |
This signal is emitted whenever the socket is ready for reading -- i.e., there is data to be read in the buffers.
The subsequent read operation is guaranteed to be non-blocking.
You can toggle the emission of this signal with the enableRead function. This signal is by default enabled.
void KNetwork::KClientSocketBase::readyWrite | ( | ) | [signal] |
This signal is emitted whenever the socket is ready for writing -- i.e., whenever there's space available in the buffers to receive more data.
The subsequent write operation is guaranteed to be non-blocking.
You can toggle the emission of this signal with the enableWrite function. This signal is by default disabled. You will want to disable this signal after the first reception, since it'll probably fire at every event loop.
void KClientSocketBase::setFamily | ( | int | families | ) |
Sets the allowed families for the resolutions.
- Parameters:
-
families the families that we want/accept
- See also:
- KResolver::SocketFamilies for possible values
Definition at line 125 of file kclientsocketbase.cpp.
void KClientSocketBase::setResolutionEnabled | ( | bool | enable | ) |
Enables or disables name resolution.
If this flag is set to true, bind and connect operations will trigger name lookup operations (i.e., converting a hostname into its binary form). If the flag is set to false, those operations will instead try to convert a string representation of an address without attempting name resolution.
This is useful, for instance, when IP addresses are in their string representation (such as "1.2.3.4") or come from other sources like KSocketAddress.
- Parameters:
-
enable whether to enable
Definition at line 111 of file kclientsocketbase.cpp.
bool KClientSocketBase::setSocketOptions | ( | int | opts | ) | [protected, virtual] |
Sets the socket options.
Reimplemented from KSocketBase.
Reimplemented from KNetwork::KSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 75 of file kclientsocketbase.cpp.
void KClientSocketBase::setState | ( | SocketState | state | ) | [protected] |
Sets the socket state to state
.
This function does not emit the stateChanged signal.
Definition at line 69 of file kclientsocketbase.cpp.
void KClientSocketBase::slotReadActivity | ( | ) | [protected, virtual, slot] |
This slot is connected to the read notifier's signal meaning the socket can read more data.
The default implementation only emits the readyRead signal.
Override if your class requires processing of incoming data.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 413 of file kclientsocketbase.cpp.
void KClientSocketBase::slotWriteActivity | ( | ) | [protected, virtual, slot] |
This slot is connected to the write notifier's signal meaning the socket can write more data.
The default implementation only emits the readyWrite signal.
Override if your class writes data from another source (like a buffer).
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 419 of file kclientsocketbase.cpp.
KClientSocketBase::SocketState KClientSocketBase::state | ( | ) | const |
Returns the current state for this socket.
- See also:
- SocketState
Reimplemented from QIODevice.
Definition at line 64 of file kclientsocketbase.cpp.
void KNetwork::KClientSocketBase::stateChanged | ( | int | newstate | ) | [signal] |
This signal is emitted whenever the socket state changes.
Note: do not delete this object inside the slot called by this signal.
- Parameters:
-
newstate the new state of the socket object
void KClientSocketBase::stateChanging | ( | SocketState | newState | ) | [protected, virtual] |
This function is called by setState whenever the state changes.
You should override it if you need to specify any actions to be done when the state changes.
The default implementation acts for these states only:
- Connected: it sets up the socket notifiers to fire readyRead and readyWrite signals.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 448 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::waitForMore | ( | int | msecs, | |
bool * | timeout = 0L | |||
) | [virtual] |
Waits for more data.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 289 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::writeBlock | ( | const char * | data, | |
Q_ULONG | len, | |||
const KSocketAddress & | to | |||
) | [virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Writes data to the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket, and KNetwork::KDatagramSocket.
Definition at line 361 of file kclientsocketbase.cpp.
Q_LONG KClientSocketBase::writeBlock | ( | const char * | data, | |
Q_ULONG | len | |||
) | [virtual] |
Writes data to the socket.
Reimplemented from KSocketBase.
Implements KNetwork::KActiveSocketBase.
Reimplemented in KNetwork::KBufferedSocket.
Definition at line 349 of file kclientsocketbase.cpp.
The documentation for this class was generated from the following files: