KDECore
KNetwork::KActiveSocketBase Class Reference
Abstract class for active sockets. More...
#include <ksocketbase.h>
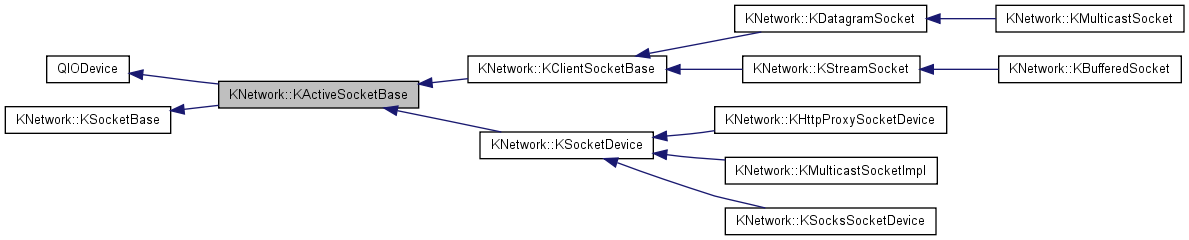
Public Member Functions | |
virtual bool | at (Offset) |
virtual Offset | at () const |
virtual bool | atEnd () const |
virtual bool | bind (const KResolverEntry &address)=0 |
virtual Q_LONG | bytesAvailable () const =0 |
virtual bool | connect (const KResolverEntry &address)=0 |
virtual bool | disconnect ()=0 |
virtual int | getch () |
KActiveSocketBase () | |
virtual KSocketAddress | localAddress () const =0 |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen, KSocketAddress &from)=0 |
virtual Q_LONG | peekBlock (char *data, Q_ULONG maxlen)=0 |
virtual KSocketAddress | peerAddress () const =0 |
virtual int | putch (int ch) |
virtual Q_LONG | readBlock (char *data, Q_ULONG maxlen, KSocketAddress &from)=0 |
virtual Q_LONG | readBlock (char *data, Q_ULONG len)=0 |
virtual Offset | size () const |
virtual int | ungetch (int) |
virtual Q_LONG | waitForMore (int msecs, bool *timeout=0L)=0 |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len, const KSocketAddress &to)=0 |
virtual Q_LONG | writeBlock (const char *data, Q_ULONG len)=0 |
virtual | ~KActiveSocketBase () |
Protected Member Functions | |
void | resetError () |
void | setError (int status, SocketError error) |
Detailed Description
Abstract class for active sockets.This class provides the standard interfaces for active sockets, i.e., sockets that are used to connect to external addresses.
Definition at line 443 of file ksocketbase.h.
Constructor & Destructor Documentation
KActiveSocketBase::KActiveSocketBase | ( | ) |
KActiveSocketBase::~KActiveSocketBase | ( | ) | [virtual] |
Member Function Documentation
This call is not supported on sockets.
Reimplemented from QIODevice. This will always return false.
Reimplemented from QIODevice.
Definition at line 521 of file ksocketbase.h.
virtual Offset KNetwork::KActiveSocketBase::at | ( | ) | const [inline, virtual] |
This call is not supported on sockets.
Reimplemented from QIODevice. This will always return 0.
Reimplemented from QIODevice.
Definition at line 514 of file ksocketbase.h.
virtual bool KNetwork::KActiveSocketBase::atEnd | ( | ) | const [inline, virtual] |
This call is not supported on sockets.
Reimplemented from QIODevice. This will always return true.
Reimplemented from QIODevice.
Definition at line 528 of file ksocketbase.h.
virtual bool KNetwork::KActiveSocketBase::bind | ( | const KResolverEntry & | address | ) | [pure virtual] |
Binds this socket to the given address.
The socket will be constructed with the address family, socket type and protocol as those given in the address
object.
- Parameters:
-
address the address to bind to
- Returns:
- true if the binding was successful, false otherwise
Implemented in KNetwork::KClientSocketBase, KNetwork::KDatagramSocket, KNetwork::KSocketDevice, KNetwork::KSocksSocketDevice, and KNetwork::KStreamSocket.
virtual Q_LONG KNetwork::KActiveSocketBase::bytesAvailable | ( | ) | const [pure virtual] |
Returns the number of bytes available for reading without blocking.
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, and KNetwork::KSocketDevice.
virtual bool KNetwork::KActiveSocketBase::connect | ( | const KResolverEntry & | address | ) | [pure virtual] |
Connect to a remote host.
This will make this socket try to connect to the remote host. If the socket is not yet created, it will be created using the address family, socket type and protocol specified in the address
object.
If this function returns with error InProgress, calling it again with the same address after a time will cause it to test if the connection has succeeded in the mean time.
- Parameters:
-
address the address to connect to
- Returns:
- true if the connection was successful or has been successfully queued; false if an error occurred.
Implemented in KNetwork::KClientSocketBase, KNetwork::KDatagramSocket, KNetwork::KHttpProxySocketDevice, KNetwork::KMulticastSocketImpl, KNetwork::KSocketDevice, KNetwork::KSocksSocketDevice, and KNetwork::KStreamSocket.
virtual bool KNetwork::KActiveSocketBase::disconnect | ( | ) | [pure virtual] |
Disconnects this socket from a connection, if possible.
If this socket was connected to an endpoint, the connection is severed, but the socket is not closed. If the socket wasn't connected, this function does nothing.
If the socket hadn't yet been created, this function does nothing either.
Not all socket types can disconnect. Most notably, only connectionless datagram protocols such as UDP support this operation.
- Returns:
- true if the socket is now disconnected or false on error.
Implemented in KNetwork::KClientSocketBase, and KNetwork::KSocketDevice.
int KActiveSocketBase::getch | ( | ) | [virtual] |
Reads one character from the socket.
Reimplementation from QIODevice. See QIODevice::getch for more information.
Reimplemented from QIODevice.
Definition at line 291 of file ksocketbase.cpp.
virtual KSocketAddress KNetwork::KActiveSocketBase::localAddress | ( | ) | const [pure virtual] |
Returns this socket's local address.
Implemented in KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
virtual Q_LONG KNetwork::KActiveSocketBase::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [pure virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Peeks the data in the socket and the source address.
This call will allow you to peek the data to be received without actually receiving it -- that is, it will be available for further peekings and for the next read call.
- Parameters:
-
data where to write the peeked data to maxlen the maximum number of bytes to peek from the address of the sender will be stored here
- Returns:
- the actual number of bytes copied into
data
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
virtual Q_LONG KNetwork::KActiveSocketBase::peekBlock | ( | char * | data, | |
Q_ULONG | maxlen | |||
) | [pure virtual] |
Peeks the data in the socket.
This call will allow you to peek the data to be received without actually receiving it -- that is, it will be available for further peekings and for the next read call.
- Parameters:
-
data where to write the peeked data to maxlen the maximum number of bytes to peek
- Returns:
- the actual number of bytes copied into
data
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
virtual KSocketAddress KNetwork::KActiveSocketBase::peerAddress | ( | ) | const [pure virtual] |
Return this socket's peer address, if we are connected.
If the address cannot be retrieved, the returned object will contain an invalid address.
Implemented in KNetwork::KClientSocketBase, KNetwork::KHttpProxySocketDevice, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
int KActiveSocketBase::putch | ( | int | ch | ) | [virtual] |
Writes one character to the socket.
Reimplementation from QIODevice. See QIODevice::putch for more information.
Reimplemented from QIODevice.
Definition at line 300 of file ksocketbase.cpp.
virtual Q_LONG KNetwork::KActiveSocketBase::readBlock | ( | char * | data, | |
Q_ULONG | maxlen, | |||
KSocketAddress & | from | |||
) | [pure virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Receives data and the source address.
This call will read data in the socket and will also place the sender's address in from
object.
- Parameters:
-
data where to write the read data to maxlen the maximum number of bytes to read from the address of the sender will be stored here
- Returns:
- the actual number of bytes read
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
virtual Q_LONG KNetwork::KActiveSocketBase::readBlock | ( | char * | data, | |
Q_ULONG | len | |||
) | [pure virtual] |
Reads data from the socket.
Reimplemented from QIODevice. See QIODevice::readBlock for more information.
Reimplemented from QIODevice.
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
void KActiveSocketBase::resetError | ( | ) | [protected] |
Resets the socket error code and the I/O Device's status.
Definition at line 315 of file ksocketbase.cpp.
void KActiveSocketBase::setError | ( | int | status, | |
SocketError | error | |||
) | [protected] |
Sets the socket's error code and the I/O Device's status.
- Parameters:
-
status the I/O Device status error the error code
Definition at line 309 of file ksocketbase.cpp.
virtual Offset KNetwork::KActiveSocketBase::size | ( | ) | const [inline, virtual] |
This call is not supported on sockets.
Reimplemented from QIODevice. This will always return 0.
Reimplemented from QIODevice.
Definition at line 507 of file ksocketbase.h.
virtual int KNetwork::KActiveSocketBase::ungetch | ( | int | ) | [inline, virtual] |
This call is not supported on sockets.
Reimplemented from QIODevice. This will always return -1;
Reimplemented from QIODevice.
Definition at line 635 of file ksocketbase.h.
virtual Q_LONG KNetwork::KActiveSocketBase::waitForMore | ( | int | msecs, | |
bool * | timeout = 0L | |||
) | [pure virtual] |
Waits up to msecs
for more data to be available on this socket.
If msecs is -1, this call will block indefinetely until more data is indeed available; if it's 0, this function returns immediately.
If timeout
is not NULL, this function will set it to indicate if a timeout occurred.
- Returns:
- the number of bytes available
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, and KNetwork::KSocketDevice.
virtual Q_LONG KNetwork::KActiveSocketBase::writeBlock | ( | const char * | data, | |
Q_ULONG | len, | |||
const KSocketAddress & | to | |||
) | [pure virtual] |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Writes the given data to the destination address.
Note that not all socket connections allow sending data to different addresses than the one the socket is connected to.
- Parameters:
-
data the data to write len the length of the data to the address to send to
- Returns:
- the number of bytes actually sent
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KDatagramSocket, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
virtual Q_LONG KNetwork::KActiveSocketBase::writeBlock | ( | const char * | data, | |
Q_ULONG | len | |||
) | [pure virtual] |
Writes the given data to the socket.
Reimplemented from QIODevice. See QIODevice::writeBlock for more information.
Reimplemented from QIODevice.
Implemented in KNetwork::KBufferedSocket, KNetwork::KClientSocketBase, KNetwork::KSocketDevice, and KNetwork::KSocksSocketDevice.
The documentation for this class was generated from the following files: