kdeui
KDoubleNumInput Class Reference
An input control for real numbers, consisting of a spinbox and a slider. More...
#include <knuminput.h>
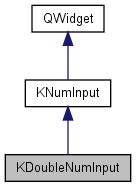
Public Slots | |
void | setPrefix (const QString &prefix) |
void | setReferencePoint (double ref) |
void | setRelativeValue (double) |
void | setSuffix (const QString &suffix) |
void | setValue (double) |
Signals | |
void | relativeValueChanged (double) |
void | valueChanged (double) |
Public Member Functions | |
virtual bool | eventFilter (QObject *, QEvent *) |
KDoubleNumInput (KNumInput *below, double lower, double upper, double value, double step=0.02, int precision=2, QWidget *parent=0, const char *name=0) | |
KDoubleNumInput (KNumInput *below, double value, QWidget *parent=0, const char *name=0) KDE_DEPRECATED | |
KDoubleNumInput (double lower, double upper, double value, double step=0.01, int precision=2, QWidget *parent=0, const char *name=0) | |
KDoubleNumInput (double value, QWidget *parent=0, const char *name=0) KDE_DEPRECATED | |
KDoubleNumInput (QWidget *parent=0, const char *name=0) | |
double | maxValue () const |
virtual QSize | minimumSizeHint () const |
double | minValue () const |
int | precision () const |
QString | prefix () const |
double | referencePoint () const |
double | relativeValue () const |
virtual void | setLabel (const QString &label, int a=AlignLeft|AlignTop) |
void | setMaxValue (double max) |
void | setMinValue (double min) |
void | setPrecision (int precision) |
void | setRange (double min, double max, double step=1, bool slider=true) |
void | setSpecialValueText (const QString &text) |
QString | specialValueText () const |
QString | suffix () const |
double | value () const |
virtual | ~KDoubleNumInput () |
Protected Member Functions | |
virtual void | doLayout () |
virtual void | resetEditBox () |
void | resizeEvent (QResizeEvent *) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KDoubleLine * | edit |
double | m_lower |
bool | m_range |
QSize | m_sizeEdit |
double | m_step |
double | m_upper |
Properties | |
double | maxValue |
double | minValue |
int | precision |
QString | prefix |
double | referencePoint |
double | relativeValue |
QString | specialValueText |
QString | suffix |
double | value |
Detailed Description
An input control for real numbers, consisting of a spinbox and a slider.KDoubleNumInput combines a QSpinBox and optionally a QSlider with a label to make an easy to use control for setting some float parameter. This is especially nice for configuration dialogs, which can have many such combinated controls.
The slider is created only when the user specifies a range for the control using the setRange function with the slider parameter set to "true".
A special feature of KDoubleNumInput, designed specifically for the situation when there are several instances in a column, is that you can specify what portion of the control is taken by the QSpinBox (the remaining portion is used by the slider). This makes it very simple to have all the sliders in a column be the same size.
It uses the KDoubleValidator validator class. KDoubleNumInput enforces the value to be in the given range, but see the class documentation of KDoubleSpinBox for the tricky interrelationship of precision and values. All of what is said there applies here, too.
- See also:
- KIntNumInput, KDoubleSpinBox
Definition at line 432 of file knuminput.h.
Constructor & Destructor Documentation
KDoubleNumInput::KDoubleNumInput | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 | |||
) |
Constructs an input control for double values with initial value 0.00.
Definition at line 544 of file knuminput.cpp.
KDoubleNumInput::KDoubleNumInput | ( | double | value, | |
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
- Deprecated:
- (value is rounded to a multiple of 1/100) Constructor
- Parameters:
-
value initial value for the control parent parent QWidget name internal name for this widget
Definition at line 567 of file knuminput.cpp.
KDoubleNumInput::KDoubleNumInput | ( | double | lower, | |
double | upper, | |||
double | value, | |||
double | step = 0.01 , |
|||
int | precision = 2 , |
|||
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
Constructor.
- Parameters:
-
lower lower boundary value upper upper boundary value value initial value for the control step step size to use for up/down arrow clicks precision number of digits after the decimal point parent parent QWidget name internal name for this widget
- Since:
- 3.1
Definition at line 550 of file knuminput.cpp.
KDoubleNumInput::~KDoubleNumInput | ( | ) | [virtual] |
KDoubleNumInput::KDoubleNumInput | ( | KNumInput * | below, | |
double | value, | |||
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
- Deprecated:
- (rounds
value
to a multiple of 1/100) Constructor
- Parameters:
-
below value initial value for the control parent parent QWidget name internal name for this widget
Definition at line 573 of file knuminput.cpp.
KDoubleNumInput::KDoubleNumInput | ( | KNumInput * | below, | |
double | lower, | |||
double | upper, | |||
double | value, | |||
double | step = 0.02 , |
|||
int | precision = 2 , |
|||
QWidget * | parent = 0 , |
|||
const char * | name = 0 | |||
) |
Constructor.
the difference here is the "below" parameter. It tells this instance that it is visually put below some other KNumInput widget. Note that these two KNumInput's need not to have the same parent widget or be in the same layout group. The effect is that it'll adjust it's layout in correspondence with the layout of the other KNumInput's (you can build an arbitrary long chain).
- Parameters:
-
below append KDoubleNumInput to the KDoubleNumInput chain lower lower boundary value upper upper boundary value value initial value for the control step step size to use for up/down arrow clicks precision number of digits after the decimal point parent parent QWidget name internal name for this widget
- Since:
- 3.1
Definition at line 558 of file knuminput.cpp.
Member Function Documentation
void KDoubleNumInput::doLayout | ( | ) | [protected, virtual] |
You need to overwrite this method and implement your layout calculations there.
See KIntNumInput::doLayout and KDoubleNumInput::doLayout implementation for details.
Implements KNumInput.
Definition at line 720 of file knuminput.cpp.
Definition at line 587 of file knuminput.cpp.
double KDoubleNumInput::maxValue | ( | ) | const |
- Returns:
- the maximum value.
QSize KDoubleNumInput::minimumSizeHint | ( | void | ) | const [virtual] |
double KDoubleNumInput::minValue | ( | ) | const |
- Returns:
- the minimum value.
int KDoubleNumInput::precision | ( | ) | const |
QString KDoubleNumInput::prefix | ( | ) | const |
double KDoubleNumInput::referencePoint | ( | ) | const |
- Returns:
- the reference point for relativeValue calculation
- Since:
- 3.1
double KDoubleNumInput::relativeValue | ( | ) | const |
- Returns:
- the current value in units of referencePoint.
- Since:
- 3.1
void KDoubleNumInput::relativeValueChanged | ( | double | ) | [signal] |
This is an overloaded member function, provided for convenience.
It essentially behaves like the above function.
Contains the value in units of referencePoint.
- Since:
- 3.1
void KDoubleNumInput::resetEditBox | ( | ) | [protected, virtual] |
Definition at line 591 of file knuminput.cpp.
void KDoubleNumInput::resizeEvent | ( | QResizeEvent * | e | ) | [protected] |
void KDoubleNumInput::setLabel | ( | const QString & | label, | |
int | a = AlignLeft | AlignTop | |||
) | [virtual] |
Sets the text and alignment of the main description label.
- Parameters:
-
label The text of the label. Use QString::null to remove an existing one. a one of AlignLeft
,AlignHCenter
, YAlignRight andAlignTop
,AlignVCenter
,AlignBottom
. default isAlignLeft
|AlignTop
.
AlignTop
The label is placed above the edit/sliderAlignVCenter
The label is placed left beside the editAlignBottom
The label is placed below the edit/slider
Reimplemented from KNumInput.
Definition at line 873 of file knuminput.cpp.
void KDoubleNumInput::setMaxValue | ( | double | max | ) |
void KDoubleNumInput::setMinValue | ( | double | min | ) |
void KDoubleNumInput::setPrecision | ( | int | precision | ) |
void KDoubleNumInput::setPrefix | ( | const QString & | prefix | ) | [slot] |
Sets the prefix to be displayed to prefix
.
Use QString::null to disable this feature. Note that the prefix is attached to the value without any spacing.
- See also:
- setPrefix()
Definition at line 846 of file knuminput.cpp.
void KDoubleNumInput::setRange | ( | double | min, | |
double | max, | |||
double | step = 1 , |
|||
bool | slider = true | |||
) |
- Parameters:
-
min minimum value max maximum value step step size for the QSlider slider whether the slider is created or not
Definition at line 746 of file knuminput.cpp.
void KDoubleNumInput::setReferencePoint | ( | double | ref | ) | [slot] |
Sets the reference Point to ref
.
It ref
== 0, emitting of relativeValueChanged is blocked and relativeValue just returns 0.
- Since:
- 3.1
Definition at line 739 of file knuminput.cpp.
void KDoubleNumInput::setRelativeValue | ( | double | r | ) | [slot] |
void KDoubleNumInput::setSpecialValueText | ( | const QString & | text | ) |
Sets the special value text.
If set, the spin box will display this text instead of the numeric value whenever the current value is equal to minVal(). Typically this is used for indicating that the choice has a special (default) meaning.
Definition at line 865 of file knuminput.cpp.
void KDoubleNumInput::setSuffix | ( | const QString & | suffix | ) | [slot] |
Sets the suffix to be displayed to suffix
.
Use QString::null to disable this feature. Note that the suffix is attached to the value without any spacing. So if you prefer to display a space separator, set suffix to something like " cm".
- See also:
- setSuffix()
Definition at line 839 of file knuminput.cpp.
void KDoubleNumInput::setValue | ( | double | val | ) | [slot] |
QString KDoubleNumInput::specialValueText | ( | ) | const [inline] |
- Returns:
- the string displayed for a special value.
- See also:
- setSpecialValueText()
Definition at line 547 of file knuminput.h.
QString KDoubleNumInput::suffix | ( | ) | const |
double KDoubleNumInput::value | ( | ) | const |
- Returns:
- the current value.
void KDoubleNumInput::valueChanged | ( | double | ) | [signal] |
Emitted every time the value changes (by calling setValue() or by user interaction).
void KDoubleNumInput::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
KDoubleLine* KDoubleNumInput::edit [protected] |
Definition at line 665 of file knuminput.h.
double KDoubleNumInput::m_lower [protected] |
Definition at line 668 of file knuminput.h.
bool KDoubleNumInput::m_range [protected] |
Definition at line 667 of file knuminput.h.
QSize KDoubleNumInput::m_sizeEdit [protected] |
Definition at line 671 of file knuminput.h.
double KDoubleNumInput::m_step [protected] |
Definition at line 668 of file knuminput.h.
double KDoubleNumInput::m_upper [protected] |
Definition at line 668 of file knuminput.h.
Property Documentation
double KDoubleNumInput::maxValue [read, write] |
Definition at line 437 of file knuminput.h.
double KDoubleNumInput::minValue [read, write] |
Definition at line 436 of file knuminput.h.
int KDoubleNumInput::precision [read, write] |
Definition at line 441 of file knuminput.h.
QString KDoubleNumInput::prefix [read, write] |
Definition at line 439 of file knuminput.h.
double KDoubleNumInput::referencePoint [read, write] |
Definition at line 442 of file knuminput.h.
double KDoubleNumInput::relativeValue [read, write] |
Definition at line 443 of file knuminput.h.
QString KDoubleNumInput::specialValueText [read, write] |
Definition at line 440 of file knuminput.h.
QString KDoubleNumInput::suffix [read, write] |
Definition at line 438 of file knuminput.h.
double KDoubleNumInput::value [read, write] |
Definition at line 435 of file knuminput.h.
The documentation for this class was generated from the following files: