kdeui
KIntNumInput Class Reference
An input widget for integer numbers, consisting of a spinbox and a slider. More...
#include <knuminput.h>
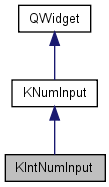
Public Slots | |
void | setEditFocus (bool mark=true) |
void | setPrefix (const QString &prefix) |
void | setReferencePoint (int) |
void | setRelativeValue (double) |
void | setSuffix (const QString &suffix) |
void | setValue (int) |
Signals | |
void | relativeValueChanged (double) |
void | valueChanged (int) |
Public Member Functions | |
KIntNumInput (KNumInput *below, int value, QWidget *parent=0, int base=10, const char *name=0) | |
KIntNumInput (int value, QWidget *parent=0, int base=10, const char *name=0) | |
KIntNumInput (QWidget *parent=0, const char *name=0) | |
int | maxValue () const |
virtual QSize | minimumSizeHint () const |
int | minValue () const |
QString | prefix () const |
int | referencePoint () const |
double | relativeValue () const |
virtual void | setLabel (const QString &label, int a=AlignLeft|AlignTop) |
void | setMaxValue (int max) |
void | setMinValue (int min) |
void | setRange (int min, int max, int step=1, bool slider=true) |
void | setSpecialValueText (const QString &text) |
QString | specialValueText () const |
QString | suffix () const |
int | value () const |
virtual | ~KIntNumInput () |
Protected Member Functions | |
virtual void | doLayout () |
void | resizeEvent (QResizeEvent *) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
QSize | m_sizeSpin |
KIntSpinBox * | m_spin |
Properties | |
int | maxValue |
int | minValue |
QString | prefix |
int | referencePoint |
double | relativeValue |
QString | specialValueText |
QString | suffix |
int | value |
Detailed Description
An input widget for integer numbers, consisting of a spinbox and a slider.KIntNumInput combines a QSpinBox and optionally a QSlider with a label to make an easy to use control for setting some integer parameter. This is especially nice for configuration dialogs, which can have many such combinated controls.
The slider is created only when the user specifies a range for the control using the setRange function with the slider parameter set to "true".
A special feature of KIntNumInput, designed specifically for the situation when there are several KIntNumInputs in a column, is that you can specify what portion of the control is taken by the QSpinBox (the remaining portion is used by the slider). This makes it very simple to have all the sliders in a column be the same size.
It uses KIntValidator validator class. KIntNumInput enforces the value to be in the given range, and can display it in any base between 2 and 36.
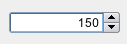
KDE Int Number Input Spinbox
- Version:
- $Id$
Definition at line 187 of file knuminput.h.
Constructor & Destructor Documentation
KIntNumInput::KIntNumInput | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 | |||
) |
Constructs an input control for integer values with base 10 and initial value 0.
Definition at line 273 of file knuminput.cpp.
KIntNumInput::KIntNumInput | ( | int | value, | |
QWidget * | parent = 0 , |
|||
int | base = 10 , |
|||
const char * | name = 0 | |||
) |
Constructor It constructs a QSpinBox that allows the input of integer numbers in the range of -INT_MAX to +INT_MAX.
To set a descriptive label, use setLabel(). To enforce the value being in a range and optionally to attach a slider to it, use setRange().
- Parameters:
-
value initial value for the control base numeric base used for display parent parent QWidget name internal name for this widget
Definition at line 279 of file knuminput.cpp.
KIntNumInput::KIntNumInput | ( | KNumInput * | below, | |
int | value, | |||
QWidget * | parent = 0 , |
|||
int | base = 10 , |
|||
const char * | name = 0 | |||
) |
Constructor.
the difference to the one above is the "below" parameter. It tells this instance that it is visually put below some other KNumInput widget. Note that these two KNumInput's need not to have the same parent widget or be in the same layout group. The effect is that it'll adjust it's layout in correspondence with the layout of the other KNumInput's (you can build an arbitrary long chain).
- Parameters:
-
below append KIntNumInput to the KNumInput chain value initial value for the control base numeric base used for display parent parent QWidget name internal name for this widget
Definition at line 266 of file knuminput.cpp.
KIntNumInput::~KIntNumInput | ( | ) | [virtual] |
Member Function Documentation
void KIntNumInput::doLayout | ( | ) | [protected, virtual] |
You need to overwrite this method and implement your layout calculations there.
See KIntNumInput::doLayout and KDoubleNumInput::doLayout implementation for details.
Implements KNumInput.
Definition at line 440 of file knuminput.cpp.
int KIntNumInput::maxValue | ( | ) | const |
- Returns:
- the maximum value.
QSize KIntNumInput::minimumSizeHint | ( | void | ) | const [virtual] |
This method returns the minimum size necessary to display the control.
The minimum size is enough to show all the labels in the current font (font change may invalidate the return value).
- Returns:
- the minimum size necessary to show the control
Reimplemented from QWidget.
Definition at line 415 of file knuminput.cpp.
int KIntNumInput::minValue | ( | ) | const |
- Returns:
- the minimum value.
QString KIntNumInput::prefix | ( | ) | const |
int KIntNumInput::referencePoint | ( | ) | const |
- Returns:
- the current reference point
- Since:
- 3.1
double KIntNumInput::relativeValue | ( | ) | const |
- Returns:
- the curent value in units of the referencePoint.
- Since:
- 3.1
void KIntNumInput::relativeValueChanged | ( | double | ) | [signal] |
Emitted whenever valueChanged is.
Contains the change relative to the referencePoint.
- Since:
- 3.1
void KIntNumInput::resizeEvent | ( | QResizeEvent * | e | ) | [protected] |
void KIntNumInput::setEditFocus | ( | bool | mark = true |
) | [slot] |
sets focus to the edit widget and marks all text in if mark == true
Definition at line 410 of file knuminput.cpp.
void KIntNumInput::setLabel | ( | const QString & | label, | |
int | a = AlignLeft | AlignTop | |||
) | [virtual] |
Sets the text and alignment of the main description label.
- Parameters:
-
label The text of the label. Use QString::null to remove an existing one. a one of AlignLeft
,AlignHCenter
, YAlignRight andAlignTop
,AlignVCenter
,AlignBottom
. default isAlignLeft
|AlignTop
.
AlignTop
The label is placed above the edit/sliderAlignVCenter
The label is placed left beside the editAlignBottom
The label is placed below the edit/slider
Reimplemented from KNumInput.
Definition at line 523 of file knuminput.cpp.
void KIntNumInput::setMaxValue | ( | int | max | ) |
void KIntNumInput::setMinValue | ( | int | min | ) |
void KIntNumInput::setPrefix | ( | const QString & | prefix | ) | [slot] |
Sets the prefix to prefix
.
Use QString::null to disable this feature. Formatting has to be provided (see above).
- See also:
- QSpinBox::setPrefix(), setSuffix()
Definition at line 398 of file knuminput.cpp.
void KIntNumInput::setRange | ( | int | min, | |
int | max, | |||
int | step = 1 , |
|||
bool | slider = true | |||
) |
- Parameters:
-
min minimum value max maximum value step step size for the QSlider slider whether the slider is created or not
Definition at line 327 of file knuminput.cpp.
void KIntNumInput::setReferencePoint | ( | int | ref | ) | [slot] |
void KIntNumInput::setRelativeValue | ( | double | r | ) | [slot] |
Sets the value in units of the referencePoint.
- Since:
- 3.1
Definition at line 495 of file knuminput.cpp.
void KIntNumInput::setSpecialValueText | ( | const QString & | text | ) |
Sets the special value text.
If set, the SpinBox will display this text instead of the numeric value whenever the current value is equal to minVal(). Typically this is used for indicating that the choice has a special (default) meaning.
Definition at line 512 of file knuminput.cpp.
void KIntNumInput::setSuffix | ( | const QString & | suffix | ) | [slot] |
Sets the suffix to suffix
.
Use QString::null to disable this feature. Formatting has to be provided (e.g. a space separator between the prepended value
and the suffix's text has to be provided as the first character in the suffix).
- See also:
- QSpinBox::setSuffix(), setPrefix()
Definition at line 386 of file knuminput.cpp.
void KIntNumInput::setValue | ( | int | val | ) | [slot] |
QString KIntNumInput::specialValueText | ( | ) | const |
QString KIntNumInput::suffix | ( | ) | const |
int KIntNumInput::value | ( | ) | const |
- Returns:
- the current value.
void KIntNumInput::valueChanged | ( | int | ) | [signal] |
Emitted every time the value changes (by calling setValue() or by user interaction).
void KIntNumInput::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
QSize KIntNumInput::m_sizeSpin [protected] |
Definition at line 388 of file knuminput.h.
KIntSpinBox* KIntNumInput::m_spin [protected] |
Definition at line 387 of file knuminput.h.
Property Documentation
int KIntNumInput::maxValue [read, write] |
Definition at line 192 of file knuminput.h.
int KIntNumInput::minValue [read, write] |
Definition at line 191 of file knuminput.h.
QString KIntNumInput::prefix [read, write] |
Definition at line 196 of file knuminput.h.
int KIntNumInput::referencePoint [read, write] |
Definition at line 193 of file knuminput.h.
double KIntNumInput::relativeValue [read, write] |
Definition at line 194 of file knuminput.h.
QString KIntNumInput::specialValueText [read, write] |
Definition at line 197 of file knuminput.h.
QString KIntNumInput::suffix [read, write] |
Definition at line 195 of file knuminput.h.
int KIntNumInput::value [read, write] |
Definition at line 190 of file knuminput.h.
The documentation for this class was generated from the following files: