kdeui
KFontChooser Class Reference
A font selection widget. More...
#include <kfontdialog.h>
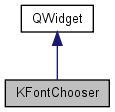
Public Types | |
enum | FontColumn { FamilyList = 0x01, StyleList = 0x02, SizeList = 0x04 } |
enum | FontDiff { FontDiffFamily = 0x01, FontDiffStyle = 0x02, FontDiffSize = 0x04 } |
enum | FontListCriteria { FixedWidthFonts = 0x01, ScalableFonts = 0x02, SmoothScalableFonts = 0x04 } |
Signals | |
void | fontSelected (const QFont &font) |
Public Member Functions | |
QColor | backgroundColor () const |
QColor | color () const |
void | enableColumn (int column, bool state) |
QFont | font () const |
int | fontDiffFlags () |
KFontChooser (QWidget *parent=0L, const char *name=0L, bool onlyFixed=false, const QStringList &fontList=QStringList(), bool makeFrame=true, int visibleListSize=8, bool diff=false, QButton::ToggleState *sizeIsRelativeState=0L) | |
QString | sampleText () const |
void | setBackgroundColor (const QColor &col) |
void | setColor (const QColor &col) |
void | setFont (const QFont &font, bool onlyFixed=false) |
void | setSampleBoxVisible (bool visible) |
void | setSampleText (const QString &text) |
void | setSizeIsRelative (QButton::ToggleState relative) |
virtual QSize | sizeHint (void) const |
QButton::ToggleState | sizeIsRelative () const |
virtual | ~KFontChooser () |
Static Public Member Functions | |
static void | getFontList (QStringList &list, uint fontListCriteria) |
static QString | getXLFD (const QFont &theFont) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QFont | font |
Detailed Description
A font selection widget.While KFontChooser as an ordinary widget can be embedded in custom dialogs and therefore is very flexible, in most cases it is preferable to use the convenience functions in KFontDialog.
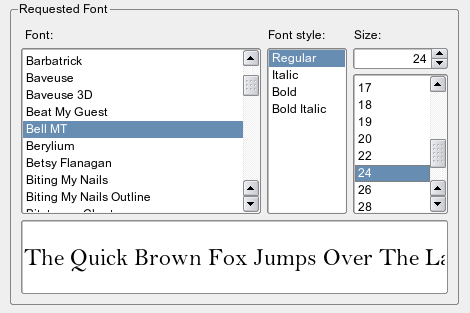
KDE Font Chooser
- Version:
- Id
- kfontdialog.h 465272 2005-09-29 09:47:40Z mueller
Definition at line 54 of file kfontdialog.h.
Member Enumeration Documentation
FamilyList
- Identifies the family (leftmost) list.StyleList
- Identifies the style (center) list.SizeList
- Identifies the size (rightmost) list.
Definition at line 65 of file kfontdialog.h.
FontDiffFamily
- Identifies a requested change in the font family.FontDiffStyle
- Identifies a requested change in the font style.FontDiffSize
- Identifies a requested change in the font size.
Definition at line 72 of file kfontdialog.h.
The selection criteria for the font families shown in the dialog.
FixedWidthFont
when included only fixed-width fonts are returned. The fonts where the width of every character is equal.ScalableFont
when included only scalable fonts are returned; certain configurations allow bitmap fonts to remain unscaled and thus these fonts have limited number of sizes.SmoothScalableFont
when included only return smooth scalable fonts. this will return only non-bitmap fonts which are scalable to any size requested. Setting this option to true will mean the "scalable" flag is irrelavant.
Definition at line 241 of file kfontdialog.h.
Constructor & Destructor Documentation
KFontChooser::KFontChooser | ( | QWidget * | parent = 0L , |
|
const char * | name = 0L , |
|||
bool | onlyFixed = false , |
|||
const QStringList & | fontList = QStringList() , |
|||
bool | makeFrame = true , |
|||
int | visibleListSize = 8 , |
|||
bool | diff = false , |
|||
QButton::ToggleState * | sizeIsRelativeState = 0L | |||
) |
Constructs a font picker widget.
It normally comes up with all font families present on the system; the getFont method below does allow some more fine-tuning of the selection of fonts that will be displayed in the dialog.
Consider the following code snippet;
QStringList list; KFontChooser::getFontList(list,SmoothScalableFonts); KFontChooser chooseFont = new KFontChooser(0, "FontList", false, list);
The above creates a font chooser dialog with only SmoothScaleble fonts.
- Parameters:
-
parent The parent widget. name The widget name. onlyFixed Only display fonts which have fixed-width character sizes. fontList A list of fonts to display, in XLFD format. If no list is formatted, the internal KDE font list is used. If that has not been created, X is queried, and all fonts available on the system are displayed. diff Display the difference version dialog. See KFontDialog::getFontDiff(). makeFrame Draws a frame with titles around the contents. visibleListSize The minimum number of visible entries in the fontlists. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState, user choice may be retrieved by calling sizeIsRelative().
Definition at line 92 of file kfontdialog.cpp.
KFontChooser::~KFontChooser | ( | ) | [virtual] |
Member Function Documentation
QColor KFontChooser::backgroundColor | ( | ) | const |
- Returns:
- The background color currently used in the preview (default: the base color of the active colorgroup)
Definition at line 383 of file kfontdialog.cpp.
QColor KFontChooser::color | ( | ) | const |
- Returns:
- The color currently used in the preview (default: the text color of the active color group)
Definition at line 370 of file kfontdialog.cpp.
void KFontChooser::enableColumn | ( | int | column, | |
bool | state | |||
) |
Enables or disable a font column in the chooser.
Use this function if your application does not need or supports all font properties.
- Parameters:
-
column Specify the columns. An or'ed combination of FamilyList
,StyleList
andSizeList
is possible.state If false
the columns are disabled.
Definition at line 412 of file kfontdialog.cpp.
QFont KFontChooser::font | ( | ) | const [inline] |
- Returns:
- The currently selected font in the chooser.
Reimplemented from QWidget.
Definition at line 150 of file kfontdialog.h.
int KFontChooser::fontDiffFlags | ( | ) |
- Returns:
- The bitmask corresponding to the attributes the user wishes to change.
Definition at line 446 of file kfontdialog.cpp.
void KFontChooser::fontSelected | ( | const QFont & | font | ) | [signal] |
Emitted whenever the selected font changes.
void KFontChooser::getFontList | ( | QStringList & | list, | |
uint | fontListCriteria | |||
) | [static] |
Creates a list of font strings.
- Parameters:
-
list The list is returned here. fontListCriteria should contain all the restrictions for font selection as OR-ed values
- See also:
- KFontChooser::FontListCriteria for the individual values
Definition at line 641 of file kfontdialog.cpp.
Converts a QFont into the corresponding X Logical Font Description (XLFD).
- Parameters:
-
theFont The font to convert.
- Returns:
- A string representing the given font in XLFD format.
Definition at line 227 of file kfontdialog.h.
QString KFontChooser::sampleText | ( | ) | const [inline] |
- Returns:
- The current text in the sample text input area.
Definition at line 192 of file kfontdialog.h.
void KFontChooser::setBackgroundColor | ( | const QColor & | col | ) |
void KFontChooser::setColor | ( | const QColor & | col | ) |
void KFontChooser::setFont | ( | const QFont & | font, | |
bool | onlyFixed = false | |||
) |
Sets the currently selected font in the chooser.
- Parameters:
-
font The font to select. onlyFixed Readjust the font list to display only fixed width fonts if true
, or vice-versa.
Definition at line 429 of file kfontdialog.cpp.
void KFontChooser::setSampleBoxVisible | ( | bool | visible | ) | [inline] |
Shows or hides the sample text box.
- Parameters:
-
visible Set it to true to show the box, to false to hide it.
- Since:
- 3.5
Definition at line 215 of file kfontdialog.h.
void KFontChooser::setSampleText | ( | const QString & | text | ) | [inline] |
Sets the sample text.
Normally you should not change this text, but it can be better to do this if the default text is too large for the edit area when using the default font of your application.
- Parameters:
-
text The new sample text. The current will be removed.
Definition at line 204 of file kfontdialog.h.
void KFontChooser::setSizeIsRelative | ( | QButton::ToggleState | relative | ) |
Sets the state of the checkbox indicating whether the font size is to be interpreted as relative size.
NOTE: If parameter sizeIsRelative was not set in the constructor of the widget this setting will be ignored.
Definition at line 388 of file kfontdialog.cpp.
QSize KFontChooser::sizeHint | ( | void | ) | const [virtual] |
Reimplemented for internal reasons.
Reimplemented from QWidget.
Definition at line 406 of file kfontdialog.cpp.
QButton::ToggleState KFontChooser::sizeIsRelative | ( | ) | const |
- Returns:
- Whether the font size is to be interpreted as relative size (default: QButton:Off)
Definition at line 399 of file kfontdialog.cpp.
void KFontChooser::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 795 of file kfontdialog.cpp.
Property Documentation
QFont KFontChooser::font [read, write] |
Definition at line 57 of file kfontdialog.h.
The documentation for this class was generated from the following files: