kdeui
KFontDialog Class Reference
A font selection dialog. More...
#include <kfontdialog.h>
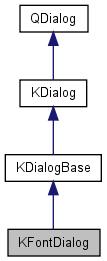
Signals | |
void | fontSelected (const QFont &font) |
Public Member Functions | |
QFont | font () const |
KFontDialog (QWidget *parent=0L, const char *name=0, bool onlyFixed=false, bool modal=false, const QStringList &fontlist=QStringList(), bool makeFrame=true, bool diff=false, QButton::ToggleState *sizeIsRelativeState=0L) | |
void | setFont (const QFont &font, bool onlyFixed=false) |
void | setSizeIsRelative (QButton::ToggleState relative) |
QButton::ToggleState | sizeIsRelative () const |
Static Public Member Functions | |
static int | getFont (QFont &theFont, bool onlyFixed=false, QWidget *parent=0L, bool makeFrame=true, QButton::ToggleState *sizeIsRelativeState=0L) |
static int | getFontAndText (QFont &theFont, QString &theString, bool onlyFixed=false, QWidget *parent=0L, bool makeFrame=true, QButton::ToggleState *sizeIsRelativeState=0L) |
static int | getFontDiff (QFont &theFont, int &diffFlags, bool onlyFixed=false, QWidget *parent=0L, bool makeFrame=true, QButton::ToggleState *sizeIsRelativeState=0L) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KFontChooser * | chooser |
Detailed Description
A font selection dialog.The KFontDialog provides a dialog for interactive font selection. It is basically a thin wrapper around the KFontChooser widget, which can also be used standalone. In most cases, the simplest use of this class is the static method KFontDialog::getFont(), which pops up the dialog, allows the user to select a font, and returns when the dialog is closed.
Example:
QFont myFont; int result = KFontDialog::getFont( myFont ); if ( result == KFontDialog::Accepted ) ...
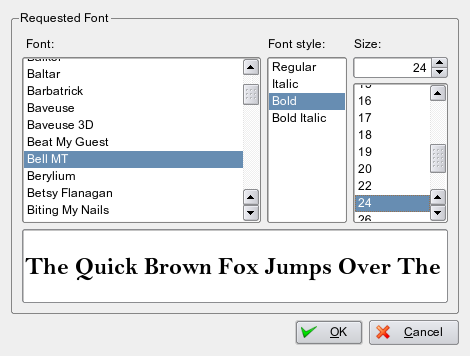
KDE Font Dialog
- Version:
- Id
- kfontdialog.h 465272 2005-09-29 09:47:40Z mueller
Definition at line 339 of file kfontdialog.h.
Constructor & Destructor Documentation
KFontDialog::KFontDialog | ( | QWidget * | parent = 0L , |
|
const char * | name = 0 , |
|||
bool | onlyFixed = false , |
|||
bool | modal = false , |
|||
const QStringList & | fontlist = QStringList() , |
|||
bool | makeFrame = true , |
|||
bool | diff = false , |
|||
QButton::ToggleState * | sizeIsRelativeState = 0L | |||
) |
Constructs a font selection dialog.
- Parameters:
-
parent The parent widget of the dialog, if any. name The name of the dialog. modal Specifies whether the dialog is modal or not. onlyFixed only display fonts which have fixed-width character sizes. fontlist a list of fonts to display, in XLFD format. If no list is formatted, the internal KDE font list is used. If that has not been created, X is queried, and all fonts available on the system are displayed. makeFrame Draws a frame with titles around the contents. diff Display the difference version dialog. See getFontDiff(). sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState, user choice may be retrieved by calling sizeIsRelative().
Definition at line 724 of file kfontdialog.cpp.
Member Function Documentation
QFont KFontDialog::font | ( | ) | const [inline] |
void KFontDialog::fontSelected | ( | const QFont & | font | ) | [signal] |
Emitted whenever the currently selected font changes.
Connect to this to monitor the font as it is selected if you are not running modal.
int KFontDialog::getFont | ( | QFont & | theFont, | |
bool | onlyFixed = false , |
|||
QWidget * | parent = 0L , |
|||
bool | makeFrame = true , |
|||
QButton::ToggleState * | sizeIsRelativeState = 0L | |||
) | [static] |
Creates a modal font dialog, lets the user choose a font, and returns when the dialog is closed.
- Parameters:
-
theFont a reference to the font to write the chosen font into. onlyFixed if true, only select from fixed-width fonts. parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). makeFrame Draws a frame with titles around the contents. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns:
- QDialog::result().
Definition at line 756 of file kfontdialog.cpp.
int KFontDialog::getFontAndText | ( | QFont & | theFont, | |
QString & | theString, | |||
bool | onlyFixed = false , |
|||
QWidget * | parent = 0L , |
|||
bool | makeFrame = true , |
|||
QButton::ToggleState * | sizeIsRelativeState = 0L | |||
) | [static] |
When you are not only interested in the font selected, but also in the example string typed in, you can call this method.
- Parameters:
-
theFont a reference to the font to write the chosen font into. theString a reference to the example text that was typed. onlyFixed if true, only select from fixed-width fonts. parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). makeFrame Draws a frame with titles around the contents. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns:
- The result of the dialog.
Definition at line 775 of file kfontdialog.cpp.
int KFontDialog::getFontDiff | ( | QFont & | theFont, | |
int & | diffFlags, | |||
bool | onlyFixed = false , |
|||
QWidget * | parent = 0L , |
|||
bool | makeFrame = true , |
|||
QButton::ToggleState * | sizeIsRelativeState = 0L | |||
) | [static] |
Creates a modal font difference dialog, lets the user choose a selection of changes that should be made to a set of fonts, and returns when the dialog is closed.
Useful for choosing slight adjustments to the font set when the user would otherwise have to manually edit a number of fonts.
- Parameters:
-
theFont a reference to the font to write the chosen font into. diffFlags a reference to the int into which the chosen difference selection bitmask should be written. Check the returned bitmask like: if ( diffFlags & KFontChooser::FontDiffFamily ) [...] if ( diffFlags & KFontChooser::FontDiffStyle ) [...] if ( diffFlags & KFontChooser::FontDiffSize ) [...]
onlyFixed if true, only select from fixed-width fonts. parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). makeFrame Draws a frame with titles around the contents. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns:
- QDialog::result().
Definition at line 737 of file kfontdialog.cpp.
void KFontDialog::setFont | ( | const QFont & | font, | |
bool | onlyFixed = false | |||
) | [inline] |
Sets the currently selected font in the dialog.
- Parameters:
-
font The font to select. onlyFixed readjust the font list to display only fixed width fonts if true, or vice-versa
Definition at line 378 of file kfontdialog.h.
void KFontDialog::setSizeIsRelative | ( | QButton::ToggleState | relative | ) | [inline] |
Sets the state of the checkbox indicating whether the font size is to be interpreted as relative size.
NOTE: If parameter sizeIsRelative was not set in the constructor of the dialog this setting will be ignored.
Definition at line 392 of file kfontdialog.h.
QButton::ToggleState KFontDialog::sizeIsRelative | ( | ) | const [inline] |
- Returns:
- Whether the font size is to be interpreted as relative size (default: false)
Definition at line 399 of file kfontdialog.h.
void KFontDialog::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
KFontChooser* KFontDialog::chooser [protected] |
Definition at line 494 of file kfontdialog.h.
The documentation for this class was generated from the following files: