kdeui
KHistoryCombo Class Reference
A combobox for offering a history and completion. More...
#include <kcombobox.h>
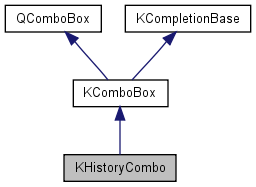
Public Slots | |
void | addToHistory (const QString &item) |
void | clearHistory () |
Signals | |
void | cleared () |
Public Member Functions | |
QStringList | historyItems () const |
KHistoryCombo (bool useCompletion, QWidget *parent=0L, const char *name=0L) | |
KHistoryCombo (QWidget *parent=0L, const char *name=0L) | |
KPixmapProvider * | pixmapProvider () const |
bool | removeFromHistory (const QString &item) |
void | reset () |
void | setHistoryItems (QStringList items, bool setCompletionList) |
void | setHistoryItems (QStringList items) |
void | setPixmapProvider (KPixmapProvider *prov) |
~KHistoryCombo () | |
Protected Member Functions | |
void | insertItems (const QStringList &items) |
virtual void | keyPressEvent (QKeyEvent *) |
bool | useCompletion () const |
virtual void | virtual_hook (int id, void *data) |
virtual void | wheelEvent (QWheelEvent *ev) |
Properties | |
QStringList | historyItems |
Detailed Description
A combobox for offering a history and completion.A combobox which implements a history like a unix shell. You can navigate through all the items by using the Up or Down arrows (configurable of course). Additionally, weighted completion is available. So you should load and save the completion list to preserve the weighting between sessions.
KHistoryCombo obeys the HISTCONTROL environment variable to determine whether duplicates in the history should be tolerated in addToHistory() or not. During construction of KHistoryCombo, duplicates will be disabled when HISTCONTROL is set to "ignoredups" or "ignoreboth". Otherwise, duplicates are enabled by default.
Definition at line 533 of file kcombobox.h.
Constructor & Destructor Documentation
KHistoryCombo::KHistoryCombo | ( | QWidget * | parent = 0L , |
|
const char * | name = 0L | |||
) |
Constructs a "read-write" combobox.
A read-only history combobox doesn't make much sense, so it is only available as read-write. Completion will be used automatically for the items in the combo.
The insertion-policy is set to NoInsertion, you have to add the items yourself via the slot addToHistory. If you want every item added, use
connect( combo, SIGNAL( activated( const QString& )), combo, SLOT( addToHistory( const QString& )));
Use QComboBox::setMaxCount() to limit the history.
parent
the parent object of this widget. name
the name of this widget.
Definition at line 348 of file kcombobox.cpp.
KHistoryCombo::KHistoryCombo | ( | bool | useCompletion, | |
QWidget * | parent = 0L , |
|||
const char * | name = 0L | |||
) |
Same as the previous constructor, but additionally has the option to specify whether you want to let KHistoryCombo handle completion or not.
If set to true
, KHistoryCombo will sync the completion to the contents of the combobox.
Definition at line 355 of file kcombobox.cpp.
KHistoryCombo::~KHistoryCombo | ( | ) |
Destructs the combo, the completion-object and the pixmap-provider.
Definition at line 386 of file kcombobox.cpp.
Member Function Documentation
void KHistoryCombo::addToHistory | ( | const QString & | item | ) | [slot] |
Adds an item to the end of the history list and to the completion list.
If maxCount() is reached, the first item of the list will be removed.
If the last inserted item is the same as item
, it will not be inserted again.
If duplicatesEnabled() is false, any equal existing item will be removed before item
is added.
Note: By using this method and not the Q and KComboBox insertItem() methods, you make sure that the combobox stays in sync with the completion. It would be annoying if completion would give an item not in the combobox, and vice versa.
Definition at line 450 of file kcombobox.cpp.
void KHistoryCombo::cleared | ( | ) | [signal] |
Emitted when the history was cleared by the entry in the popup menu.
void KHistoryCombo::clearHistory | ( | ) | [slot] |
QStringList KHistoryCombo::historyItems | ( | ) | const |
Returns the list of history items.
Empty, when this is not a read-write combobox.
- See also:
- setHistoryItems
void KHistoryCombo::insertItems | ( | const QStringList & | items | ) | [protected] |
Inserts items
into the combo, honoring pixmapProvider() Does not update the completionObject.
Note: duplicatesEnabled() is not honored here.
Called from setHistoryItems() and setPixmapProvider()
Definition at line 656 of file kcombobox.cpp.
void KHistoryCombo::keyPressEvent | ( | QKeyEvent * | e | ) | [protected, virtual] |
Handling key-events, the shortcuts to rotate the items.
Definition at line 597 of file kcombobox.cpp.
KPixmapProvider* KHistoryCombo::pixmapProvider | ( | ) | const [inline] |
- Returns:
- the current pixmap provider.
- See also:
- setPixmapProvider
KPixmapProvider
Definition at line 664 of file kcombobox.h.
bool KHistoryCombo::removeFromHistory | ( | const QString & | item | ) |
Removes all items named item
.
- Returns:
true
if at least one item was removed.
- See also:
- addToHistory
Definition at line 502 of file kcombobox.cpp.
void KHistoryCombo::reset | ( | ) | [inline] |
Resets the current position of the up/down history.
Call this when you manually call setCurrentItem() or clearEdit().
Definition at line 670 of file kcombobox.h.
void KHistoryCombo::setHistoryItems | ( | QStringList | items, | |
bool | setCompletionList | |||
) |
Inserts items
into the combobox.
items
might get truncated if it is longer than maxCount()
Set setCompletionList
to true, if you don't have a list of completions. This tells KHistoryCombo to use all the items for the completion object as well. You won't have the benefit of weighted completion though, so normally you should do something like
KConfig *config = kapp->config(); QStringList list; // load the history and completion list after creating the history combo list = config->readListEntry( "Completion list" ); combo->completionObject()->setItems( list ); list = config->readListEntry( "History list" ); combo->setHistoryItems( list ); [...] // save the history and completion list when the history combo is // destroyed list = combo->completionObject()->items() config->writeEntry( "Completion list", list ); list = combo->historyItems(); config->writeEntry( "History list", list );
Be sure to use different names for saving with KConfig if you have more than one KHistoryCombo.
Note: When setCompletionList
is true, the items are inserted into the KCompletion object with mode KCompletion::Insertion and the mode is set to KCompletion::Weighted afterwards.
- See also:
- historyItems
KComboBox::completionObject
KCompletion::setItems
KCompletion::items
Definition at line 391 of file kcombobox.cpp.
void KHistoryCombo::setHistoryItems | ( | QStringList | items | ) | [inline] |
Inserts items
into the combobox.
items
might get truncated if it is longer than maxCount()
- See also:
- historyItems
Definition at line 581 of file kcombobox.h.
void KHistoryCombo::setPixmapProvider | ( | KPixmapProvider * | prov | ) |
Sets a pixmap provider, so that items in the combobox can have a pixmap.
KPixmapProvider is just an abstract class with the one pure virtual method KPixmapProvider::pixmapFor(). This method is called whenever an item is added to the KHistoryComboBox. Implement it to return your own custom pixmaps, or use the KURLPixmapProvider from libkio, which uses KMimeType::pixmapForURL to resolve icons.
Set prov
to 0L if you want to disable pixmaps. Default no pixmaps.
- See also:
- pixmapProvider
Definition at line 638 of file kcombobox.cpp.
bool KHistoryCombo::useCompletion | ( | ) | const [inline, protected] |
void KHistoryCombo::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
void KHistoryCombo::wheelEvent | ( | QWheelEvent * | ev | ) | [protected, virtual] |
Handling wheel-events, to rotate the items.
Reimplemented from KComboBox.
Definition at line 613 of file kcombobox.cpp.
Property Documentation
QStringList KHistoryCombo::historyItems [read, write] |
Definition at line 536 of file kcombobox.h.
The documentation for this class was generated from the following files: