kdeui
KKeyChooser Class Reference
Widget for configuration of KAccel and KGlobalAccel. More...
#include <kkeydialog.h>
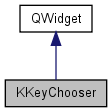
Public Types | |
enum | ActionType { Application, ApplicationGlobal, Standard, Global } |
Public Slots | |
void | allDefault () |
void | listSync () |
void | setPreferFourModifierKeys (bool preferFourModifierKeys) |
Signals | |
void | keyChange () |
Public Member Functions | |
void | commitChanges () |
bool | insert (KActionCollection *, const QString &title) |
bool | insert (KActionCollection *) |
KKeyChooser (KGlobalAccel *actions, QWidget *parent, bool bCheckAgainstStdKeys, bool bAllowLetterShortcuts, bool bAllowWinKey=false) | |
KKeyChooser (KAccel *actions, QWidget *parent, bool bCheckAgainstStdKeys, bool bAllowLetterShortcuts, bool bAllowWinKey=false) | |
KKeyChooser (KShortcutList *, QWidget *parent, ActionType type=Application, bool bAllowLetterShortcuts=true) | |
KKeyChooser (KGlobalAccel *actions, QWidget *parent) | |
KKeyChooser (KAccel *actions, QWidget *parent, bool bAllowLetterShortcuts=true) | |
KKeyChooser (KActionCollection *coll, QWidget *parent, bool bAllowLetterShortcuts=true) | |
KKeyChooser (QWidget *parent, ActionType type=Application, bool bAllowLetterShortcuts=true) | |
void | save () |
void | syncToConfig (const QString &sConfigGroup, KConfigBase *pConfig, bool bClearUnset) |
virtual | ~KKeyChooser () |
Static Public Member Functions | |
static bool | checkGlobalShortcutsConflict (const KShortcut &cut, bool warnUser, QWidget *parent) |
static bool | checkStandardShortcutsConflict (const KShortcut &cut, bool warnUser, QWidget *parent) |
Protected Types | |
enum | { NoKey = 1, DefaultKey, CustomKey } |
Protected Slots | |
void | capturedShortcut (const KShortcut &cut) |
void | slotCustomKey () |
void | slotDefaultKey () |
void | slotListItemDoubleClicked (QListViewItem *ipoQListViewItem, const QPoint &ipoQPoint, int c) |
void | slotListItemSelected (QListViewItem *item) |
void | slotNoKey () |
void | slotSettingsChanged (int) |
Protected Member Functions | |
void | _warning (const KKeySequence &seq, QString sAction, QString sTitle) |
void | buildListView (uint iList, const QString &title=QString::null) |
void | fontChange (const QFont &_font) |
void | initGUI (ActionType type, bool bAllowLetterShortcuts) |
bool | insert (KShortcutList *) |
bool | insert (KGlobalAccel *) |
bool | insert (KAccel *) |
bool | isKeyPresent (const KShortcut &cut, bool warnuser=true) |
bool | isKeyPresentLocally (const KShortcut &cut, KKeyChooserItem *ignoreItem, const QString &warnText) |
void | readGlobalKeys () |
void | setShortcut (const KShortcut &cut) |
void | updateButtons () |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_bAllowLetterShortcuts |
bool | m_bAllowWinKey |
bool | m_bPreferFourModifierKeys |
QRadioButton * | m_prbCustom |
QRadioButton * | m_prbDef |
QRadioButton * | m_prbNone |
ActionType | m_type |
Detailed Description
Widget for configuration of KAccel and KGlobalAccel.Configure dictionaries of key/action associations for KAccel and KGlobalAccel.
The class takes care of all aspects of configuration, including handling key conflicts internally. Connect to the allDefault() slot if you want to set all configurable shortcuts to their default values.
- See also:
- KKeyDialog
Definition at line 58 of file kkeydialog.h.
Member Enumeration Documentation
anonymous enum [protected] |
Definition at line 62 of file kkeydialog.h.
Constructor & Destructor Documentation
KKeyChooser::KKeyChooser | ( | QWidget * | parent, | |
ActionType | type = Application , |
|||
bool | bAllowLetterShortcuts = true | |||
) |
Constructor.
- Parameters:
-
parent the parent widget for this widget type the ActionType for this KKeyChooser bAllowLetterShortcuts Set to false if unmodified alphanumeric keys ('A', '1', etc.) are not permissible shortcuts.
Definition at line 154 of file kkeydialog.cpp.
KKeyChooser::KKeyChooser | ( | KActionCollection * | coll, | |
QWidget * | parent, | |||
bool | bAllowLetterShortcuts = true | |||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
parent parent widget coll the KActionCollection to configure bAllowLetterShortcuts Set to false if unmodified alphanumeric keys ('A', '1', etc.) are not permissible shortcuts.
Definition at line 160 of file kkeydialog.cpp.
KKeyChooser::KKeyChooser | ( | KAccel * | actions, | |
QWidget * | parent, | |||
bool | bAllowLetterShortcuts = true | |||
) |
Definition at line 167 of file kkeydialog.cpp.
KKeyChooser::KKeyChooser | ( | KGlobalAccel * | actions, | |
QWidget * | parent | |||
) |
Definition at line 174 of file kkeydialog.cpp.
KKeyChooser::KKeyChooser | ( | KShortcutList * | pList, | |
QWidget * | parent, | |||
ActionType | type = Application , |
|||
bool | bAllowLetterShortcuts = true | |||
) |
Definition at line 181 of file kkeydialog.cpp.
KKeyChooser::~KKeyChooser | ( | ) | [virtual] |
Definition at line 224 of file kkeydialog.cpp.
KKeyChooser::KKeyChooser | ( | KAccel * | actions, | |
QWidget * | parent, | |||
bool | bCheckAgainstStdKeys, | |||
bool | bAllowLetterShortcuts, | |||
bool | bAllowWinKey = false | |||
) |
KKeyChooser::KKeyChooser | ( | KGlobalAccel * | actions, | |
QWidget * | parent, | |||
bool | bCheckAgainstStdKeys, | |||
bool | bAllowLetterShortcuts, | |||
bool | bAllowWinKey = false | |||
) |
Member Function Documentation
void KKeyChooser::_warning | ( | const KKeySequence & | seq, | |
QString | sAction, | |||
QString | sTitle | |||
) | [protected] |
Definition at line 938 of file kkeydialog.cpp.
void KKeyChooser::allDefault | ( | ) | [slot] |
void KKeyChooser::capturedShortcut | ( | const KShortcut & | cut | ) | [protected, slot] |
Definition at line 652 of file kkeydialog.cpp.
bool KKeyChooser::checkGlobalShortcutsConflict | ( | const KShortcut & | cut, | |
bool | warnUser, | |||
QWidget * | parent | |||
) | [static] |
Checks whether the given shortcut conflicts with global keyboard shortcuts.
If yes, and the warnUser argument is true, warns the user and gives them a chance to reassign the shortcut from the global shortcut.
- Returns:
- true if there was conflict (and the user didn't reassign the shortcut)
- Parameters:
-
cut the shortcut that will be checked for conflicts warnUser if true, the user will be warned about a conflict and given a chance to reassign the shortcut parent parent widget for the warning dialog
- Since:
- 3.2
Definition at line 849 of file kkeydialog.cpp.
bool KKeyChooser::checkStandardShortcutsConflict | ( | const KShortcut & | cut, | |
bool | warnUser, | |||
QWidget * | parent | |||
) | [static] |
Checks whether the given shortcut conflicts with standard keyboard shortcuts.
If yes, and the warnUser argument is true, warns the user and gives them a chance to reassign the shortcut from the standard shortcut.
- Returns:
- true if there was conflict (and the user didn't reassign the shortcut)
- Parameters:
-
cut the shortcut that will be checked for conflicts warnUser if true, the user will be warned about a conflict and given a chance to reassign the shortcut parent parent widget for the warning dialog
- Since:
- 3.2
Definition at line 831 of file kkeydialog.cpp.
void KKeyChooser::commitChanges | ( | ) |
This function writes any shortcut changes back to the original action set(s).
Definition at line 273 of file kkeydialog.cpp.
void KKeyChooser::fontChange | ( | const QFont & | _font | ) | [protected] |
void KKeyChooser::initGUI | ( | ActionType | type, | |
bool | bAllowLetterShortcuts | |||
) | [protected] |
Definition at line 292 of file kkeydialog.cpp.
bool KKeyChooser::insert | ( | KShortcutList * | pList | ) | [protected] |
Definition at line 266 of file kkeydialog.cpp.
bool KKeyChooser::insert | ( | KGlobalAccel * | pAccel | ) | [protected] |
Definition at line 259 of file kkeydialog.cpp.
bool KKeyChooser::insert | ( | KAccel * | pAccel | ) | [protected] |
Definition at line 252 of file kkeydialog.cpp.
bool KKeyChooser::insert | ( | KActionCollection * | pColl, | |
const QString & | title | |||
) |
Insert an action collection, i.e.
add all its actions to the ones already associated with the KKeyChooser object.
- Parameters:
-
title subtree title of this collection of shortcut.
- Since:
- 3.1
Definition at line 238 of file kkeydialog.cpp.
bool KKeyChooser::insert | ( | KActionCollection * | pColl | ) |
Insert an action collection, i.e.
add all its actions to the ones already associated with the KKeyChooser object.
Definition at line 233 of file kkeydialog.cpp.
bool KKeyChooser::isKeyPresent | ( | const KShortcut & | cut, | |
bool | warnuser = true | |||
) | [protected] |
Definition at line 753 of file kkeydialog.cpp.
bool KKeyChooser::isKeyPresentLocally | ( | const KShortcut & | cut, | |
KKeyChooserItem * | ignoreItem, | |||
const QString & | warnText | |||
) | [protected] |
Definition at line 799 of file kkeydialog.cpp.
void KKeyChooser::keyChange | ( | ) | [signal] |
Emitted when an action's shortcut has been changed.
void KKeyChooser::listSync | ( | ) | [slot] |
Rebuild list entries based on underlying map.
Use this if you changed the underlying map.
Definition at line 662 of file kkeydialog.cpp.
void KKeyChooser::readGlobalKeys | ( | ) | [protected] |
Definition at line 580 of file kkeydialog.cpp.
void KKeyChooser::save | ( | ) |
This commits and then saves the actions to disk.
Any KActionCollection objects with the xmlFile() value set will be written to an XML file. All other will be written to the application's rc file.
Definition at line 285 of file kkeydialog.cpp.
void KKeyChooser::setPreferFourModifierKeys | ( | bool | preferFourModifierKeys | ) | [slot] |
Specifies whether to use the 3 or 4 modifier key scheme.
This determines which default is used when the 'Default' button is clicked.
Definition at line 647 of file kkeydialog.cpp.
void KKeyChooser::setShortcut | ( | const KShortcut & | cut | ) | [protected] |
Definition at line 702 of file kkeydialog.cpp.
void KKeyChooser::slotCustomKey | ( | ) | [protected, slot] |
Definition at line 575 of file kkeydialog.cpp.
void KKeyChooser::slotDefaultKey | ( | ) | [protected, slot] |
Definition at line 567 of file kkeydialog.cpp.
void KKeyChooser::slotListItemDoubleClicked | ( | QListViewItem * | ipoQListViewItem, | |
const QPoint & | ipoQPoint, | |||
int | c | |||
) | [protected, slot] |
Definition at line 635 of file kkeydialog.cpp.
void KKeyChooser::slotListItemSelected | ( | QListViewItem * | item | ) | [protected, slot] |
Definition at line 630 of file kkeydialog.cpp.
void KKeyChooser::slotNoKey | ( | ) | [protected, slot] |
Definition at line 555 of file kkeydialog.cpp.
void KKeyChooser::slotSettingsChanged | ( | int | category | ) | [protected, slot] |
Definition at line 596 of file kkeydialog.cpp.
void KKeyChooser::syncToConfig | ( | const QString & | sConfigGroup, | |
KConfigBase * | pConfig, | |||
bool | bClearUnset | |||
) |
Definition at line 678 of file kkeydialog.cpp.
void KKeyChooser::updateButtons | ( | ) | [protected] |
Definition at line 508 of file kkeydialog.cpp.
void KKeyChooser::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 1203 of file kkeydialog.cpp.
Member Data Documentation
bool KKeyChooser::m_bAllowLetterShortcuts [protected] |
Definition at line 197 of file kkeydialog.h.
bool KKeyChooser::m_bAllowWinKey [protected] |
Definition at line 198 of file kkeydialog.h.
bool KKeyChooser::m_bPreferFourModifierKeys [protected] |
Definition at line 201 of file kkeydialog.h.
QRadioButton* KKeyChooser::m_prbCustom [protected] |
Definition at line 205 of file kkeydialog.h.
QRadioButton* KKeyChooser::m_prbDef [protected] |
Definition at line 204 of file kkeydialog.h.
QRadioButton* KKeyChooser::m_prbNone [protected] |
Definition at line 203 of file kkeydialog.h.
ActionType KKeyChooser::m_type [protected] |
Definition at line 196 of file kkeydialog.h.
The documentation for this class was generated from the following files: