KHTML
KJavaAppletServer Class Reference
#include <kjavaappletserver.h>
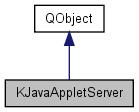
Public Member Functions | |
QString | appletLabel () |
bool | callMember (QStringList &args, QStringList &ret_args) |
bool | createApplet (int contextId, int appletId, const QString &name, const QString &clazzName, const QString &baseURL, const QString &user, const QString &password, const QString &authname, const QString &codeBase, const QString &jarFile, QSize size, const QMap< QString, QString > ¶ms, const QString &windowTitle) |
void | createContext (int contextId, KJavaAppletContext *context) |
void | derefObject (QStringList &args) |
void | destroyApplet (int contextId, int appletId) |
void | destroyContext (int contextId) |
void | endWaitForReturnData () |
bool | getMember (QStringList &args, QStringList &ret_args) |
void | initApplet (int contextId, int appletId) |
KJavaProcess * | javaProcess () |
KJavaAppletServer () | |
bool | putMember (QStringList &args) |
void | quit () |
void | removeDataJob (int loaderID) |
void | sendURLData (int loaderID, int code, const QByteArray &data) |
void | showConsole () |
void | startApplet (int contextId, int appletId) |
void | stopApplet (int contextId, int appletId) |
bool | usingKIO () |
void | waitForReturnData (JSStackFrame *) |
~KJavaAppletServer () | |
Static Public Member Functions | |
static KJavaAppletServer * | allocateJavaServer () |
static void | freeJavaServer () |
static QString | getAppletLabel () |
Protected Slots | |
void | checkShutdown () |
void | slotJavaRequest (const QByteArray &qb) |
void | timerEvent (QTimerEvent *) |
Protected Member Functions | |
void | setupJava (KJavaProcess *p) |
Protected Attributes | |
KJavaProcess * | process |
Detailed Description
Definition at line 42 of file kjavaappletserver.h.
Constructor & Destructor Documentation
KJavaAppletServer::KJavaAppletServer | ( | ) |
Create the applet server.
These shouldn't be used directly, use allocateJavaServer instead
Definition at line 134 of file kjavaappletserver.cpp.
KJavaAppletServer::~KJavaAppletServer | ( | ) |
Definition at line 154 of file kjavaappletserver.cpp.
Member Function Documentation
KJavaAppletServer * KJavaAppletServer::allocateJavaServer | ( | ) | [static] |
A factory method that returns the default server.
This is the way this class is usually instantiated.
Definition at line 175 of file kjavaappletserver.cpp.
QString KJavaAppletServer::appletLabel | ( | ) |
Definition at line 170 of file kjavaappletserver.cpp.
bool KJavaAppletServer::callMember | ( | QStringList & | args, | |
QStringList & | ret_args | |||
) |
Definition at line 756 of file kjavaappletserver.cpp.
void KJavaAppletServer::checkShutdown | ( | ) | [protected, slot] |
Definition at line 206 of file kjavaappletserver.cpp.
bool KJavaAppletServer::createApplet | ( | int | contextId, | |
int | appletId, | |||
const QString & | name, | |||
const QString & | clazzName, | |||
const QString & | baseURL, | |||
const QString & | user, | |||
const QString & | password, | |||
const QString & | authname, | |||
const QString & | codeBase, | |||
const QString & | jarFile, | |||
QSize | size, | |||
const QMap< QString, QString > & | params, | |||
const QString & | windowTitle | |||
) |
Create an applet in the specified context with the specified id.
The applet name, class etc. are specified in the same way as in the HTML APPLET tag.
Definition at line 336 of file kjavaappletserver.cpp.
void KJavaAppletServer::createContext | ( | int | contextId, | |
KJavaAppletContext * | context | |||
) |
Create an applet context with the specified id.
Definition at line 313 of file kjavaappletserver.cpp.
void KJavaAppletServer::derefObject | ( | QStringList & | args | ) |
Definition at line 766 of file kjavaappletserver.cpp.
Destroy an applet in the specified context with the specified id.
Definition at line 404 of file kjavaappletserver.cpp.
void KJavaAppletServer::destroyContext | ( | int | contextId | ) |
Destroy the applet context with the specified id.
All the applets in the context will be destroyed as well.
Definition at line 325 of file kjavaappletserver.cpp.
void KJavaAppletServer::endWaitForReturnData | ( | ) |
Definition at line 710 of file kjavaappletserver.cpp.
void KJavaAppletServer::freeJavaServer | ( | ) | [static] |
When you are done using your reference to the AppletServer, you must dereference it by calling freeJavaServer().
Definition at line 187 of file kjavaappletserver.cpp.
QString KJavaAppletServer::getAppletLabel | ( | ) | [static] |
This allows the KJavaAppletWidget to display some feedback in a QLabel while the applet is being loaded.
If the java process could not be started, an error message is displayed instead.
Definition at line 162 of file kjavaappletserver.cpp.
bool KJavaAppletServer::getMember | ( | QStringList & | args, | |
QStringList & | ret_args | |||
) |
Definition at line 735 of file kjavaappletserver.cpp.
This should be called by the KJavaAppletWidget.
Definition at line 394 of file kjavaappletserver.cpp.
KJavaProcess* KJavaAppletServer::javaProcess | ( | ) | [inline] |
Definition at line 135 of file kjavaappletserver.h.
bool KJavaAppletServer::putMember | ( | QStringList & | args | ) |
Definition at line 745 of file kjavaappletserver.cpp.
void KJavaAppletServer::quit | ( | ) |
void KJavaAppletServer::removeDataJob | ( | int | loaderID | ) |
Removes KJavaDownloader from the list (deletes it too).
Definition at line 449 of file kjavaappletserver.cpp.
void KJavaAppletServer::sendURLData | ( | int | loaderID, | |
int | code, | |||
const QByteArray & | data | |||
) |
Send data we got back from a KJavaDownloader back to the appropriate class loader.
Definition at line 440 of file kjavaappletserver.cpp.
void KJavaAppletServer::setupJava | ( | KJavaProcess * | p | ) | [protected] |
Definition at line 215 of file kjavaappletserver.cpp.
void KJavaAppletServer::showConsole | ( | ) |
void KJavaAppletServer::slotJavaRequest | ( | const QByteArray & | qb | ) | [protected, slot] |
Definition at line 467 of file kjavaappletserver.cpp.
void KJavaAppletServer::timerEvent | ( | QTimerEvent * | ) | [protected, slot] |
bool KJavaAppletServer::usingKIO | ( | ) |
Definition at line 770 of file kjavaappletserver.cpp.
void KJavaAppletServer::waitForReturnData | ( | JSStackFrame * | frame | ) |
Definition at line 724 of file kjavaappletserver.cpp.
Member Data Documentation
KJavaProcess* KJavaAppletServer::process [protected] |
Definition at line 151 of file kjavaappletserver.h.
The documentation for this class was generated from the following files: