kio
KBookmarkManager Class Reference
This class implements the reading/writing of bookmarks in XML. More...
#include <kbookmarkmanager.h>
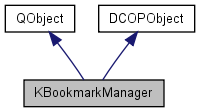
Public Slots | |
void | slotEditBookmarks () |
void | slotEditBookmarksAtAddress (const QString &address) |
Signals | |
void | changed (const QString &groupAddress, const QString &caller) |
Public Member Functions | |
KBookmarkGroup | addBookmarkDialog (const QString &_url, const QString &_title, const QString &_parentBookmarkAddress=QString::null) |
void | emitChanged (KBookmarkGroup &group) |
void | emitConfigChanged () |
KBookmark | findByAddress (const QString &address, bool tolerate=false) |
const QDomDocument & | internalDocument () const |
KBookmarkNotifier & | notifier () |
QString | path () |
KBookmarkGroup | root () const |
bool | save (bool toolbarCache=true) const |
bool | saveAs (const QString &filename, bool toolbarCache=true) const |
void | setEditorOptions (const QString &caption, bool browser) |
void | setShowNSBookmarks (bool show) |
void | setUpdate (bool update) |
bool | showNSBookmarks () const |
KBookmarkGroup | toolbar () |
bool | updateAccessMetadata (const QString &url, bool emitSignal=true) |
void | updateFavicon (const QString &url, const QString &faviconurl, bool emitSignal=true) |
~KBookmarkManager () | |
Static Public Member Functions | |
static KBookmarkManager * | createTempManager () |
static KBookmarkManager * | managerForFile (const QString &bookmarksFile, bool bImportDesktopFiles=true) |
static QString | userBookmarksFile () |
static KBookmarkManager * | userBookmarksManager () |
Protected Member Functions | |
void | importDesktopFiles () |
KBookmarkManager () | |
KBookmarkManager (const QString &bookmarksFile, bool bImportDesktopFiles=true) | |
void | parse () const |
Static Protected Member Functions | |
static void | convertAttribute (QDomElement elem, const QString &oldName, const QString &newName) |
static void | convertToXBEL (QDomElement &group) |
Detailed Description
This class implements the reading/writing of bookmarks in XML.The bookmarks file is read and written using the XBEL standard (http://pyxml.sourceforge.net/topics/xbel/)
A sample file looks like this :
<xbel> <bookmark href="http://developer.kde.org"><title>Developer Web Site</title></bookmark> <folder folded="no"> <title>Title of this folder</title> <bookmark icon="kde" href="http://www.kde.org"><title>KDE Web Site</title></bookmark> <folder toolbar="yes"> <title>My own bookmarks</title> <bookmark href="http://www.koffice.org"><title>KOffice Web Site</title></bookmark> <separator/> <bookmark href="http://www.kdevelop.org"><title>KDevelop Web Site</title></bookmark> </folder> </folder> </xbel>
Definition at line 53 of file kbookmarkmanager.h.
Constructor & Destructor Documentation
KBookmarkManager::KBookmarkManager | ( | const QString & | bookmarksFile, | |
bool | bImportDesktopFiles = true | |||
) | [protected] |
Creates a bookmark manager with a path to the bookmarks.
By default, it will use the KDE standard dirs to find and create the correct location. If you are using your own app-specific bookmarks directory, you must instantiate this class with your own path before KBookmarkManager::managerForFile() is ever called.
- Parameters:
-
bookmarksFile full path to the bookmarks file, Use ~/.kde/share/apps/konqueror/bookmarks.xml for the konqueror bookmarks bImportDesktopFiles if true, and if the bookmarksFile doesn't already exist, import bookmarks from desktop files
Definition at line 130 of file kbookmarkmanager.cc.
KBookmarkManager::KBookmarkManager | ( | ) | [protected] |
KBookmarkManager::~KBookmarkManager | ( | ) |
Member Function Documentation
KBookmarkGroup KBookmarkManager::addBookmarkDialog | ( | const QString & | _url, | |
const QString & | _title, | |||
const QString & | _parentBookmarkAddress = QString::null | |||
) |
Signals that the group (or any of its children) with the address groupAddress
(e.g.
"/4/5") has been modified by the caller caller
.
void KBookmarkManager::convertAttribute | ( | QDomElement | elem, | |
const QString & | oldName, | |||
const QString & | newName | |||
) | [static, protected] |
Definition at line 302 of file kbookmarkmanager.cc.
void KBookmarkManager::convertToXBEL | ( | QDomElement & | group | ) | [static, protected] |
Definition at line 251 of file kbookmarkmanager.cc.
KBookmarkManager * KBookmarkManager::createTempManager | ( | ) | [static] |
Definition at line 117 of file kbookmarkmanager.cc.
void KBookmarkManager::emitChanged | ( | KBookmarkGroup & | group | ) |
Saves the bookmark file and notifies everyone.
- Parameters:
-
group the parent of all changed bookmarks
Definition at line 535 of file kbookmarkmanager.cc.
void KBookmarkManager::emitConfigChanged | ( | ) |
Definition at line 552 of file kbookmarkmanager.cc.
- Returns:
- the bookmark designated by
address
- Parameters:
-
address the address belonging to the bookmark you're looking for tolerate when true tries to find the most tolerable bookmark position
- See also:
- KBookmark::address
Definition at line 425 of file kbookmarkmanager.cc.
void KBookmarkManager::importDesktopFiles | ( | ) | [protected] |
Definition at line 311 of file kbookmarkmanager.cc.
const QDomDocument & KBookmarkManager::internalDocument | ( | ) | const |
KBookmarkManager * KBookmarkManager::managerForFile | ( | const QString & | bookmarksFile, | |
bool | bImportDesktopFiles = true | |||
) | [static] |
This static function will return an instance of the KBookmarkManager, responsible for the given bookmarksFile
.
If you do not instantiate this class either natively or in a derived class, then it will return an object with the default behaviors. If you wish to use different behaviors, you must derive your own class and instantiate it before this method is ever called.
- Parameters:
-
bookmarksFile full path to the bookmarks file, Use ~/.kde/share/apps/konqueror/bookmarks.xml for the konqueror bookmarks bImportDesktopFiles if true, and if the bookmarksFile doesn't already exist, import bookmarks from desktop files
- Returns:
- a pointer to an instance of the KBookmarkManager.
Definition at line 100 of file kbookmarkmanager.cc.
KBookmarkNotifier& KBookmarkManager::notifier | ( | ) | [inline] |
Access to bookmark notifier, for emitting signals.
We need this object to exist in one instance only, so we could connectDCOP to it by name.
Definition at line 237 of file kbookmarkmanager.h.
void KBookmarkManager::notifyChanged | ( | QString | groupAddress | ) |
Emit the changed signal for the group whose address is given.
- See also:
- KBookmark::address() Called by the instance of konqueror that saved the file after a small change (new bookmark or new folder).
Definition at line 577 of file kbookmarkmanager.cc.
void KBookmarkManager::notifyCompleteChange | ( | QString | caller | ) |
Reparse the whole bookmarks file and notify about the change (Called by the bookmark editor).
Definition at line 557 of file kbookmarkmanager.cc.
void KBookmarkManager::notifyConfigChanged | ( | ) |
Definition at line 570 of file kbookmarkmanager.cc.
void KBookmarkManager::parse | ( | ) | const [protected] |
Definition at line 198 of file kbookmarkmanager.cc.
QString KBookmarkManager::path | ( | ) | [inline] |
This will return the path that this manager is using to read the bookmarks.
For internal use only.
- Returns:
- the path containing the bookmarks
Definition at line 134 of file kbookmarkmanager.h.
KBookmarkGroup KBookmarkManager::root | ( | ) | const |
This will return the root bookmark.
It is used to iterate through the bookmarks manually. It is mostly used internally.
- Returns:
- the root (top-level) bookmark
Definition at line 379 of file kbookmarkmanager.cc.
bool KBookmarkManager::save | ( | bool | toolbarCache = true |
) | const |
Save the bookmarks to the default konqueror XML file on disk.
You should use emitChanged() instead of this function, it saves and notifies everyone that the file has changed.
- Parameters:
-
toolbarCache iff true save a cache of the toolbar folder, too
- Returns:
- true if saving was successful
Definition at line 321 of file kbookmarkmanager.cc.
bool KBookmarkManager::saveAs | ( | const QString & | filename, | |
bool | toolbarCache = true | |||
) | const |
Save the bookmarks to the given XML file on disk.
- Parameters:
-
filename full path to the desired bookmarks file location toolbarCache iff true save a cache of the toolbar folder, too
- Returns:
- true if saving was successful
Definition at line 326 of file kbookmarkmanager.cc.
void KBookmarkManager::setEditorOptions | ( | const QString & | caption, | |
bool | browser | |||
) |
Set options with which slotEditBookmarks called keditbookmarks this can be used to change the appearance of the keditbookmarks in order to provide a slightly differing outer shell depending on the bookmarks file / app which calls it.
- Parameters:
-
caption the --caption string, for instance "Konsole" browser iff false display no browser specific menu items in keditbookmarks :: --nobrowser
- Since:
- 3.2
Definition at line 608 of file kbookmarkmanager.cc.
void KBookmarkManager::setShowNSBookmarks | ( | bool | show | ) |
Shows an extra menu for NS bookmarks.
Set this to false, if you don't want this.
Definition at line 597 of file kbookmarkmanager.cc.
void KBookmarkManager::setUpdate | ( | bool | update | ) |
Set the update flag.
Defaults to true. TODO - check
- Parameters:
-
update if true then KBookmarkManager will listen to DCOP update requests.
Definition at line 182 of file kbookmarkmanager.cc.
bool KBookmarkManager::showNSBookmarks | ( | ) | const |
- Returns:
- true if the NS bookmarks should be dynamically shown in the toplevel kactionmenu
Definition at line 592 of file kbookmarkmanager.cc.
void KBookmarkManager::slotEditBookmarks | ( | ) | [slot] |
Definition at line 614 of file kbookmarkmanager.cc.
void KBookmarkManager::slotEditBookmarksAtAddress | ( | const QString & | address | ) | [slot] |
Definition at line 626 of file kbookmarkmanager.cc.
KBookmarkGroup KBookmarkManager::toolbar | ( | ) |
This returns the root of the toolbar menu.
In the XML, this is the group with the attribute TOOLBAR=1
- Returns:
- the toolbar group
Definition at line 384 of file kbookmarkmanager.cc.
bool KBookmarkManager::updateAccessMetadata | ( | const QString & | url, | |
bool | emitSignal = true | |||
) |
Update access time stamps for a given url.
- Parameters:
-
url the viewed url emitSignal iff true emit KBookmarkNotifier signal
- Since:
- 3.2
- Returns:
- true if any metadata was modified (bookmarks file is not saved automatically)
Definition at line 645 of file kbookmarkmanager.cc.
void KBookmarkManager::updateFavicon | ( | const QString & | url, | |
const QString & | faviconurl, | |||
bool | emitSignal = true | |||
) |
Definition at line 666 of file kbookmarkmanager.cc.
QString KBookmarkManager::userBookmarksFile | ( | ) | [static] |
Returns the path to the user's main bookmark collection file.
- Since:
- 3.5.5
Definition at line 691 of file kbookmarkmanager.cc.
KBookmarkManager * KBookmarkManager::userBookmarksManager | ( | ) | [static] |
Returns a pointer to the users main bookmark collection.
- Since:
- 3.2
Definition at line 696 of file kbookmarkmanager.cc.
The documentation for this class was generated from the following files: