kio
KFileTreeView Class Reference
The filetreeview offers a treeview on the file system which behaves like a QTreeView showing files and/or directories in the file system. More...
#include <kfiletreeview.h>
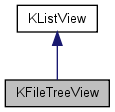
Public Slots | |
virtual void | setShowFolderOpenPixmap (bool showIt=true) |
Signals | |
void | dropped (KFileTreeView *, QDropEvent *, QListViewItem *, QListViewItem *) |
void | dropped (QDropEvent *e, QListViewItem *parent, QListViewItem *after) |
void | dropped (KFileTreeView *, QDropEvent *, QListViewItem *) |
void | dropped (QDropEvent *e, QListViewItem *after) |
void | dropped (QWidget *, QDropEvent *, KURL::List &, KURL &) |
void | dropped (KURL::List &, KURL &) |
void | dropped (QWidget *, QDropEvent *, KURL::List &) |
void | dropped (QWidget *, QDropEvent *) |
void | onItem (const QString &) |
Public Member Functions | |
virtual KFileTreeBranch * | addBranch (KFileTreeBranch *) |
virtual KFileTreeBranch * | addBranch (const KURL &path, const QString &name, const QPixmap &pix, bool showHidden=false) |
KFileTreeBranch * | addBranch (const KURL &path, const QString &name, bool showHidden=false) |
KFileTreeBranch * | branch (const QString &searchName) |
KFileTreeBranchList & | branches () |
KFileTreeViewItem * | currentKFileTreeViewItem () const |
KURL | currentURL () const |
KFileTreeViewItem * | findItem (const QString &branchName, const QString &relUrl) |
KFileTreeViewItem * | findItem (KFileTreeBranch *brnch, const QString &relUrl) |
KFileTreeView (QWidget *parent, const char *name=0) | |
virtual bool | removeBranch (KFileTreeBranch *branch) |
virtual void | setDirOnlyMode (KFileTreeBranch *branch, bool) |
bool | showFolderOpenPixmap () const |
virtual | ~KFileTreeView () |
Protected Slots | |
virtual QPixmap | itemIcon (KFileTreeViewItem *, int gap=0) const |
virtual void | slotNewTreeViewItems (KFileTreeBranch *, const KFileTreeViewItemList &) |
virtual void | slotSetNextUrlToSelect (const KURL &url) |
Protected Member Functions | |
virtual bool | acceptDrag (QDropEvent *event) const |
virtual void | contentsDragEnterEvent (QDragEnterEvent *e) |
virtual void | contentsDragLeaveEvent (QDragLeaveEvent *e) |
virtual void | contentsDragMoveEvent (QDragMoveEvent *e) |
virtual void | contentsDropEvent (QDropEvent *ev) |
virtual QDragObject * | dragObject () |
virtual void | startAnimation (KFileTreeViewItem *item, const char *iconBaseName="kde", uint iconCount=6) |
virtual void | stopAnimation (KFileTreeViewItem *item) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
KURL | m_nextUrlToSelect |
Detailed Description
The filetreeview offers a treeview on the file system which behaves like a QTreeView showing files and/or directories in the file system.KFileTreeView is able to handle more than one URL, represented by KFileTreeBranch.
Typical usage: 1. create a KFileTreeView fitting in your layout and add columns to it 2. call addBranch to create one or more branches 3. retrieve the root item with KFileTreeBranch::root() and set it open if desired. That starts the listing.
Definition at line 66 of file kfiletreeview.h.
Constructor & Destructor Documentation
KFileTreeView::KFileTreeView | ( | QWidget * | parent, | |
const char * | name = 0 | |||
) |
Definition at line 42 of file kfiletreeview.cpp.
KFileTreeView::~KFileTreeView | ( | ) | [virtual] |
Definition at line 85 of file kfiletreeview.cpp.
Member Function Documentation
bool KFileTreeView::acceptDrag | ( | QDropEvent * | event | ) | const [protected, virtual] |
- Returns:
- true if we can decode the drag and support the action
Definition at line 228 of file kfiletreeview.cpp.
KFileTreeBranch * KFileTreeView::addBranch | ( | KFileTreeBranch * | newBranch | ) | [virtual] |
same as the function above but letting the user create the branch.
Definition at line 376 of file kfiletreeview.cpp.
KFileTreeBranch * KFileTreeView::addBranch | ( | const KURL & | path, | |
const QString & | name, | |||
const QPixmap & | pix, | |||
bool | showHidden = false | |||
) | [virtual] |
same as the function above but with a pixmap to set for the branch.
Definition at line 365 of file kfiletreeview.cpp.
KFileTreeBranch * KFileTreeView::addBranch | ( | const KURL & | path, | |
const QString & | name, | |||
bool | showHidden = false | |||
) |
Adds a branch to the treeview item.
This high-level function creates the branch, adds it to the treeview and connects some signals. Note that directory listing does not start until a branch is expanded either by opening the root item by user or by setOpen on the root item.
- Returns:
- a pointer to the new branch or zero
- Parameters:
-
path is the base url of the branch name is the name of the branch, which will be the text for column 0 showHidden says if hidden files and directories should be visible
Definition at line 357 of file kfiletreeview.cpp.
KFileTreeBranch * KFileTreeView::branch | ( | const QString & | searchName | ) |
- Returns:
- a pointer to the KFileTreeBranch in the KFileTreeView or zero on failure.
- Parameters:
-
searchName is the name of a branch
Definition at line 390 of file kfiletreeview.cpp.
KFileTreeBranchList & KFileTreeView::branches | ( | ) |
- Returns:
- a list of pointers to all existing branches in the treeview.
Definition at line 408 of file kfiletreeview.cpp.
void KFileTreeView::contentsDragEnterEvent | ( | QDragEnterEvent * | e | ) | [protected, virtual] |
Definition at line 111 of file kfiletreeview.cpp.
void KFileTreeView::contentsDragLeaveEvent | ( | QDragLeaveEvent * | e | ) | [protected, virtual] |
Definition at line 167 of file kfiletreeview.cpp.
void KFileTreeView::contentsDragMoveEvent | ( | QDragMoveEvent * | e | ) | [protected, virtual] |
Definition at line 133 of file kfiletreeview.cpp.
void KFileTreeView::contentsDropEvent | ( | QDropEvent * | ev | ) | [protected, virtual] |
Definition at line 182 of file kfiletreeview.cpp.
KFileTreeViewItem * KFileTreeView::currentKFileTreeViewItem | ( | ) | const |
KURL KFileTreeView::currentURL | ( | ) | const |
QDragObject * KFileTreeView::dragObject | ( | ) | [protected, virtual] |
Definition at line 252 of file kfiletreeview.cpp.
void KFileTreeView::dropped | ( | KFileTreeView * | , | |
QDropEvent * | , | |||
QListViewItem * | , | |||
QListViewItem * | ||||
) | [signal] |
void KFileTreeView::dropped | ( | QDropEvent * | e, | |
QListViewItem * | parent, | |||
QListViewItem * | after | |||
) | [signal] |
void KFileTreeView::dropped | ( | KFileTreeView * | , | |
QDropEvent * | , | |||
QListViewItem * | ||||
) | [signal] |
void KFileTreeView::dropped | ( | QDropEvent * | e, | |
QListViewItem * | after | |||
) | [signal] |
void KFileTreeView::dropped | ( | QWidget * | , | |
QDropEvent * | , | |||
KURL::List & | , | |||
KURL & | ||||
) | [signal] |
void KFileTreeView::dropped | ( | KURL::List & | , | |
KURL & | ||||
) | [signal] |
void KFileTreeView::dropped | ( | QWidget * | , | |
QDropEvent * | , | |||
KURL::List & | ||||
) | [signal] |
void KFileTreeView::dropped | ( | QWidget * | , | |
QDropEvent * | ||||
) | [signal] |
KFileTreeViewItem * KFileTreeView::findItem | ( | const QString & | branchName, | |
const QString & | relUrl | |||
) |
see method above, differs only in the first parameter.
Finds the branch by its name.
Definition at line 619 of file kfiletreeview.cpp.
KFileTreeViewItem * KFileTreeView::findItem | ( | KFileTreeBranch * | brnch, | |
const QString & | relUrl | |||
) |
searches a branch for a KFileTreeViewItem identified by the relative url given as second parameter.
The method adds the branches base url to the relative path and finds the item.
- Returns:
- a pointer to the item or zero if the item does not exist.
- Parameters:
-
brnch is a pointer to the branch to search in relUrl is the branch relativ url
Definition at line 625 of file kfiletreeview.cpp.
QPixmap KFileTreeView::itemIcon | ( | KFileTreeViewItem * | item, | |
int | gap = 0 | |||
) | const [protected, virtual, slot] |
Definition at line 474 of file kfiletreeview.cpp.
void KFileTreeView::onItem | ( | const QString & | ) | [signal] |
bool KFileTreeView::removeBranch | ( | KFileTreeBranch * | branch | ) | [virtual] |
removes the branch from the treeview.
- Parameters:
-
branch is a pointer to the branch
- Returns:
- true on success.
Definition at line 414 of file kfiletreeview.cpp.
void KFileTreeView::setDirOnlyMode | ( | KFileTreeBranch * | branch, | |
bool | bom | |||
) | [virtual] |
set the directory mode for branches.
If true is passed, only directories will be loaded.
- Parameters:
-
branch is a pointer to a KFileTreeBranch
Definition at line 428 of file kfiletreeview.cpp.
virtual void KFileTreeView::setShowFolderOpenPixmap | ( | bool | showIt = true |
) | [inline, virtual, slot] |
set the flag to show 'extended' folder icons on or off.
If switched on, folders will have an open folder pixmap displayed if their children are visible, and the standard closed folder pixmap (from mimetype folder) if they are closed. If switched off, the plain mime pixmap is displayed.
- Parameters:
-
showIt = false displays mime type pixmap only
Definition at line 163 of file kfiletreeview.h.
bool KFileTreeView::showFolderOpenPixmap | ( | ) | const [inline] |
- Returns:
- a flag indicating if extended folder pixmaps are displayed or not.
Definition at line 152 of file kfiletreeview.h.
void KFileTreeView::slotNewTreeViewItems | ( | KFileTreeBranch * | branch, | |
const KFileTreeViewItemList & | itemList | |||
) | [protected, virtual, slot] |
Definition at line 443 of file kfiletreeview.cpp.
virtual void KFileTreeView::slotSetNextUrlToSelect | ( | const KURL & | url | ) | [inline, protected, virtual, slot] |
Definition at line 185 of file kfiletreeview.h.
void KFileTreeView::startAnimation | ( | KFileTreeViewItem * | item, | |
const char * | iconBaseName = "kde" , |
|||
uint | iconCount = 6 | |||
) | [protected, virtual] |
Definition at line 538 of file kfiletreeview.cpp.
void KFileTreeView::stopAnimation | ( | KFileTreeViewItem * | item | ) | [protected, virtual] |
Definition at line 555 of file kfiletreeview.cpp.
void KFileTreeView::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 674 of file kfiletreeview.cpp.
Member Data Documentation
KURL KFileTreeView::m_nextUrlToSelect [protected] |
Definition at line 223 of file kfiletreeview.h.
The documentation for this class was generated from the following files: