kio
KIO::Connection Class Reference
This class provides a simple means for IPC between two applications via a pipe. More...
#include <connection.h>
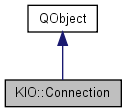
Public Member Functions | |
void | close () |
void | connect (QObject *receiver=0, const char *member=0) |
Connection () | |
int | fd_from () const |
int | fd_to () const |
void | init (int fd_in, int fd_out) |
void | init (KSocket *sock) |
bool | inited () const |
int | read (int *_cmd, QByteArray &data) |
void | resume () |
void | send (int cmd, const QByteArray &arr=QByteArray()) |
bool | sendnow (int _cmd, const QByteArray &data) |
void | suspend () |
bool | suspended () const |
virtual | ~Connection () |
Protected Slots | |
void | dequeue () |
Detailed Description
This class provides a simple means for IPC between two applications via a pipe.It handles a queue of commands to be sent which makes it possible to queue data before an actual connection has been established.
Definition at line 49 of file connection.h.
Constructor & Destructor Documentation
Connection::Connection | ( | ) |
Connection::~Connection | ( | ) | [virtual] |
Definition at line 60 of file connection.cpp.
Member Function Documentation
void Connection::close | ( | ) |
void Connection::connect | ( | QObject * | receiver = 0 , |
|
const char * | member = 0 | |||
) |
Definition at line 161 of file connection.cpp.
void Connection::dequeue | ( | ) | [protected, slot] |
Definition at line 110 of file connection.cpp.
int KIO::Connection::fd_from | ( | ) | const [inline] |
Returns the input file descriptor.
- Returns:
- the input file descriptor
Definition at line 82 of file connection.h.
int KIO::Connection::fd_to | ( | ) | const [inline] |
Returns the output file descriptor.
- Returns:
- the output file descriptor
Definition at line 87 of file connection.h.
void Connection::init | ( | int | fd_in, | |
int | fd_out | |||
) |
Initialize the connection to use the given file descriptors.
- Parameters:
-
fd_in the input file descriptor to use fd_out the output file descriptor to use
- See also:
- inited()
Definition at line 144 of file connection.cpp.
void Connection::init | ( | KSocket * | sock | ) |
Initialize this connection to use the given socket.
- Parameters:
-
sock the socket to use
- See also:
- inited()
Definition at line 124 of file connection.cpp.
bool KIO::Connection::inited | ( | ) | const [inline] |
Checks whether the connection has been initialized.
- Returns:
- true if the initialized
- See also:
- init()
Definition at line 94 of file connection.h.
int Connection::read | ( | int * | _cmd, | |
QByteArray & | data | |||
) |
Receive data.
- Parameters:
-
_cmd the received command will be written here data the received data will be written here
- Returns:
- >=0 indicates the received data size upon success -1 indicates error
Definition at line 209 of file connection.cpp.
void Connection::resume | ( | ) |
void Connection::send | ( | int | cmd, | |
const QByteArray & | arr = QByteArray() | |||
) |
Sends/queues the given command to be sent.
- Parameters:
-
cmd the command to set arr the bytes to send
Definition at line 98 of file connection.cpp.
bool Connection::sendnow | ( | int | _cmd, | |
const QByteArray & | data | |||
) |
Sends the given command immediately.
- Parameters:
-
_cmd the command to set data the bytes to send
- Returns:
- true if successful, false otherwise
Definition at line 175 of file connection.cpp.
void Connection::suspend | ( | ) |
bool KIO::Connection::suspended | ( | ) | const [inline] |
Returns status of connection.
- Returns:
- true if suspended, false otherwise
Definition at line 135 of file connection.h.
The documentation for this class was generated from the following files: