kio
KIO::ForwardingSlaveBase Class Reference
This class should be used as a base for ioslaves acting as a forwarder to other ioslaves. More...
#include <forwardingslavebase.h>
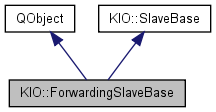
Public Member Functions | |
virtual void | chmod (const KURL &url, int permissions) |
virtual void | copy (const KURL &src, const KURL &dest, int permissions, bool overwrite) |
virtual void | del (const KURL &url, bool isfile) |
ForwardingSlaveBase (const QCString &protocol, const QCString &poolSocket, const QCString &appSocket) | |
virtual void | get (const KURL &url) |
virtual void | listDir (const KURL &url) |
virtual void | mimetype (const KURL &url) |
virtual void | mkdir (const KURL &url, int permissions) |
virtual void | put (const KURL &url, int permissions, bool overwrite, bool resume) |
virtual void | rename (const KURL &src, const KURL &dest, bool overwrite) |
virtual void | stat (const KURL &url) |
virtual void | symlink (const QString &target, const KURL &dest, bool overwrite) |
virtual | ~ForwardingSlaveBase () |
Protected Member Functions | |
virtual void | prepareUDSEntry (KIO::UDSEntry &entry, bool listing=false) const |
KURL | processedURL () const |
KURL | requestedURL () const |
virtual bool | rewriteURL (const KURL &url, KURL &newURL)=0 |
Detailed Description
This class should be used as a base for ioslaves acting as a forwarder to other ioslaves.It has been designed to support only local filesystem like ioslaves.
If the resulting ioslave should be a simple proxy, you only need to implement the ForwardingSlaveBase::rewriteURL() method.
For more advanced behavior, the classic ioslave methods should be reimplemented, because their default behavior in this class is to forward using the ForwardingSlaveBase::rewriteURL() method.
A possible code snippet for an advanced stat() behavior would look like this in the child class:
void ChildProtocol::stat(const KURL &url) { bool is_special = false; // Process the URL to see if it should have // a special treatment if ( is_special ) { // Handle the URL ourselves KIO::UDSEntry entry; // Fill entry with UDSAtom instances statEntry(entry); finished(); } else { // Setup the ioslave internal state if // required by ChildProtocol::rewriteURL() ForwardingSlaveBase::stat(url); } }
Of course in this case, you surely need to reimplement listDir() and get() accordingly.
If you want view on directories to be correctly refreshed when something changes on a forwarded URL, you'll need a companion kded module to emit the KDirNotify Files*() DCOP signals.
This class was initially used for media:/ ioslave. This ioslave code and the MediaDirNotify class of its companion kded module can be a good source of inspiration.
- See also:
- ForwardingSlaveBase::rewriteURL()
- Since:
- 3.4
Definition at line 88 of file forwardingslavebase.h.
Constructor & Destructor Documentation
KIO::ForwardingSlaveBase::ForwardingSlaveBase | ( | const QCString & | protocol, | |
const QCString & | poolSocket, | |||
const QCString & | appSocket | |||
) |
Definition at line 37 of file forwardingslavebase.cpp.
KIO::ForwardingSlaveBase::~ForwardingSlaveBase | ( | ) | [virtual] |
Definition at line 44 of file forwardingslavebase.cpp.
Member Function Documentation
void KIO::ForwardingSlaveBase::chmod | ( | const KURL & | url, | |
int | permissions | |||
) | [virtual] |
Change permissions on path
The slave emits ERR_DOES_NOT_EXIST or ERR_CANNOT_CHMOD.
Reimplemented from KIO::SlaveBase.
Definition at line 235 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::copy | ( | const KURL & | src, | |
const KURL & | dest, | |||
int | permissions, | |||
bool | overwrite | |||
) | [virtual] |
Copy src
into dest
.
If the slave returns an error ERR_UNSUPPORTED_ACTION, the job will ask for get + put instead.
- Parameters:
-
src where to copy the file from (decoded) dest where to copy the file to (decoded) permissions may be -1. In this case no special permission mode is set. overwrite if true, any existing file will be overwritten
Reimplemented from KIO::SlaveBase.
Definition at line 249 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::del | ( | const KURL & | url, | |
bool | isfile | |||
) | [virtual] |
Delete a file or directory.
- Parameters:
-
url file/directory to delete isfile if true, a file should be deleted. if false, a directory should be deleted.
Reimplemented from KIO::SlaveBase.
Definition at line 265 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::get | ( | const KURL & | url | ) | [virtual] |
get, aka read.
- Parameters:
-
url the full url for this request. Host, port and user of the URL can be assumed to be the same as in the last setHost() call. The slave emits the data through data
Reimplemented from KIO::SlaveBase.
Definition at line 119 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::listDir | ( | const KURL & | url | ) | [virtual] |
Lists the contents of url
.
The slave should emit ERR_CANNOT_ENTER_DIRECTORY if it doesn't exist, if we don't have enough permissions, or if it is a file It should also emit totalFiles as soon as it knows how many files it will list.
Reimplemented from KIO::SlaveBase.
Definition at line 177 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::mimetype | ( | const KURL & | url | ) | [virtual] |
Finds mimetype for one file or directory.
This method should either emit 'mimeType' or it should send a block of data big enough to be able to determine the mimetype.
If the slave doesn't reimplement it, a get will be issued, i.e. the whole file will be downloaded before determining the mimetype on it - this is obviously not a good thing in most cases.
Reimplemented from KIO::SlaveBase.
Definition at line 163 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::mkdir | ( | const KURL & | url, | |
int | permissions | |||
) | [virtual] |
Create a directory.
- Parameters:
-
url path to the directory to create permissions the permissions to set after creating the directory (-1 if no permissions to be set) The slave emits ERR_COULD_NOT_MKDIR if failure.
Reimplemented from KIO::SlaveBase.
Definition at line 191 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::prepareUDSEntry | ( | KIO::UDSEntry & | entry, | |
bool | listing = false | |||
) | const [protected, virtual] |
Allow to modify a UDSEntry before it's sent to the ioslave enpoint.
This is the default implementation working in most case, but sometimes you could make use of more forwarding black magic (for example dynamically transform any desktop file into a fake directory...)
- Parameters:
-
entry the UDSEntry to post-process listing indicate if this entry it created during a listDir operation
Definition at line 66 of file forwardingslavebase.cpp.
KURL KIO::ForwardingSlaveBase::processedURL | ( | ) | const [inline, protected] |
Return the URL being processed by the ioslave Only access it inside prepareUDSEntry().
Definition at line 154 of file forwardingslavebase.h.
void KIO::ForwardingSlaveBase::put | ( | const KURL & | url, | |
int | permissions, | |||
bool | overwrite, | |||
bool | resume | |||
) | [virtual] |
put, i.e.
write data into a file.
- Parameters:
-
url where to write the file permissions may be -1. In this case no special permission mode is set. overwrite if true, any existing file will be overwritten. If the file indeed already exists, the slave should NOT apply the permissions change to it. resume currently unused, please ignore. The support for resuming using .part files is done by calling canResume().
- See also:
- canResume()
Reimplemented from KIO::SlaveBase.
Definition at line 133 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::rename | ( | const KURL & | src, | |
const KURL & | dest, | |||
bool | overwrite | |||
) | [virtual] |
Rename oldname
into newname
.
If the slave returns an error ERR_UNSUPPORTED_ACTION, the job will ask for copy + del instead.
- Parameters:
-
src where to move the file from dest where to move the file to overwrite if true, any existing file will be overwritten
Reimplemented from KIO::SlaveBase.
Definition at line 205 of file forwardingslavebase.cpp.
KURL KIO::ForwardingSlaveBase::requestedURL | ( | ) | const [inline, protected] |
Return the URL asked to the ioslave Only access it inside prepareUDSEntry().
Definition at line 160 of file forwardingslavebase.h.
virtual bool KIO::ForwardingSlaveBase::rewriteURL | ( | const KURL & | url, | |
KURL & | newURL | |||
) | [protected, pure virtual] |
Rewrite an url to it's forwarded counterpart.
It should return true if everything was ok, and false otherwise.
If a problem is detected it's up to this method to trigger error() before returning. Returning false silently cancel the current slave operation.
- Parameters:
-
url The URL as given during the slave call newURL The new URL to forward the slave call to
- Returns:
- true if the given url could be correctly rewritten
void KIO::ForwardingSlaveBase::stat | ( | const KURL & | url | ) | [virtual] |
Finds all details for one file or directory.
The information returned is the same as what listDir returns, but only for one file or directory.
Reimplemented from KIO::SlaveBase.
Definition at line 149 of file forwardingslavebase.cpp.
void KIO::ForwardingSlaveBase::symlink | ( | const QString & | target, | |
const KURL & | dest, | |||
bool | overwrite | |||
) | [virtual] |
Creates a symbolic link named dest
, pointing to target
, which may be a relative or an absolute path.
- Parameters:
-
target The string that will become the "target" of the link (can be relative) dest The symlink to create. overwrite whether to automatically overwrite if the dest exists
Reimplemented from KIO::SlaveBase.
Definition at line 220 of file forwardingslavebase.cpp.
The documentation for this class was generated from the following files: