kio
KProtocolInfo Class Reference
Information about I/O (Internet, etc. More...
#include <kprotocolinfo.h>
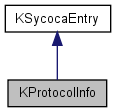
Classes | |
struct | ExtraField |
Definition of an extra field in the UDS entries, returned by a listDir operation. More... | |
Public Types | |
typedef QValueList< ExtraField > | ExtraFieldList |
enum | FileNameUsedForCopying { Name, FromURL } |
typedef KSharedPtr< KProtocolInfo > | Ptr |
enum | Type { T_STREAM, T_FILESYSTEM, T_NONE, T_ERROR } |
Public Member Functions | |
virtual bool | isValid () const |
KProtocolInfo (QDataStream &_str, int offset) | |
KProtocolInfo (const QString &path) | |
virtual void | load (QDataStream &) |
virtual QString | name () const |
virtual void | save (QDataStream &) |
virtual | ~KProtocolInfo () |
Static Public Member Functions | |
static bool | canCopyFromFile (const QString &protocol) KDE_DEPRECATED |
static bool | canCopyFromFile (const KURL &url) |
static bool | canCopyToFile (const QString &protocol) KDE_DEPRECATED |
static bool | canCopyToFile (const KURL &url) |
static bool | canDeleteRecursive (const KURL &url) |
static bool | canRenameFromFile (const KURL &url) |
static bool | canRenameToFile (const KURL &url) |
static QStringList | capabilities (const QString &protocol) |
static QString | config (const QString &protocol) |
static QString | defaultMimetype (const QString &protocol) KDE_DEPRECATED |
static QString | defaultMimetype (const KURL &url) |
static bool | determineMimetypeFromExtension (const QString &protocol) |
static QString | docPath (const QString &protocol) |
static QString | exec (const QString &protocol) |
static ExtraFieldList | extraFields (const KURL &url) |
static FileNameUsedForCopying | fileNameUsedForCopying (const KURL &url) |
static QString | icon (const QString &protocol) |
static Type | inputType (const QString &protocol) KDE_DEPRECATED |
static Type | inputType (const KURL &url) |
static bool | isFilterProtocol (const QString &protocol) |
static bool | isFilterProtocol (const KURL &url) |
static bool | isHelperProtocol (const QString &protocol) |
static bool | isHelperProtocol (const KURL &url) |
static bool | isKnownProtocol (const QString &protocol) |
static bool | isKnownProtocol (const KURL &url) |
static bool | isSourceProtocol (const QString &protocol) KDE_DEPRECATED |
static bool | isSourceProtocol (const KURL &url) |
static QStringList | listing (const QString &protocol) KDE_DEPRECATED |
static QStringList | listing (const KURL &url) |
static int | maxSlaves (const QString &protocol) |
static Type | outputType (const QString &protocol) KDE_DEPRECATED |
static Type | outputType (const KURL &url) |
static QString | protocolClass (const QString &protocol) |
static QStringList | protocols () |
static QString | proxiedBy (const QString &protocol) |
static bool | showFilePreview (const QString &protocol) |
static bool | supportsDeleting (const QString &protocol) KDE_DEPRECATED |
static bool | supportsDeleting (const KURL &url) |
static bool | supportsLinking (const QString &protocol) KDE_DEPRECATED |
static bool | supportsLinking (const KURL &url) |
static bool | supportsListing (const QString &protocol) KDE_DEPRECATED |
static bool | supportsListing (const KURL &url) |
static bool | supportsMakeDir (const QString &protocol) KDE_DEPRECATED |
static bool | supportsMakeDir (const KURL &url) |
static bool | supportsMoving (const QString &protocol) KDE_DEPRECATED |
static bool | supportsMoving (const KURL &url) |
static bool | supportsReading (const QString &protocol) KDE_DEPRECATED |
static bool | supportsReading (const KURL &url) |
static bool | supportsWriting (const QString &protocol) KDE_DEPRECATED |
static bool | supportsWriting (const KURL &url) |
static KURL::URIMode | uriParseMode (const QString &protocol) |
Protected Member Functions | |
bool | canDeleteRecursive () const |
bool | canRenameFromFile () const |
bool | canRenameToFile () const |
FileNameUsedForCopying | fileNameUsedForCopying () const |
virtual void | virtual_hook (int id, void *data) |
Static Protected Member Functions | |
static KProtocolInfo * | findProtocol (const KURL &url) |
Protected Attributes | |
bool | m_canCopyFromFile |
bool | m_canCopyToFile |
QString | m_config |
QString | m_defaultMimetype |
bool | m_determineMimetypeFromExtension |
QString | m_exec |
QString | m_icon |
Type | m_inputType |
bool | m_isHelperProtocol |
bool | m_isSourceProtocol |
QStringList | m_listing |
int | m_maxSlaves |
QString | m_name |
Type | m_outputType |
bool | m_supportsDeleting |
bool | m_supportsLinking |
bool | m_supportsListing |
bool | m_supportsMakeDir |
bool | m_supportsMoving |
bool | m_supportsReading |
bool | m_supportsWriting |
Detailed Description
Information about I/O (Internet, etc.) protocols supported by KDE.
This class is useful if you want to know which protocols KDE supports. In addition you can find out lots of information about a certain protocol. A KProtocolInfo instance represents a single protocol. Most of the functionality is provided by the static methods that scan the *.protocol files of all installed kioslaves to get this information.
*.protocol files are installed in the "services" resource.
Definition at line 44 of file kprotocolinfo.h.
Member Typedef Documentation
Definition at line 183 of file kprotocolinfo.h.
typedef KSharedPtr<KProtocolInfo> KProtocolInfo::Ptr |
Definition at line 50 of file kprotocolinfo.h.
Member Enumeration Documentation
enum KProtocolInfo::Type |
Describes the type of a protocol.
- Enumerator:
-
T_STREAM protocol returns a stream T_FILESYSTEM protocol describes location in a file system T_NONE no information about the tyope available T_ERROR used to signal an error
Definition at line 121 of file kprotocolinfo.h.
Constructor & Destructor Documentation
KProtocolInfo::KProtocolInfo | ( | const QString & | path | ) |
Read a protocol description file.
- Parameters:
-
path the path of the description file
KProtocolInfo::KProtocolInfo | ( | QDataStream & | _str, | |
int | offset | |||
) |
virtual KProtocolInfo::~KProtocolInfo | ( | ) | [virtual] |
Member Function Documentation
static bool KProtocolInfo::canCopyFromFile | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::canCopyFromFile | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can copy files/objects directly from the filesystem itself.
If not, the application will read files from the filesystem using the file-protocol and pass the data on to the destination protocol.
This corresponds to the "copyFromFile=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can copy files from the local file system
Definition at line 193 of file kprotocolinfo.cpp.
static bool KProtocolInfo::canCopyToFile | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::canCopyToFile | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can copy files/objects directly to the filesystem itself.
If not, the application will receive the data from the source protocol and store it in the filesystem using the file-protocol.
This corresponds to the "copyToFile=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can copy files to the local file system
Definition at line 203 of file kprotocolinfo.cpp.
bool KProtocolInfo::canDeleteRecursive | ( | ) | const [protected] |
bool KProtocolInfo::canDeleteRecursive | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can recursively delete directories by itself.
If not (the usual case) then KIO will list the directory and delete files and empty directories one by one.
This corresponds to the "deleteRecursive=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can delete non-empty directories by itself.
- Since:
- 3.4
Definition at line 231 of file kprotocolinfo.cpp.
bool KProtocolInfo::canRenameFromFile | ( | ) | const [protected] |
bool KProtocolInfo::canRenameFromFile | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can rename (i.e.
move fast) files/objects directly from the filesystem itself. If not, the application will read files from the filesystem using the file-protocol and pass the data on to the destination protocol.
This corresponds to the "renameFromFile=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can rename/move files from the local file system
- Since:
- 3.4
Definition at line 212 of file kprotocolinfo.cpp.
bool KProtocolInfo::canRenameToFile | ( | ) | const [protected] |
bool KProtocolInfo::canRenameToFile | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can rename (i.e.
move fast) files/objects directly to the filesystem itself. If not, the application will receive the data from the source protocol and store it in the filesystem using the file-protocol.
This corresponds to the "renameToFile=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can rename files to the local file system
- Since:
- 3.4
Definition at line 222 of file kprotocolinfo.cpp.
static QStringList KProtocolInfo::capabilities | ( | const QString & | protocol | ) | [static] |
Returns the list of capabilities provided by the kioslave implementing this protocol.
This corresponds to the "Capabilities=" field in the protocol description file.
The capability names are not defined globally, they are up to each slave implementation. For example when adding support for a new special command for mounting, one would add the string "Mount" to the capabilities list, and applications could check for that string before sending a special() command that would otherwise do nothing on older kioslave implementations.
- Parameters:
-
protocol the protocol to check
- Returns:
- the list of capabilities.
- Since:
- 3.3
Returns the name of the config file associated with the specified protocol.
This is useful if two similar protocols need to share a single config file, e.g. http and https.
This corresponds to the "config=" field in the protocol description file. The default is the protocol name, see name()
- Parameters:
-
protocol the protocol to check
- Returns:
- the config file, or null if unknown
QString KProtocolInfo::defaultMimetype | ( | const KURL & | url | ) | [static] |
Returns default mimetype for this URL based on the protocol.
This corresponds to the "defaultMimetype=" field in the protocol description file.
- Parameters:
-
url the url to check
- Returns:
- the default mime type of the protocol, or null if unknown
Definition at line 249 of file kprotocolinfo.cpp.
static bool KProtocolInfo::determineMimetypeFromExtension | ( | const QString & | protocol | ) | [static] |
Returns whether mimetypes can be determined based on extension for this protocol.
For some protocols, e.g. http, the filename extension in the URL can not be trusted to truly reflect the file type.
This corresponds to the "determineMimetypeFromExtension=" field in the protocol description file. Valid values for this field are "true" (default) or "false".
- Parameters:
-
protocol the protocol to check
- Returns:
- true if the mime types can be determined by extension
Returns the documentation path for the specified protocol.
This corresponds to the "DocPath=" field in the protocol description file.
- Parameters:
-
protocol the protocol to check
- Returns:
- the docpath of the protocol, or null if unknown
- Since:
- 3.2
Returns the library / executable to open for the protocol protocol
Example : "kio_ftp", meaning either the executable "kio_ftp" or the library "kio_ftp.la" (recommended), whichever is available.
This corresponds to the "exec=" field in the protocol description file.
- Parameters:
-
protocol the protocol to check
- Returns:
- the executable of library to open, or QString::null for unsupported protocols
- See also:
- KURL::protocol()
static ExtraFieldList KProtocolInfo::extraFields | ( | const KURL & | url | ) | [static] |
Definition of extra fields in the UDS entries, returned by a listDir operation.
This corresponds to the "ExtraNames=" and "ExtraTypes=" fields in the protocol description file. Those two lists should be separated with ',' in the protocol description file. See ExtraField for details about names and types
- Since:
- 3.2
FileNameUsedForCopying KProtocolInfo::fileNameUsedForCopying | ( | ) | const [protected] |
KProtocolInfo::FileNameUsedForCopying KProtocolInfo::fileNameUsedForCopying | ( | const KURL & | url | ) | [static] |
This setting defines the strategy to use for generating a filename, when copying a file or directory to another directory.
By default the destination filename is made out of the filename in the source URL. However if the ioslave displays names that are different from the filename of the URL (e.g. kio_fonts shows Arial for arial.ttf, or kio_trash shows foo.txt and uses some internal URL), using Name means that the display name (UDS_NAME) will be used to as the filename in the destination directory.
This corresponds to the "fileNameUsedForCopying=" field in the protocol description file. Valid values for this field are "Name" or "FromURL" (default).
- Parameters:
-
url the url to check
- Returns:
- how to generate the filename in the destination directory when copying/moving
- Since:
- 3.4
Definition at line 240 of file kprotocolinfo.cpp.
KProtocolInfo * KProtocolInfo::findProtocol | ( | const KURL & | url | ) | [static, protected] |
Definition at line 33 of file kprotocolinfo.cpp.
Returns the name of the icon, associated with the specified protocol.
This corresponds to the "Icon=" field in the protocol description file.
- Parameters:
-
protocol the protocol to check
- Returns:
- the icon of the protocol, or null if unknown
KProtocolInfo::Type KProtocolInfo::inputType | ( | const KURL & | url | ) | [static] |
Returns whether the protocol should be treated as a filesystem or as a stream when reading from it.
This corresponds to the "input=" field in the protocol description file. Valid values for this field are "filesystem", "stream" or "none" (default).
- Parameters:
-
url the url to check
- Returns:
- the input type of the given
url
Definition at line 51 of file kprotocolinfo.cpp.
bool KProtocolInfo::isFilterProtocol | ( | const QString & | protocol | ) | [static] |
Same as above except you can supply just the protocol instead of the whole URL.
Definition at line 84 of file kprotocolinfo.cpp.
bool KProtocolInfo::isFilterProtocol | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can act as a filter protocol.
A filter protocol can operate on data that is passed to it but does not retrieve/store data itself, like gzip. A filter protocol is the opposite of a source protocol.
The "source=" field in the protocol description file determines whether a protocol is a source protocol or a filter protocol. Valid values for this field are "true" (default) for source protocol or "false" for filter protocol.
- Parameters:
-
url the url to check
- Returns:
- true if the protocol is a filter (e.g. gzip), false if the protocol is a helper or source
Definition at line 79 of file kprotocolinfo.cpp.
bool KProtocolInfo::isHelperProtocol | ( | const QString & | protocol | ) | [static] |
Same as above except you can supply just the protocol instead of the whole URL.
Definition at line 99 of file kprotocolinfo.cpp.
bool KProtocolInfo::isHelperProtocol | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can act as a helper protocol.
A helper protocol invokes an external application and does not return a file or stream.
This corresponds to the "helper=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol is a helper protocol (e.g. vnc), false if not (e.g. http)
Definition at line 94 of file kprotocolinfo.cpp.
bool KProtocolInfo::isKnownProtocol | ( | const QString & | protocol | ) | [static] |
Same as above except you can supply just the protocol instead of the whole URL.
Definition at line 114 of file kprotocolinfo.cpp.
bool KProtocolInfo::isKnownProtocol | ( | const KURL & | url | ) | [static] |
Returns whether a protocol is installed that is able to handle url
.
- Parameters:
-
url the url to check
- Returns:
- true if the protocol is known
- See also:
- name()
Definition at line 109 of file kprotocolinfo.cpp.
static bool KProtocolInfo::isSourceProtocol | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::isSourceProtocol | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can act as a source protocol.
A source protocol retrieves data from or stores data to the location specified by a URL. A source protocol is the opposite of a filter protocol.
The "source=" field in the protocol description file determines whether a protocol is a source protocol or a filter protocol.
- Parameters:
-
url the url to check
- Returns:
- true if the protocol is a source of data (e.g. http), false if the protocol is a filter (e.g. gzip)
Definition at line 70 of file kprotocolinfo.cpp.
virtual bool KProtocolInfo::isValid | ( | ) | const [inline, virtual] |
Returns whether the protocol description file is valid.
- Returns:
- true if valid, false otherwise
Definition at line 64 of file kprotocolinfo.h.
static QStringList KProtocolInfo::listing | ( | const QString & | protocol | ) | [static] |
- Deprecated:
- Returns the list of fields this protocol returns when listing The current possibilities are Name, Type, Size, Date, AccessDate, Access, Owner, Group, Link, URL, MimeType
QStringList KProtocolInfo::listing | ( | const KURL & | url | ) | [static] |
Returns the list of fields this protocol returns when listing The current possibilities are Name, Type, Size, Date, AccessDate, Access, Owner, Group, Link, URL, MimeType as well as Extra1, Extra2 etc.
for extra fields (see extraFields).
This corresponds to the "listing=" field in the protocol description file. The supported fields should be separated with ',' in the protocol description file.
- Parameters:
-
url the url to check
- Returns:
- a list of field names
Definition at line 130 of file kprotocolinfo.cpp.
virtual void KProtocolInfo::load | ( | QDataStream & | ) | [virtual] |
For internal use only.
Load the protocol info from a stream.
static int KProtocolInfo::maxSlaves | ( | const QString & | protocol | ) | [static] |
Returns the soft limit on the number of slaves for this protocol.
This limits the number of slaves used for a single operation, note that multiple operations may result in a number of instances that exceeds this soft limit.
This corresponds to the "maxInstances=" field in the protocol description file. The default is 1.
- Parameters:
-
protocol the protocol to check
- Returns:
- the maximum number of slaves, or 1 if unknown
virtual QString KProtocolInfo::name | ( | ) | const [inline, virtual] |
Returns the name of the protocol.
This corresponds to the "protocol=" field in the protocol description file.
- Returns:
- the name of the protocol
- See also:
- KURL::protocol()
Definition at line 74 of file kprotocolinfo.h.
KProtocolInfo::Type KProtocolInfo::outputType | ( | const KURL & | url | ) | [static] |
Returns whether the protocol should be treated as a filesystem or as a stream when writing to it.
This corresponds to the "output=" field in the protocol description file. Valid values for this field are "filesystem", "stream" or "none" (default).
- Parameters:
-
url the url to check
- Returns:
- the output type of the given
url
Definition at line 60 of file kprotocolinfo.cpp.
Returns the protocol class for the specified protocol.
This corresponds to the "Class=" field in the protocol description file.
The following classes are defined:
- ":internet" for common internet protocols
- ":local" for protocols that access local resources
- Parameters:
-
protocol the protocol to check
- Returns:
- the class of the protocol, or null if unknown
- Since:
- 3.2
static QStringList KProtocolInfo::protocols | ( | ) | [static] |
Returns list of all known protocols.
- Returns:
- a list of all known protocols
Returns the name of the protocol through which the request will be routed if proxy support is enabled.
A good example of this is the ftp protocol for which proxy support is commonly handled by the http protocol.
This corresponds to the "ProxiedBy=" in the protocol description file.
- Since:
- 3.3
virtual void KProtocolInfo::save | ( | QDataStream & | ) | [virtual] |
For internal use only.
Save the protocol info to a stream.
static bool KProtocolInfo::showFilePreview | ( | const QString & | protocol | ) | [static] |
Returns whether file previews should be shown for the specified protocol.
This corresponds to the "ShowPreviews=" field in the protocol description file.
By default previews are shown if protocolClass is :local.
- Parameters:
-
protocol the protocol to check
- Returns:
- true if previews should be shown by default, false otherwise
- Since:
- 3.2
static bool KProtocolInfo::supportsDeleting | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsDeleting | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can delete files/objects.
This corresponds to the "deleting=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol supports deleting
Definition at line 166 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsLinking | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsLinking | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can create links between files/objects.
This corresponds to the "linking=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol supports linking
Definition at line 175 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsListing | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsListing | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can list files/objects.
If a protocol supports listing it can be browsed in e.g. file-dialogs and konqueror.
Whether a protocol supports listing is determined by the "listing=" field in the protocol description file. If the protocol support listing it should list the fields it provides in this field. If the protocol does not support listing this field should remain empty (default.)
- Parameters:
-
url the url to check
- Returns:
- true if the protocol support listing
- See also:
- listing()
Definition at line 121 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsMakeDir | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsMakeDir | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can create directories/folders.
This corresponds to the "makedir=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol can create directories
Definition at line 157 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsMoving | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsMoving | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can move files/objects between different locations.
This corresponds to the "moving=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol supports moving
Definition at line 184 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsReading | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsReading | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can retrieve data from URLs.
This corresponds to the "reading=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if it is possible to read from the URL
Definition at line 139 of file kprotocolinfo.cpp.
static bool KProtocolInfo::supportsWriting | ( | const QString & | protocol | ) | [static] |
bool KProtocolInfo::supportsWriting | ( | const KURL & | url | ) | [static] |
Returns whether the protocol can store data to URLs.
This corresponds to the "writing=" field in the protocol description file. Valid values for this field are "true" or "false" (default).
- Parameters:
-
url the url to check
- Returns:
- true if the protocol supports writing
Definition at line 148 of file kprotocolinfo.cpp.
static KURL::URIMode KProtocolInfo::uriParseMode | ( | const QString & | protocol | ) | [static] |
Returns the suggested URI parsing mode for the KURL parser.
This corresponds to the "URIMode=" field in the protocol description file.
The following parsing modes are defined:
- "url" for a standards compliant URL
- "rawuri" for a non-conformant URI for which URL parsing would be meaningless
- "mailto" for a mailto style URI (the path part contains only an email address)
- Parameters:
-
protocol the protocol to check
- Returns:
- the suggested parsing mode, or KURL::Auto if unspecified
- Since:
- 3.2
virtual void KProtocolInfo::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
bool KProtocolInfo::m_canCopyFromFile [protected] |
Definition at line 667 of file kprotocolinfo.h.
bool KProtocolInfo::m_canCopyToFile [protected] |
Definition at line 668 of file kprotocolinfo.h.
QString KProtocolInfo::m_config [protected] |
Definition at line 669 of file kprotocolinfo.h.
QString KProtocolInfo::m_defaultMimetype [protected] |
Definition at line 664 of file kprotocolinfo.h.
bool KProtocolInfo::m_determineMimetypeFromExtension [protected] |
Definition at line 665 of file kprotocolinfo.h.
QString KProtocolInfo::m_exec [protected] |
Definition at line 651 of file kprotocolinfo.h.
QString KProtocolInfo::m_icon [protected] |
Definition at line 666 of file kprotocolinfo.h.
Type KProtocolInfo::m_inputType [protected] |
Definition at line 652 of file kprotocolinfo.h.
bool KProtocolInfo::m_isHelperProtocol [protected] |
Definition at line 656 of file kprotocolinfo.h.
bool KProtocolInfo::m_isSourceProtocol [protected] |
Definition at line 655 of file kprotocolinfo.h.
QStringList KProtocolInfo::m_listing [protected] |
Definition at line 654 of file kprotocolinfo.h.
int KProtocolInfo::m_maxSlaves [protected] |
Definition at line 670 of file kprotocolinfo.h.
QString KProtocolInfo::m_name [protected] |
Definition at line 650 of file kprotocolinfo.h.
Type KProtocolInfo::m_outputType [protected] |
Definition at line 653 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsDeleting [protected] |
Definition at line 661 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsLinking [protected] |
Definition at line 662 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsListing [protected] |
Definition at line 657 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsMakeDir [protected] |
Definition at line 660 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsMoving [protected] |
Definition at line 663 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsReading [protected] |
Definition at line 658 of file kprotocolinfo.h.
bool KProtocolInfo::m_supportsWriting [protected] |
Definition at line 659 of file kprotocolinfo.h.
The documentation for this class was generated from the following files: