kio
KServiceGroup Class Reference
KServiceGroup represents a group of service, for example screensavers. More...
#include <kservicegroup.h>
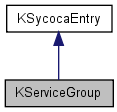
Public Types | |
typedef QValueList< SPtr > | List |
typedef KSharedPtr< KServiceGroup > | Ptr |
typedef KSharedPtr< KSycocaEntry > | SPtr |
Public Member Functions | |
bool | allowInline () const |
QString | baseGroupName () const |
QString | caption () const |
int | childCount () |
QString | comment () const |
QString | directoryEntryPath () const |
virtual List | entries (bool sorted=false) |
virtual List | entries (bool sorted, bool excludeNoDisplay) |
List | entries (bool sorted, bool excludeNoDisplay, bool allowSeparators, bool sortByGenericName=false) |
QString | icon () const |
bool | inlineAlias () const |
int | inlineValue () const |
bool | isValid () const |
KServiceGroup (QDataStream &_str, int offset, bool deep) | |
KServiceGroup (const QString &_fullpath, const QString &_relpath) | |
KServiceGroup (const QString &name) | |
QStringList | layoutInfo () const |
virtual void | load (QDataStream &) |
virtual QString | name () const |
bool | noDisplay () const |
void | parseAttribute (const QString &item, bool &showEmptyMenu, bool &showInline, bool &showInlineHeader, bool &showInlineAlias, int &inlineValue) |
virtual QString | relPath () const |
virtual void | save (QDataStream &) |
void | setAllowInline (bool _b) |
void | setInlineAlias (bool _b) |
void | setInlineValue (int _val) |
void | setLayoutInfo (const QStringList &layout) |
void | setShowEmptyMenu (bool b) |
void | setShowInlineHeader (bool _b) |
bool | showEmptyMenu () const |
bool | showInlineHeader () const |
QStringList | suppressGenericNames () const |
virtual | ~KServiceGroup () |
Static Public Member Functions | |
static Ptr | baseGroup (const QString &baseGroupName) |
static Ptr | childGroup (const QString &parent) |
static Ptr | group (const QString &relPath) |
static Ptr | root () |
Protected Member Functions | |
void | addEntry (KSycocaEntry *entry) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_bDeep |
int | m_childCount |
List | m_serviceList |
QString | m_strBaseGroupName |
QString | m_strCaption |
QString | m_strComment |
QString | m_strIcon |
Detailed Description
KServiceGroup represents a group of service, for example screensavers.This class is typically used like this:
// Lookup screensaver group KServiceGroup::Ptr group = KServiceGroup::baseGroup("screensavers"); if (!group || !group->isValid()) return; KServiceGroup::List list = group->entries(); // Iterate over all entries in the group for( KServiceGroup::List::ConstIterator it = list.begin(); it != list.end(); it++) { KSycocaEntry *p = (*it); if (p->isType(KST_KService)) { KService *s = static_cast<KService *>(p); printf("Name = %s\n", s->name().latin1()); } else if (p->isType(KST_KServiceGroup)) { KServiceGroup *g = static_cast<KServiceGroup *>(p); // Sub group ... } }
Definition at line 67 of file kservicegroup.h.
Member Typedef Documentation
typedef QValueList<SPtr> KServiceGroup::List |
Definition at line 75 of file kservicegroup.h.
typedef KSharedPtr<KServiceGroup> KServiceGroup::Ptr |
Definition at line 73 of file kservicegroup.h.
typedef KSharedPtr<KSycocaEntry> KServiceGroup::SPtr |
Definition at line 74 of file kservicegroup.h.
Constructor & Destructor Documentation
KServiceGroup::KServiceGroup | ( | const QString & | name | ) |
Construct a dummy servicegroup indexed with name
.
- Parameters:
-
name the name of the service group
- Since:
- 3.1
Definition at line 46 of file kservicegroup.cpp.
Construct a service and take all informations from a config file.
- Parameters:
-
_fullpath full path to the config file _relpath relative path to the config file
Definition at line 54 of file kservicegroup.cpp.
KServiceGroup::KServiceGroup | ( | QDataStream & | _str, | |
int | offset, | |||
bool | deep | |||
) |
For internal use only.
construct a service from a stream. The stream must already be positionned at the correct offset
Definition at line 106 of file kservicegroup.cpp.
KServiceGroup::~KServiceGroup | ( | ) | [virtual] |
Definition at line 114 of file kservicegroup.cpp.
Member Function Documentation
void KServiceGroup::addEntry | ( | KSycocaEntry * | entry | ) | [protected] |
For internal use only.
Add a service to this group
Definition at line 249 of file kservicegroup.cpp.
bool KServiceGroup::allowInline | ( | ) | const |
- Returns:
- true if we allow to inline menu.
- Since:
- 3.5
Definition at line 186 of file kservicegroup.cpp.
KServiceGroup::Ptr KServiceGroup::baseGroup | ( | const QString & | baseGroupName | ) | [static] |
Returns the group for the given baseGroupName.
Can return 0L if the directory (or the .directory file) was deleted.
- Returns:
- the base group with the given name, or 0 if not available.
Definition at line 637 of file kservicegroup.cpp.
QString KServiceGroup::baseGroupName | ( | ) | const [inline] |
Returns a non-empty string if the group is a special base group.
By default, "Settings/" is the kcontrol base group ("settings") and "System/Screensavers/" is the screensavers base group ("screensavers"). This allows moving the groups without breaking those apps.
The base group is defined by the X-KDE-BaseGroup key in the .directory file.
- Returns:
- the base group name, or null if no base group
Definition at line 251 of file kservicegroup.h.
QString KServiceGroup::caption | ( | ) | const [inline] |
Returns the caption of this group.
- Returns:
- the caption of this group
Definition at line 121 of file kservicegroup.h.
int KServiceGroup::childCount | ( | ) |
Returns the total number of displayable services in this group and any of its subgroups.
- Returns:
- the number of child services
Definition at line 119 of file kservicegroup.cpp.
KServiceGroup::Ptr KServiceGroup::childGroup | ( | const QString & | parent | ) | [static] |
Returns the group of services that have X-KDE-ParentApp equal to parent
(siblings).
- Parameters:
-
parent the name of the service's parent
- Returns:
- the services group
- Since:
- 3.1
Definition at line 656 of file kservicegroup.cpp.
QString KServiceGroup::comment | ( | ) | const [inline] |
Returns the comment about this service group.
- Returns:
- the descriptive comment for the group, if there is one, or QString::null if not set
Definition at line 135 of file kservicegroup.h.
QString KServiceGroup::directoryEntryPath | ( | ) | const |
Returns a path to the .directory file describing this service group.
The path is either absolute or relative to the "apps" resource.
- Since:
- 3.2
Definition at line 662 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted = false |
) | [virtual] |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters:
-
sorted true to sort items
- Returns:
- the list of entried
Definition at line 293 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, | |
bool | excludeNoDisplay | |||
) | [virtual] |
Definition at line 299 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, | |
bool | excludeNoDisplay, | |||
bool | allowSeparators, | |||
bool | sortByGenericName = false | |||
) |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters:
-
sorted true to sort items excludeNoDisplay true to exclude items marked "NoDisplay" allowSeparators true to allow separator items to be included sortByGenericName true to sort GenericName+Name instead of Name+GenericName
- Returns:
- the list of entries
- Since:
- 3.2
Definition at line 313 of file kservicegroup.cpp.
KServiceGroup::Ptr KServiceGroup::group | ( | const QString & | relPath | ) | [static] |
Returns the group with the given relative path.
- Parameters:
-
relPath the path of the service group
- Returns:
- the group with the given relative path name.
Definition at line 649 of file kservicegroup.cpp.
QString KServiceGroup::icon | ( | ) | const [inline] |
Returns the name of the icon associated with the group.
- Returns:
- the name of the icon associated with the group, or QString::null if not set
Definition at line 128 of file kservicegroup.h.
bool KServiceGroup::inlineAlias | ( | ) | const |
- Returns:
- true to show an inline alias item into menu
- Since:
- 3.5
Definition at line 156 of file kservicegroup.cpp.
int KServiceGroup::inlineValue | ( | ) | const |
bool KServiceGroup::isValid | ( | ) | const [inline] |
Checks whether the entry is valid, returns always true.
- Returns:
- true
Definition at line 103 of file kservicegroup.h.
QStringList KServiceGroup::layoutInfo | ( | ) | const |
For internal use only.
Returns information related to the layout of services in this group.
Definition at line 631 of file kservicegroup.cpp.
void KServiceGroup::load | ( | QDataStream & | s | ) | [virtual] |
For internal use only.
Load the service from a stream.
Definition at line 206 of file kservicegroup.cpp.
virtual QString KServiceGroup::name | ( | ) | const [inline, virtual] |
Name used for indexing.
- Returns:
- the service group's name
Definition at line 109 of file kservicegroup.h.
bool KServiceGroup::noDisplay | ( | ) | const |
Returns true if the NoDisplay flag was set, i.e.
if this group should be hidden from menus, while still being in ksycoca.
- Returns:
- true to hide this service group, false to display it
- Since:
- 3.1
Definition at line 196 of file kservicegroup.cpp.
void KServiceGroup::parseAttribute | ( | const QString & | item, | |
bool & | showEmptyMenu, | |||
bool & | showInline, | |||
bool & | showInlineHeader, | |||
bool & | showInlineAlias, | |||
int & | inlineValue | |||
) |
This function parse attributes into menu.
- Since:
- 3.5
Definition at line 593 of file kservicegroup.cpp.
virtual QString KServiceGroup::relPath | ( | ) | const [inline, virtual] |
Returns the relative path of the service group.
- Returns:
- the service group's relative path
Definition at line 115 of file kservicegroup.h.
KServiceGroup::Ptr KServiceGroup::root | ( | ) | [static] |
Returns the root service group.
- Returns:
- the root service group
Definition at line 643 of file kservicegroup.cpp.
void KServiceGroup::save | ( | QDataStream & | s | ) | [virtual] |
For internal use only.
Save the service to a stream.
Definition at line 254 of file kservicegroup.cpp.
void KServiceGroup::setAllowInline | ( | bool | _b | ) |
Definition at line 191 of file kservicegroup.cpp.
void KServiceGroup::setInlineAlias | ( | bool | _b | ) |
Definition at line 161 of file kservicegroup.cpp.
void KServiceGroup::setInlineValue | ( | int | _val | ) |
Definition at line 181 of file kservicegroup.cpp.
void KServiceGroup::setLayoutInfo | ( | const QStringList & | layout | ) |
For internal use only.
Sets information related to the layout of services in this group.
Definition at line 626 of file kservicegroup.cpp.
void KServiceGroup::setShowEmptyMenu | ( | bool | b | ) |
Definition at line 166 of file kservicegroup.cpp.
void KServiceGroup::setShowInlineHeader | ( | bool | _b | ) |
Definition at line 171 of file kservicegroup.cpp.
bool KServiceGroup::showEmptyMenu | ( | ) | const |
Return true if we want to display empty menu entry.
- Returns:
- true to show this service group as menu entry is empty, false to hide it
- Since:
- 3.4
Definition at line 151 of file kservicegroup.cpp.
bool KServiceGroup::showInlineHeader | ( | ) | const |
- Returns:
- true to show an inline header into menu
- Since:
- 3.5
Definition at line 146 of file kservicegroup.cpp.
QStringList KServiceGroup::suppressGenericNames | ( | ) | const |
Returns a list of untranslated generic names that should be be supressed when showing this group.
E.g. The group "Games/Arcade" might want to suppress the generic name "Arcade Game" since it's redundant in this particular context.
- Since:
- 3.2
Definition at line 201 of file kservicegroup.cpp.
void KServiceGroup::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 668 of file kservicegroup.cpp.
Member Data Documentation
bool KServiceGroup::m_bDeep [protected] |
Definition at line 307 of file kservicegroup.h.
int KServiceGroup::m_childCount [protected] |
Definition at line 309 of file kservicegroup.h.
List KServiceGroup::m_serviceList [protected] |
Definition at line 306 of file kservicegroup.h.
QString KServiceGroup::m_strBaseGroupName [protected] |
Definition at line 308 of file kservicegroup.h.
QString KServiceGroup::m_strCaption [protected] |
Definition at line 302 of file kservicegroup.h.
QString KServiceGroup::m_strComment [protected] |
Definition at line 304 of file kservicegroup.h.
QString KServiceGroup::m_strIcon [protected] |
Definition at line 303 of file kservicegroup.h.
The documentation for this class was generated from the following files: