kio
KServiceType Class Reference
A service type is the generic notion for a mimetype, a type of service instead of a type of file. More...
#include <kservicetype.h>
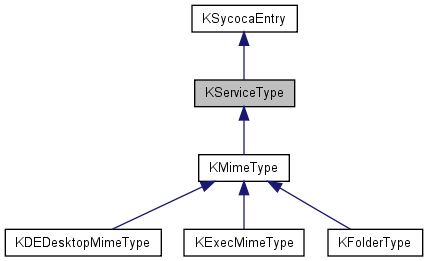
Public Types | |
typedef QValueList< Ptr > | List |
typedef KSharedPtr< KServiceType > | Ptr |
Public Member Functions | |
void | addService (KService::Ptr service) |
QString | comment () const |
QString | desktopEntryPath () const |
QString | icon () const |
bool | inherits (const QString &servTypeName) const |
bool | isDerived () const |
bool | isValid () const |
KServiceType (QDataStream &_str, int offset) | |
KServiceType (KDesktopFile *config) | |
KServiceType (const QString &_fullpath) | |
KServiceType (const QString &_fullpath, const QString &_name, const QString &_icon, const QString &_comment) | |
virtual void | load (QDataStream &) |
QString | name () const |
QString | parentServiceType () const |
Ptr | parentType () |
virtual QVariant | property (const QString &_name) const |
virtual QVariant::Type | propertyDef (const QString &_name) const |
virtual QStringList | propertyDefNames () const |
virtual const QMap< QString, QVariant::Type > & | propertyDefs () const |
virtual QStringList | propertyNames () const |
virtual void | save (QDataStream &) |
KService::List | services () |
virtual | ~KServiceType () |
Static Public Member Functions | |
static List | allServiceTypes () |
static KService::List | offers (const QString &_servicetype) |
static Ptr | serviceType (const QString &_name) |
Protected Member Functions | |
void | init (KDesktopFile *config) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_bDerived:1 |
bool | m_bValid:1 |
QMap< QString, QVariant::Type > | m_mapPropDefs |
QMap< QString, QVariant > | m_mapProps |
QString | m_strComment |
QString | m_strIcon |
QString | m_strName |
Detailed Description
A service type is the generic notion for a mimetype, a type of service instead of a type of file.For instance, KOfficeFilter is a service type. It is associated to services according to the user profile (kuserprofile.h). Service types are stored as desktop files in $KDEHOME/share/servicetypes.
- See also:
- KService
Definition at line 45 of file kservicetype.h.
Member Typedef Documentation
typedef QValueList<Ptr> KServiceType::List |
typedef KSharedPtr<KServiceType> KServiceType::Ptr |
Constructor & Destructor Documentation
KServiceType::KServiceType | ( | const QString & | _fullpath, | |
const QString & | _name, | |||
const QString & | _icon, | |||
const QString & | _comment | |||
) |
Constructor.
You may pass in arguments to create a servicetype with specific properties.
- Parameters:
-
_fullpath the path of the service type's desktop file _name the name of the service type _icon the icon name of the service type (can be null) _comment a comment (can be null)
Definition at line 109 of file kservicetype.cpp.
KServiceType::KServiceType | ( | const QString & | _fullpath | ) |
Construct a service type and take all informations from a config file.
- Parameters:
-
_fullpath path of the desktop file, set to "" if calling from a inherited constructor.
Definition at line 43 of file kservicetype.cpp.
KServiceType::KServiceType | ( | KDesktopFile * | config | ) |
Construct a service type and take all informations from a deskop file.
- Parameters:
-
config the configuration file
Definition at line 51 of file kservicetype.cpp.
KServiceType::KServiceType | ( | QDataStream & | _str, | |
int | offset | |||
) |
For internal use only.
construct a service from a stream. The stream must already be positionned at the correct offset
Definition at line 119 of file kservicetype.cpp.
KServiceType::~KServiceType | ( | ) | [virtual] |
Definition at line 146 of file kservicetype.cpp.
Member Function Documentation
void KServiceType::addService | ( | KService::Ptr | service | ) |
For internal use only.
Register service that provides this service type
Definition at line 346 of file kservicetype.cpp.
KServiceType::List KServiceType::allServiceTypes | ( | ) | [static] |
Returns a list of all the supported servicetypes.
Useful for showing the list of available servicetypes in a listbox, for example. More memory consuming than the ones above, don't use unless really necessary.
- Returns:
- the list of all services
Definition at line 320 of file kservicetype.cpp.
QString KServiceType::comment | ( | ) | const [inline] |
Returns the descriptive comment associated, if any.
- Returns:
- the comment, or QString::null
Reimplemented in KMimeType.
Definition at line 100 of file kservicetype.h.
QString KServiceType::desktopEntryPath | ( | ) | const [inline] |
Returns the relative path to the desktop entry file responsible for this servicetype.
For instance inode/directory.desktop, or kpart.desktop
- Returns:
- the path of the desktop file
Definition at line 114 of file kservicetype.h.
QString KServiceType::icon | ( | ) | const [inline] |
Returns the icon associated with this service type.
Some derived classes offer special functions which take for example an URL and returns a special icon for this URL. An example is KMimeType, KFolderType and others.
- Returns:
- the name of the icon, can be QString::null.
Definition at line 94 of file kservicetype.h.
bool KServiceType::inherits | ( | const QString & | servTypeName | ) | const |
Checks whether this service type is or inherits from servTypeName
.
- Returns:
- true if this servicetype is or inherits from
servTypeName
- Since:
- 3.1
Definition at line 157 of file kservicetype.cpp.
void KServiceType::init | ( | KDesktopFile * | config | ) | [protected] |
bool KServiceType::isDerived | ( | ) | const [inline] |
Checks whether this service type inherits another one.
- Returns:
- true if this service type inherits another one
- See also:
- parentServiceType()
Definition at line 121 of file kservicetype.h.
bool KServiceType::isValid | ( | ) | const [inline] |
Checks whether the service type is valid.
- Returns:
- true if the service is valid (e.g. name is not empty)
Definition at line 158 of file kservicetype.h.
void KServiceType::load | ( | QDataStream & | _str | ) | [virtual] |
For internal use only.
Load ourselves from the data stream.
Reimplemented in KMimeType.
Definition at line 126 of file kservicetype.cpp.
QString KServiceType::name | ( | ) | const [inline] |
Returns the name of this service type.
- Returns:
- the name of the service type
Definition at line 106 of file kservicetype.h.
KService::List KServiceType::offers | ( | const QString & | _servicetype | ) | [static] |
Returns all services supporting the given servicetype name.
This doesn't take care of the user profile. In fact it is used by KServiceTypeProfile, which is used by KTrader, and that's the one you should use.
- Parameters:
-
_servicetype the name of the service type to search
- Returns:
- the list of all services of the given type
Definition at line 251 of file kservicetype.cpp.
QString KServiceType::parentServiceType | ( | ) | const |
If this service type inherits from another service type, return the name of the parent.
- Returns:
- the parent service type, or QString:: null if not set
- See also:
- isDerived()
Definition at line 151 of file kservicetype.cpp.
KServiceType::Ptr KServiceType::parentType | ( | ) |
For internal use only.
Pointer to parent serice type
Definition at line 325 of file kservicetype.cpp.
Returns the requested property.
Some often used properties have convenience access functions like name(), comment() etc.
- Parameters:
-
_name the name of the property
- Returns:
- the property, or invalid if not found
Reimplemented in KMimeType.
Definition at line 174 of file kservicetype.cpp.
QVariant::Type KServiceType::propertyDef | ( | const QString & | _name | ) | const [virtual] |
Returns the type of the property with the given _name
.
- Parameters:
-
_name the name of the property
- Returns:
- the property type, or null if not found
Definition at line 210 of file kservicetype.cpp.
QStringList KServiceType::propertyDefNames | ( | ) | const [virtual] |
Definition at line 219 of file kservicetype.cpp.
Definition at line 169 of file kservicetype.h.
QStringList KServiceType::propertyNames | ( | ) | const [virtual] |
Returns the list of all properties of this service type.
- Returns:
- the list of properties
Reimplemented in KMimeType.
Definition at line 194 of file kservicetype.cpp.
void KServiceType::save | ( | QDataStream & | _str | ) | [virtual] |
For internal use only.
Save ourselves to the data stream.
Reimplemented in KMimeType.
Definition at line 136 of file kservicetype.cpp.
KService::List KServiceType::services | ( | ) |
For internal use only.
List serices that provide this service type
Definition at line 357 of file kservicetype.cpp.
KServiceType::Ptr KServiceType::serviceType | ( | const QString & | _name | ) | [static] |
Returns a pointer to the servicetype '_name' or 0L if the service type is unknown.
VERY IMPORTANT : don't store the result in a KServiceType * !
- Parameters:
-
_name the name of the service type to search
- Returns:
- the pointer to the service type, or 0
Definition at line 230 of file kservicetype.cpp.
void KServiceType::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KMimeType, KFolderType, KDEDesktopMimeType, and KExecMimeType.
Definition at line 365 of file kservicetype.cpp.
Member Data Documentation
bool KServiceType::m_bDerived [protected] |
Definition at line 240 of file kservicetype.h.
bool KServiceType::m_bValid [protected] |
Definition at line 239 of file kservicetype.h.
QMap<QString,QVariant::Type> KServiceType::m_mapPropDefs [protected] |
Definition at line 237 of file kservicetype.h.
QMap<QString,QVariant> KServiceType::m_mapProps [protected] |
Definition at line 236 of file kservicetype.h.
QString KServiceType::m_strComment [protected] |
Definition at line 235 of file kservicetype.h.
QString KServiceType::m_strIcon [protected] |
Definition at line 234 of file kservicetype.h.
QString KServiceType::m_strName [protected] |
Definition at line 233 of file kservicetype.h.
The documentation for this class was generated from the following files: