KParts
KParts::BrowserRun Class Reference
This class extends KRun to provide additional functionality for browsers:. More...
#include <browserrun.h>
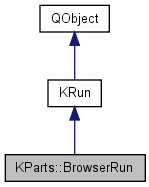
Public Types | |
enum | AskEmbedOrSaveFlags { InlineDisposition = 0, AttachmentDisposition = 1 } |
enum | AskSaveResult { Save, Open, Cancel } |
Public Member Functions | |
BrowserRun (const KURL &url, const KParts::URLArgs &args, KParts::ReadOnlyPart *part, QWidget *window, bool removeReferrer, bool trustedSource, bool hideErrorDialog) | |
BrowserRun (const KURL &url, const KParts::URLArgs &args, KParts::ReadOnlyPart *part, QWidget *window, bool removeReferrer, bool trustedSource) | |
QString | contentDisposition () const |
bool | hideErrorDialog () const |
virtual void | save (const KURL &url, const QString &suggestedFilename) |
bool | serverSuggestsSave () const |
QString | suggestedFilename () const |
KURL | url () const |
virtual | ~BrowserRun () |
Static Public Member Functions | |
static bool | allowExecution (const QString &serviceType, const KURL &url) |
static AskSaveResult | askEmbedOrSave (const KURL &url, const QString &mimeType, const QString &suggestedFilename=QString::null, int flags=0) |
static AskSaveResult | askSave (const KURL &url, KService::Ptr offer, const QString &mimeType, const QString &suggestedFilename=QString::null) |
static bool | isExecutable (const QString &serviceType) |
static bool | isTextExecutable (const QString &serviceType) |
static void | simpleSave (const KURL &url, const QString &suggestedFilename) |
static void | simpleSave (const KURL &url, const QString &suggestedFilename, QWidget *window) |
Protected Types | |
enum | NonEmbeddableResult { Handled, NotHandled, Delayed } |
Protected Slots | |
void | slotBrowserMimetype (KIO::Job *job, const QString &type) |
void | slotBrowserScanFinished (KIO::Job *job) |
void | slotCopyToTempFileResult (KIO::Job *job) |
virtual void | slotStatResult (KIO::Job *job) |
Protected Member Functions | |
virtual void | handleError (KIO::Job *job) |
NonEmbeddableResult | handleNonEmbeddable (const QString &mimeType) |
virtual void | init () |
virtual void | scanFile () |
Protected Attributes | |
KParts::URLArgs | m_args |
bool | m_bRemoveReferrer |
bool | m_bTrustedSource |
KParts::ReadOnlyPart * | m_part |
QString | m_sMimeType |
QString | m_suggestedFilename |
QGuardedPtr< QWidget > | m_window |
Detailed Description
This class extends KRun to provide additional functionality for browsers:.
- "save or open" dialog boxes
- "save" functionality
- support for HTTP POST (including saving the result to a temp file if opening a separate application)
- warning before launching executables off the web
- custom error handling (i.e. treating errors as HTML pages)
- generation of SSL metadata depending on the previous URL shown by the part
Definition at line 42 of file browserrun.h.
Member Enumeration Documentation
enum KParts::BrowserRun::NonEmbeddableResult [protected] |
NotHandled means that foundMimeType should call KRun::foundMimeType, i.e.
launch an external app.
Definition at line 164 of file browserrun.h.
Constructor & Destructor Documentation
BrowserRun::BrowserRun | ( | const KURL & | url, | |
const KParts::URLArgs & | args, | |||
KParts::ReadOnlyPart * | part, | |||
QWidget * | window, | |||
bool | removeReferrer, | |||
bool | trustedSource | |||
) |
- Parameters:
-
url the URL we're probing args URL args - includes data for a HTTP POST, etc. part the part going to open this URL - can be 0L if not created yet window the mainwindow - passed to KIO::Job::setWindow() removeReferrer if true, the "referrer" metadata from args
isn't passed ontrustedSource if false, a warning will be shown before launching an executable Always pass false for trustedSource
, except for local directory views.
Definition at line 42 of file browserrun.cpp.
BrowserRun::BrowserRun | ( | const KURL & | url, | |
const KParts::URLArgs & | args, | |||
KParts::ReadOnlyPart * | part, | |||
QWidget * | window, | |||
bool | removeReferrer, | |||
bool | trustedSource, | |||
bool | hideErrorDialog | |||
) |
- Parameters:
-
url the URL we're probing args URL args - includes data for a HTTP POST, etc. part the part going to open this URL - can be 0L if not created yet window the mainwindow - passed to KIO::Job::setWindow() removeReferrer if true, the "referrer" metadata from args
isn't passed ontrustedSource if false, a warning will be shown before launching an executable. Always pass false for trustedSource
, except for local directory views.hideErrorDialog if true, no dialog will be shown in case of errors.
Definition at line 54 of file browserrun.cpp.
BrowserRun::~BrowserRun | ( | ) | [virtual] |
Definition at line 65 of file browserrun.cpp.
Member Function Documentation
Definition at line 267 of file browserrun.cpp.
BrowserRun::AskSaveResult BrowserRun::askEmbedOrSave | ( | const KURL & | url, | |
const QString & | mimeType, | |||
const QString & | suggestedFilename = QString::null , |
|||
int | flags = 0 | |||
) | [static] |
Similar to askSave() but for the case where the current application is able to embed the url itself (instead of passing it to another app).
- Parameters:
-
url the URL in question mimeType the mimetype of the URL suggestedFilename optional filename suggested by the server flags set to AttachmentDisposition if suggested by the server
- Returns:
- Save, Open or Cancel.
Definition at line 323 of file browserrun.cpp.
BrowserRun::AskSaveResult BrowserRun::askSave | ( | const KURL & | url, | |
KService::Ptr | offer, | |||
const QString & | mimeType, | |||
const QString & | suggestedFilename = QString::null | |||
) | [static] |
Ask the user whether to save or open a url in another application.
- Parameters:
-
url the URL in question offer the application that will be used to open the URL mimeType the mimetype of the URL suggestedFilename optional filename suggested by the server
- Returns:
- Save, Open or Cancel.
Definition at line 300 of file browserrun.cpp.
QString BrowserRun::contentDisposition | ( | ) | const |
- Returns:
- Suggested disposition by the server (e.g. HTTP content-disposition)
- Since:
- 3.5.2
Definition at line 515 of file browserrun.cpp.
void BrowserRun::handleError | ( | KIO::Job * | job | ) | [protected, virtual] |
Called when an error happens.
NOTE: job
could be 0L, if you passed hideErrorDialog=true. The default implementation shows a message box, but only when job != 0 .... It is strongly recommended to reimplement this method if you passed hideErrorDialog=true.
Definition at line 444 of file browserrun.cpp.
BrowserRun::NonEmbeddableResult BrowserRun::handleNonEmbeddable | ( | const QString & | mimeType | ) | [protected] |
Helper for foundMimeType: call this if the mimetype couldn't be embedded.
Definition at line 203 of file browserrun.cpp.
bool BrowserRun::hideErrorDialog | ( | ) | const |
- Returns:
- true if no dialog will be shown in case of errors
Definition at line 510 of file browserrun.cpp.
void BrowserRun::init | ( | ) | [protected, virtual] |
BIC: Obsoleted by KRun::isExecutable( const QString &serviceType );.
Reimplemented from KRun.
Definition at line 505 of file browserrun.cpp.
Definition at line 499 of file browserrun.cpp.
Definition at line 360 of file browserrun.cpp.
void BrowserRun::scanFile | ( | ) | [protected, virtual] |
bool KParts::BrowserRun::serverSuggestsSave | ( | ) | const [inline] |
Definition at line 101 of file browserrun.h.
void BrowserRun::simpleSave | ( | const KURL & | url, | |
const QString & | suggestedFilename, | |||
QWidget * | window | |||
) | [static] |
Definition at line 371 of file browserrun.cpp.
Definition at line 179 of file browserrun.cpp.
void BrowserRun::slotBrowserScanFinished | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 156 of file browserrun.cpp.
void BrowserRun::slotCopyToTempFileResult | ( | KIO::Job * | job | ) | [protected, slot] |
Definition at line 486 of file browserrun.cpp.
void BrowserRun::slotStatResult | ( | KIO::Job * | job | ) | [protected, virtual, slot] |
QString KParts::BrowserRun::suggestedFilename | ( | ) | const [inline] |
- Returns:
- Suggested filename given by the server (e.g. HTTP content-disposition filename)
Definition at line 93 of file browserrun.h.
KURL KParts::BrowserRun::url | ( | ) | const [inline] |
Member Data Documentation
KParts::URLArgs KParts::BrowserRun::m_args [protected] |
Definition at line 178 of file browserrun.h.
bool KParts::BrowserRun::m_bRemoveReferrer [protected] |
Definition at line 185 of file browserrun.h.
bool KParts::BrowserRun::m_bTrustedSource [protected] |
Definition at line 186 of file browserrun.h.
KParts::ReadOnlyPart* KParts::BrowserRun::m_part [protected] |
Definition at line 179 of file browserrun.h.
QString KParts::BrowserRun::m_sMimeType [protected] |
Definition at line 184 of file browserrun.h.
QString KParts::BrowserRun::m_suggestedFilename [protected] |
Definition at line 183 of file browserrun.h.
QGuardedPtr<QWidget> KParts::BrowserRun::m_window [protected] |
Definition at line 180 of file browserrun.h.
The documentation for this class was generated from the following files: