kio
KIO::Job Class Reference
The base class for all jobs. More...
#include <jobclasses.h>
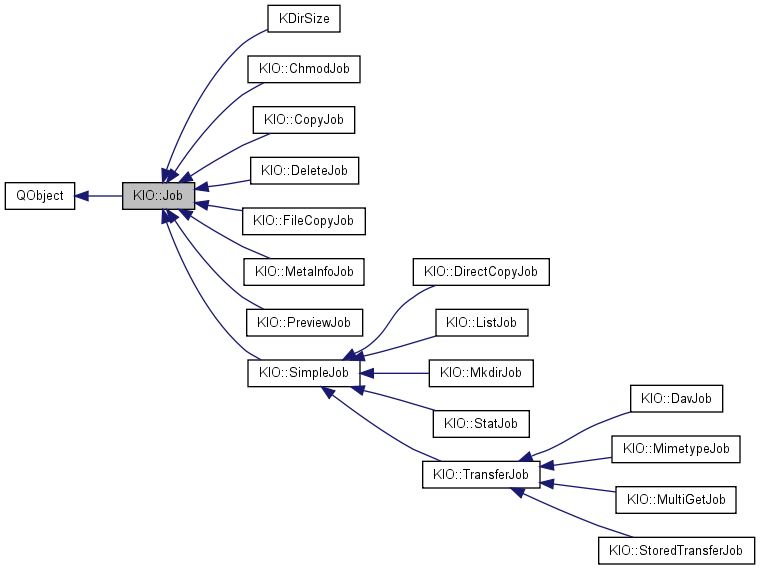
Signals | |
void | canceled (KIO::Job *job) |
void | connected (KIO::Job *job) |
void | infoMessage (KIO::Job *job, const QString &msg) |
void | percent (KIO::Job *job, unsigned long percent) |
void | processedSize (KIO::Job *job, KIO::filesize_t size) |
void | result (KIO::Job *job) |
void | speed (KIO::Job *job, unsigned long speed) |
void | totalSize (KIO::Job *job, KIO::filesize_t size) |
void | warning (KIO::Job *job, const QString &msg) |
Public Member Functions | |
void | addMetaData (const QMap< QString, QString > &values) |
void | addMetaData (const QString &key, const QString &value) |
QStringList | detailedErrorStrings (const KURL *reqUrl=0L, int method=-1) const |
int | error () const |
QString | errorString () const |
const QString & | errorText () const |
KIO::filesize_t | getProcessedSize () |
bool | isAutoErrorHandlingEnabled () const |
bool | isAutoWarningHandlingEnabled () const |
bool | isInteractive () const |
virtual void | kill (bool quietly=true) |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
int | progressId () const |
QString | queryMetaData (const QString &key) |
void | setAutoErrorHandlingEnabled (bool enable, QWidget *parentWidget=0) |
void | setAutoWarningHandlingEnabled (bool enable) |
void | setInteractive (bool enable) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | setWindow (QWidget *window) |
void | showErrorDialog (QWidget *parent=0L) |
void | updateUserTimestamp (unsigned long time) |
QWidget * | window () const |
virtual | ~Job () |
Protected Types | |
enum | { EF_TransferJobAsync = (1 << 0), EF_TransferJobNeedData = (1 << 1), EF_TransferJobDataSent = (1 << 2), EF_ListJobUnrestricted = (1 << 3) } |
Protected Slots | |
void | slotInfoMessage (KIO::Job *job, const QString &msg) |
virtual void | slotResult (KIO::Job *job) |
void | slotSpeed (KIO::Job *job, unsigned long speed) |
void | slotSpeedTimeout () |
Protected Member Functions | |
virtual void | addSubjob (Job *job, bool inheritMetaData=true) |
void | emitPercent (KIO::filesize_t processedSize, KIO::filesize_t totalSize) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
int & | extraFlags () |
Job (bool showProgressInfo) | |
void | removeSubjob (Job *job, bool mergeMetaData, bool emitResultIfLast) |
virtual void | removeSubjob (Job *job) |
void | setProcessedSize (KIO::filesize_t size) |
unsigned long | userTimestamp () const |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
int | m_error |
QString | m_errorText |
MetaData | m_incomingMetaData |
MetaData | m_outgoingMetaData |
unsigned long | m_percent |
int | m_progressId |
QTimer * | m_speedTimer |
QGuardedPtr< QWidget > | m_window |
QPtrList< Job > | subjobs |
Detailed Description
The base class for all jobs.For all jobs created in an application, the code looks like
KIO::Job * job = KIO::someoperation( some parameters ); connect( job, SIGNAL( result( KIO::Job * ) ), this, SLOT( slotResult( KIO::Job * ) ) );
And slotResult is usually at least:
if ( job->error() ) job->showErrorDialog( this or 0L );
- See also:
- KIO::Scheduler
Definition at line 68 of file jobclasses.h.
Member Enumeration Documentation
anonymous enum [protected] |
For internal use only.
Some extra storage space for jobs that don't have their own private d pointer.
- Enumerator:
-
EF_TransferJobAsync EF_TransferJobNeedData EF_TransferJobDataSent EF_ListJobUnrestricted
Definition at line 500 of file jobclasses.h.
Constructor & Destructor Documentation
Member Function Documentation
Add key/value pairs to the meta data that is sent to the slave.
If a certain key already existed, it will be overridden.
- Parameters:
-
values the meta data to add
- See also:
- setMetaData()
Add key/value pair to the meta data that is sent to the slave.
- Parameters:
-
key the key of the meta data value the value of the meta data
- See also:
- setMetaData()
void Job::addSubjob | ( | Job * | job, | |
bool | inheritMetaData = true | |||
) | [protected, virtual] |
void KIO::Job::canceled | ( | KIO::Job * | job | ) | [signal] |
- Deprecated:
- . Don't use ! Emitted when the job is canceled. Signal result() is emitted as well, and error() is, in this case, ERR_USER_CANCELED.
- Parameters:
-
job the job that emitted this signal
void KIO::Job::connected | ( | KIO::Job * | job | ) | [signal] |
Emitted when the slave successfully connected to the host.
There is no guarantee the slave will send this, and this is currently unused (in the applications).
- Parameters:
-
job the job that emitted this signal
KIO_EXPORT QStringList KIO::Job::detailedErrorStrings | ( | const KURL * | reqUrl = 0L , |
|
int | method = -1 | |||
) | const |
Converts an error code and a non-i18n error message into i18n strings suitable for presentation in a detailed error message box.
- Parameters:
-
reqUrl the request URL that generated this error message method the method that generated this error message (unimplemented)
- Returns:
- the following strings: caption, error + description, causes+solutions
Definition at line 458 of file global.cpp.
void Job::emitPercent | ( | KIO::filesize_t | processedSize, | |
KIO::filesize_t | totalSize | |||
) | [protected] |
void Job::emitResult | ( | ) | [protected] |
void Job::emitSpeed | ( | unsigned long | speed | ) | [protected] |
int KIO::Job::error | ( | ) | const [inline] |
Returns the error code, if there has been an error.
Only call this method from the slot connected to result().
- Returns:
- the error code for this job, 0 if no error. Error codes are defined in KIO::Error.
Definition at line 95 of file jobclasses.h.
KIO_EXPORT QString KIO::Job::errorString | ( | ) | const |
Converts an error code and a non-i18n error message into an error message in the current language.
The low level (non-i18n) error message (usually a url) is put into the translated error message using 1.
Example for errid == ERR_CANNOT_OPEN_FOR_READING:
i18n( "Could not read\n%1" ).arg( errortext );
- Returns:
- the error message and if there is no error, a message telling the user that the app is broken, so check with error() whether there is an error
Definition at line 208 of file global.cpp.
const QString& KIO::Job::errorText | ( | ) | const [inline] |
Returns the error text if there has been an error.
Only call if error is not 0. This is really internal, better use errorString() or errorDialog().
- Returns:
- a string to help understand the error, usually the url related to the error. Only valid if error() is not 0.
Definition at line 111 of file jobclasses.h.
KIO::filesize_t Job::getProcessedSize | ( | ) |
Emitted to display information about this job, as sent by the slave.
Examples of message are "Resolving host", "Connecting to host...", etc.
- Parameters:
-
job the job that emitted this signal msg the info message
bool Job::isAutoErrorHandlingEnabled | ( | ) | const |
Returns whether automatic error handling is enabled or disabled.
- Returns:
- true if automatic error handling is enabled
- See also:
- setAutoErrorHandlingEnabled()
bool Job::isAutoWarningHandlingEnabled | ( | ) | const |
Returns whether automatic warning handling is enabled or disabled.
See also setAutoWarningHandlingEnabled .
- Returns:
- true if automatic warning handling is enabled
- See also:
- setAutoWarningHandlingEnabled()
- Since:
- 3.5
bool Job::isInteractive | ( | ) | const |
Returns whether message display is enabled or disabled.
- Returns:
- true if message display is enabled
- See also:
- setInteractive()
- Since:
- 3.4.1
void Job::kill | ( | bool | quietly = true |
) | [virtual] |
Abort this job.
This kills all subjobs and deletes the job.
- Parameters:
-
quietly if false, Job will emit signal result and ask kio_uiserver to close the progress window. quietly
is set to true for subjobs. Whether applications should call with true or false depends on whether they rely on result being emitted or not.
Reimplemented in KIO::SimpleJob, and KIO::PreviewJob.
Add key/value pairs to the meta data that is sent to the slave.
If a certain key already existed, it will remain unchanged.
- Parameters:
-
values the meta data to merge
- See also:
- setMetaData()
MetaData Job::metaData | ( | ) | const |
MetaData Job::outgoingMetaData | ( | ) | const |
Job * Job::parentJob | ( | ) | const |
Returns the parent job, if there is one.
- Returns:
- the parent job, or 0 if there is none
- See also:
- setParentJob
- Since:
- 3.1
void KIO::Job::percent | ( | KIO::Job * | job, | |
unsigned long | percent | |||
) | [signal] |
Progress signal showing the overall progress of the job This is valid for any kind of job, and allows using a a progress bar very easily.
(see KProgress). Note that this signal is not emitted for finished jobs.
- Parameters:
-
job the job that emitted this signal percent the percentage
void KIO::Job::processedSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [signal] |
Regularly emitted to show the progress of this job (current data size for transfers, entries listed).
- Parameters:
-
job the job that emitted this signal size the processed size in bytes
int KIO::Job::progressId | ( | ) | const [inline] |
Returns the progress id for this job.
- Returns:
- the progress id for this job, as returned by uiserver
Definition at line 101 of file jobclasses.h.
void Job::removeSubjob | ( | Job * | job, | |
bool | mergeMetaData, | |||
bool | emitResultIfLast | |||
) | [protected] |
void Job::removeSubjob | ( | Job * | job | ) | [protected, virtual] |
void KIO::Job::result | ( | KIO::Job * | job | ) | [signal] |
Emitted when the job is finished, in any case (completed, canceled, failed.
..). Use error to know the result.
- Parameters:
-
job the job that emitted this signal
void Job::setAutoErrorHandlingEnabled | ( | bool | enable, | |
QWidget * | parentWidget = 0 | |||
) |
Enable or disable the automatic error handling.
When automatic error handling is enabled and an error occurs, then showErrorDialog() is called with the specified parentWidget
(if supplied) , right before the emission of the result signal.
The default is false.
- Parameters:
-
enable enable or disable automatic error handling parentWidget the parent widget, passed to showErrorDialog. Can be 0 for top-level
void Job::setAutoWarningHandlingEnabled | ( | bool | enable | ) |
Enable or disable the automatic warning handling.
When automatic warning handling is enabled and an error occurs, then a message box is displayed with the warning message
The default is true.
See also isAutoWarningHandlingEnabled , showErrorDialog
- Parameters:
-
enable enable or disable automatic warning handling
- See also:
- isAutoWarningHandlingEnabled()
- Since:
- 3.5
void Job::setInteractive | ( | bool | enable | ) |
Enable or disable the message display from the job.
The default is true.
- Parameters:
-
enable enable or disable message display
- Since:
- 3.4.1
Reimplemented in KIO::CopyJob.
void Job::setMetaData | ( | const KIO::MetaData & | metaData | ) |
Set meta data to be sent to the slave, replacing existing meta data.
- Parameters:
-
metaData the meta data to set
- See also:
- addMetaData()
void Job::setParentJob | ( | Job * | parentJob | ) |
Set the parent Job.
One example use of this is when FileCopyJob calls open_RenameDlg, it must pass the correct progress ID of the parent CopyJob (to hide the progress dialog). You can set the parent job only once. By default a job does not have a parent job.
- Parameters:
-
parentJob the new parent job
- Since:
- 3.1
void Job::setProcessedSize | ( | KIO::filesize_t | size | ) | [protected] |
void Job::setWindow | ( | QWidget * | window | ) |
void Job::showErrorDialog | ( | QWidget * | parent = 0L |
) |
void Job::slotResult | ( | KIO::Job * | job | ) | [protected, virtual, slot] |
Called whenever a subjob finishes.
Default implementation checks for errors and propagates to parent job, then calls removeSubjob. Override if you don't want subjobs errors to be propagated.
- Parameters:
-
job the subjob
- See also:
- result()
Reimplemented in KDirSize, KIO::ChmodJob, KIO::TransferJob, KIO::FileCopyJob, KIO::ListJob, KIO::CopyJob, KIO::DeleteJob, KIO::MetaInfoJob, and KIO::PreviewJob.
void Job::slotSpeed | ( | KIO::Job * | job, | |
unsigned long | speed | |||
) | [protected, slot] |
void Job::slotSpeedTimeout | ( | ) | [protected, slot] |
void KIO::Job::speed | ( | KIO::Job * | job, | |
unsigned long | speed | |||
) | [signal] |
Emitted to display information about the speed of this job.
- Parameters:
-
job the job that emitted this signal speed the speed in bytes/s
void KIO::Job::totalSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [signal] |
Emitted when we know the size of this job (data size for transfers, number of entries for listings).
- Parameters:
-
job the job that emitted this signal size the total size in bytes
void Job::updateUserTimestamp | ( | unsigned long | time | ) |
unsigned long Job::userTimestamp | ( | ) | const [protected] |
void Job::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented in KDirSize, KIO::ChmodJob, KIO::SimpleJob, KIO::StatJob, KIO::MkdirJob, KIO::TransferJob, KIO::MultiGetJob, KIO::MimetypeJob, KIO::FileCopyJob, KIO::ListJob, KIO::CopyJob, KIO::DeleteJob, and KIO::PreviewJob.
Emitted to display a warning about this job, as sent by the slave.
- Parameters:
-
job the job that emitted this signal msg the info message
- Since:
- 3.5
QWidget * Job::window | ( | ) | const |
Member Data Documentation
int KIO::Job::m_error [protected] |
Definition at line 507 of file jobclasses.h.
QString KIO::Job::m_errorText [protected] |
Definition at line 508 of file jobclasses.h.
MetaData KIO::Job::m_incomingMetaData [protected] |
Definition at line 514 of file jobclasses.h.
MetaData KIO::Job::m_outgoingMetaData [protected] |
Definition at line 513 of file jobclasses.h.
unsigned long KIO::Job::m_percent [protected] |
Definition at line 509 of file jobclasses.h.
int KIO::Job::m_progressId [protected] |
Definition at line 510 of file jobclasses.h.
QTimer* KIO::Job::m_speedTimer [protected] |
Definition at line 511 of file jobclasses.h.
QGuardedPtr<QWidget> KIO::Job::m_window [protected] |
Definition at line 512 of file jobclasses.h.
QPtrList<Job> KIO::Job::subjobs [protected] |
Definition at line 506 of file jobclasses.h.
The documentation for this class was generated from the following files: