kio
KIO::SimpleJob Class Reference
A simple job (one url and one command). More...
#include <jobclasses.h>
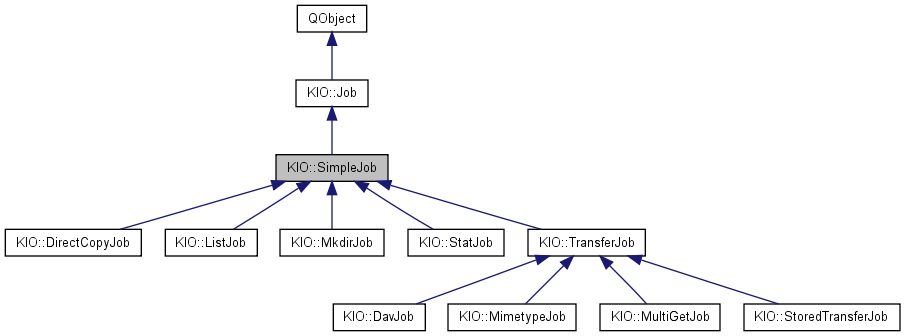
Public Slots | |
virtual void | slotError (int, const QString &) |
void | slotTotalSize (KIO::filesize_t data_size) |
Public Member Functions | |
int | command () const |
virtual void | kill (bool quietly=true) |
virtual void | putOnHold () |
SimpleJob (const KURL &url, int command, const QByteArray &packedArgs, bool showProgressInfo) | |
Slave * | slave () const |
void | slaveDone () |
virtual void | start (Slave *slave) |
const KURL & | url () const |
~SimpleJob () | |
Static Public Member Functions | |
static void | removeOnHold () |
Protected Slots | |
void | slotConnected () |
virtual void | slotFinished () |
void | slotInfoMessage (const QString &s) |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
void | slotNeedProgressId () |
void | slotProcessedSize (KIO::filesize_t data_size) |
void | slotSpeed (unsigned long speed) |
void | slotWarning (const QString &) |
Protected Member Functions | |
void | storeSSLSessionFromJob (const KURL &m_redirectionURL) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
int | m_command |
QByteArray | m_packedArgs |
Slave * | m_slave |
KURL | m_subUrl |
KIO::filesize_t | m_totalSize |
KURL | m_url |
Detailed Description
A simple job (one url and one command).This is the base class for all jobs that are scheduled. Other jobs are high-level jobs (CopyJob, DeleteJob, FileCopyJob...) that manage subjobs but aren't scheduled directly.
Definition at line 528 of file jobclasses.h.
Constructor & Destructor Documentation
SimpleJob::SimpleJob | ( | const KURL & | url, | |
int | command, | |||
const QByteArray & | packedArgs, | |||
bool | showProgressInfo | |||
) |
Creates a new simple job.
You don't need to use this constructor, unless you create a new job that inherits from SimpleJob.
- Parameters:
-
url the url of the job command the command of the job packedArgs the arguments showProgressInfo true to show progress information to the user
Member Function Documentation
int KIO::SimpleJob::command | ( | ) | const [inline] |
void SimpleJob::kill | ( | bool | quietly = true |
) | [virtual] |
void SimpleJob::putOnHold | ( | ) | [virtual] |
void SimpleJob::removeOnHold | ( | ) | [static] |
Slave* KIO::SimpleJob::slave | ( | ) | const [inline] |
void SimpleJob::slaveDone | ( | ) |
void SimpleJob::slotConnected | ( | ) | [protected, slot] |
void SimpleJob::slotError | ( | int | error, | |
const QString & | errorText | |||
) | [virtual, slot] |
void SimpleJob::slotFinished | ( | ) | [protected, virtual, slot] |
Called when the slave marks the job as finished.
Reimplemented in KIO::DavJob, KIO::StatJob, KIO::MkdirJob, KIO::TransferJob, KIO::MultiGetJob, KIO::MimetypeJob, and KIO::ListJob.
void SimpleJob::slotInfoMessage | ( | const QString & | s | ) | [protected, slot] |
void SimpleJob::slotMetaData | ( | const KIO::MetaData & | _metaData | ) | [protected, virtual, slot] |
MetaData from the slave is received.
- Parameters:
-
_metaData the meta data
- See also:
- metaData()
Reimplemented in KIO::StatJob, KIO::TransferJob, and KIO::ListJob.
void SimpleJob::slotNeedProgressId | ( | ) | [protected, slot] |
void SimpleJob::slotProcessedSize | ( | KIO::filesize_t | data_size | ) | [protected, slot] |
Forward signal from the slave.
- Parameters:
-
data_size the processed size in bytes
- See also:
- processedSize()
void SimpleJob::slotSpeed | ( | unsigned long | speed | ) | [protected, slot] |
void SimpleJob::slotTotalSize | ( | KIO::filesize_t | data_size | ) | [slot] |
void SimpleJob::slotWarning | ( | const QString & | errorText | ) | [protected, slot] |
void SimpleJob::start | ( | Slave * | slave | ) | [virtual] |
For internal use only.
Called by the scheduler when a slave gets to work on this job.
Reimplemented in KIO::StatJob, KIO::MkdirJob, KIO::DirectCopyJob, KIO::TransferJob, KIO::MultiGetJob, KIO::MimetypeJob, and KIO::ListJob.
void SimpleJob::storeSSLSessionFromJob | ( | const KURL & | m_redirectionURL | ) | [protected] |
const KURL& KIO::SimpleJob::url | ( | ) | const [inline] |
void SimpleJob::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Reimplemented from KIO::Job.
Reimplemented in KIO::StatJob, KIO::MkdirJob, KIO::TransferJob, KIO::MultiGetJob, KIO::MimetypeJob, and KIO::ListJob.
Member Data Documentation
int KIO::SimpleJob::m_command [protected] |
Definition at line 668 of file jobclasses.h.
QByteArray KIO::SimpleJob::m_packedArgs [protected] |
Definition at line 665 of file jobclasses.h.
Slave* KIO::SimpleJob::m_slave [protected] |
Definition at line 664 of file jobclasses.h.
KURL KIO::SimpleJob::m_subUrl [protected] |
Definition at line 667 of file jobclasses.h.
KIO::filesize_t KIO::SimpleJob::m_totalSize [protected] |
Definition at line 669 of file jobclasses.h.
KURL KIO::SimpleJob::m_url [protected] |
Definition at line 666 of file jobclasses.h.
The documentation for this class was generated from the following files: