kio
KIO::FileCopyJob Class Reference
The FileCopyJob copies data from one place to another. More...
#include <jobclasses.h>
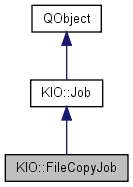
Public Slots | |
void | slotData (KIO::Job *, const QByteArray &data) |
void | slotDataReq (KIO::Job *, QByteArray &data) |
void | slotMimetype (KIO::Job *, const QString &type) |
void | slotStart () |
Signals | |
void | mimetype (KIO::Job *job, const QString &type) |
Public Member Functions | |
KURL | destURL () const |
FileCopyJob (const KURL &src, const KURL &dest, int permissions, bool move, bool overwrite, bool resume, bool showProgressInfo) | |
void | setModificationTime (time_t mtime) |
void | setSourceSize (off_t size) KDE_DEPRECATED |
void | setSourceSize64 (KIO::filesize_t size) |
KURL | srcURL () const |
~FileCopyJob () | |
Protected Slots | |
void | slotCanResume (KIO::Job *job, KIO::filesize_t offset) |
void | slotPercent (KIO::Job *job, unsigned long pct) |
void | slotProcessedSize (KIO::Job *job, KIO::filesize_t size) |
virtual void | slotResult (KIO::Job *job) |
void | slotTotalSize (KIO::Job *job, KIO::filesize_t size) |
Protected Member Functions | |
void | connectSubjob (SimpleJob *job) |
void | startCopyJob (const KURL &slave_url) |
void | startCopyJob () |
void | startDataPump () |
void | startRenameJob (const KURL &slave_url) |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
QByteArray | m_buffer |
bool | m_canResume:1 |
SimpleJob * | m_copyJob |
KURL | m_dest |
TransferJob * | m_getJob |
bool | m_move:1 |
SimpleJob * | m_moveJob |
bool | m_overwrite:1 |
int | m_permissions |
TransferJob * | m_putJob |
bool | m_resume:1 |
bool | m_resumeAnswerSent:1 |
KURL | m_src |
KIO::filesize_t | m_totalSize |
Detailed Description
The FileCopyJob copies data from one place to another.
- See also:
- KIO::file_copy()
Definition at line 1250 of file jobclasses.h.
Constructor & Destructor Documentation
FileCopyJob::FileCopyJob | ( | const KURL & | src, | |
const KURL & | dest, | |||
int | permissions, | |||
bool | move, | |||
bool | overwrite, | |||
bool | resume, | |||
bool | showProgressInfo | |||
) |
Do not create a FileCopyJob directly.
Use KIO::file_move() or KIO::file_copy() instead.
- Parameters:
-
src the source URL dest the destination URL permissions the permissions of the resulting resource move true to move, false to copy overwrite true to allow overwriting, false otherwise resume true to resume an operation, false otherwise showProgressInfo true to show progress information to the user
Member Function Documentation
void FileCopyJob::connectSubjob | ( | SimpleJob * | job | ) | [protected] |
KURL KIO::FileCopyJob::destURL | ( | ) | const [inline] |
Returns the destination URL.
- Returns:
- the destination URL
Definition at line 1301 of file jobclasses.h.
Mimetype determined during a file copy.
This is never emitted during a move, and might not be emitted during a copy, depending on the slave.
- Parameters:
-
job the job that emitted this signal type the mime type
- Since:
- 3.5.7
void FileCopyJob::setModificationTime | ( | time_t | mtime | ) |
Sets the modification time of the file.
Note that this is ignored if a direct copy (SlaveBase::copy) can be done, in which case the mtime of the source is applied to the destination (if the protocol supports the concept).
void FileCopyJob::setSourceSize | ( | off_t | size | ) |
void FileCopyJob::setSourceSize64 | ( | KIO::filesize_t | size | ) |
void FileCopyJob::slotCanResume | ( | KIO::Job * | job, | |
KIO::filesize_t | offset | |||
) | [protected, slot] |
void FileCopyJob::slotData | ( | KIO::Job * | , | |
const QByteArray & | data | |||
) | [slot] |
void FileCopyJob::slotDataReq | ( | KIO::Job * | , | |
QByteArray & | data | |||
) | [slot] |
void FileCopyJob::slotPercent | ( | KIO::Job * | job, | |
unsigned long | pct | |||
) | [protected, slot] |
void FileCopyJob::slotProcessedSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [protected, slot] |
void FileCopyJob::slotResult | ( | KIO::Job * | job | ) | [protected, virtual, slot] |
void FileCopyJob::slotTotalSize | ( | KIO::Job * | job, | |
KIO::filesize_t | size | |||
) | [protected, slot] |
KURL KIO::FileCopyJob::srcURL | ( | ) | const [inline] |
void FileCopyJob::startCopyJob | ( | const KURL & | slave_url | ) | [protected] |
void FileCopyJob::startRenameJob | ( | const KURL & | slave_url | ) | [protected] |
void FileCopyJob::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
QByteArray KIO::FileCopyJob::m_buffer [protected] |
Definition at line 1372 of file jobclasses.h.
bool KIO::FileCopyJob::m_canResume [protected] |
Definition at line 1370 of file jobclasses.h.
SimpleJob* KIO::FileCopyJob::m_copyJob [protected] |
Definition at line 1374 of file jobclasses.h.
KURL KIO::FileCopyJob::m_dest [protected] |
Definition at line 1365 of file jobclasses.h.
TransferJob* KIO::FileCopyJob::m_getJob [protected] |
Definition at line 1375 of file jobclasses.h.
bool KIO::FileCopyJob::m_move [protected] |
Definition at line 1367 of file jobclasses.h.
SimpleJob* KIO::FileCopyJob::m_moveJob [protected] |
Definition at line 1373 of file jobclasses.h.
bool KIO::FileCopyJob::m_overwrite [protected] |
Definition at line 1368 of file jobclasses.h.
int KIO::FileCopyJob::m_permissions [protected] |
Definition at line 1366 of file jobclasses.h.
TransferJob* KIO::FileCopyJob::m_putJob [protected] |
Definition at line 1376 of file jobclasses.h.
bool KIO::FileCopyJob::m_resume [protected] |
Definition at line 1369 of file jobclasses.h.
bool KIO::FileCopyJob::m_resumeAnswerSent [protected] |
Definition at line 1371 of file jobclasses.h.
KURL KIO::FileCopyJob::m_src [protected] |
Definition at line 1364 of file jobclasses.h.
KIO::filesize_t KIO::FileCopyJob::m_totalSize [protected] |
Definition at line 1377 of file jobclasses.h.
The documentation for this class was generated from the following files: