kio
KIO::TransferJob Class Reference
The transfer job pumps data into and/or out of a Slave. More...
#include <jobclasses.h>
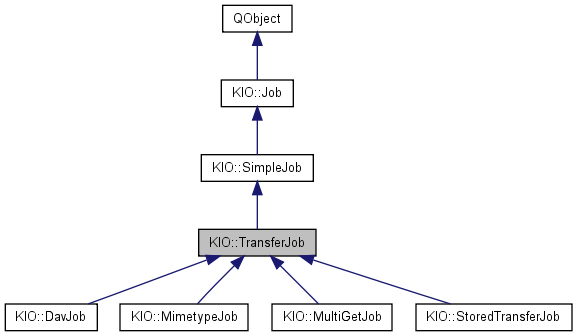
Signals | |
void | canResume (KIO::Job *job, KIO::filesize_t offset) |
void | data (KIO::Job *job, const QByteArray &data) |
void | dataReq (KIO::Job *job, QByteArray &data) |
void | mimetype (KIO::Job *job, const QString &type) |
void | permanentRedirection (KIO::Job *job, const KURL &fromUrl, const KURL &toUrl) |
void | redirection (KIO::Job *job, const KURL &url) |
Public Member Functions | |
bool | isErrorPage () const |
bool | isSuspended () const |
bool | reportDataSent () |
void | resume () |
void | sendAsyncData (const QByteArray &data) |
void | setAsyncDataEnabled (bool enabled) |
void | setReportDataSent (bool enabled) |
virtual void | slotResult (KIO::Job *job) |
virtual void | start (Slave *slave) |
void | suspend () |
TransferJob (const KURL &url, int command, const QByteArray &packedArgs, const QByteArray &_staticData, bool showProgressInfo) | |
Protected Slots | |
void | slotCanResume (KIO::filesize_t offset) |
virtual void | slotData (const QByteArray &data) |
virtual void | slotDataReq () |
void | slotErrorPage () |
virtual void | slotFinished () |
virtual void | slotMetaData (const KIO::MetaData &_metaData) |
virtual void | slotMimetype (const QString &mimetype) |
virtual void | slotNeedSubURLData () |
void | slotPostRedirection () |
virtual void | slotRedirection (const KURL &url) |
virtual void | slotSubURLData (KIO::Job *, const QByteArray &) |
Protected Member Functions | |
virtual void | virtual_hook (int id, void *data) |
Protected Attributes | |
bool | m_errorPage |
QString | m_mimetype |
KURL::List | m_redirectionList |
KURL | m_redirectionURL |
TransferJob * | m_subJob |
bool | m_suspended |
QByteArray | staticData |
Detailed Description
The transfer job pumps data into and/or out of a Slave.Data is sent to the slave on request of the slave ( dataReq). If data coming from the slave can not be handled, the reading of data from the slave should be suspended.
Definition at line 875 of file jobclasses.h.
Constructor & Destructor Documentation
TransferJob::TransferJob | ( | const KURL & | url, | |
int | command, | |||
const QByteArray & | packedArgs, | |||
const QByteArray & | _staticData, | |||
bool | showProgressInfo | |||
) |
Do not create a TransferJob.
Use KIO::get() or KIO::put() instead.
- Parameters:
-
url the url to get or put command the command to issue packedArgs the arguments _staticData additional data to transmit (e.g. in a HTTP Post) showProgressInfo true to show progress information to the user
Member Function Documentation
void KIO::TransferJob::canResume | ( | KIO::Job * | job, | |
KIO::filesize_t | offset | |||
) | [signal] |
For internal use only.
Emitted if the "put" job found an existing partial file (in which case offset is the size of that file) and emitted by the "get" job if it supports resuming to the given offset - in this case offset
is unused)
void KIO::TransferJob::data | ( | KIO::Job * | job, | |
const QByteArray & | data | |||
) | [signal] |
Data from the slave has arrived.
- Parameters:
-
job the job that emitted this signal data data received from the slave.
void KIO::TransferJob::dataReq | ( | KIO::Job * | job, | |
QByteArray & | data | |||
) | [signal] |
Request for data.
Please note, that you shouldn't put too large chunks of data in it as this requires copies within the frame work, so you should rather split the data you want to pass here in reasonable chunks (about 1MB maximum)
- Parameters:
-
job the job that emitted this signal data buffer to fill with data to send to the slave. An empty buffer indicates end of data. (EOD)
bool KIO::TransferJob::isErrorPage | ( | ) | const [inline] |
Checks whether we got an error page.
This currently only happens with HTTP urls. Call this from your slot connected to result().
- Returns:
- true if we got an (HTML) error page from the server instead of what we asked for.
Definition at line 931 of file jobclasses.h.
bool KIO::TransferJob::isSuspended | ( | ) | const [inline] |
Mimetype determined.
- Parameters:
-
job the job that emitted this signal type the mime type
void KIO::TransferJob::permanentRedirection | ( | KIO::Job * | job, | |
const KURL & | fromUrl, | |||
const KURL & | toUrl | |||
) | [signal] |
Signals a permanent redirection.
The redirection itself is handled internally.
- Parameters:
-
job the job that emitted this signal fromUrl the original URL toUrl the new URL
- Since:
- 3.1
void KIO::TransferJob::redirection | ( | KIO::Job * | job, | |
const KURL & | url | |||
) | [signal] |
Signals a redirection.
Use to update the URL shown to the user. The redirection itself is handled internally.
- Parameters:
-
job the job that emitted this signal url the new URL
bool TransferJob::reportDataSent | ( | ) |
void TransferJob::resume | ( | ) |
void TransferJob::sendAsyncData | ( | const QByteArray & | data | ) |
void TransferJob::setAsyncDataEnabled | ( | bool | enabled | ) |
Enable the async data mode.
When async data is enabled, data should be provided to the job by calling sendAsyncData() instead of returning data in the dataReq() signal.
- Since:
- 3.2
void TransferJob::setReportDataSent | ( | bool | enabled | ) |
When enabled, the job reports the amount of data that has been sent, instead of the amount of data that that has been received.
- See also:
- slotProcessedSize
- Since:
- 3.2
void TransferJob::slotCanResume | ( | KIO::filesize_t | offset | ) | [protected, slot] |
void TransferJob::slotData | ( | const QByteArray & | data | ) | [protected, virtual, slot] |
void TransferJob::slotDataReq | ( | ) | [protected, virtual, slot] |
void TransferJob::slotFinished | ( | ) | [protected, virtual, slot] |
Called when the slave marks the job as finished.
Reimplemented from KIO::SimpleJob.
Reimplemented in KIO::DavJob, KIO::MultiGetJob, and KIO::MimetypeJob.
void TransferJob::slotMetaData | ( | const KIO::MetaData & | _metaData | ) | [protected, virtual, slot] |
MetaData from the slave is received.
- Parameters:
-
_metaData the meta data
- See also:
- metaData()
Reimplemented from KIO::SimpleJob.
void TransferJob::slotMimetype | ( | const QString & | mimetype | ) | [protected, virtual, slot] |
void TransferJob::slotNeedSubURLData | ( | ) | [protected, virtual, slot] |
void TransferJob::slotPostRedirection | ( | ) | [protected, slot] |
void TransferJob::slotRedirection | ( | const KURL & | url | ) | [protected, virtual, slot] |
void TransferJob::slotResult | ( | KIO::Job * | job | ) | [virtual] |
void TransferJob::slotSubURLData | ( | KIO::Job * | , | |
const QByteArray & | data | |||
) | [protected, virtual, slot] |
void TransferJob::start | ( | Slave * | slave | ) | [virtual] |
For internal use only.
Called by the scheduler when a slave
gets to work on this job.
- Parameters:
-
slave the slave that starts working on this job
Reimplemented from KIO::SimpleJob.
Reimplemented in KIO::MultiGetJob, and KIO::MimetypeJob.
void TransferJob::suspend | ( | ) |
void TransferJob::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Member Data Documentation
bool KIO::TransferJob::m_errorPage [protected] |
Definition at line 1043 of file jobclasses.h.
QString KIO::TransferJob::m_mimetype [protected] |
Definition at line 1047 of file jobclasses.h.
KURL::List KIO::TransferJob::m_redirectionList [protected] |
Definition at line 1046 of file jobclasses.h.
KURL KIO::TransferJob::m_redirectionURL [protected] |
Definition at line 1045 of file jobclasses.h.
TransferJob* KIO::TransferJob::m_subJob [protected] |
bool KIO::TransferJob::m_suspended [protected] |
QByteArray KIO::TransferJob::staticData [protected] |
Definition at line 1044 of file jobclasses.h.
The documentation for this class was generated from the following files: