kio
KIO::CopyJob Class Reference
CopyJob is used to move, copy or symlink files and directories. More...
#include <jobclasses.h>
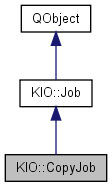
Public Types | |
enum | CopyMode { Copy, Move, Link } |
Signals | |
void | aboutToCreate (KIO::Job *job, const QValueList< KIO::CopyInfo > &files) |
void | copying (KIO::Job *job, const KURL &from, const KURL &to) |
void | copyingDone (KIO::Job *job, const KURL &from, const KURL &to, bool directory, bool renamed) |
void | copyingLinkDone (KIO::Job *job, const KURL &from, const QString &target, const KURL &to) |
void | creatingDir (KIO::Job *job, const KURL &dir) |
void | linking (KIO::Job *job, const QString &target, const KURL &to) |
void | moving (KIO::Job *job, const KURL &from, const KURL &to) |
void | processedDirs (KIO::Job *job, unsigned long dirs) |
void | processedFiles (KIO::Job *job, unsigned long files) |
void | renamed (KIO::Job *job, const KURL &from, const KURL &to) |
void | totalDirs (KIO::Job *job, unsigned long dirs) |
void | totalFiles (KIO::Job *job, unsigned long files) |
Public Member Functions | |
CopyJob (const KURL::List &src, const KURL &dest, CopyMode mode, bool asMethod, bool showProgressInfo) | |
KURL | destURL () const |
void | setDefaultPermissions (bool b) |
void | setInteractive (bool b) |
KURL::List | srcURLs () const |
virtual | ~CopyJob () |
Protected Slots | |
void | slotEntries (KIO::Job *, const KIO::UDSEntryList &list) |
void | slotProcessedSize (KIO::Job *, KIO::filesize_t data_size) |
void | slotReport () |
virtual void | slotResult (KIO::Job *job) |
void | slotStart () |
void | slotTotalSize (KIO::Job *, KIO::filesize_t size) |
Protected Member Functions | |
void | copyNextFile () |
void | createNextDir () |
void | deleteNextDir () |
void | setNextDirAttribute () |
void | skip (const KURL &sourceURL) |
void | slotResultConflictCopyingFiles (KIO::Job *job) |
void | slotResultConflictCreatingDirs (KIO::Job *job) |
void | slotResultCopyingFiles (KIO::Job *job) |
void | slotResultCreatingDirs (KIO::Job *job) |
void | slotResultDeletingDirs (KIO::Job *job) |
void | slotResultRenaming (KIO::Job *job) |
void | slotResultStating (KIO::Job *job) |
void | startListing (const KURL &src) |
void | statCurrentSrc () |
void | statNextSrc () |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
CopyJob is used to move, copy or symlink files and directories.Don't create the job directly, but use KIO::copy(), KIO::move(), KIO::link() and friends.
Definition at line 1508 of file jobclasses.h.
Member Enumeration Documentation
Constructor & Destructor Documentation
CopyJob::CopyJob | ( | const KURL::List & | src, | |
const KURL & | dest, | |||
CopyMode | mode, | |||
bool | asMethod, | |||
bool | showProgressInfo | |||
) |
Do not create a CopyJob directly.
Use KIO::copy(), KIO::move(), KIO::link() and friends instead.
- Parameters:
-
src the list of source URLs dest the destination URL mode specifies whether the job should copy, move or link asMethod if true, behaves like KIO::copyAs(), KIO::moveAs() or KIO::linkAs() showProgressInfo true to show progress information to the user
States: STATE_STATING for the dest STATE_STATING for each src url (statNextSrc) for each: if dir -> STATE_LISTING (filling 'dirs' and 'files') but if direct rename possible: STATE_RENAMING instead. STATE_CREATING_DIRS (createNextDir, iterating over 'dirs') if conflict: STATE_CONFLICT_CREATING_DIRS STATE_COPYING_FILES (copyNextFile, iterating over 'files') if conflict: STATE_CONFLICT_COPYING_FILES STATE_DELETING_DIRS (deleteNextDir) (if moving) STATE_SETTING_DIR_ATTRIBUTES (setNextDirAttribute, iterating over d->m_directoriesCopied) done.
Member Function Documentation
void KIO::CopyJob::aboutToCreate | ( | KIO::Job * | job, | |
const QValueList< KIO::CopyInfo > & | files | |||
) | [signal] |
Emitted when it is known which files / directories are going to be created.
Note that this may still change e.g. when existing files with the same name are discovered.
- Parameters:
-
job the job that emitted this signal files a list of items that are about to be created.
void KIO::CopyJob::copying | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to | |||
) | [signal] |
The job is copying a file or directory.
- Parameters:
-
job the job that emitted this signal from the URl of the file or directory that is currently being copied to the destination of the current operation
void KIO::CopyJob::copyingDone | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to, | |||
bool | directory, | |||
bool | renamed | |||
) | [signal] |
The job emits this signal when copying or moving a file or directory successfully finished.
This signal is mainly for the Undo feature.
- Parameters:
-
job the job that emitted this signal from the source URL to the destination URL directory indicates whether a file or directory was successfully copied/moved. true for a directoy, false for file renamed indicates that the destination URL was created using a rename operation (i.e. fast directory moving). true if is has been renamed
void KIO::CopyJob::copyingLinkDone | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const QString & | target, | |||
const KURL & | to | |||
) | [signal] |
The job is copying or moving a symbolic link, that points to target.
The new link is created in to
. The existing one is/was in from
. This signal is mainly for the Undo feature.
- Parameters:
-
job the job that emitted this signal from the source URL target the target to the destination URL
void KIO::CopyJob::creatingDir | ( | KIO::Job * | job, | |
const KURL & | dir | |||
) | [signal] |
The job is creating the directory dir
.
- Parameters:
-
job the job that emitted this signal dir the directory that is currently being created
KURL KIO::CopyJob::destURL | ( | ) | const [inline] |
Returns the destination URL.
- Returns:
- the destination URL
Definition at line 1548 of file jobclasses.h.
The job is creating a symbolic link.
- Parameters:
-
job the job that emitted this signal target the URl of the file or directory that is currently being linked to the destination of the current operation
void KIO::CopyJob::moving | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to | |||
) | [signal] |
The job is moving a file or directory.
- Parameters:
-
job the job that emitted this signal from the URl of the file or directory that is currently being moved to the destination of the current operation
void KIO::CopyJob::processedDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [signal] |
Sends the number of processed directories.
- Parameters:
-
job the job that emitted this signal dirs the number of processed dirs
void KIO::CopyJob::processedFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [signal] |
Sends the number of processed files.
- Parameters:
-
job the job that emitted this signal files the number of processed files
void KIO::CopyJob::renamed | ( | KIO::Job * | job, | |
const KURL & | from, | |||
const KURL & | to | |||
) | [signal] |
The user chose to rename from
to to
.
- Parameters:
-
job the job that emitted this signal from the original name to the new name
void KIO::CopyJob::setDefaultPermissions | ( | bool | b | ) |
By default the permissions of the copied files will be those of the source files.
But when copying "template" files to "new" files, people prefer the umask to apply, rather than the template's permissions. For that case, call setDefaultPermissions(true)
TODO KDE4: consider adding this as bool to copy/copyAs?
- Since:
- 3.2.3
void KIO::CopyJob::setInteractive | ( | bool | b | ) |
When an error happens while copying/moving a file, the user will be presented with a dialog for skipping the file that can't be copied/moved.
Or if the error is that the destination file already exists, the standard rename dialog is shown. If the program doesn't want CopyJob to show dialogs, but to simply fail on error, call setInteractive( false ).
KDE4: remove, already in Job
- Since:
- 3.4
Reimplemented from KIO::Job.
void CopyJob::slotEntries | ( | KIO::Job * | job, | |
const KIO::UDSEntryList & | list | |||
) | [protected, slot] |
void CopyJob::slotProcessedSize | ( | KIO::Job * | , | |
KIO::filesize_t | data_size | |||
) | [protected, slot] |
void CopyJob::slotResult | ( | KIO::Job * | job | ) | [protected, virtual, slot] |
void CopyJob::slotResultConflictCopyingFiles | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultConflictCreatingDirs | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultCopyingFiles | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultCreatingDirs | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultDeletingDirs | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultRenaming | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotResultStating | ( | KIO::Job * | job | ) | [protected] |
void CopyJob::slotStart | ( | ) | [protected, slot] |
void CopyJob::slotTotalSize | ( | KIO::Job * | , | |
KIO::filesize_t | size | |||
) | [protected, slot] |
KURL::List KIO::CopyJob::srcURLs | ( | ) | const [inline] |
Returns the list of source URLs.
- Returns:
- the list of source URLs.
Definition at line 1542 of file jobclasses.h.
void CopyJob::startListing | ( | const KURL & | src | ) | [protected] |
void KIO::CopyJob::totalDirs | ( | KIO::Job * | job, | |
unsigned long | dirs | |||
) | [signal] |
Emitted when the toal number of direcotries is known.
- Parameters:
-
job the job that emitted this signal dirs the total number of directories
void KIO::CopyJob::totalFiles | ( | KIO::Job * | job, | |
unsigned long | files | |||
) | [signal] |
Emitted when the total number of files is known.
- Parameters:
-
job the job that emitted this signal files the total number of files
void CopyJob::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
The documentation for this class was generated from the following files: