kio
KIO::Scheduler Class Reference
The KIO::Scheduler manages io-slaves for the application. More...
#include <scheduler.h>
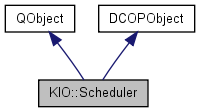
Public Types | |
typedef QPtrList< SimpleJob > | JobList |
Public Slots | |
void | slotSlaveDied (KIO::Slave *slave) |
void | slotSlaveStatus (pid_t pid, const QCString &protocol, const QString &host, bool connected) |
Signals | |
void | slaveConnected (KIO::Slave *slave) |
void | slaveError (KIO::Slave *slave, int error, const QString &errorMsg) |
Public Member Functions | |
bool | connect (const QObject *sender, const char *signal, const char *member) |
void | debug_info () |
virtual QCStringList | functions () |
virtual bool | process (const QCString &fun, const QByteArray &data, QCString &replyType, QByteArray &replyData) |
~Scheduler () | |
Static Public Member Functions | |
static bool | assignJobToSlave (KIO::Slave *slave, KIO::SimpleJob *job) |
static void | cancelJob (SimpleJob *job) |
static void | checkSlaveOnHold (bool b) |
static bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *member) |
static bool | connect (const char *signal, const QObject *receiver, const char *member) |
static bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *member) |
static bool | disconnectSlave (KIO::Slave *slave) |
static void | doJob (SimpleJob *job) |
static KIO::Slave * | getConnectedSlave (const KURL &url, const KIO::MetaData &config=MetaData()) |
static void | jobFinished (KIO::SimpleJob *job, KIO::Slave *slave) |
static void | publishSlaveOnHold () |
static void | putSlaveOnHold (KIO::SimpleJob *job, const KURL &url) |
static void | registerWindow (QWidget *wid) |
static void | removeSlaveOnHold () |
static void | scheduleJob (SimpleJob *job) |
static void | unregisterWindow (QObject *wid) |
Protected Slots | |
void | slotCleanIdleSlaves () |
void | slotScheduleCoSlave () |
void | slotSlaveConnected () |
void | slotSlaveError (int error, const QString &errorMsg) |
void | slotUnregisterWindow (QObject *) |
void | startStep () |
Protected Member Functions | |
Scheduler () | |
void | setupSlave (KIO::Slave *slave, const KURL &url, const QString &protocol, const QString &proxy, bool newSlave, const KIO::MetaData *config=0) |
bool | startJobDirect () |
bool | startJobScheduled (ProtocolInfo *protInfo) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
The KIO::Scheduler manages io-slaves for the application.It also queues jobs and assigns the job to a slave when one becomes available.
There are 3 possible ways for a job to get a slave:
1. Direct
This is the default. When you create a job the KIO::Scheduler will be notified and will find either an existing slave that is idle or it will create a new slave for the job.
Example:
TransferJob *job = KIO::get(KURL("http://www.kde.org"));
2. Scheduled
If you create a lot of jobs, you might want not want to have a slave for each job. If you schedule a job, a maximum number of slaves will be created. When more jobs arrive, they will be queued. When a slave is finished with a job, it will be assigned a job from the queue.
Example:
TransferJob *job = KIO::get(KURL("http://www.kde.org")); KIO::Scheduler::scheduleJob(job);
3. Connection Oriented
For some operations it is important that multiple jobs use the same connection. This can only be ensured if all these jobs use the same slave.
You can ask the scheduler to open a slave for connection oriented operations. You can then use the scheduler to assign jobs to this slave. The jobs will be queued and the slave will handle these jobs one after the other.
Example:
Slave *slave = KIO::Scheduler::getConnectedSlave( KURL("pop3://bastian:password@mail.kde.org")); TransferJob *job1 = KIO::get( KURL("pop3://bastian:password@mail.kde.org/msg1")); KIO::Scheduler::assignJobToSlave(slave, job1); TransferJob *job2 = KIO::get( KURL("pop3://bastian:password@mail.kde.org/msg2")); KIO::Scheduler::assignJobToSlave(slave, job2); TransferJob *job3 = KIO::get( KURL("pop3://bastian:password@mail.kde.org/msg3")); KIO::Scheduler::assignJobToSlave(slave, job3); // ... Wait for jobs to finish... KIO::Scheduler::disconnectSlave(slave);
Note that you need to explicitly disconnect the slave when the connection goes down, so your error handler should contain:
if (error == KIO::ERR_CONNECTION_BROKEN) KIO::Scheduler::disconnectSlave(slave);
- See also:
- KIO::Slave
Definition at line 111 of file scheduler.h.
Member Typedef Documentation
typedef QPtrList<SimpleJob> KIO::Scheduler::JobList |
Definition at line 115 of file scheduler.h.
Constructor & Destructor Documentation
Scheduler::~Scheduler | ( | ) |
Definition at line 148 of file scheduler.cpp.
Scheduler::Scheduler | ( | ) | [protected] |
Definition at line 126 of file scheduler.cpp.
Member Function Documentation
static bool KIO::Scheduler::assignJobToSlave | ( | KIO::Slave * | slave, | |
KIO::SimpleJob * | job | |||
) | [inline, static] |
Definition at line 215 of file scheduler.h.
static void KIO::Scheduler::cancelJob | ( | SimpleJob * | job | ) | [inline, static] |
Stop the execution of a job.
- Parameters:
-
job the job to cancel
Definition at line 145 of file scheduler.h.
static void KIO::Scheduler::checkSlaveOnHold | ( | bool | b | ) | [inline, static] |
When true, the next job will check whether KLauncher has a slave on hold that is suitable for the job.
- Parameters:
-
b true when KLauncher has a job on hold
Definition at line 280 of file scheduler.h.
bool KIO::Scheduler::connect | ( | const QObject * | sender, | |
const char * | signal, | |||
const char * | member | |||
) | [inline] |
Definition at line 271 of file scheduler.h.
static bool KIO::Scheduler::connect | ( | const char * | signal, | |
const QObject * | receiver, | |||
const char * | member | |||
) | [inline, static] |
Function to connect signals emitted by the scheduler.
- See also:
- slaveConnected()
Definition at line 259 of file scheduler.h.
void Scheduler::debug_info | ( | ) |
Definition at line 162 of file scheduler.cpp.
static bool KIO::Scheduler::disconnectSlave | ( | KIO::Slave * | slave | ) | [inline, static] |
Definition at line 230 of file scheduler.h.
static void KIO::Scheduler::doJob | ( | SimpleJob * | job | ) | [inline, static] |
Register job
with the scheduler.
The default is to create a new slave for the job if no slave is available. This can be changed by calling scheduleJob.
- Parameters:
-
job the job to register
Definition at line 129 of file scheduler.h.
QCStringList Scheduler::functions | ( | ) | [virtual] |
Definition at line 193 of file scheduler.cpp.
static KIO::Slave* KIO::Scheduler::getConnectedSlave | ( | const KURL & | url, | |
const KIO::MetaData & | config = MetaData() | |||
) | [inline, static] |
Requests a slave for use in connection-oriented mode.
- Parameters:
-
url This defines the username,password,host & port to connect with. config Configuration data for the slave.
- Returns:
- A pointer to a connected slave or 0 if an error occurred.
- See also:
- assignJobToSlave()
Definition at line 196 of file scheduler.h.
static void KIO::Scheduler::jobFinished | ( | KIO::SimpleJob * | job, | |
KIO::Slave * | slave | |||
) | [inline, static] |
Called when a job is done.
- Parameters:
-
job the finished job slave the slave that executed the job
Definition at line 153 of file scheduler.h.
bool Scheduler::process | ( | const QCString & | fun, | |
const QByteArray & | data, | |||
QCString & | replyType, | |||
QByteArray & | replyData | |||
) | [virtual] |
Definition at line 166 of file scheduler.cpp.
static void KIO::Scheduler::publishSlaveOnHold | ( | ) | [inline, static] |
Send the slave that was put on hold back to KLauncher.
This allows another process to take over the slave and resume the job that was started.
Definition at line 182 of file scheduler.h.
static void KIO::Scheduler::putSlaveOnHold | ( | KIO::SimpleJob * | job, | |
const KURL & | url | |||
) | [inline, static] |
Puts a slave on notice.
A next job may reuse this slave if it requests the same URL.
A job can be put on hold after it has emit'ed its mimetype. Based on the mimetype, the program can give control to another component in the same process which can then resume the job by simply asking for the same URL again.
- Parameters:
-
job the job that should be stopped url the URL that is handled by the url
Definition at line 167 of file scheduler.h.
static void KIO::Scheduler::registerWindow | ( | QWidget * | wid | ) | [inline, static] |
Send the slave that was put on hold back to KLauncher.
This allows another process to take over the slave and resume the job the that was started. Register the mainwindow wid
with the KIO subsystem Do not call this, it is called automatically from void KIO::Job::setWindow(QWidget*).
- Parameters:
-
wid the window to register
- Since:
- 3.1
Definition at line 243 of file scheduler.h.
static void KIO::Scheduler::removeSlaveOnHold | ( | ) | [inline, static] |
Removes any slave that might have been put on hold.
If a slave was put on hold it will be killed.
Definition at line 174 of file scheduler.h.
static void KIO::Scheduler::scheduleJob | ( | SimpleJob * | job | ) | [inline, static] |
Calling ths function makes that job
gets scheduled for later execution, if multiple jobs are registered it might wait for other jobs to finish.
- Parameters:
-
job the job to schedule
Definition at line 138 of file scheduler.h.
void Scheduler::setupSlave | ( | KIO::Slave * | slave, | |
const KURL & | url, | |||
const QString & | protocol, | |||
const QString & | proxy, | |||
bool | newSlave, | |||
const KIO::MetaData * | config = 0 | |||
) | [protected] |
Definition at line 283 of file scheduler.cpp.
void KIO::Scheduler::slaveConnected | ( | KIO::Slave * | slave | ) | [signal] |
void KIO::Scheduler::slaveError | ( | KIO::Slave * | slave, | |
int | error, | |||
const QString & | errorMsg | |||
) | [signal] |
void Scheduler::slotCleanIdleSlaves | ( | ) | [protected, slot] |
Definition at line 634 of file scheduler.cpp.
void Scheduler::slotScheduleCoSlave | ( | ) | [protected, slot] |
Definition at line 727 of file scheduler.cpp.
void Scheduler::slotSlaveConnected | ( | ) | [protected, slot] |
Definition at line 770 of file scheduler.cpp.
void Scheduler::slotSlaveDied | ( | KIO::Slave * | slave | ) | [slot] |
Definition at line 610 of file scheduler.cpp.
void Scheduler::slotSlaveError | ( | int | error, | |
const QString & | errorMsg | |||
) | [protected, slot] |
Definition at line 784 of file scheduler.cpp.
void Scheduler::slotSlaveStatus | ( | pid_t | pid, | |
const QCString & | protocol, | |||
const QString & | host, | |||
bool | connected | |||
) | [slot] |
Definition at line 567 of file scheduler.cpp.
void Scheduler::slotUnregisterWindow | ( | QObject * | obj | ) | [protected, slot] |
bool Scheduler::startJobDirect | ( | ) | [protected] |
Definition at line 413 of file scheduler.cpp.
bool Scheduler::startJobScheduled | ( | ProtocolInfo * | protInfo | ) | [protected] |
Definition at line 331 of file scheduler.cpp.
void Scheduler::startStep | ( | ) | [protected, slot] |
Definition at line 269 of file scheduler.cpp.
static void KIO::Scheduler::unregisterWindow | ( | QObject * | wid | ) | [inline, static] |
For internal use only.
Unregisters the window registered by registerWindow().
Definition at line 250 of file scheduler.h.
void Scheduler::virtual_hook | ( | int | id, | |
void * | data | |||
) | [protected, virtual] |
Definition at line 917 of file scheduler.cpp.
The documentation for this class was generated from the following files: