KUtils
KFindDialog Class Reference
[Main classes, Find and Replace classes]
A generic "find" dialog.
More...
#include <kfinddialog.h>
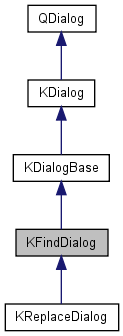
Public Types | |
enum | Options { WholeWordsOnly = 1, FromCursor = 2, SelectedText = 4, CaseSensitive = 8, FindBackwards = 16, RegularExpression = 32, FindIncremental = 64, MinimumUserOption = 65536 } |
Public Member Functions | |
QWidget * | findExtension () |
QStringList | findHistory () const |
KFindDialog (bool modal, QWidget *parent=0, const char *name=0, long options=0, const QStringList &findStrings=QStringList(), bool hasSelection=false) | |
KFindDialog (QWidget *parent=0, const char *name=0, long options=0, const QStringList &findStrings=QStringList(), bool hasSelection=false) | |
long | options () const |
QString | pattern () const |
void | setFindHistory (const QStringList &history) |
void | setHasCursor (bool hasCursor) |
void | setHasSelection (bool hasSelection) |
void | setOptions (long options) |
void | setPattern (const QString &pattern) |
void | setSupportsBackwardsFind (bool supports) |
void | setSupportsCaseSensitiveFind (bool supports) |
void | setSupportsRegularExpressionFind (bool supports) |
void | setSupportsWholeWordsFind (bool supports) |
virtual | ~KFindDialog () |
Protected Slots | |
void | showPatterns () |
void | showPlaceholders () |
void | slotOk () |
void | slotSelectedTextToggled (bool) |
void | textSearchChanged (const QString &) |
Protected Member Functions | |
virtual void | showEvent (QShowEvent *) |
Detailed Description
A generic "find" dialog.Detail:
This widget inherits from KDialogBase and implements the following additional functionalities: a find string object and an area for a user-defined widget to extend the dialog.
Example:
To use the basic modal find dialog, and then run the search:
KFindDialog dlg(....) if ( dlg.exec() != QDialog::Accepted ) return; // proceed with KFind from here
To create a non-modal find dialog:
if ( m_findDia ) KWin::setActiveWindow( m_findDia->winId() ); else { m_findDia = new KFindDialog(false,...); connect( m_findDia, SIGNAL(okClicked()), this, SLOT(findTextNext()) ); }
To use your own extensions: see findExtension().
Definition at line 74 of file kfinddialog.h.
Member Enumeration Documentation
Options for the search.
- Enumerator:
Reimplemented in KReplaceDialog.
Definition at line 87 of file kfinddialog.h.
Constructor & Destructor Documentation
KFindDialog::KFindDialog | ( | QWidget * | parent = 0 , |
|
const char * | name = 0 , |
|||
long | options = 0 , |
|||
const QStringList & | findStrings = QStringList() , |
|||
bool | hasSelection = false | |||
) |
Construct a modal find dialog.
- Parameters:
-
parent The parent object of this widget. name The name of this widget. options A bitfield of the Options to be checked. findStrings The find history, see findHistory() hasSelection Whether a selection exists
Definition at line 54 of file kfinddialog.cpp.
KFindDialog::KFindDialog | ( | bool | modal, | |
QWidget * | parent = 0 , |
|||
const char * | name = 0 , |
|||
long | options = 0 , |
|||
const QStringList & | findStrings = QStringList() , |
|||
bool | hasSelection = false | |||
) |
Construct a non-modal find dialog.
- Parameters:
-
modal set to false
to get a non-modal dialogparent The parent object of this widget. name The name of this widget. options A bitfield of the Options to be checked. findStrings The find history, see findHistory() hasSelection Whether a selection exists
Definition at line 65 of file kfinddialog.cpp.
KFindDialog::~KFindDialog | ( | ) | [virtual] |
Member Function Documentation
QWidget * KFindDialog::findExtension | ( | ) |
Returns an empty widget which the user may fill with additional UI elements as required.
The widget occupies the width of the dialog, and is positioned immediately below the regular expression support widgets for the pattern string.
- Returns:
- An extensible QWidget.
Definition at line 90 of file kfinddialog.cpp.
QStringList KFindDialog::findHistory | ( | ) | const |
Returns the list of history items.
- Returns:
- The find history.
- See also:
- setFindHistory
Definition at line 101 of file kfinddialog.cpp.
long KFindDialog::options | ( | ) | const |
Returns the state of the options.
Disabled options may be returned in an indeterminate state.
- Returns:
- The options.
- See also:
- Options, setOptions
Reimplemented in KReplaceDialog.
Definition at line 302 of file kfinddialog.cpp.
QString KFindDialog::pattern | ( | ) | const |
Returns the pattern to find.
- Returns:
- The search text.
Definition at line 321 of file kfinddialog.cpp.
void KFindDialog::setFindHistory | ( | const QStringList & | history | ) |
Provide the list of strings
to be displayed as the history of find strings.
strings
might get truncated if it is too long.
- Parameters:
-
history The find history.
- See also:
- findHistory
Definition at line 334 of file kfinddialog.cpp.
void KFindDialog::setHasCursor | ( | bool | hasCursor | ) |
Hide/show the 'from cursor' option, depending on whether the application implements a cursor.
- Parameters:
-
hasCursor true
if the application features a cursor This is assumed to be the case by default.
Definition at line 366 of file kfinddialog.cpp.
void KFindDialog::setHasSelection | ( | bool | hasSelection | ) |
Enable/disable the 'search in selection' option, depending on whether there actually is a selection.
- Parameters:
-
hasSelection true
if a selection exists
Definition at line 346 of file kfinddialog.cpp.
void KFindDialog::setOptions | ( | long | options | ) |
Set the options which are checked.
- Parameters:
-
options The setting of the Options.
- See also:
- Options
Reimplemented in KReplaceDialog.
Definition at line 410 of file kfinddialog.cpp.
void KFindDialog::setPattern | ( | const QString & | pattern | ) |
Sets the pattern to find.
- Parameters:
-
pattern The new search pattern.
Definition at line 326 of file kfinddialog.cpp.
void KFindDialog::setSupportsBackwardsFind | ( | bool | supports | ) |
Enable/disable the 'Find backwards' option, depending on whether the application supports it.
- Parameters:
-
supports true
if the application supports backwards find This is assumed to be the case by default.
- Since:
- 3.4
Definition at line 374 of file kfinddialog.cpp.
void KFindDialog::setSupportsCaseSensitiveFind | ( | bool | supports | ) |
Enable/disable the 'Case sensitive' option, depending on whether the application supports it.
- Parameters:
-
supports true
if the application supports case sensitive find This is assumed to be the case by default.
- Since:
- 3.4
Definition at line 383 of file kfinddialog.cpp.
void KFindDialog::setSupportsRegularExpressionFind | ( | bool | supports | ) |
Enable/disable the 'Regular expression' option, depending on whether the application supports it.
- Parameters:
-
supports true
if the application supports regular expression find This is assumed to be the case by default.
- Since:
- 3.4
Definition at line 401 of file kfinddialog.cpp.
void KFindDialog::setSupportsWholeWordsFind | ( | bool | supports | ) |
Enable/disable the 'Whole words only' option, depending on whether the application supports it.
- Parameters:
-
supports true
if the application supports whole words only find This is assumed to be the case by default.
- Since:
- 3.4
Definition at line 392 of file kfinddialog.cpp.
void KFindDialog::showEvent | ( | QShowEvent * | e | ) | [protected, virtual] |
void KFindDialog::showPatterns | ( | ) | [protected, slot] |
Definition at line 422 of file kfinddialog.cpp.
void KFindDialog::showPlaceholders | ( | ) | [protected, slot] |
Definition at line 489 of file kfinddialog.cpp.
void KFindDialog::slotOk | ( | void | ) | [protected, virtual, slot] |
Reimplemented from KDialogBase.
Reimplemented in KReplaceDialog.
Definition at line 518 of file kfinddialog.cpp.
void KFindDialog::slotSelectedTextToggled | ( | bool | selec | ) | [protected, slot] |
Definition at line 358 of file kfinddialog.cpp.
void KFindDialog::textSearchChanged | ( | const QString & | text | ) | [protected, slot] |
Definition at line 279 of file kfinddialog.cpp.
The documentation for this class was generated from the following files: