kpilot
PilotDaemon Class Reference
#include <pilotDaemon.h>
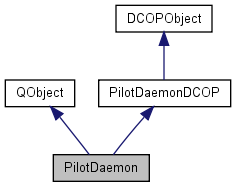
Public Types | |
enum | DaemonStatus { HOTSYNC_START, HOTSYNC_END, FILE_INSTALL_REQ, ERROR, READY, INIT, NOT_LISTENING } |
Public Slots | |
virtual ASYNC | requestSync (int) |
Public Member Functions | |
virtual void | addInstallFiles (const QStringList &) |
virtual QStringList | configuredConduitList () |
virtual bool | isListening () |
virtual bool | killDaemonOnExit () |
virtual QDateTime | lastSyncDate () |
virtual QString | logFileName () |
virtual int | nextSyncType () const |
PilotDaemon () | |
virtual QString | pilotDevice () |
virtual ASYNC | quitNow () |
virtual ASYNC | reloadSettings () |
virtual ASYNC | requestRegularSyncNext () |
virtual ASYNC | requestSyncOptions (bool, bool) |
virtual ASYNC | requestSyncType (QString) |
virtual ASYNC | setTempDevice (QString d) |
virtual QString | shortStatusString () |
void | showTray () |
virtual void | startListening () |
DaemonStatus | status () const |
virtual QString | statusString () |
virtual void | stopListening () |
virtual QString | userName () |
~PilotDaemon () | |
Protected Types | |
enum | postSyncActions { None = 0, ReloadSettings = 1, Quit = 2 } |
Protected Slots | |
void | endHotSync () |
void | logError (const QString &) |
void | logMessage (const QString &) |
void | logProgress (const QString &, int) |
void | slotFilesChanged () |
void | slotRunConfig () |
void | slotRunKPilot () |
void | startHotSync (KPilotLink *lnk) |
Protected Member Functions | |
LoggerDCOP_stub & | getFileLogger () |
KPilotDCOP_stub & | getKPilot () |
LoggerDCOP_stub & | getLogger () |
Protected Attributes | |
DaemonStatus | fDaemonStatus |
bool | fIsListening |
LogFile * | fLogFile |
int | fPostSyncAction |
Detailed Description
Definition at line 127 of file pilotDaemon.h.
Member Enumeration Documentation
Definition at line 139 of file pilotDaemon.h.
enum PilotDaemon::postSyncActions [protected] |
Constructor & Destructor Documentation
PilotDaemon::PilotDaemon | ( | ) |
Definition at line 280 of file pilotDaemon.cc.
PilotDaemon::~PilotDaemon | ( | ) |
Definition at line 320 of file pilotDaemon.cc.
Member Function Documentation
void PilotDaemon::addInstallFiles | ( | const QStringList & | ) | [virtual] |
Some other useful functionality.
Implements PilotDaemonDCOP.
Definition at line 331 of file pilotDaemon.cc.
QStringList PilotDaemon::configuredConduitList | ( | ) | [virtual] |
void PilotDaemon::endHotSync | ( | ) | [protected, slot] |
Definition at line 1153 of file pilotDaemon.cc.
LoggerDCOP_stub& PilotDaemon::getFileLogger | ( | ) | [inline, protected] |
Definition at line 265 of file pilotDaemon.h.
KPilotDCOP_stub& PilotDaemon::getKPilot | ( | ) | [inline, protected] |
Definition at line 266 of file pilotDaemon.h.
LoggerDCOP_stub& PilotDaemon::getLogger | ( | ) | [inline, protected] |
Provide access to KPilot's DCOP interface through a stub.
Definition at line 264 of file pilotDaemon.h.
virtual bool PilotDaemon::isListening | ( | ) | [inline, virtual] |
bool PilotDaemon::killDaemonOnExit | ( | ) | [virtual] |
QDateTime PilotDaemon::lastSyncDate | ( | ) | [virtual] |
Functions reporting same status data, e.g.
DCOP Functions reporting some status data, e.g.
for the kontact plugin.
Implements PilotDaemonDCOP.
Definition at line 715 of file pilotDaemon.cc.
void PilotDaemon::logError | ( | const QString & | s | ) | [protected, slot] |
Definition at line 1135 of file pilotDaemon.cc.
QString PilotDaemon::logFileName | ( | ) | [virtual] |
void PilotDaemon::logMessage | ( | const QString & | s | ) | [protected, slot] |
Definition at line 1126 of file pilotDaemon.cc.
void PilotDaemon::logProgress | ( | const QString & | s, | |
int | i | |||
) | [protected, slot] |
Definition at line 1144 of file pilotDaemon.cc.
int PilotDaemon::nextSyncType | ( | ) | const [virtual] |
Query what type is set most recently.
Implements PilotDaemonDCOP.
Definition at line 707 of file pilotDaemon.cc.
QString PilotDaemon::pilotDevice | ( | ) | [virtual] |
void PilotDaemon::quitNow | ( | ) | [virtual] |
Functions for the KPilot UI, indicating what the daemon should do.
Implements PilotDaemonDCOP.
Definition at line 613 of file pilotDaemon.cc.
void PilotDaemon::reloadSettings | ( | ) | [virtual] |
void PilotDaemon::requestRegularSyncNext | ( | ) | [virtual] |
Shortcut for using requestSync(1).
Implements PilotDaemonDCOP.
Definition at line 637 of file pilotDaemon.cc.
void PilotDaemon::requestSync | ( | int | ) | [virtual, slot] |
Start a HotSync.
What kind of HotSync is determined by the int parameter (use the enum in kpilot.kcfg, or better yet, use requestSyncType and pass the name). Using a value of 0 (zero, which isn't a legal mode for sync actions) uses the configuration file default.
Implements PilotDaemonDCOP.
Definition at line 643 of file pilotDaemon.cc.
void PilotDaemon::requestSyncOptions | ( | bool | test, | |
bool | local | |||
) | [virtual] |
Set the mix-ins (see SyncAction::SyncMode for details).
Implements PilotDaemonDCOP.
Definition at line 694 of file pilotDaemon.cc.
void PilotDaemon::requestSyncType | ( | QString | ) | [virtual] |
Request a particular kind of sync next; pass in the name of a sync type instead.
Implements PilotDaemonDCOP.
Definition at line 674 of file pilotDaemon.cc.
void PilotDaemon::setTempDevice | ( | QString | d | ) | [virtual] |
QString PilotDaemon::shortStatusString | ( | ) | [virtual] |
void PilotDaemon::showTray | ( | ) |
Display the daemon's system tray icon (if there is one, depending on the DockDaemon setting in the config file).
Definition at line 384 of file pilotDaemon.cc.
void PilotDaemon::slotFilesChanged | ( | ) | [protected, slot] |
Called after a file has been installed to notify any observers, like KPilot, that files have been installed.
[Here that means: copied to the pending_install directory and thus *waiting* for installation on the Palm]
Definition at line 1198 of file pilotDaemon.cc.
void PilotDaemon::slotRunConfig | ( | ) | [protected, slot] |
void PilotDaemon::slotRunKPilot | ( | ) | [protected, slot] |
void PilotDaemon::startHotSync | ( | KPilotLink * | lnk | ) | [protected, slot] |
Definition at line 990 of file pilotDaemon.cc.
void PilotDaemon::startListening | ( | ) | [virtual] |
DaemonStatus PilotDaemon::status | ( | ) | const [inline] |
Definition at line 150 of file pilotDaemon.h.
QString PilotDaemon::statusString | ( | ) | [virtual] |
Functions requesting the status of the daemon.
Implements PilotDaemonDCOP.
Definition at line 531 of file pilotDaemon.cc.
void PilotDaemon::stopListening | ( | ) | [virtual] |
QString PilotDaemon::userName | ( | ) | [virtual] |
Member Data Documentation
DaemonStatus PilotDaemon::fDaemonStatus [protected] |
Definition at line 191 of file pilotDaemon.h.
bool PilotDaemon::fIsListening [protected] |
Definition at line 269 of file pilotDaemon.h.
LogFile* PilotDaemon::fLogFile [protected] |
Definition at line 266 of file pilotDaemon.h.
int PilotDaemon::fPostSyncAction [protected] |
Definition at line 198 of file pilotDaemon.h.
The documentation for this class was generated from the following files: