kpilot
KPilotLink Class Reference
KPilotLink handles some aspects of communication with a Handheld. More...
#include <kpilotlink.h>
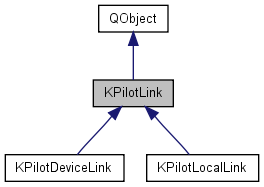
Public Types | |
enum | CustomEvents { EventTickleTimeout = 1066 } |
typedef QValueList< struct DBInfo > | DBInfoList |
enum | EndOfSyncFlags { NoUpdate, UpdateUserInfo } |
Public Slots | |
virtual void | close ()=0 |
virtual void | reset ()=0 |
virtual bool | tickle ()=0 |
Signals | |
void | deviceReady (KPilotLink *) |
void | logError (const QString &) |
void | logMessage (const QString &) |
void | logProgress (const QString &s, int p) |
void | timeout () |
Public Member Functions | |
void | addSyncLogEntry (const QString &entry, bool log=true) |
virtual PilotDatabase * | database (const DBInfo *info) |
virtual PilotDatabase * | database (const QString &name)=0 |
virtual void | endSync (EndOfSyncFlags f)=0 |
virtual bool | event (QEvent *e) |
virtual int | findDatabase (const char *name, struct DBInfo *info, int index=0, unsigned long type=0, unsigned long creator=0)=0 |
virtual const KPilotCard * | getCardInfo (int card=0)=0 |
virtual DBInfoList | getDBList (int cardno=0, int flags=dlpDBListRAM)=0 |
virtual int | getNextDatabase (int index, struct DBInfo *db)=0 |
KPilotUser & | getPilotUser () |
const KPilotSysInfo & | getSysInfo () |
unsigned int | installFiles (const QStringList &l, const bool deleteFiles) |
virtual bool | isConnected () const =0 |
KPilotLink (QObject *parent=0, const char *name=0) | |
QString | pilotPath () const |
virtual void | reset (const QString &pilotPath)=0 |
virtual bool | retrieveDatabase (const QString &path, struct DBInfo *db)=0 |
virtual QString | statusString () const =0 |
virtual | ~KPilotLink () |
Protected Member Functions | |
virtual void | addSyncLogEntryImpl (const QString &s)=0 |
virtual bool | installFile (const QString &f, const bool deleteFile)=0 |
virtual int | openConduit () |
virtual int | pilotSocket () const |
void | startTickle (unsigned int timeout=0) |
void | stopTickle () |
Protected Attributes | |
QString | fPilotPath |
KPilotSysInfo * | fPilotSysInfo |
KPilotUser * | fPilotUser |
Detailed Description
KPilotLink handles some aspects of communication with a Handheld.A KPilotLink object represents a connection to a device (which may be active or inactive -- the latter in cases where the link is waiting for a device to show up). The object handles waiting, protocol initialization and some general tasks such as getting system information or user data.
The actual communication with the handheld should use the PilotDatabase methods or use pilot-link dlp_* functions directly on the file descriptor returned by handle().
Implementations of this abstract class are KPilotDeviceLink (for real physical devices) and KPilotLocalLink (for devices represented by an on-disk directory).
General
A KPilotLink object (or one of its subclasses) represents a single (potential) link to a handheld device. The handheld device may be a real physical one (subclass KPilotDeviceLink) or a virtual one (subclass KPilotLocalLink). Every KPilotLink is associated with exactly one identifier for what device it is attached to. Physical devices have physical locations as interpreted by libpisock -- /dev/ttyUSB0 for instance, or net:any -- while virtual devices are associated with a location in the filesystem.A particular KPilotLink object may be connected -- communicating with a device -- or not. For physical devices, that means that the device is attached to the system (for USB-connected devices, think of it as a metaphor in the case of net:any) and that the HotSync button has been pressed. Virtual devices are immediately connected on creation, since there is no sensible "not connected" state. A connected KPilotLink has access to the data on the handheld and can give that data to the rest of the application.
The data access API is divided into roughly three parts, with tickle handling being a special fourth part (see section below). These are:
- Message logging
- System information access
- Database access
Lifecycle
The life-cycle of a KPilotLink object is as follows:# Object is created (one of the concrete subclasses, anyway) # Object gets a location assigned through reset(const QString &) # Object is connected to the handheld device (somehow, depends on subclass) # Object emits signal deviceReady()
After this, the application is free to use the API to access the information from the handheld. When the device connection is no longer needed, call either endOfSync() or finishSync() to wrap up the communications. The object remains alive and may be re-used by calling reset() to use the same location or reset(const QString &) to give it a new location.
handling.
During a HotSync, the Pilot expects to be kept awake by (nearly) continuous communication with the PC. The Pilot doesn't like long periods of inactivity, since they drain the batteries while the communications hardware is kept powered up. If the period of inactivity is too long, the Pilot times out, shuts down the communication, and the HotSync is broken.Sometimes, however, periods of inactivity cannot be avoided -- for instance, if you _have_ to ask the user something during a sync, or if you are fetching a large amount of data from a slow source (libkabc can do that, if your addressbook is on an LDAP server). During these periods of inactivity (as far as the Pilot can tell), you can "tickle" the Pilot to keep it awake. This prevents the communications from being shut down. It's not a good idea to do this all the time -- battery life and possible corruption of the dlp_ communications streams. Hence, you should start and stop tickling the Pilot around any computation which:
- may take a long time
- does not in itself ever communicate directly with the Pilot
You can call slot tickle() whenever you like just to do a dlp_tickle() call on the Pilot. It will return true if the tickle was successful, false otherwise (this can be used to detect if the communication with the Pilot has shut down for some reason).
The protected methods startTickle() and stopTickle() are intended to be called only from SyncActions -- I can't think of any other legitimate use, since everything being done during a HotSync is done via subclasses of SyncActions anyway, and SyncAction provides access to these methods though its own start- and stopTickle().
Call startTickle with a timeout in seconds, or 0 for no timeout. This timeout is _unrelated_ to the timeout in the Pilot's communications. Instead, it indicates how long to continue tickling the Pilot before emitting the timeout() signal. This can be useful for placing an upper bound on the amount of time to wait for, say, user interaction -- you don't want an inattentive user to drain the batteries during a sync because he doesn't click on "Yes" for some question. If you pass a timeout of 0, the Pilot will continue to be tickled until you call stopTickle().
Call stopTickle() to stop tickling the Pilot and continue with normal operation. You must call stopTickle() before calling anything else that might communicate with the Pilot, to avoid corrupting the dlp_ communications stream. (TODO: Mutex the heck out of this to avoid this problem). Note that stopTickle() may hang up the caller for a small amount of time (up to 200ms) before returning.
event() and TickleTimeoutEvent are part of the implementation of tickling, and are only accidentally visible.
Signal timeout() is emitted if startTickle() has been called with a non-zero timeout and that timeout has elapsed. The tickler is stopped before timeout is emitted.
Definition at line 171 of file kpilotlink.h.
Member Typedef Documentation
typedef QValueList<struct DBInfo> KPilotLink::DBInfoList |
Member Enumeration Documentation
When ending the sync, you can do so gracefully, updating the last-sync time to indicate a successful sync and setting the user name on the device, or you can skip that (for unsuccessful syncs, generally).
- Enumerator:
-
NoUpdate Do not update the user info. UpdateUserInfo Update user info and last successful sync date.
Definition at line 359 of file kpilotlink.h.
Constructor & Destructor Documentation
KPilotLink::KPilotLink | ( | QObject * | parent = 0 , |
|
const char * | name = 0 | |||
) |
Constructor.
Use reset() to start looking for a device.
Definition at line 140 of file kpilotlink.cc.
KPilotLink::~KPilotLink | ( | ) | [virtual] |
Destructor.
This rudely interrupts any communication in progress. It is best to call endOfSync() or finishSync() before destroying the device.
Definition at line 165 of file kpilotlink.cc.
Member Function Documentation
void KPilotLink::addSyncLogEntry | ( | const QString & | entry, | |
bool | log = true | |||
) |
Write a log entry to the handheld.
If log
is true, then the signal logMessage() is also emitted. This function is supposed to only write to the handheld's log (with a physical device, that is what appears on screen at the end of a sync).
Definition at line 244 of file kpilotlink.cc.
virtual void KPilotLink::addSyncLogEntryImpl | ( | const QString & | s | ) | [protected, pure virtual] |
Actually write an entry to the device link.
The message s
must be guaranteed non-empty.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual void KPilotLink::close | ( | ) | [pure virtual, slot] |
Release all resources, including the master pilot socket, timers, etc.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
PilotDatabase * KPilotLink::database | ( | const DBInfo * | info | ) | [virtual] |
Return a database object for manipulating the database with the name stored in the DBInfo structure info
.
The default version goes through method database( const QString & ), above.
- Returns:
- pointer to database object, or 0 on error.
- Note:
- ownership of the database object is given to the caller.
Reimplemented in KPilotDeviceLink.
Definition at line 267 of file kpilotlink.cc.
virtual PilotDatabase* KPilotLink::database | ( | const QString & | name | ) | [pure virtual] |
Return a database object for manipulating the database with name name
on the link.
This database may be local or remote, depending on the kind of link in use.
- Returns:
- pointer to database object, or 0 on error.
- Note:
- ownership of the database object is given to the caller, who must delete the object in time.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
void KPilotLink::deviceReady | ( | KPilotLink * | ) | [signal] |
Emitted once the user information has been read and the HotSync is really ready to go.
virtual void KPilotLink::endSync | ( | EndOfSyncFlags | f | ) | [pure virtual] |
End the sync in a gracuful manner.
If f
is UpdateUserInfo, the sync was successful and the user info and last successful sync timestamp are updated.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
bool KPilotLink::event | ( | QEvent * | e | ) | [virtual] |
Allows our class to receive custom events that our threads will be giving to us, including tickle timeouts and device communication events.
Reimplemented from QObject.
Reimplemented in KPilotDeviceLink.
Definition at line 172 of file kpilotlink.cc.
virtual int KPilotLink::findDatabase | ( | const char * | name, | |
struct DBInfo * | info, | |||
int | index = 0 , |
|||
unsigned long | type = 0 , |
|||
unsigned long | creator = 0 | |||
) | [pure virtual] |
Find a database with the given name
(and optionally, type type
and creator ID (from pi_mktag) creator
, on searching from index index
on the handheld.
Fills in the DBInfo structure info
if found.
- Returns:
- >=0 on success. See the documentation for each subclass for particular meanings.
< 0 on error.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual const KPilotCard* KPilotLink::getCardInfo | ( | int | card = 0 |
) | [pure virtual] |
Retrieve information about the data card card
; I don't think that any pilot supports card numbers other than 0.
Non-device links return something fake.
This function may return NULL (non-device links or on error).
- Note:
- Ownership of the KPilotCard object is given to the caller, who must delete it.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual DBInfoList KPilotLink::getDBList | ( | int | cardno = 0 , |
|
int | flags = dlpDBListRAM | |||
) | [pure virtual] |
Returns a list of DBInfo structures describing all the databases available on the link (ie.
device) with the given card number cardno
and flags flags
. No known handheld uses a cardno other than 0; use flags to indicate what kind of databases to fetch -- dlpDBListRAM
or dlpDBListROM
.
- Returns:
- list of DBInfo objects, one for each database
- Note:
- ownership of the DBInfo objects is passed to the caller, who must delete the objects.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual int KPilotLink::getNextDatabase | ( | int | index, | |
struct DBInfo * | db | |||
) | [pure virtual] |
Fill the DBInfo structure db
with information about the next database (in some ordering) counting from index
.
- Returns:
- < 0 on error
Implemented in KPilotDeviceLink, and KPilotLocalLink.
KPilotUser& KPilotLink::getPilotUser | ( | ) | [inline] |
Retrieve the user information from the device.
Ownership is kept by the link, and at the end of a sync the user information is synced back to the link -- so it may be modified, but don't make local copies of it.
- Note:
- Do not call this before the sync begins!
Definition at line 323 of file kpilotlink.h.
const KPilotSysInfo& KPilotLink::getSysInfo | ( | ) | [inline] |
System information about the handheld.
Ownership is kept by the link. For non-device links, something fake is returned.
- Note:
- Do not call this before the sync begins!
Definition at line 335 of file kpilotlink.h.
virtual bool KPilotLink::installFile | ( | const QString & | f, | |
const bool | deleteFile | |||
) | [protected, pure virtual] |
Install a single file onto the device link.
Full pathname f
is used; in addition, if deleteFile
is true remove the source file. Returns true
if the install succeeded.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
unsigned int KPilotLink::installFiles | ( | const QStringList & | l, | |
const bool | deleteFiles | |||
) |
Install the list of files (full paths!) named by l
onto the handheld (or whatever this link represents).
If deleteFiles
is true, the source files are removed.
- Returns:
- the number of files successfully installed.
Definition at line 221 of file kpilotlink.cc.
virtual bool KPilotLink::isConnected | ( | ) | const [pure virtual] |
True if HotSync has been started but not finished yet (ie.
the physical Pilot is waiting for sync commands)
Implemented in KPilotDeviceLink, and KPilotLocalLink.
void KPilotLink::logError | ( | const QString & | ) | [signal] |
Signal that an error has occurred, for logging.
void KPilotLink::logMessage | ( | const QString & | ) | [signal] |
Signal that a message has been written to the sync log.
void KPilotLink::logProgress | ( | const QString & | s, | |
int | p | |||
) | [signal] |
Signal that progress has been made, for logging purposes.
p
is the percentage completed (0 <= s <= 100). The string s
is logged as well, if non-Null.
int KPilotLink::openConduit | ( | ) | [protected, virtual] |
Notify the Pilot user that a conduit is running now.
On real devices, this prints out (on screen) which database is now opened; useful for progress reporting.
- Returns:
- -1 on error
- Note:
- the default implementation returns 0
Reimplemented in KPilotDeviceLink, and KPilotLocalLink.
Definition at line 257 of file kpilotlink.cc.
QString KPilotLink::pilotPath | ( | ) | const [inline] |
Information on what kind of device we're dealing with.
A link is associated with a path -- either the node in /dev that the physical device is attached to, or an IP address, or a filesystem path for local links. Whichever is being used, this function returns its name in a human-readable form.
Definition at line 207 of file kpilotlink.h.
int KPilotLink::pilotSocket | ( | ) | const [protected, virtual] |
Returns a file handle for raw operations.
Not recommended. On links with no physical device backing, returns -1.
- Note:
- the default implementation returns -1
Reimplemented in KPilotDeviceLink, and KPilotLocalLink.
Definition at line 262 of file kpilotlink.cc.
virtual void KPilotLink::reset | ( | ) | [pure virtual, slot] |
Assuming things have been set up at least once already by a call to reset() with parameters, use this slot to re-start with the same settings.
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual void KPilotLink::reset | ( | const QString & | pilotPath | ) | [pure virtual] |
Return the device link to the Init state and try connecting to the given device path (if it's non-empty).
What the path means depends on the kind of link we're instantiating.
- See also:
- reset()
Implemented in KPilotDeviceLink, and KPilotLocalLink.
virtual bool KPilotLink::retrieveDatabase | ( | const QString & | path, | |
struct DBInfo * | db | |||
) | [pure virtual] |
Retrieve the database indicated by DBInfo *db
into the local file path
.
This copies all the data, and you can create a PilotLocalDatabase from the resulting path
.
- Returns:
true
on success
Implemented in KPilotDeviceLink, and KPilotLocalLink.
void KPilotLink::startTickle | ( | unsigned int | timeout = 0 |
) | [protected] |
Start tickling the Handheld (every few seconds).
This lasts until timeout
seconds have passed (or forever if timeout
is zero).
- Note:
- Do not call startTickle() twice with no intervening stopTickle().
Definition at line 186 of file kpilotlink.cc.
virtual QString KPilotLink::statusString | ( | ) | const [pure virtual] |
void KPilotLink::stopTickle | ( | ) | [protected] |
Stop tickling the Handheld.
This may block for some time (less than a second) to allow the tickle thread to finish.
Definition at line 210 of file kpilotlink.cc.
virtual bool KPilotLink::tickle | ( | ) | [pure virtual, slot] |
void KPilotLink::timeout | ( | ) | [signal] |
A timeout associated with tickling has occurred.
Each time startTickle() is called, you can state how long tickling should last (at most) before timing out.
You can only get a timeout when the Qt event loop is running, which somewhat limits the usefulness of timeouts.
Member Data Documentation
QString KPilotLink::fPilotPath [protected] |
Path of the device special file that will be used.
Usually /dev/pilot, /dev/ttySx, or /dev/usb/x. May be a filesystem path for local links.
Definition at line 432 of file kpilotlink.h.
KPilotSysInfo* KPilotLink::fPilotSysInfo [protected] |
System information about the device.
Filled in when the sync starts. Non-device links need to fake something.
Definition at line 492 of file kpilotlink.h.
KPilotUser* KPilotLink::fPilotUser [protected] |
User information structure.
Should be filled in when a sync starts, so that conduits can use the information.
Definition at line 486 of file kpilotlink.h.
The documentation for this class was generated from the following files: