kpilot
KPilotDeviceLink Class Reference
Definition of the device link class for physical handheld devices, which communicate with the PC using DLP / SLP via the pilot-link library. More...
#include <kpilotdevicelink.h>
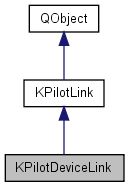
Public Member Functions | |
virtual void | close () |
virtual PilotDatabase * | database (const DBInfo *info) |
virtual PilotDatabase * | database (const QString &name) |
virtual void | endSync (EndOfSyncFlags f) |
virtual bool | event (QEvent *e) |
virtual int | findDatabase (const char *name, struct DBInfo *, int index=0, unsigned long type=0, unsigned long creator=0) |
virtual const KPilotCard * | getCardInfo (int card) |
virtual DBInfoList | getDBList (int cardno=0, int flags=dlpDBListRAM) |
virtual int | getNextDatabase (int index, struct DBInfo *) |
virtual bool | isConnected () const |
KPilotDeviceLink (QObject *parent=0, const char *name=0, const QString &tempDevice=QString::null) | |
virtual int | openConduit () |
virtual void | reset () |
virtual void | reset (const QString &) |
virtual bool | retrieveDatabase (const QString &path, struct DBInfo *db) |
void | setDevice (const QString &device) |
void | setTempDevice (const QString &device) |
void | setWorkarounds (bool usb) |
LinkStatus | status () const |
virtual QString | statusString () const |
virtual bool | tickle () |
virtual | ~KPilotDeviceLink () |
Static Public Member Functions | |
static QString | statusString (LinkStatus l) |
Protected Member Functions | |
virtual void | addSyncLogEntryImpl (const QString &s) |
void | checkDevice () |
virtual bool | installFile (const QString &, const bool deleteFile) |
virtual int | pilotSocket () const |
void | startCommThread () |
void | stopCommThread () |
Protected Attributes | |
int | fAcceptedCount |
DeviceCommThread * | fDeviceCommThread |
Messages * | fMessages |
int | fPilotSocket |
QString | fRealPilotPath |
QString | fTempDevice |
bool | fWorkaroundUSB |
Detailed Description
Definition of the device link class for physical handheld devices, which communicate with the PC using DLP / SLP via the pilot-link library.Definition at line 72 of file kpilotdevicelink.h.
Constructor & Destructor Documentation
KPilotDeviceLink::KPilotDeviceLink | ( | QObject * | parent = 0 , |
|
const char * | name = 0 , |
|||
const QString & | tempDevice = QString::null | |||
) |
Constructor.
Creates a link that can sync to a physical handheld. Call reset() on it to start looking for a device.
- Parameters:
-
parent Parent object. name Name of this object. tempDevice Path to device node to use as an alternative to the "normal" one set by KPilot.
Definition at line 530 of file kpilotdevicelink.cc.
KPilotDeviceLink::~KPilotDeviceLink | ( | ) | [virtual] |
Destructor.
This rudely ends the communication with the handheld. It is best to call endOfSync() or finishSync() before destroying the device.
Definition at line 542 of file kpilotdevicelink.cc.
Member Function Documentation
void KPilotDeviceLink::addSyncLogEntryImpl | ( | const QString & | s | ) | [protected, virtual] |
Actually write an entry to the device link.
The message s
must be guaranteed non-empty.
Implements KPilotLink.
Definition at line 741 of file kpilotdevicelink.cc.
void KPilotDeviceLink::checkDevice | ( | ) | [protected] |
Check for device permissions and existence, emitting warnings for weird situations.
This is primarily intended to inform the user.
Definition at line 697 of file kpilotdevicelink.cc.
void KPilotDeviceLink::close | ( | ) | [virtual] |
Release all resources, including the master pilot socket, timers, etc.
Implements KPilotLink.
Definition at line 630 of file kpilotdevicelink.cc.
PilotDatabase * KPilotDeviceLink::database | ( | const DBInfo * | info | ) | [virtual] |
Return a database object for manipulating the database with the name stored in the DBInfo structure info
.
The default version goes through method database( const QString & ), above.
- Returns:
- pointer to database object, or 0 on error.
- Note:
- ownership of the database object is given to the caller.
Reimplemented from KPilotLink.
Definition at line 962 of file kpilotdevicelink.cc.
PilotDatabase * KPilotDeviceLink::database | ( | const QString & | name | ) | [virtual] |
Return a database object for manipulating the database with name name
on the link.
This database may be local or remote, depending on the kind of link in use.
- Returns:
- pointer to database object, or 0 on error.
- Note:
- ownership of the database object is given to the caller, who must delete the object in time.
Implements KPilotLink.
Definition at line 957 of file kpilotdevicelink.cc.
void KPilotDeviceLink::endSync | ( | EndOfSyncFlags | f | ) | [virtual] |
End the sync in a gracuful manner.
If f
is UpdateUserInfo, the sync was successful and the user info and last successful sync timestamp are updated.
Implements KPilotLink.
Definition at line 834 of file kpilotdevicelink.cc.
bool KPilotDeviceLink::event | ( | QEvent * | e | ) | [virtual] |
Allows our class to receive custom events that our threads will be giving to us, including tickle timeouts and device communication events.
Reimplemented from KPilotLink.
Definition at line 556 of file kpilotdevicelink.cc.
int KPilotDeviceLink::findDatabase | ( | const char * | name, | |
struct DBInfo * | info, | |||
int | index = 0 , |
|||
unsigned long | type = 0 , |
|||
unsigned long | creator = 0 | |||
) | [virtual] |
Find a database with the given name
(and optionally, type type
and creator ID (from pi_mktag) creator
, on searching from index index
on the handheld.
Fills in the DBInfo structure info
if found.
- Returns:
- >=0 on success. See the documentation for each subclass for particular meanings.
< 0 on error.
Implements KPilotLink.
Definition at line 867 of file kpilotdevicelink.cc.
const KPilotCard * KPilotDeviceLink::getCardInfo | ( | int | card | ) | [virtual] |
Retrieve information about the data card card
; I don't think that any pilot supports card numbers other than 0.
Non-device links return something fake.
This function may return NULL (non-device links or on error).
- Note:
- Ownership of the KPilotCard object is given to the caller, who must delete it.
Implements KPilotLink.
Definition at line 944 of file kpilotdevicelink.cc.
KPilotLink::DBInfoList KPilotDeviceLink::getDBList | ( | int | cardno = 0 , |
|
int | flags = dlpDBListRAM | |||
) | [virtual] |
Returns a list of DBInfo structures describing all the databases available on the link (ie.
device) with the given card number cardno
and flags flags
. No known handheld uses a cardno other than 0; use flags to indicate what kind of databases to fetch -- dlpDBListRAM
or dlpDBListROM
.
- Returns:
- list of DBInfo objects, one for each database
- Note:
- ownership of the DBInfo objects is passed to the caller, who must delete the objects.
Implements KPilotLink.
Definition at line 910 of file kpilotdevicelink.cc.
int KPilotDeviceLink::getNextDatabase | ( | int | index, | |
struct DBInfo * | db | |||
) | [virtual] |
Fill the DBInfo structure db
with information about the next database (in some ordering) counting from index
.
- Returns:
- < 0 on error
Implements KPilotLink.
Definition at line 853 of file kpilotdevicelink.cc.
bool KPilotDeviceLink::installFile | ( | const QString & | f, | |
const bool | deleteFile | |||
) | [protected, virtual] |
Install a single file onto the device link.
Full pathname f
is used; in addition, if deleteFile
is true remove the source file. Returns true
if the install succeeded.
Implements KPilotLink.
Definition at line 747 of file kpilotdevicelink.cc.
bool KPilotDeviceLink::isConnected | ( | ) | const [virtual] |
True if HotSync has been started but not finished yet (ie.
the physical Pilot is waiting for sync commands)
Implements KPilotLink.
Definition at line 551 of file kpilotdevicelink.cc.
int KPilotDeviceLink::openConduit | ( | ) | [virtual] |
Notify the Pilot user that a conduit is running now.
On real devices, this prints out (on screen) which database is now opened; useful for progress reporting.
- Returns:
- -1 on error
- Note:
- the default implementation returns 0
Reimplemented from KPilotLink.
Definition at line 786 of file kpilotdevicelink.cc.
virtual int KPilotDeviceLink::pilotSocket | ( | ) | const [inline, protected, virtual] |
Returns a file handle for raw operations.
Not recommended. On links with no physical device backing, returns -1.
- Note:
- the default implementation returns -1
Reimplemented from KPilotLink.
Definition at line 136 of file kpilotdevicelink.h.
void KPilotDeviceLink::reset | ( | ) | [virtual] |
Assuming things have been set up at least once already by a call to reset() with parameters, use this slot to re-start with the same settings.
Implements KPilotLink.
Definition at line 683 of file kpilotdevicelink.cc.
void KPilotDeviceLink::reset | ( | const QString & | pilotPath | ) | [virtual] |
Return the device link to the Init state and try connecting to the given device path (if it's non-empty).
What the path means depends on the kind of link we're instantiating.
- See also:
- reset()
Implements KPilotLink.
Definition at line 639 of file kpilotdevicelink.cc.
bool KPilotDeviceLink::retrieveDatabase | ( | const QString & | path, | |
struct DBInfo * | db | |||
) | [virtual] |
Retrieve the database indicated by DBInfo *db
into the local file path
.
This copies all the data, and you can create a PilotLocalDatabase from the resulting path
.
- Returns:
true
on success
Implements KPilotLink.
Definition at line 875 of file kpilotdevicelink.cc.
void KPilotDeviceLink::setDevice | ( | const QString & | device | ) | [inline] |
Sets the device to use.
Used by probe dialog, since we know what device to use, but we don't want to start the detection immediately.
Definition at line 171 of file kpilotdevicelink.h.
void KPilotDeviceLink::setTempDevice | ( | const QString & | device | ) |
Sets an additional device, which should be tried as fallback.
Useful for hotplug enviroments, this device is used once for accepting a connection.
Definition at line 728 of file kpilotdevicelink.cc.
void KPilotDeviceLink::setWorkarounds | ( | bool | usb | ) | [inline] |
Special-cases.
Call this after a reset to set device- specific workarounds; the only one currently known is the Zire 31/72 T5 quirk of doing a non-HotSync connect when it's switched on.
Definition at line 154 of file kpilotdevicelink.h.
void KPilotDeviceLink::startCommThread | ( | ) | [protected] |
LinkStatus KPilotDeviceLink::status | ( | ) | const [inline] |
Get the status (state enum) of this link.
- Returns:
- The LinkStatus enum for the link's current state.
Definition at line 104 of file kpilotdevicelink.h.
QString KPilotDeviceLink::statusString | ( | ) | const [virtual] |
Provides a human-readable status string.
Implements KPilotLink.
Definition at line 829 of file kpilotdevicelink.cc.
QString KPilotDeviceLink::statusString | ( | LinkStatus | l | ) | [static] |
Get a human-readable string for the given status l
.
Definition at line 791 of file kpilotdevicelink.cc.
void KPilotDeviceLink::stopCommThread | ( | ) | [protected] |
Definition at line 595 of file kpilotdevicelink.cc.
bool KPilotDeviceLink::tickle | ( | ) | [virtual] |
Tickle the underlying device exactly once.
Implements KPilotLink.
Definition at line 734 of file kpilotdevicelink.cc.
Member Data Documentation
int KPilotDeviceLink::fAcceptedCount [protected] |
Handle cases where we can't accept or open the device, and data remains available on the pilot socket.
Definition at line 206 of file kpilotdevicelink.h.
DeviceCommThread* KPilotDeviceLink::fDeviceCommThread [protected] |
Definition at line 216 of file kpilotdevicelink.h.
Messages* KPilotDeviceLink::fMessages [protected] |
Definition at line 215 of file kpilotdevicelink.h.
int KPilotDeviceLink::fPilotSocket [protected] |
Pilot-link library handles for the device once it's opened.
Definition at line 199 of file kpilotdevicelink.h.
QString KPilotDeviceLink::fRealPilotPath [protected] |
Path with resolved symlinks, to prevent double binding to the same device.
Definition at line 194 of file kpilotdevicelink.h.
QString KPilotDeviceLink::fTempDevice [protected] |
Definition at line 200 of file kpilotdevicelink.h.
bool KPilotDeviceLink::fWorkaroundUSB [protected] |
Should we work around the Zire31/72 quirk?
- See also:
- setWorkarounds()
Definition at line 179 of file kpilotdevicelink.h.
The documentation for this class was generated from the following files: