kpilot
PilotLocalDatabase Class Reference
PilotLocalDatabase represents databases in the same binary format as on the handheld but which are stored on local disk. More...
#include <pilotLocalDatabase.h>
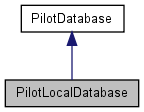
Public Member Functions | |
char * | appInfo () |
int | appInfoSize () const |
virtual int | cleanup () |
virtual bool | createDatabase (long creator=0, long type=0, int cardno=0, int flags=0, int version=0) |
virtual QString | dbPathName () const |
virtual DBType | dbType () const |
virtual int | deleteDatabase () |
virtual int | deleteRecord (recordid_t id, bool all=false) |
const PilotRecord * | findNextNewRecord () |
struct DBInfo & | getDBInfo () const |
QString | getDBName () const |
virtual QValueList< recordid_t > | idList () |
PilotLocalDatabase (const QString &name) | |
PilotLocalDatabase (const QString &path, const QString &name, bool useDefaultPath=true) | |
virtual int | readAppBlock (unsigned char *buffer, int maxLen) |
virtual PilotRecord * | readNextModifiedRec (int *ind=0L) |
virtual PilotRecord * | readNextRecInCategory (int category) |
virtual PilotRecord * | readRecordById (recordid_t id) |
virtual PilotRecord * | readRecordByIndex (int index) |
virtual unsigned int | recordCount () const |
virtual int | resetDBIndex () |
virtual int | resetSyncFlags () |
void | setDBInfo (const struct DBInfo &dbi) |
virtual recordid_t | updateID (recordid_t id) |
virtual int | writeAppBlock (unsigned char *buffer, int len) |
virtual recordid_t | writeRecord (PilotRecord *newRecord) |
virtual | ~PilotLocalDatabase () |
Static Public Member Functions | |
static const QString & | getDBPath () |
static bool | infoFromFile (const QString &path, DBInfo *d) |
static void | setDBPath (const QString &) |
Protected Member Functions | |
virtual void | closeDatabase () |
void | fixupDBName () |
virtual void | openDatabase () |
Detailed Description
PilotLocalDatabase represents databases in the same binary format as on the handheld but which are stored on local disk.Definition at line 43 of file pilotLocalDatabase.h.
Constructor & Destructor Documentation
PilotLocalDatabase::PilotLocalDatabase | ( | const QString & | path, | |
const QString & | name, | |||
bool | useDefaultPath = true | |||
) |
Opens the local database.
If the database cannot be found at the given position, a default path is used ($KDEHOME/share/apps/kpilot/DBBackup) and if the file is found there, it is opened. In some cases this should not be done, so the parameter useDefaultPath controls this behavior. If it is set to true, the default path is used if the file cannot be found in the explicitly given location. If it is set to false and the database cannot be found, no database is opened. It can then be created explicitly at the specified location.
Definition at line 84 of file pilotLocalDatabase.cc.
PilotLocalDatabase::PilotLocalDatabase | ( | const QString & | name | ) |
Opens the local database.
This is primarily for testing purposes; only tries the given path.
Definition at line 118 of file pilotLocalDatabase.cc.
PilotLocalDatabase::~PilotLocalDatabase | ( | ) | [virtual] |
Definition at line 143 of file pilotLocalDatabase.cc.
Member Function Documentation
char* PilotLocalDatabase::appInfo | ( | ) | [inline] |
Definition at line 151 of file pilotLocalDatabase.h.
int PilotLocalDatabase::appInfoSize | ( | ) | const [inline] |
Accessor functions for the application info block.
Definition at line 149 of file pilotLocalDatabase.h.
int PilotLocalDatabase::cleanup | ( | ) | [virtual] |
Purges all Archived/Deleted records from Palm Pilot database.
Implements PilotDatabase.
Definition at line 559 of file pilotLocalDatabase.cc.
void PilotLocalDatabase::closeDatabase | ( | ) | [protected, virtual] |
bool PilotLocalDatabase::createDatabase | ( | long | creator = 0 , |
|
long | type = 0 , |
|||
int | cardno = 0 , |
|||
int | flags = 0 , |
|||
int | version = 0 | |||
) | [virtual] |
Creates the database with the given creator, type and flags on the given card (default is RAM).
If the database already exists, this function does nothing.
Implements PilotDatabase.
Definition at line 159 of file pilotLocalDatabase.cc.
QString PilotLocalDatabase::dbPathName | ( | ) | const [virtual] |
Returns the full path of the current database, based on the path and dbname passed to the constructor, and including the .pdb extension.
Implements PilotDatabase.
Definition at line 591 of file pilotLocalDatabase.cc.
PilotDatabase::DBType PilotLocalDatabase::dbType | ( | ) | const [virtual] |
int PilotLocalDatabase::deleteDatabase | ( | ) | [virtual] |
Deletes the database (by name, as given in the constructor and stored in the fDBName field.
)
Implements PilotDatabase.
Definition at line 200 of file pilotLocalDatabase.cc.
int PilotLocalDatabase::deleteRecord | ( | recordid_t | id, | |
bool | all = false | |||
) | [virtual] |
Deletes a record with the given recordid_t from the database, or all records, if all is set to true.
The recordid_t will be ignored in this case. Return value is negative on error, 0 otherwise.
Implements PilotDatabase.
Definition at line 491 of file pilotLocalDatabase.cc.
const PilotRecord * PilotLocalDatabase::findNextNewRecord | ( | ) |
Returns the next "new" record, ie.
the next record that has not been synced yet. These records all have ID=0, so are not easy to find with the other methods. The record is the one contained in the database, not a copy like the read*() functions give you -- so be careful with it. Don't delete it, in any case. Casting it to non-const and marking it deleted is OK, though, which is mostly its intended use.
Definition at line 371 of file pilotLocalDatabase.cc.
void PilotLocalDatabase::fixupDBName | ( | ) | [protected] |
Definition at line 153 of file pilotLocalDatabase.cc.
struct DBInfo& PilotLocalDatabase::getDBInfo | ( | ) | const [inline, read] |
Definition at line 153 of file pilotLocalDatabase.h.
QString PilotLocalDatabase::getDBName | ( | ) | const [inline] |
Return the name of the database (as it would be on the handheld).
Definition at line 137 of file pilotLocalDatabase.h.
static const QString& PilotLocalDatabase::getDBPath | ( | ) | [inline, static] |
QValueList< recordid_t > PilotLocalDatabase::idList | ( | ) | [virtual] |
Returns a QValueList of all record ids in the database.
This implementation is really bad.
Reimplemented from PilotDatabase.
Definition at line 273 of file pilotLocalDatabase.cc.
bool PilotLocalDatabase::infoFromFile | ( | const QString & | path, | |
DBInfo * | d | |||
) | [static] |
Reads local file path
and fills in the DBInfo structure d
with the DBInfo from the file.
- Returns:
false
if d is NULLfalse
if the filepath
does not existtrue
if reading the DBInfo succeeds
- Note:
- Relatively expensive operation, since the pilot-link library doesn't provide a cheap way of getting this information.
Definition at line 734 of file pilotLocalDatabase.cc.
void PilotLocalDatabase::openDatabase | ( | ) | [protected, virtual] |
int PilotLocalDatabase::readAppBlock | ( | unsigned char * | buffer, | |
int | maxLen | |||
) | [virtual] |
Reads the application block info, returns size.
Implements PilotDatabase.
Definition at line 223 of file pilotLocalDatabase.cc.
PilotRecord * PilotLocalDatabase::readNextModifiedRec | ( | int * | ind = 0L |
) | [virtual] |
Reads the next record from database that has the dirty flag set.
ind (if a valid pointer is given) will receive the index of the returned record.
Implements PilotDatabase.
Definition at line 396 of file pilotLocalDatabase.cc.
PilotRecord * PilotLocalDatabase::readNextRecInCategory | ( | int | category | ) | [virtual] |
Reads the next record from database in category 'category'.
Implements PilotDatabase.
Definition at line 347 of file pilotLocalDatabase.cc.
PilotRecord * PilotLocalDatabase::readRecordById | ( | recordid_t | id | ) | [virtual] |
Reads a record from database by id, returns record length.
Implements PilotDatabase.
Definition at line 292 of file pilotLocalDatabase.cc.
PilotRecord * PilotLocalDatabase::readRecordByIndex | ( | int | index | ) | [virtual] |
Reads a record from database, returns the record length.
Implements PilotDatabase.
Definition at line 317 of file pilotLocalDatabase.cc.
unsigned int PilotLocalDatabase::recordCount | ( | ) | const [virtual] |
Returns the number of records in the database.
If the database is not open, return -1.
Implements PilotDatabase.
Definition at line 259 of file pilotLocalDatabase.cc.
int PilotLocalDatabase::resetDBIndex | ( | ) | [virtual] |
Resets next record index to beginning.
Implements PilotDatabase.
Definition at line 546 of file pilotLocalDatabase.cc.
int PilotLocalDatabase::resetSyncFlags | ( | ) | [virtual] |
Resets all records in the database to not dirty.
Implements PilotDatabase.
Definition at line 528 of file pilotLocalDatabase.cc.
void PilotLocalDatabase::setDBInfo | ( | const struct DBInfo & | dbi | ) | [inline] |
Definition at line 154 of file pilotLocalDatabase.h.
void PilotLocalDatabase::setDBPath | ( | const QString & | s | ) | [static] |
For databases opened by name only (constructor 2 -- which is the preferred one, too) try this path first before the default path.
Set statically so it's shared for all local databases.
Definition at line 709 of file pilotLocalDatabase.cc.
recordid_t PilotLocalDatabase::updateID | ( | recordid_t | id | ) | [virtual] |
Update the ID of the current record in the database with the specified.
- Parameters:
-
id . This is allowed only after reading or writing a modified or new record.
Definition at line 430 of file pilotLocalDatabase.cc.
int PilotLocalDatabase::writeAppBlock | ( | unsigned char * | buffer, | |
int | len | |||
) | [virtual] |
Writes the application block info.
Implements PilotDatabase.
Definition at line 240 of file pilotLocalDatabase.cc.
recordid_t PilotLocalDatabase::writeRecord | ( | PilotRecord * | newRecord | ) | [virtual] |
Writes a new record to database (if 'id' == 0, one will be assigned to newRecord).
Implements PilotDatabase.
Definition at line 450 of file pilotLocalDatabase.cc.
The documentation for this class was generated from the following files: