kpilot
PilotDatabase Class Reference
Methods to access a database on the pilot. More...
#include <pilotDatabase.h>
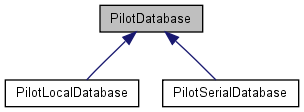
Public Types | |
enum | DBType { eNone = 0, eLocalDB = 1, eSerialDB = 2 } |
Public Member Functions | |
virtual int | cleanup ()=0 |
virtual bool | createDatabase (long creator=0, long type=0, int cardno=0, int flags=0, int version=0)=0 |
virtual QString | dbPathName () const =0 |
virtual DBType | dbType () const =0 |
virtual int | deleteDatabase ()=0 |
virtual int | deleteRecord (recordid_t id, bool all=false)=0 |
virtual Pilot::RecordIDList | idList () |
bool | isOpen () const |
virtual Pilot::RecordIDList | modifiedIDList () |
QString | name () const |
PilotDatabase (const QString &name=QString::null) | |
virtual int | readAppBlock (unsigned char *buffer, int maxLen)=0 |
virtual PilotRecord * | readNextModifiedRec (int *ind=NULL)=0 |
virtual PilotRecord * | readNextRecInCategory (int category)=0 |
virtual PilotRecord * | readRecordById (recordid_t id)=0 |
virtual PilotRecord * | readRecordByIndex (int index)=0 |
virtual unsigned int | recordCount () const =0 |
virtual int | resetDBIndex ()=0 |
virtual int | resetSyncFlags ()=0 |
virtual int | writeAppBlock (unsigned char *buffer, int len)=0 |
virtual recordid_t | writeRecord (PilotRecord *newRecord)=0 |
virtual | ~PilotDatabase () |
Static Public Member Functions | |
static int | instanceCount () |
static bool | isResource (struct DBInfo *info) |
Protected Member Functions | |
virtual void | closeDatabase ()=0 |
virtual void | openDatabase ()=0 |
void | setDBOpen (bool yesno) |
Detailed Description
Methods to access a database on the pilot.NOTE: It is the users responsibility to delete PilotRecords returned by PilotDatabase methods when finished with them!
Definition at line 50 of file pilotDatabase.h.
Member Enumeration Documentation
Use this instead of RTTI to determine the type of a PilotDatabase, for those cases where it's important.
Definition at line 155 of file pilotDatabase.h.
Constructor & Destructor Documentation
PilotDatabase::PilotDatabase | ( | const QString & | name = QString::null |
) |
Definition at line 47 of file pilotDatabase.cc.
PilotDatabase::~PilotDatabase | ( | ) | [virtual] |
Definition at line 60 of file pilotDatabase.cc.
Member Function Documentation
virtual int PilotDatabase::cleanup | ( | ) | [pure virtual] |
Purges all Archived/Deleted records from Palm Pilot database.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual void PilotDatabase::closeDatabase | ( | ) | [protected, pure virtual] |
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual bool PilotDatabase::createDatabase | ( | long | creator = 0 , |
|
long | type = 0 , |
|||
int | cardno = 0 , |
|||
int | flags = 0 , |
|||
int | version = 0 | |||
) | [pure virtual] |
Creates the database with the given creator, type and flags on the given card (default is RAM).
If the database already exists, this function does nothing.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual QString PilotDatabase::dbPathName | ( | ) | const [pure virtual] |
Returns some sensible human-readable identifier for the database.
Serial databases get Pilot:, local databases return the full path.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual DBType PilotDatabase::dbType | ( | ) | const [pure virtual] |
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual int PilotDatabase::deleteDatabase | ( | ) | [pure virtual] |
Deletes the database (by name, as given in the constructor, the database name is stored depending on the implementation of PilotLocalDatabase and PilotSerialDatabas).
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual int PilotDatabase::deleteRecord | ( | recordid_t | id, | |
bool | all = false | |||
) | [pure virtual] |
Deletes a record with the given recordid_t from the database, or all records, if all
is set to true.
The recordid_t will be ignored in this case.
Return value is negative on error, 0 otherwise.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
Pilot::RecordIDList PilotDatabase::idList | ( | ) | [virtual] |
Returns a QValueList of all record ids in the database.
This implementation is really bad.
Reimplemented in PilotLocalDatabase, and PilotSerialDatabase.
Definition at line 82 of file pilotDatabase.cc.
int PilotDatabase::instanceCount | ( | ) | [static] |
Debugging information: tally how many databases are created or destroyed.
Returns the count of currently existing databases.
Definition at line 70 of file pilotDatabase.cc.
bool PilotDatabase::isOpen | ( | ) | const [inline] |
Definition at line 143 of file pilotDatabase.h.
static bool PilotDatabase::isResource | ( | struct DBInfo * | info | ) | [inline, static] |
Definition at line 160 of file pilotDatabase.h.
Pilot::RecordIDList PilotDatabase::modifiedIDList | ( | ) | [virtual] |
Returns a list of all record ids that have been modified in the database.
This implementation is really bad.
Definition at line 97 of file pilotDatabase.cc.
QString PilotDatabase::name | ( | ) | const [inline] |
Definition at line 57 of file pilotDatabase.h.
virtual void PilotDatabase::openDatabase | ( | ) | [protected, pure virtual] |
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual int PilotDatabase::readAppBlock | ( | unsigned char * | buffer, | |
int | maxLen | |||
) | [pure virtual] |
Reads the application block info, returns size.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual PilotRecord* PilotDatabase::readNextModifiedRec | ( | int * | ind = NULL |
) | [pure virtual] |
Reads the next record from database that has the dirty flag set.
If ind
is non-NULL, *ind is set to the index of the current record (i.e. before the record pointer moves to the next modified record).
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual PilotRecord* PilotDatabase::readNextRecInCategory | ( | int | category | ) | [pure virtual] |
Reads the next record from database in category 'category'.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual PilotRecord* PilotDatabase::readRecordById | ( | recordid_t | id | ) | [pure virtual] |
Reads a record from database by id, returns record length.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual PilotRecord* PilotDatabase::readRecordByIndex | ( | int | index | ) | [pure virtual] |
Reads a record from database, returns the record length.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual unsigned int PilotDatabase::recordCount | ( | ) | const [pure virtual] |
Returns the number of records in the database.
If the database is not open, return -1.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
virtual int PilotDatabase::resetDBIndex | ( | ) | [pure virtual] |
virtual int PilotDatabase::resetSyncFlags | ( | ) | [pure virtual] |
Resets all records in the database to not dirty.
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
void PilotDatabase::setDBOpen | ( | bool | yesno | ) | [inline, protected] |
Definition at line 169 of file pilotDatabase.h.
virtual int PilotDatabase::writeAppBlock | ( | unsigned char * | buffer, | |
int | len | |||
) | [pure virtual] |
virtual recordid_t PilotDatabase::writeRecord | ( | PilotRecord * | newRecord | ) | [pure virtual] |
Writes a new record to database (if 'id' == 0, one will be assigned to newRecord).
Implemented in PilotLocalDatabase, and PilotSerialDatabase.
The documentation for this class was generated from the following files: