kpilot
KPilotLocalLink Class Reference
Implementation of the device link for file-system backed (ie. More...
#include <kpilotlocallink.h>
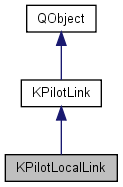
Public Slots | |
void | ready () |
Public Member Functions | |
virtual void | close () |
virtual PilotDatabase * | database (const QString &name) |
virtual void | endSync (EndOfSyncFlags f) |
virtual int | findDatabase (const char *name, struct DBInfo *, int index=0, unsigned long type=0, unsigned long creator=0) |
virtual const KPilotCard * | getCardInfo (int card) |
virtual DBInfoList | getDBList (int cardno=0, int flags=dlpDBListRAM) |
virtual int | getNextDatabase (int index, struct DBInfo *) |
virtual bool | isConnected () const |
KPilotLocalLink (QObject *parent=0L, const char *name=0L) | |
virtual int | openConduit () |
virtual void | reset () |
virtual void | reset (const QString &) |
virtual bool | retrieveDatabase (const QString &path, struct DBInfo *db) |
virtual QString | statusString () const |
virtual bool | tickle () |
virtual | ~KPilotLocalLink () |
Protected Member Functions | |
virtual void | addSyncLogEntryImpl (const QString &s) |
unsigned int | findAvailableDatabases (Private &, const QString &path) |
virtual bool | installFile (const QString &, const bool deleteFile) |
virtual int | pilotSocket () const |
Protected Attributes | |
Private * | d |
QString | fPath |
bool | fReady |
Detailed Description
Implementation of the device link for file-system backed (ie.local, fake) devices. Uses a directory specified in the reset() call to serve databases.
Definition at line 43 of file kpilotlocallink.h.
Constructor & Destructor Documentation
KPilotLocalLink::KPilotLocalLink | ( | QObject * | parent = 0L , |
|
const char * | name = 0L | |||
) |
Definition at line 121 of file kpilotlocallink.cc.
KPilotLocalLink::~KPilotLocalLink | ( | ) | [virtual] |
Definition at line 129 of file kpilotlocallink.cc.
Member Function Documentation
void KPilotLocalLink::addSyncLogEntryImpl | ( | const QString & | s | ) | [protected, virtual] |
Actually write an entry to the device link.
The message s
must be guaranteed non-empty.
Implements KPilotLink.
Definition at line 263 of file kpilotlocallink.cc.
void KPilotLocalLink::close | ( | ) | [virtual] |
Release all resources, including the master pilot socket, timers, etc.
Implements KPilotLink.
Definition at line 171 of file kpilotlocallink.cc.
PilotDatabase * KPilotLocalLink::database | ( | const QString & | name | ) | [virtual] |
Return a database object for manipulating the database with name name
on the link.
This database may be local or remote, depending on the kind of link in use.
- Returns:
- pointer to database object, or 0 on error.
- Note:
- ownership of the database object is given to the caller, who must delete the object in time.
Implements KPilotLink.
Definition at line 353 of file kpilotlocallink.cc.
void KPilotLocalLink::endSync | ( | EndOfSyncFlags | f | ) | [virtual] |
End the sync in a gracuful manner.
If f
is UpdateUserInfo, the sync was successful and the user info and last successful sync timestamp are updated.
Implements KPilotLink.
Definition at line 186 of file kpilotlocallink.cc.
unsigned int KPilotLocalLink::findAvailableDatabases | ( | KPilotLocalLink::Private & | info, | |
const QString & | path | |||
) | [protected] |
Pre-process the directory path
to find out which databases live there.
- Returns:
- Number of database in
path
.
Definition at line 77 of file kpilotlocallink.cc.
int KPilotLocalLink::findDatabase | ( | const char * | name, | |
struct DBInfo * | info, | |||
int | index = 0 , |
|||
unsigned long | type = 0 , |
|||
unsigned long | creator = 0 | |||
) | [virtual] |
Find a database with the given name
(and optionally, type type
and creator ID (from pi_mktag) creator
, on searching from index index
on the handheld.
Fills in the DBInfo structure info
if found.
- Returns:
- >=0 on success. See the documentation for each subclass for particular meanings.
< 0 on error.
Implements KPilotLink.
Definition at line 221 of file kpilotlocallink.cc.
const KPilotCard * KPilotLocalLink::getCardInfo | ( | int | card | ) | [virtual] |
Retrieve information about the data card card
; I don't think that any pilot supports card numbers other than 0.
Non-device links return something fake.
This function may return NULL (non-device links or on error).
- Note:
- Ownership of the KPilotCard object is given to the caller, who must delete it.
Implements KPilotLink.
Definition at line 181 of file kpilotlocallink.cc.
KPilotLink::DBInfoList KPilotLocalLink::getDBList | ( | int | cardno = 0 , |
|
int | flags = dlpDBListRAM | |||
) | [virtual] |
Returns a list of DBInfo structures describing all the databases available on the link (ie.
device) with the given card number cardno
and flags flags
. No known handheld uses a cardno other than 0; use flags to indicate what kind of databases to fetch -- dlpDBListRAM
or dlpDBListROM
.
- Returns:
- list of DBInfo objects, one for each database
- Note:
- ownership of the DBInfo objects is passed to the caller, who must delete the objects.
Implements KPilotLink.
Definition at line 340 of file kpilotlocallink.cc.
int KPilotLocalLink::getNextDatabase | ( | int | index, | |
struct DBInfo * | db | |||
) | [virtual] |
Fill the DBInfo structure db
with information about the next database (in some ordering) counting from index
.
- Returns:
- < 0 on error
Implements KPilotLink.
Definition at line 199 of file kpilotlocallink.cc.
bool KPilotLocalLink::installFile | ( | const QString & | f, | |
const bool | deleteFile | |||
) | [protected, virtual] |
Install a single file onto the device link.
Full pathname f
is used; in addition, if deleteFile
is true remove the source file. Returns true
if the install succeeded.
Implements KPilotLink.
Definition at line 269 of file kpilotlocallink.cc.
bool KPilotLocalLink::isConnected | ( | ) | const [virtual] |
True if HotSync has been started but not finished yet (ie.
the physical Pilot is waiting for sync commands)
Implements KPilotLink.
Definition at line 140 of file kpilotlocallink.cc.
int KPilotLocalLink::openConduit | ( | ) | [virtual] |
Notify the Pilot user that a conduit is running now.
On real devices, this prints out (on screen) which database is now opened; useful for progress reporting.
- Returns:
- -1 on error
- Note:
- the default implementation returns 0
Reimplemented from KPilotLink.
Definition at line 192 of file kpilotlocallink.cc.
virtual int KPilotLocalLink::pilotSocket | ( | ) | const [inline, protected, virtual] |
Returns a file handle for raw operations.
Not recommended. On links with no physical device backing, returns -1.
- Note:
- the default implementation returns -1
Reimplemented from KPilotLink.
Definition at line 72 of file kpilotlocallink.h.
void KPilotLocalLink::ready | ( | ) | [slot] |
Definition at line 361 of file kpilotlocallink.cc.
void KPilotLocalLink::reset | ( | ) | [virtual] |
Assuming things have been set up at least once already by a call to reset() with parameters, use this slot to re-start with the same settings.
Implements KPilotLink.
Definition at line 152 of file kpilotlocallink.cc.
void KPilotLocalLink::reset | ( | const QString & | pilotPath | ) | [virtual] |
Return the device link to the Init state and try connecting to the given device path (if it's non-empty).
What the path means depends on the kind of link we're instantiating.
- See also:
- reset()
Implements KPilotLink.
Definition at line 145 of file kpilotlocallink.cc.
bool KPilotLocalLink::retrieveDatabase | ( | const QString & | path, | |
struct DBInfo * | db | |||
) | [virtual] |
Retrieve the database indicated by DBInfo *db
into the local file path
.
This copies all the data, and you can create a PilotLocalDatabase from the resulting path
.
- Returns:
true
on success
Implements KPilotLink.
Definition at line 296 of file kpilotlocallink.cc.
QString KPilotLocalLink::statusString | ( | ) | const [virtual] |
Provides a human-readable status string.
Implements KPilotLink.
Definition at line 135 of file kpilotlocallink.cc.
bool KPilotLocalLink::tickle | ( | ) | [virtual] |
Tickle the underlying device exactly once.
Implements KPilotLink.
Definition at line 176 of file kpilotlocallink.cc.
Member Data Documentation
Private* KPilotLocalLink::d [protected] |
Definition at line 81 of file kpilotlocallink.h.
QString KPilotLocalLink::fPath [protected] |
Definition at line 79 of file kpilotlocallink.h.
bool KPilotLocalLink::fReady [protected] |
Definition at line 78 of file kpilotlocallink.h.
The documentation for this class was generated from the following files: