kpilot
SyncAction Class Reference
#include <syncAction.h>
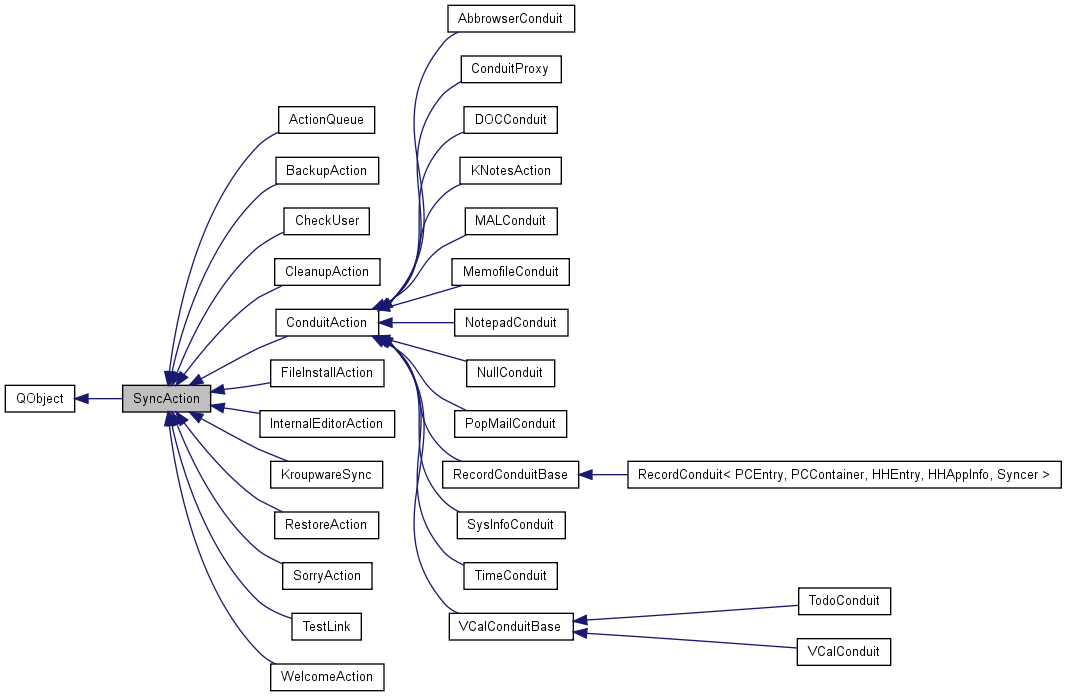
Classes | |
class | SyncMode |
This class encapsulates the different sync modes that can be used, and enforces a little discipline in changing the mode and messing around in general. More... | |
Public Types | |
enum | BackupFrequency { eEveryHotSync = 0, eOnRequestOnly } |
enum | ConflictResolution { eUseGlobalSetting = -1, eAskUser = 0, eDoNothing, eHHOverrides, ePCOverrides, ePreviousSyncOverrides, eDuplicate, eDelete, eCROffset = -1 } |
enum | Status { Error = -1 } |
Public Slots | |
void | execConduit () |
Signals | |
void | logError (const QString &) |
void | logMessage (const QString &) |
void | logProgress (const QString &, int) |
void | syncDone (SyncAction *) |
void | timeout () |
Public Member Functions | |
void | addLogError (const QString &msg) |
void | addLogMessage (const QString &msg) |
void | addLogProgress (const QString &msg, int prog) |
void | addSyncLogEntry (const QString &e, bool log=true) |
int | status () const |
virtual QString | statusString () const |
SyncAction (KPilotLink *p, QWidget *visibleparent, const char *name=0L) | |
SyncAction (KPilotLink *p, const char *name=0L) | |
~SyncAction () | |
Protected Slots | |
void | delayedDoneSlot () |
Protected Member Functions | |
bool | delayDone () |
KPilotLink * | deviceLink () const |
virtual bool | exec ()=0 |
int | openConduit () |
int | pilotSocket () const |
int | questionYesNo (const QString &question, const QString &caption=QString::null, const QString &key=QString::null, unsigned timeout=20, const QString &yes=QString::null, const QString &no=QString::null) |
int | questionYesNoCancel (const QString &question, const QString &caption=QString::null, const QString &key=QString::null, unsigned timeout=20, const QString &yes=QString::null, const QString &no=QString::null) |
void | startTickle (unsigned count=0) |
void | stopTickle () |
Protected Attributes | |
int | fActionStatus |
KPilotLink * | fHandle |
QWidget * | fParent |
Detailed Description
Definition at line 50 of file syncAction.h.
Member Enumeration Documentation
This MUST stay in sync with the combobox in kpilotConfigDialog_backup.ui.
If it does not, you need to either change this enum or the combobox.
Definition at line 354 of file syncAction.h.
- Enumerator:
-
eUseGlobalSetting eAskUser eDoNothing eHHOverrides ePCOverrides ePreviousSyncOverrides eDuplicate eDelete eCROffset
Definition at line 336 of file syncAction.h.
enum SyncAction::Status |
Reimplemented in KNotesAction, BackupAction, and RestoreAction.
Definition at line 62 of file syncAction.h.
Constructor & Destructor Documentation
SyncAction::SyncAction | ( | KPilotLink * | p, | |
const char * | name = 0L | |||
) |
Definition at line 58 of file syncAction.cc.
SyncAction::SyncAction | ( | KPilotLink * | p, | |
QWidget * | visibleparent, | |||
const char * | name = 0L | |||
) |
Definition at line 67 of file syncAction.cc.
SyncAction::~SyncAction | ( | ) |
Definition at line 77 of file syncAction.cc.
Member Function Documentation
void SyncAction::addLogError | ( | const QString & | msg | ) | [inline] |
Log an error message in KPilot (the PC side of things).
Definition at line 146 of file syncAction.h.
void SyncAction::addLogMessage | ( | const QString & | msg | ) | [inline] |
Public API for adding a message to the log in KPilot.
Adds msg
to the synclog maintained on the PC.
Definition at line 141 of file syncAction.h.
void SyncAction::addLogProgress | ( | const QString & | msg, | |
int | prog | |||
) | [inline] |
void SyncAction::addSyncLogEntry | ( | const QString & | e, | |
bool | log = true | |||
) | [inline] |
Public API for adding a sync log entry, see the implementation in KPilotLink::addSyncLogEntry().
- Parameters:
-
e Message to add to the sync log log If true
, also add the entry to the log in KPilot
- Note:
- Having messages appear on the handheld but not in KPilot should be a very rare occurrence.
Definition at line 131 of file syncAction.h.
bool SyncAction::delayDone | ( | ) | [protected] |
It might not be safe to emit syncDone() from exec().
So instead, call delayDone() to wait for the main event loop to return if you manage to do all processing immediately.
delayDone() returns true, so that return delayDone(); is a sensible final statement in exec().
Definition at line 114 of file syncAction.cc.
void SyncAction::delayedDoneSlot | ( | ) | [protected, slot] |
This slot emits syncDone(), and does nothing else.
This is safe, since the method returns immediately after the emit -- even if syncDone() causes the SyncAction to be deleted.
Definition at line 109 of file syncAction.cc.
KPilotLink* SyncAction::deviceLink | ( | ) | const [inline, protected] |
virtual bool SyncAction::exec | ( | ) | [protected, pure virtual] |
This function starts the actual processing done by the conduit.
It should return false if the processing cannot be initiated, f.ex. because some parameters were not set or a needed library is missing. This will be reported to the user. It should return true if processing is started normally. If processing starts normally, it is the _conduit's_ responsibility to eventually emit syncDone(); if processing does not start normally (ie. exec() returns false) then the environment will deal with syncDone().
Implemented in AbbrowserConduit, DOCConduit, KNotesAction, MALConduit, MemofileConduit, NotepadConduit, NullConduit, PopMailConduit, SysInfoConduit, TimeConduit, VCalConduitBase, CheckUser, BackupAction, FileInstallAction, RestoreAction, InternalEditorAction, KroupwareSync, ActionQueue, WelcomeAction, SorryAction, CleanupAction, TestLink, ConduitProxy, and RecordConduitBase.
void SyncAction::execConduit | ( | ) | [slot] |
void SyncAction::logError | ( | const QString & | ) | [signal] |
void SyncAction::logMessage | ( | const QString & | ) | [signal] |
void SyncAction::logProgress | ( | const QString & | , | |
int | ||||
) | [signal] |
int SyncAction::openConduit | ( | ) | [inline, protected] |
Tells the handheld device that someone is talking to it now.
Useful (repeatedly) to inform the user of what is going on. May return < 0 on error (or if there is no device attached).
Definition at line 179 of file syncAction.h.
int SyncAction::pilotSocket | ( | ) | const [inline, protected] |
Returns the file descriptor for the device link -- that is, the raw handle to the OS's connection to the device.
Use with care. May return -1 if there is no device.
Definition at line 170 of file syncAction.h.
int SyncAction::questionYesNo | ( | const QString & | question, | |
const QString & | caption = QString::null , |
|||
const QString & | key = QString::null , |
|||
unsigned | timeout = 20 , |
|||
const QString & | yes = QString::null , |
|||
const QString & | no = QString::null | |||
) | [protected] |
Ask a yes-no question of the user.
This has a timeout so that you don't wait forever for inattentive users. It's much like KMessageBox::questionYesNo(), but with this extra timeout-on- no-answer feature. Returns a KDialogBase::ButtonCode value - Yes,No or Cancel on timeout. If there is a key set and the user indicates not to ask again, the selected answer (Yes or No) is remembered for future reference.
caption
Message Box caption, uses "Question" if null. key
Key for the "Don't ask again" code. timeout
Timeout, in seconds.
Definition at line 295 of file syncAction.cc.
int SyncAction::questionYesNoCancel | ( | const QString & | question, | |
const QString & | caption = QString::null , |
|||
const QString & | key = QString::null , |
|||
unsigned | timeout = 20 , |
|||
const QString & | yes = QString::null , |
|||
const QString & | no = QString::null | |||
) | [protected] |
Definition at line 403 of file syncAction.cc.
void SyncAction::startTickle | ( | unsigned | count = 0 |
) | [protected] |
Call startTickle() some time before showing a dialog to the user (we're assuming a local event loop here) so that while the dialog is up and the user is thinking, the pilot stays awake.
Afterwards, call stopTickle().
The parameter to startTickle indicates the timeout, in seconds, before signal timeout is emitted. You can connect to that, again, to take down the user interface part if the user isn't reacting.
Definition at line 265 of file syncAction.cc.
int SyncAction::status | ( | ) | const [inline] |
A syncaction has a status, which can be expressed as an integer.
Subclasses are expected to define their own status values as needed.
Definition at line 68 of file syncAction.h.
QString SyncAction::statusString | ( | ) | const [virtual] |
Return a human-readable representation of the status.
Reimplemented in KNotesAction, BackupAction, FileInstallAction, and RestoreAction.
Definition at line 81 of file syncAction.cc.
void SyncAction::stopTickle | ( | ) | [protected] |
Definition at line 280 of file syncAction.cc.
void SyncAction::syncDone | ( | SyncAction * | ) | [signal] |
void SyncAction::timeout | ( | ) | [signal] |
Member Data Documentation
int SyncAction::fActionStatus [protected] |
Definition at line 158 of file syncAction.h.
KPilotLink* SyncAction::fHandle [protected] |
QWidget* SyncAction::fParent [protected] |
Definition at line 381 of file syncAction.h.
The documentation for this class was generated from the following files: