kpilot
RecordConduitBase Class Reference
An intermediate class that introduces the slots we need for our sync implementation. More...
#include <recordConduit.h>
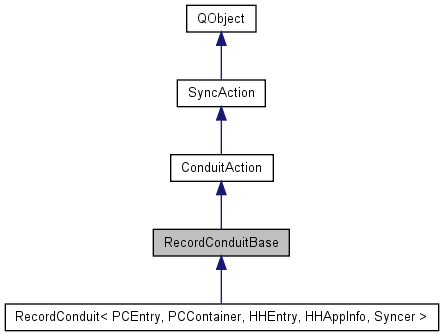
Public Types | |
enum | States { Initialize, PalmToPC, PCToPalm, Cleanup } |
enum | SyncProgress { NotDone = 0, Done = 1, Error = 2 } |
Public Member Functions | |
RecordConduitBase (KPilotDeviceLink *o, const char *n, const QStringList a=QStringList()) | |
virtual | ~RecordConduitBase () |
Static Public Member Functions | |
static QString | name (States s) |
static QString | name (SyncProgress s) |
Protected Slots | |
void | process () |
Protected Member Functions | |
virtual SyncProgress | cleanup ()=0 |
virtual bool | exec () |
virtual SyncProgress | loadPC ()=0 |
virtual SyncProgress | palmRecToPC ()=0 |
virtual SyncProgress | pcRecToPalm ()=0 |
Detailed Description
An intermediate class that introduces the slots we need for our sync implementation.This is here _only_ because mixing moc with template classes sounds really scary.
Definition at line 56 of file recordConduit.h.
Member Enumeration Documentation
State of the conduit's sync.
This is changed by process().
Definition at line 86 of file recordConduit.h.
Return values for the processing functions.
Each should return NotDone if it needs to be called again (e.g. to process another record), Done if it is finished and something else should be done, and Error if the sync cannot be completed.
Definition at line 80 of file recordConduit.h.
Constructor & Destructor Documentation
RecordConduitBase::RecordConduitBase | ( | KPilotDeviceLink * | o, | |
const char * | n, | |||
const QStringList | a = QStringList() | |||
) | [inline] |
Constructor.
The QStringList a
sets flags for the ConduitAction.
Definition at line 62 of file recordConduit.h.
virtual RecordConduitBase::~RecordConduitBase | ( | ) | [inline, virtual] |
Member Function Documentation
virtual SyncProgress RecordConduitBase::cleanup | ( | ) | [protected, pure virtual] |
Function called at the end of this conduit's sync, which should reset DB flags and write changed config data out to disk.
- Returns:
- Done when the cleanup is complete.
- See also:
- process()
Implemented in RecordConduit< PCEntry, PCContainer, HHEntry, HHAppInfo, Syncer >.
bool RecordConduitBase::exec | ( | ) | [protected, virtual] |
This function starts the actual processing done by the conduit.
It should return false if the processing cannot be initiated, f.ex. because some parameters were not set or a needed library is missing. This will be reported to the user. It should return true if processing is started normally. If processing starts normally, it is the _conduit's_ responsibility to eventually emit syncDone(); if processing does not start normally (ie. exec() returns false) then the environment will deal with syncDone().
Implements SyncAction.
Definition at line 59 of file recordConduit.cc.
virtual SyncProgress RecordConduitBase::loadPC | ( | ) | [protected, pure virtual] |
Function called at the beginning of a sync to load data from the PC.
- Returns:
- Done when the load has finished.
- See also:
- process
Implemented in RecordConduit< PCEntry, PCContainer, HHEntry, HHAppInfo, Syncer >.
QString RecordConduitBase::name | ( | RecordConduitBase::States | s | ) | [static] |
Definition at line 187 of file recordConduit.cc.
QString RecordConduitBase::name | ( | RecordConduitBase::SyncProgress | s | ) | [static] |
Returns a human-readable name for the progress indicator s
.
Definition at line 173 of file recordConduit.cc.
virtual SyncProgress RecordConduitBase::palmRecToPC | ( | ) | [protected, pure virtual] |
Function called repeatedly to fetch the next modified entry from the Palm and sync it with the PC by looking up the record, and calling the syncer for it.
- Returns:
- Dome when there are no more modified records on the Palm
- See also:
- process()
Implemented in RecordConduit< PCEntry, PCContainer, HHEntry, HHAppInfo, Syncer >.
virtual SyncProgress RecordConduitBase::pcRecToPalm | ( | ) | [protected, pure virtual] |
Function called repeatedly to fetch the next modified entry from the PC and sync it with the Palm by looking up the record and calling the syncer for it.
- Returns:
- Done when there are no more modified records on the PC
- See also:
- process()
Implemented in RecordConduit< PCEntry, PCContainer, HHEntry, HHAppInfo, Syncer >.
void RecordConduitBase::process | ( | ) | [protected, slot] |
Slot used for the implementation of a state machine: calls each of the relevant other slots (above) as needed until they return true.
Definition at line 84 of file recordConduit.cc.
The documentation for this class was generated from the following files: