kpilot
KNotesAction Class Reference
#include <knotes-action.h>
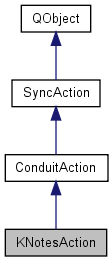
Public Types | |
enum | Status { Init, ModifiedNotesToPilot, DeleteNotesOnPilot, NewNotesToPilot, MemosToKNotes, Cleanup, Done } |
Public Member Functions | |
KNotesAction (KPilotLink *o, const char *n=0L, const QStringList &a=QStringList()) | |
virtual QString | statusString () const |
virtual | ~KNotesAction () |
Protected Slots | |
void | process () |
Protected Member Functions | |
void | addMemoToKNotes (const PilotMemo *) |
bool | addNewNoteToPilot () |
int | addNoteToPilot () |
void | cleanupMemos () |
bool | deleteNoteOnPilot () |
virtual bool | exec () |
void | getAppInfo () |
void | getConfigInfo () |
void | listNotes () |
bool | modifyNoteOnPilot () |
bool | openKNotesResource () |
void | resetIndexes () |
bool | syncMemoToKNotes () |
void | test () |
void | updateNote (const NoteAndMemo &, const PilotMemo *) |
Detailed Description
Definition at line 37 of file knotes-action.h.
Member Enumeration Documentation
enum KNotesAction::Status |
Constructor & Destructor Documentation
KNotesAction::KNotesAction | ( | KPilotLink * | o, | |
const char * | n = 0L , |
|||
const QStringList & | a = QStringList() | |||
) |
Definition at line 187 of file knotes-action.cc.
KNotesAction::~KNotesAction | ( | ) | [virtual] |
Definition at line 204 of file knotes-action.cc.
Member Function Documentation
void KNotesAction::addMemoToKNotes | ( | const PilotMemo * | memo | ) | [protected] |
bool KNotesAction::addNewNoteToPilot | ( | ) | [protected] |
Definition at line 547 of file knotes-action.cc.
int KNotesAction::addNoteToPilot | ( | ) | [protected] |
Add the Note currently being processed to the pilot as a new memo.
Returns the id of the record.
Definition at line 719 of file knotes-action.cc.
void KNotesAction::cleanupMemos | ( | ) | [protected] |
Definition at line 758 of file knotes-action.cc.
bool KNotesAction::deleteNoteOnPilot | ( | ) | [protected] |
Definition at line 515 of file knotes-action.cc.
bool KNotesAction::exec | ( | ) | [protected, virtual] |
This function starts the actual processing done by the conduit.
It should return false if the processing cannot be initiated, f.ex. because some parameters were not set or a needed library is missing. This will be reported to the user. It should return true if processing is started normally. If processing starts normally, it is the _conduit's_ responsibility to eventually emit syncDone(); if processing does not start normally (ie. exec() returns false) then the environment will deal with syncDone().
Implements SyncAction.
Definition at line 211 of file knotes-action.cc.
void KNotesAction::getAppInfo | ( | ) | [protected] |
For actual processing.
These are called by process and it is critical that fP->fIndex is set properly.
Each returns true when it is completely finished processing, if it returns a bool. Void functions need only be called once.
Definition at line 430 of file knotes-action.cc.
void KNotesAction::getConfigInfo | ( | ) | [protected] |
Definition at line 390 of file knotes-action.cc.
void KNotesAction::listNotes | ( | ) | [protected] |
bool KNotesAction::modifyNoteOnPilot | ( | ) | [protected] |
Definition at line 438 of file knotes-action.cc.
bool KNotesAction::openKNotesResource | ( | ) | [protected] |
Loads the KNotes resource and retrieve the list of notes it has.
- Returns:
- false if the the resource could not be opened and a new resource could not be created. Modifies fP to store the notes in.
Definition at line 254 of file knotes-action.cc.
void KNotesAction::process | ( | ) | [protected, slot] |
Definition at line 307 of file knotes-action.cc.
void KNotesAction::resetIndexes | ( | ) | [protected] |
Definition at line 278 of file knotes-action.cc.
QString KNotesAction::statusString | ( | ) | const [virtual] |
Return a human-readable representation of the status.
Reimplemented from SyncAction.
Definition at line 849 of file knotes-action.cc.
bool KNotesAction::syncMemoToKNotes | ( | ) | [protected] |
Definition at line 581 of file knotes-action.cc.
void KNotesAction::test | ( | ) | [protected] |
void KNotesAction::updateNote | ( | const NoteAndMemo & | m, | |
const PilotMemo * | memo | |||
) | [protected] |
Definition at line 690 of file knotes-action.cc.
The documentation for this class was generated from the following files: