libkcal
KCal::IncidenceBase Class Reference
This class provides the base class common to all calendar components. More...
#include <incidencebase.h>
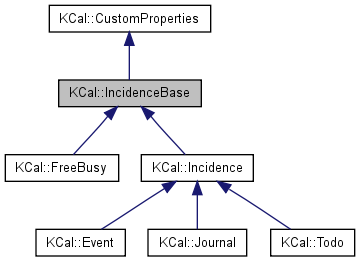
Classes | |
class | Observer |
class | Visitor |
This class provides the interface for a visitor of calendar components. More... | |
Public Types | |
enum | { SYNCNONE = 0, SYNCMOD = 1, SYNCDEL = 3 } |
Public Member Functions | |
virtual bool | accept (Visitor &) |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
Attendee * | attendeeByMail (const QString &) const |
Attendee * | attendeeByMails (const QStringList &, const QString &email=QString::null) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
bool | doesFloat () const |
virtual QDateTime | dtStart () const |
virtual QString | dtStartDateStr (bool shortfmt=true) const |
virtual QString | dtStartStr () const |
virtual QString | dtStartTimeStr () const |
int | duration () const |
bool | hasDuration () const |
IncidenceBase (const IncidenceBase &) | |
IncidenceBase () | |
bool | isReadOnly () const |
QDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &i) |
bool | operator== (const IncidenceBase &) const |
Person | organizer () const |
unsigned long | pilotId () const |
void | registerObserver (Observer *) |
bool | removeComment (const QString &comment) |
virtual void | setDtStart (const QDateTime &dtStart) |
virtual void | setDuration (int seconds) |
void | setFloats (bool f) |
void | setHasDuration (bool) |
void | setLastModified (const QDateTime &lm) |
void | setOrganizer (const QString &o) |
void | setOrganizer (const Person &o) |
void | setPilotId (unsigned long id) |
virtual void | setReadOnly (bool) |
void | setSyncStatus (int status) |
void | setSyncStatusSilent (int status) |
void | setUid (const QString &) |
int | syncStatus () const |
virtual QCString | type () const =0 |
QString | uid () const |
void | unRegisterObserver (Observer *) |
void | updated () |
void | updatedSilent () |
virtual | ~IncidenceBase () |
Protected Member Functions | |
virtual void | customPropertyUpdated () |
Protected Attributes | |
bool | mReadOnly |
Detailed Description
This class provides the base class common to all calendar components.Definition at line 45 of file incidencebase.h.
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
IncidenceBase::IncidenceBase | ( | ) |
Definition at line 33 of file incidencebase.cpp.
IncidenceBase::IncidenceBase | ( | const IncidenceBase & | i | ) |
Definition at line 42 of file incidencebase.cpp.
IncidenceBase::~IncidenceBase | ( | ) | [virtual] |
Definition at line 65 of file incidencebase.cpp.
Member Function Documentation
virtual bool KCal::IncidenceBase::accept | ( | Visitor & | ) | [inline, virtual] |
Accept IncidenceVisitor.
A class taking part in the visitor mechanism has to provide this implementation:
bool accept(Visitor &v) { return v.visit(this); }
Reimplemented in KCal::Journal.
Definition at line 107 of file incidencebase.h.
void IncidenceBase::addAttendee | ( | Attendee * | attendee, | |
bool | doUpdate = true | |||
) |
Add Attendee to this incidence.
IncidenceBase takes ownership of the Attendee object.
- Parameters:
-
attendee a pointer to the attendee to add doUpdate If true the Observers are notified, if false they are not.
Definition at line 262 of file incidencebase.cpp.
void IncidenceBase::addComment | ( | const QString & | comment | ) |
Add a comment to this incidence.
Does not add a linefeed character. Just appends the text as passed in.
- Parameters:
-
comment The comment to add.
Definition at line 231 of file incidencebase.cpp.
Attendee * IncidenceBase::attendeeByMails | ( | const QStringList & | emails, | |
const QString & | email = QString::null | |||
) | const |
Return first Attendee with one of the given email addresses.
Definition at line 311 of file incidencebase.cpp.
int KCal::IncidenceBase::attendeeCount | ( | ) | const [inline] |
const Attendee::List& KCal::IncidenceBase::attendees | ( | ) | const [inline] |
void IncidenceBase::clearAttendees | ( | ) |
void IncidenceBase::clearComments | ( | ) |
Delete all comments associated with this incidence.
Definition at line 251 of file incidencebase.cpp.
QStringList IncidenceBase::comments | ( | ) | const |
Return all comments associated with this incidence.
Definition at line 256 of file incidencebase.cpp.
void IncidenceBase::customPropertyUpdated | ( | ) | [protected, virtual] |
Called when a custom property has been changed.
The default implementation does nothing: override in derived classes to perform change processing.
Reimplemented from KCal::CustomProperties.
Definition at line 411 of file incidencebase.cpp.
bool IncidenceBase::doesFloat | ( | ) | const |
Return true or false depending on whether the incidence "floats," i.e.
has a date but no time attached to it.
Definition at line 218 of file incidencebase.cpp.
QDateTime IncidenceBase::dtStart | ( | ) | const [virtual] |
returns an event's starting date/time as a QDateTime.
Definition at line 197 of file incidencebase.cpp.
QString IncidenceBase::dtStartDateStr | ( | bool | shortfmt = true |
) | const [virtual] |
returns an event's starting date as a string formatted according to the users locale settings
Definition at line 207 of file incidencebase.cpp.
QString IncidenceBase::dtStartStr | ( | ) | const [virtual] |
returns an event's starting date and time as a string formatted according to the users locale settings
Definition at line 212 of file incidencebase.cpp.
QString IncidenceBase::dtStartTimeStr | ( | ) | const [virtual] |
returns an event's starting time as a string formatted according to the users locale settings
Definition at line 202 of file incidencebase.cpp.
int IncidenceBase::duration | ( | ) | const |
Definition at line 345 of file incidencebase.cpp.
bool IncidenceBase::hasDuration | ( | ) | const |
Definition at line 355 of file incidencebase.cpp.
bool KCal::IncidenceBase::isReadOnly | ( | ) | const [inline] |
QDateTime IncidenceBase::lastModified | ( | ) | const |
IncidenceBase & IncidenceBase::operator= | ( | const IncidenceBase & | i | ) |
Definition at line 69 of file incidencebase.cpp.
bool IncidenceBase::operator== | ( | const IncidenceBase & | i2 | ) | const |
Definition at line 93 of file incidencebase.cpp.
Person IncidenceBase::organizer | ( | ) | const |
Definition at line 180 of file incidencebase.cpp.
unsigned long IncidenceBase::pilotId | ( | ) | const |
void IncidenceBase::registerObserver | ( | IncidenceBase::Observer * | observer | ) |
Register observer.
The observer is notified when the observed object changes.
Definition at line 391 of file incidencebase.cpp.
bool IncidenceBase::removeComment | ( | const QString & | comment | ) |
Remove a comment from the event.
Removes first comment whose string is an exact match for the string passed in.
- Returns:
- true if match found, false otherwise.
Definition at line 236 of file incidencebase.cpp.
void IncidenceBase::setDtStart | ( | const QDateTime & | dtStart | ) | [virtual] |
for setting the event's starting date/time with a QDateTime.
Reimplemented in KCal::Incidence, and KCal::Todo.
Definition at line 190 of file incidencebase.cpp.
void IncidenceBase::setDuration | ( | int | seconds | ) | [virtual] |
void IncidenceBase::setFloats | ( | bool | f | ) |
Set whether the incidence floats, i.e.
has a date but no time attached to it.
Reimplemented in KCal::Incidence.
Definition at line 223 of file incidencebase.cpp.
void IncidenceBase::setHasDuration | ( | bool | hasDuration | ) |
Definition at line 350 of file incidencebase.cpp.
void IncidenceBase::setLastModified | ( | const QDateTime & | lm | ) |
void IncidenceBase::setOrganizer | ( | const QString & | o | ) |
Definition at line 170 of file incidencebase.cpp.
void IncidenceBase::setOrganizer | ( | const Person & | o | ) |
void IncidenceBase::setPilotId | ( | unsigned long | id | ) |
void IncidenceBase::setReadOnly | ( | bool | readOnly | ) | [virtual] |
Set readonly status.
Reimplemented in KCal::Incidence.
Definition at line 185 of file incidencebase.cpp.
void IncidenceBase::setSyncStatus | ( | int | status | ) |
void IncidenceBase::setSyncStatusSilent | ( | int | status | ) |
Definition at line 367 of file incidencebase.cpp.
void IncidenceBase::setUid | ( | const QString & | uid | ) |
int IncidenceBase::syncStatus | ( | ) | const |
virtual QCString KCal::IncidenceBase::type | ( | ) | const [pure virtual] |
Implemented in KCal::Event, KCal::FreeBusy, KCal::Journal, and KCal::Todo.
QString IncidenceBase::uid | ( | ) | const |
void IncidenceBase::unRegisterObserver | ( | IncidenceBase::Observer * | observer | ) |
Unregister observer.
It isn't notified anymore about changes.
Definition at line 396 of file incidencebase.cpp.
void IncidenceBase::updated | ( | ) |
Call this to notify the observers after the IncidenceBas object has changed.
Definition at line 401 of file incidencebase.cpp.
void IncidenceBase::updatedSilent | ( | ) |
Definition at line 416 of file incidencebase.cpp.
Member Data Documentation
bool KCal::IncidenceBase::mReadOnly [protected] |
Definition at line 265 of file incidencebase.h.
The documentation for this class was generated from the following files: