libkcal
icalrecur.h File Reference
Routines for dealing with recurring time. More...
#include <time.h>
#include "icaltime.h"
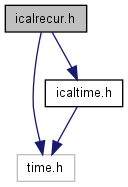
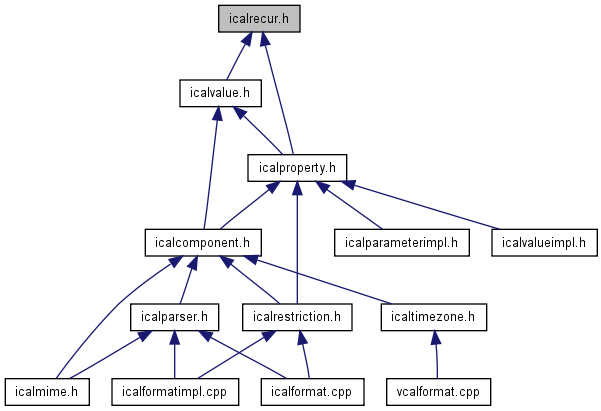
Go to the source code of this file.
Detailed Description
Routines for dealing with recurring time.How to use:
1) Get a rule and a start time from a component
icalproperty rrule; struct icalrecurrencetype recur; struct icaltimetype dtstart; rrule = icalcomponent_get_first_property(comp,ICAL_RRULE_PROPERTY); recur = icalproperty_get_rrule(rrule); start = icalproperty_get_dtstart(dtstart);
Or, just make them up:
recur = icalrecurrencetype_from_string("FREQ=YEARLY;BYDAY=SU,WE"); dtstart = icaltime_from_string("19970101T123000")
2) Create an iterator
icalrecur_iterator* ritr; ritr = icalrecur_iterator_new(recur,start);
3) Iterator over the occurrences
struct icaltimetype next; while (next = icalrecur_iterator_next(ritr) && !icaltime_is_null_time(next){ Do something with next }
Note that that the time returned by icalrecur_iterator_next is in whatever timezone that dtstart is in.
Definition in file icalrecur.h.
Define Documentation
#define ICAL_BY_DAY_SIZE 364 |
Definition at line 124 of file icalrecur.h.
#define ICAL_BY_HOUR_SIZE 25 |
Definition at line 123 of file icalrecur.h.
#define ICAL_BY_MINUTE_SIZE 61 |
Definition at line 122 of file icalrecur.h.
#define ICAL_BY_MONTH_SIZE 13 |
Definition at line 128 of file icalrecur.h.
#define ICAL_BY_MONTHDAY_SIZE 32 |
Definition at line 125 of file icalrecur.h.
#define ICAL_BY_SECOND_SIZE 61 |
#define ICAL_BY_SETPOS_SIZE 367 |
Definition at line 129 of file icalrecur.h.
#define ICAL_BY_WEEKNO_SIZE 54 |
Definition at line 127 of file icalrecur.h.
#define ICAL_BY_YEARDAY_SIZE 367 |
Definition at line 126 of file icalrecur.h.
Typedef Documentation
typedef struct icalrecur_iterator_impl icalrecur_iterator |
Enumeration Type Documentation
anonymous enum |
Definition at line 107 of file icalrecur.h.
- Enumerator:
-
ICAL_SECONDLY_RECURRENCE ICAL_MINUTELY_RECURRENCE ICAL_HOURLY_RECURRENCE ICAL_DAILY_RECURRENCE ICAL_WEEKLY_RECURRENCE ICAL_MONTHLY_RECURRENCE ICAL_YEARLY_RECURRENCE ICAL_NO_RECURRENCE
Definition at line 79 of file icalrecur.h.
- Enumerator:
-
ICAL_NO_WEEKDAY ICAL_SUNDAY_WEEKDAY ICAL_MONDAY_WEEKDAY ICAL_TUESDAY_WEEKDAY ICAL_WEDNESDAY_WEEKDAY ICAL_THURSDAY_WEEKDAY ICAL_FRIDAY_WEEKDAY ICAL_SATURDAY_WEEKDAY
Definition at line 95 of file icalrecur.h.
Function Documentation
int icalrecur_expand_recurrence | ( | char * | rule, | |
time_t | start, | |||
int | count, | |||
time_t * | array | |||
) |
Fills array up with at most 'count' time_t values, each representing an occurrence time in seconds past the POSIX epoch.
void icalrecur_iterator_decrement_count | ( | icalrecur_iterator * | ) |
void icalrecur_iterator_free | ( | icalrecur_iterator * | ) |
Free the iterator.
icalrecur_iterator* icalrecur_iterator_new | ( | struct icalrecurrencetype | rule, | |
struct icaltimetype | dtstart | |||
) |
Create a new recurrence rule iterator.
struct icaltimetype icalrecur_iterator_next | ( | icalrecur_iterator * | ) | [read] |
Get the next occurrence from an iterator.
char* icalrecurrencetype_as_string | ( | struct icalrecurrencetype * | recur | ) |
void icalrecurrencetype_clear | ( | struct icalrecurrencetype * | r | ) |
enum icalrecurrencetype_weekday icalrecurrencetype_day_day_of_week | ( | short | day | ) |
Array Encoding.
The 'day' element of the by_day array is encoded to allow representation of both the day of the week ( Monday, Tueday), but also the Nth day of the week ( First tuesday of the month, last thursday of the year) These routines decode the day values 1 == Monday, etc.
int icalrecurrencetype_day_position | ( | short | day | ) |
0 == any of day of week.
1 == first, 2 = second, -2 == second to last, etc
struct icalrecurrencetype icalrecurrencetype_from_string | ( | const char * | str | ) | [read] |
Recurrance rule parser.
Convert between strings and recurrencetype structures.