Kate
#include <expandingwidgetmodel.h>
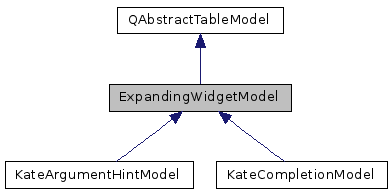
Public Types | |
enum | ExpandingType { NotExpandable =0, Expandable, Expanded } |
enum | ExpansionType { NotExpanded = 0, ExpandDownwards, ExpandUpwards } |
Public Slots | |
void | placeExpandingWidgets () |
Public Member Functions | |
ExpandingWidgetModel (QWidget *parent) | |
virtual | ~ExpandingWidgetModel () |
bool | canCollapseCurrentItem () const |
bool | canExpandCurrentItem () const |
void | clearExpanding () |
void | clearMatchQualities () |
virtual QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const |
QWidget * | expandingWidget (const QModelIndex &row) const |
int | expandingWidgetsHeight () const |
virtual bool | indexIsItem (const QModelIndex &index) const =0 |
bool | isExpandable (const QModelIndex &index) const |
bool | isExpanded (const QModelIndex &row) const |
ExpansionType | isPartiallyExpanded (const QModelIndex &index) const |
uint | matchColor (const QModelIndex &index) const |
QRect | partialExpandRect (const QModelIndex &row) const |
QString | partialExpandText (const QModelIndex &row) const |
QModelIndex | partiallyExpandedRow () const |
int | partiallyExpandWidgetHeight () const |
void | placeExpandingWidget (const QModelIndex &row) |
virtual void | rowSelected (const QModelIndex &row) |
void | setCurrentItemExpanded (bool) |
void | setExpanded (QModelIndex index, bool expanded) |
virtual QTreeView * | treeView () const =0 |
Protected Member Functions | |
int | basicRowHeight (const QModelIndex &index) const |
void | cacheIcons () const |
virtual int | contextMatchQuality (const QModelIndex &index) const =0 |
void | partiallyUnExpand (const QModelIndex &index) |
Static Protected Attributes | |
static QIcon | m_collapsedIcon |
static QIcon | m_expandedIcon |
Detailed Description
Cares about expanding/un-expanding items in a tree-view together with ExpandingDelegate.
Definition at line 36 of file expandingwidgetmodel.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NotExpandable | |
Expandable | |
Expanded |
Definition at line 43 of file expandingwidgetmodel.h.
Enumerator | |
---|---|
NotExpanded | |
ExpandDownwards | |
ExpandUpwards |
Definition at line 65 of file expandingwidgetmodel.h.
Constructor & Destructor Documentation
ExpandingWidgetModel::ExpandingWidgetModel | ( | QWidget * | parent | ) |
Definition at line 44 of file expandingwidgetmodel.cpp.
|
virtual |
Definition at line 49 of file expandingwidgetmodel.cpp.
Member Function Documentation
|
protected |
Definition at line 357 of file expandingwidgetmodel.cpp.
|
protected |
Definition at line 441 of file expandingwidgetmodel.cpp.
bool ExpandingWidgetModel::canCollapseCurrentItem | ( | ) | const |
- Returns
- whether the current item can be collapsed
bool ExpandingWidgetModel::canExpandCurrentItem | ( | ) | const |
The following three are convenience-functions for the current item that could be replaced by the later ones.
- Returns
- whether the current item can be expanded
void ExpandingWidgetModel::clearExpanding | ( | ) |
Unexpand all rows and clear all cached information about them(this includes deleting the expanding-widgets)
Definition at line 123 of file expandingwidgetmodel.cpp.
void ExpandingWidgetModel::clearMatchQualities | ( | ) |
Definition at line 112 of file expandingwidgetmodel.cpp.
|
protectedpure virtual |
- Returns
- the context-match quality from 0 to 10 if it could be determined, else -1
Implemented in KateCompletionModel, and KateArgumentHintModel.
|
virtual |
Does not request data from index, this only returns local data like highlighting for expanded rows and similar.
Reimplemented in KateCompletionModel, and KateArgumentHintModel.
Definition at line 88 of file expandingwidgetmodel.cpp.
QWidget * ExpandingWidgetModel::expandingWidget | ( | const QModelIndex & | row | ) | const |
- Returns
- the expanding-widget for the given row, if available. Expanding-widgets are in best case available for all expanded rows. This does not return the partially-expand widget.
Definition at line 431 of file expandingwidgetmodel.cpp.
int ExpandingWidgetModel::expandingWidgetsHeight | ( | ) | const |
Returns the total height added through all open expanding-widgets.
Definition at line 420 of file expandingwidgetmodel.cpp.
|
pure virtual |
Should return true if the given row should be painted like a contained item(as opposed to label-rows etc.)
Implemented in KateCompletionModel, and KateArgumentHintModel.
bool ExpandingWidgetModel::isExpandable | ( | const QModelIndex & | index | ) | const |
- Returns
- whether the row given through index is expandable
Definition at line 290 of file expandingwidgetmodel.cpp.
bool ExpandingWidgetModel::isExpanded | ( | const QModelIndex & | row | ) | const |
- Returns
- whether row is currently expanded
Definition at line 305 of file expandingwidgetmodel.cpp.
ExpandingWidgetModel::ExpansionType ExpandingWidgetModel::isPartiallyExpanded | ( | const QModelIndex & | index | ) | const |
Returns whether the given index is currently partially expanded. Does not do any other checks like calling models for data.
Definition at line 139 of file expandingwidgetmodel.cpp.
uint ExpandingWidgetModel::matchColor | ( | const QModelIndex & | index | ) | const |
Returns the match-color for the given index, or zero if match-quality could not be computed.
Definition at line 58 of file expandingwidgetmodel.cpp.
QRect ExpandingWidgetModel::partialExpandRect | ( | const QModelIndex & | row | ) | const |
Returns the rectangle for the partially expanded part of the given row.
Definition at line 251 of file expandingwidgetmodel.cpp.
QString ExpandingWidgetModel::partialExpandText | ( | const QModelIndex & | row | ) | const |
Definition at line 244 of file expandingwidgetmodel.cpp.
QModelIndex ExpandingWidgetModel::partiallyExpandedRow | ( | ) | const |
Returns the first row that is currently partially expanded.
Definition at line 116 of file expandingwidgetmodel.cpp.
int ExpandingWidgetModel::partiallyExpandWidgetHeight | ( | ) | const |
Amount by which the height of a row increases when it is partially expanded.
- Todo:
- use font-metrics text-height*2 for 2 lines
Definition at line 153 of file expandingwidgetmodel.cpp.
|
protected |
Definition at line 146 of file expandingwidgetmodel.cpp.
void ExpandingWidgetModel::placeExpandingWidget | ( | const QModelIndex & | row | ) |
Places and shows the expanding-widget for the given row, if it should be visible and is valid.
Also shows the partial-expanding-widget when it should be visible.
Definition at line 370 of file expandingwidgetmodel.cpp.
|
slot |
Place or hides all expanding-widgets to the correct positions. Should be called after the view was scrolled.
Definition at line 414 of file expandingwidgetmodel.cpp.
|
virtual |
Notifies underlying models that the item was selected, collapses any previous partially expanded line, checks whether this line should be partially expanded, and eventually does it.
Does nothing when nothing needs to be done. Does NOT show the expanding-widget. That is done immediately when painting by ExpandingDelegate, to reduce flickering.
- See also
- showPartialExpandWidget()
- Parameters
-
row The row
- Todo:
- allow multiple partially expanded rows
Reimplemented in KateCompletionModel.
Definition at line 157 of file expandingwidgetmodel.cpp.
void ExpandingWidgetModel::setCurrentItemExpanded | ( | bool | ) |
Expand/collapse the current item.
void ExpandingWidgetModel::setExpanded | ( | QModelIndex | index, |
bool | expanded | ||
) |
Change the expand-state of the row given through index. The display will be updated.
Definition at line 311 of file expandingwidgetmodel.cpp.
|
pure virtual |
Implemented in KateArgumentHintModel.
Member Data Documentation
|
staticprotected |
Definition at line 136 of file expandingwidgetmodel.h.
|
staticprotected |
Definition at line 135 of file expandingwidgetmodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.