Kate
#include <katecompletionmodel.h>
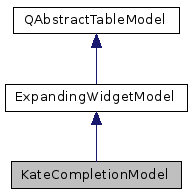
Classes | |
class | Group |
Public Types | |
enum | { BestMatchesProperty = 2*KTextEditor::CodeCompletionModel::LastProperty } |
enum | gm { ScopeType = 0x1, Scope = 0x2, AccessType = 0x4, ItemType = 0x8 } |
![]() | |
enum | ExpandingType { NotExpandable =0, Expandable, Expanded } |
enum | ExpansionType { NotExpanded = 0, ExpandDownwards, ExpandUpwards } |
Public Slots | |
void | setColumnMergingEnabled (bool enable) |
void | setFilteringEnabled (bool enable) |
void | setGroupingEnabled (bool enable) |
void | setSortingEnabled (bool enable) |
![]() | |
void | placeExpandingWidgets () |
Signals | |
void | argumentHintsChanged () |
void | contentGeometryChanged () |
void | expandIndex (const QModelIndex &index) |
Public Member Functions | |
KateCompletionModel (KateCompletionWidget *parent=0L) | |
~KateCompletionModel () | |
bool | accessIncludeConst () const |
bool | accessIncludeSignalSlot () const |
bool | accessIncludeStatic () const |
void | addCompletionModel (KTextEditor::CodeCompletionModel *model) |
void | clearCompletionModels () |
virtual int | columnCount (const QModelIndex &parent=QModelIndex()) const |
const QList< QList< int > > & | columnMerges () const |
QString | commonPrefix (QModelIndex selectedIndex) const |
QList < KTextEditor::CodeCompletionModel * > | completionModels () const |
QString | currentCompletion (KTextEditor::CodeCompletionModel *model) const |
virtual QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const |
void | debugStats () |
KTextEditor::CodeCompletionModel::CompletionProperties | filterAttributes () const |
bool | filterByAttribute () const |
bool | filterContextMatchesOnly () const |
uint | filteredItemCount () const |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const |
GroupingMethods | groupingMethod () const |
virtual bool | hasChildren (const QModelIndex &parent=QModelIndex()) const |
bool | hasGroups () const |
virtual bool | hasIndex (int row, int column, const QModelIndex &parent=QModelIndex()) const |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
virtual bool | indexIsItem (const QModelIndex &index) const |
bool | isColumnMergingEnabled () const |
bool | isFilteringEnabled () const |
bool | isGroupingEnabled () const |
bool | isSortingAlphabetical () const |
bool | isSortingByInheritanceDepth () const |
bool | isSortingEnabled () const |
bool | isSortingReverse () const |
virtual QModelIndex | mapFromSource (const QModelIndex &sourceIndex) const |
virtual QModelIndex | mapToSource (const QModelIndex &proxyIndex) const |
Qt::CaseSensitivity | matchCaseSensitivity () const |
int | maximumInheritanceDepth () const |
virtual QModelIndex | parent (const QModelIndex &index) const |
void | removeCompletionModel (KTextEditor::CodeCompletionModel *model) |
virtual int | rowCount (const QModelIndex &parent=QModelIndex()) const |
virtual void | rowSelected (const QModelIndex &row) |
void | setAccessIncludeConst (bool include) |
void | setAccessIncludeSignalSlot (bool include) |
void | setAccessIncludeStatic (bool include) |
void | setColumnMerges (const QList< QList< int > > &columnMerges) |
void | setCompletionModel (KTextEditor::CodeCompletionModel *model) |
void | setCompletionModels (const QList< KTextEditor::CodeCompletionModel * > &models) |
void | setCurrentCompletion (KTextEditor::CodeCompletionModel *model, const QString &completion) |
void | setFilterAttributes (KTextEditor::CodeCompletionModel::CompletionProperties attributes) |
void | setFilterByAttribute (bool filter) |
void | setFilterContextMatchesOnly (bool filter) |
void | setGroupingMethod (GroupingMethods m) |
void | setMatchCaseSensitivity (Qt::CaseSensitivity cs) |
void | setMaximumInheritanceDepth (int maxDepth) |
void | setSortingAlphabetical (bool alphabetical) |
void | setSortingByInheritanceDepth (bool byIneritance) |
void | setSortingCaseSensitivity (Qt::CaseSensitivity cs) |
void | setSortingReverse (bool reverse) |
bool | shouldMatchHideCompletionList () const |
virtual void | sort (int column, Qt::SortOrder order=Qt::AscendingOrder) |
Qt::CaseSensitivity | sortingCaseSensitivity () const |
int | translateColumn (int sourceColumn) const |
KateView * | view () const |
KateCompletionWidget * | widget () const |
![]() | |
ExpandingWidgetModel (QWidget *parent) | |
virtual | ~ExpandingWidgetModel () |
bool | canCollapseCurrentItem () const |
bool | canExpandCurrentItem () const |
void | clearExpanding () |
void | clearMatchQualities () |
QWidget * | expandingWidget (const QModelIndex &row) const |
int | expandingWidgetsHeight () const |
bool | isExpandable (const QModelIndex &index) const |
bool | isExpanded (const QModelIndex &row) const |
ExpansionType | isPartiallyExpanded (const QModelIndex &index) const |
uint | matchColor (const QModelIndex &index) const |
QRect | partialExpandRect (const QModelIndex &row) const |
QString | partialExpandText (const QModelIndex &row) const |
QModelIndex | partiallyExpandedRow () const |
int | partiallyExpandWidgetHeight () const |
void | placeExpandingWidget (const QModelIndex &row) |
void | setCurrentItemExpanded (bool) |
void | setExpanded (QModelIndex index, bool expanded) |
Static Public Member Functions | |
static QString | columnName (int column) |
static QString | propertyName (KTextEditor::CodeCompletionModel::CompletionProperty property) |
Static Public Attributes | |
static const int | AccessTypeMask = 0x7 |
static const int | ItemTypeMask = 0xfe0 |
static const int | ScopeTypeMask = 0x380000 |
Protected Member Functions | |
virtual int | contextMatchQuality (const QModelIndex &index) const |
![]() | |
int | basicRowHeight (const QModelIndex &index) const |
void | cacheIcons () const |
void | partiallyUnExpand (const QModelIndex &index) |
Additional Inherited Members | |
![]() | |
static QIcon | m_collapsedIcon |
static QIcon | m_expandedIcon |
Detailed Description
This class has the responsibility for filtering, sorting, and manipulating code completion data provided by a CodeCompletionModel.
Definition at line 48 of file katecompletionmodel.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
BestMatchesProperty |
Definition at line 146 of file katecompletionmodel.h.
Enumerator | |
---|---|
ScopeType | |
Scope | |
AccessType | |
ItemType |
Definition at line 139 of file katecompletionmodel.h.
Constructor & Destructor Documentation
KateCompletionModel::KateCompletionModel | ( | KateCompletionWidget * | parent = 0L | ) |
Definition at line 128 of file katecompletionmodel.cpp.
KateCompletionModel::~KateCompletionModel | ( | ) |
Definition at line 172 of file katecompletionmodel.cpp.
Member Function Documentation
bool KateCompletionModel::accessIncludeConst | ( | ) | const |
Definition at line 1360 of file katecompletionmodel.cpp.
bool KateCompletionModel::accessIncludeSignalSlot | ( | ) | const |
Definition at line 1390 of file katecompletionmodel.cpp.
bool KateCompletionModel::accessIncludeStatic | ( | ) | const |
Definition at line 1375 of file katecompletionmodel.cpp.
void KateCompletionModel::addCompletionModel | ( | KTextEditor::CodeCompletionModel * | model | ) |
Definition at line 2001 of file katecompletionmodel.cpp.
|
signal |
void KateCompletionModel::clearCompletionModels | ( | ) |
Definition at line 2253 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 373 of file katecompletionmodel.cpp.
Definition at line 1252 of file katecompletionmodel.cpp.
|
static |
Definition at line 1232 of file katecompletionmodel.cpp.
QString KateCompletionModel::commonPrefix | ( | QModelIndex | selectedIndex | ) | const |
Returns a common prefix for all current visible completion entries If there is no common prefix, extracts the next useful prefix for the selected index.
Definition at line 1006 of file katecompletionmodel.cpp.
QList< KTextEditor::CodeCompletionModel * > KateCompletionModel::completionModels | ( | ) | const |
Definition at line 2041 of file katecompletionmodel.cpp.
|
signal |
|
protectedvirtual |
- Returns
- the context-match quality from 0 to 10 if it could be determined, else -1
Implements ExpandingWidgetModel.
Definition at line 299 of file katecompletionmodel.cpp.
QString KateCompletionModel::currentCompletion | ( | KTextEditor::CodeCompletionModel * | model | ) | const |
Definition at line 1991 of file katecompletionmodel.cpp.
|
virtual |
Does not request data from index, this only returns local data like highlighting for expanded rows and similar.
Reimplemented from ExpandingWidgetModel.
Definition at line 183 of file katecompletionmodel.cpp.
void KateCompletionModel::debugStats | ( | ) |
Definition at line 1170 of file katecompletionmodel.cpp.
|
signal |
KTextEditor::CodeCompletionModel::CompletionProperties KateCompletionModel::filterAttributes | ( | ) | const |
Definition at line 1629 of file katecompletionmodel.cpp.
bool KateCompletionModel::filterByAttribute | ( | ) | const |
Definition at line 1616 of file katecompletionmodel.cpp.
bool KateCompletionModel::filterContextMatchesOnly | ( | ) | const |
Definition at line 1603 of file katecompletionmodel.cpp.
uint KateCompletionModel::filteredItemCount | ( | ) | const |
Definition at line 1712 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 348 of file katecompletionmodel.cpp.
KateCompletionModel::GroupingMethods KateCompletionModel::groupingMethod | ( | ) | const |
Definition at line 1415 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 383 of file katecompletionmodel.cpp.
bool KateCompletionModel::hasGroups | ( | ) | const |
Definition at line 783 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 463 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 407 of file katecompletionmodel.cpp.
|
virtual |
Should return true if the given row should be painted like a contained item(as opposed to label-rows etc.)
Implements ExpandingWidgetModel.
Definition at line 1152 of file katecompletionmodel.cpp.
bool KateCompletionModel::isColumnMergingEnabled | ( | ) | const |
Definition at line 1212 of file katecompletionmodel.cpp.
bool KateCompletionModel::isFilteringEnabled | ( | ) | const |
Definition at line 1222 of file katecompletionmodel.cpp.
bool KateCompletionModel::isGroupingEnabled | ( | ) | const |
Definition at line 1217 of file katecompletionmodel.cpp.
bool KateCompletionModel::isSortingAlphabetical | ( | ) | const |
Definition at line 1427 of file katecompletionmodel.cpp.
bool KateCompletionModel::isSortingByInheritanceDepth | ( | ) | const |
Definition at line 1420 of file katecompletionmodel.cpp.
bool KateCompletionModel::isSortingEnabled | ( | ) | const |
Definition at line 1227 of file katecompletionmodel.cpp.
bool KateCompletionModel::isSortingReverse | ( | ) | const |
|
virtual |
Maps from an index in a source-model to the index of the item in this display-model.
Definition at line 892 of file katecompletionmodel.cpp.
|
virtual |
Maps from this display-model into the appropriate source code-completion model.
Definition at line 875 of file katecompletionmodel.cpp.
Qt::CaseSensitivity KateCompletionModel::matchCaseSensitivity | ( | ) | const |
Definition at line 1996 of file katecompletionmodel.cpp.
int KateCompletionModel::maximumInheritanceDepth | ( | ) | const |
Definition at line 1642 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 823 of file katecompletionmodel.cpp.
|
static |
Definition at line 1897 of file katecompletionmodel.cpp.
void KateCompletionModel::removeCompletionModel | ( | KTextEditor::CodeCompletionModel * | model | ) |
Definition at line 2046 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 847 of file katecompletionmodel.cpp.
|
virtual |
Notifies underlying models that the item was selected, collapses any previous partially expanded line, checks whether this line should be partially expanded, and eventually does it.
Does nothing when nothing needs to be done. Does NOT show the expanding-widget. That is done immediately when painting by ExpandingDelegate, to reduce flickering.
- See also
- showPartialExpandWidget()
- Parameters
-
row The row
- Todo:
- delay this
- Todo:
- allow multiple partially expanded rows
Reimplemented from ExpandingWidgetModel.
Definition at line 2240 of file katecompletionmodel.cpp.
void KateCompletionModel::setAccessIncludeConst | ( | bool | include | ) |
Definition at line 1365 of file katecompletionmodel.cpp.
void KateCompletionModel::setAccessIncludeSignalSlot | ( | bool | include | ) |
Definition at line 1395 of file katecompletionmodel.cpp.
void KateCompletionModel::setAccessIncludeStatic | ( | bool | include | ) |
Definition at line 1380 of file katecompletionmodel.cpp.
Definition at line 1257 of file katecompletionmodel.cpp.
|
slot |
Definition at line 1206 of file katecompletionmodel.cpp.
void KateCompletionModel::setCompletionModel | ( | KTextEditor::CodeCompletionModel * | model | ) |
Definition at line 2016 of file katecompletionmodel.cpp.
void KateCompletionModel::setCompletionModels | ( | const QList< KTextEditor::CodeCompletionModel * > & | models | ) |
Definition at line 2022 of file katecompletionmodel.cpp.
void KateCompletionModel::setCurrentCompletion | ( | KTextEditor::CodeCompletionModel * | model, |
const QString & | completion | ||
) |
Definition at line 916 of file katecompletionmodel.cpp.
void KateCompletionModel::setFilterAttributes | ( | KTextEditor::CodeCompletionModel::CompletionProperties | attributes | ) |
Definition at line 1634 of file katecompletionmodel.cpp.
void KateCompletionModel::setFilterByAttribute | ( | bool | filter | ) |
Definition at line 1621 of file katecompletionmodel.cpp.
void KateCompletionModel::setFilterContextMatchesOnly | ( | bool | filter | ) |
Definition at line 1608 of file katecompletionmodel.cpp.
|
slot |
Definition at line 1186 of file katecompletionmodel.cpp.
|
slot |
Definition at line 1200 of file katecompletionmodel.cpp.
void KateCompletionModel::setGroupingMethod | ( | GroupingMethods | m | ) |
Definition at line 1353 of file katecompletionmodel.cpp.
void KateCompletionModel::setMatchCaseSensitivity | ( | Qt::CaseSensitivity | cs | ) |
Definition at line 368 of file katecompletionmodel.cpp.
void KateCompletionModel::setMaximumInheritanceDepth | ( | int | maxDepth | ) |
Definition at line 1647 of file katecompletionmodel.cpp.
void KateCompletionModel::setSortingAlphabetical | ( | bool | alphabetical | ) |
Definition at line 1556 of file katecompletionmodel.cpp.
void KateCompletionModel::setSortingByInheritanceDepth | ( | bool | byIneritance | ) |
Definition at line 1423 of file katecompletionmodel.cpp.
void KateCompletionModel::setSortingCaseSensitivity | ( | Qt::CaseSensitivity | cs | ) |
Definition at line 1570 of file katecompletionmodel.cpp.
|
slot |
Definition at line 1192 of file katecompletionmodel.cpp.
void KateCompletionModel::setSortingReverse | ( | bool | reverse | ) |
bool KateCompletionModel::shouldMatchHideCompletionList | ( | ) | const |
Returns whether one of the filtered items exactly matches its completion string.
Definition at line 1721 of file katecompletionmodel.cpp.
|
virtual |
Definition at line 869 of file katecompletionmodel.cpp.
Qt::CaseSensitivity KateCompletionModel::sortingCaseSensitivity | ( | ) | const |
Definition at line 1432 of file katecompletionmodel.cpp.
int KateCompletionModel::translateColumn | ( | int | sourceColumn | ) | const |
Definition at line 1263 of file katecompletionmodel.cpp.
KateView * KateCompletionModel::view | ( | ) | const |
Definition at line 363 of file katecompletionmodel.cpp.
KateCompletionWidget * KateCompletionModel::widget | ( | ) | const |
Definition at line 359 of file katecompletionmodel.cpp.
Member Data Documentation
|
static |
Definition at line 153 of file katecompletionmodel.h.
|
static |
Definition at line 154 of file katecompletionmodel.h.
|
static |
Definition at line 152 of file katecompletionmodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.