KTextEditor
#include <codecompletionmodel.h>
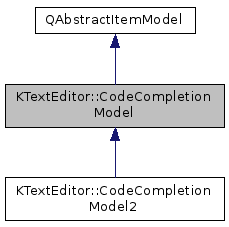
Signals | |
void | hasGroupsChanged (KTextEditor::CodeCompletionModel *model, bool hasGroups) |
void | waitForReset () |
Public Member Functions | |
CodeCompletionModel (QObject *parent) | |
virtual | ~CodeCompletionModel () |
virtual int | columnCount (const QModelIndex &parent=QModelIndex()) const |
virtual void | completionInvoked (KTextEditor::View *view, const KTextEditor::Range &range, InvocationType invocationType) |
virtual void | executeCompletionItem (Document *document, const Range &word, int row) const |
bool | hasGroups () const |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const |
virtual QModelIndex | parent (const QModelIndex &index) const |
virtual int | rowCount (const QModelIndex &parent=QModelIndex()) const |
void | setRowCount (int rowCount) |
Static Public Attributes | |
static const int | ColumnCount = Postfix + 1 |
static const int | LastItemDataRole = AccessibilityAccept |
Protected Member Functions | |
void | setHasGroups (bool hasGroups) |
Detailed Description
An item model for providing code completion, and meta information for enhanced presentation.
Introduction
The CodeCompletionModel is the actual workhorse to provide code completions in a KTextEditor::View. It is meant to be used in conjunction with the CodeCompletionInterface. The CodeCompletionModel is not meant to be used as is. Rather you need to implement a subclass of CodeCompletionModel to actually generate completions appropriate for your type of Document.
Implementing a CodeCompletionModel
The CodeCompletionModel is a QAbstractItemModel, and can be subclassed in the same way. It provides default implementations of several members, however, so in most cases (if your completions are essentially a non-hierarchical, flat list of matches) you will only need to overload few virtual functions.
Implementing a CodeCompletionModel for a flat list
For the simple case of a flat list of completions, you will need to:
- Implement completionInvoked() to actually generate/update the list of completion matches
- implement itemData() (or QAbstractItemModel::data()) to return the information that should be displayed for each match.
- use setRowCount() to reflect the number of matches.
Columns and roles
- Todo:
- document the meaning and usage of the columns and roles used by the CodeCompletionInterface
Using the new CodeCompletionModel
To start using your CodeCompletionModel, refer to CodeCompletionInterface.
ControllerInterface to get more control
To have more control over code completion implement CodeCompletionModelControllerInterface in your CodeCompletionModel.
Definition at line 77 of file codecompletionmodel.h.
Member Enumeration Documentation
Definition at line 85 of file codecompletionmodel.h.
Definition at line 98 of file codecompletionmodel.h.
Meta information is passed through extra {Qt::ItemDataRole}s.
This information should be returned when requested on the Name column.
Enumerator | |
---|---|
CompletionRole |
The model should return a set of CompletionProperties. |
ScopeIndex |
The model should return an index to the scope -1 represents no scope.
|
MatchQuality |
If requested, your model should try to determine whether the completion in question is a suitable match for the context (ie. is accessible, exported, + returns the data type required). The returned data should ideally be matched against the argument-hint context set earlier by SetMatchContext. Return an integer value that should be positive if the completion is suitable, and zero if the completion is not suitable. The value should be between 0 an 10, where 10 means perfect match. Return QVariant::Invalid if you are unable to determine this. |
SetMatchContext |
Is requested before MatchQuality(..) is requested. The item on which this is requested is an argument-hint item(
Feel free to ignore this, but ideally you should return QVariant::Invalid to make clear that your model does not support this. |
HighlightingMethod |
Define which highlighting method will be used:
|
CustomHighlight |
Allows an item to provide custom highlighting. Return a QList<QVariant> in the following format:
Repeat this triplet as many times as required, however each column must be >= the previous, and startColumn != endColumn. |
InheritanceDepth |
Returns the inheritance depth of the completion. For example, a completion which comes from the base class would have depth 0, one from a parent class would have depth 1, one from that class' parent 2, etc. you can use this to symbolize the general distance of a completion-item from a user. It will be used for sorting. |
IsExpandable |
This allows items in the completion-list to be expandable. If a model returns an QVariant bool value that evaluates to true, the completion-widget will draw a handle to expand the item, and will also make that action accessible through keyboard. |
ExpandingWidget |
After a model returned true for a row on IsExpandable, the row may be expanded by the user. When this happens, ExpandingWidget is requested. The model may return two types of values: QWidget*: If the model returns a QVariant of type QWidget*, the code-completion takes over the given widget and embeds it into the completion-list under the completion-item. The widget will be automatically deleted at some point. The completion-widget will use the height of the widget as a hint for its preferred size, but it will resize the widget at will. QString: If the mode returns a QVariant of type QString, it will create a small html-widget showing the given html-code, and embed it into the completion-list under the completion-item. Warning: QWidget* widget; return QVariant(widget); Will not work correctly! Use the following instead.: QVariant v; v.setValue<QWidget*>(widget); return v; |
ItemSelected |
Whenever an item is selected, this will be requested from the underlying model. It may be used as a simple notification that the item was selected. Above that, the model may return a QString, which then should then contain html-code. A html-widget will then be displayed as a one- or two-liner under the currently selected item(it will be partially expanded) |
ArgumentHintDepth |
Is this completion-item an argument-hint? The model should return an integral positive number if the item is an argument-hint, and QVariant() or 0 if it is not one. The returned depth-integer is important for sorting and matching. Example: "otherFunction(function1(function2(" all functions named function2 should have ArgumentHintDepth 1, all functions found for function1 should have ArgumentHintDepth 2, and all functions named otherFunction should have ArgumentHintDepth 3 Later, a completed item may then be matched with the first argument of function2, the return-type of function2 with the first argument-type of function1, and the return-type of function1 with the argument-type of otherFunction. If the model returns a positive value on this role for a row, the content will be treated specially:
Example: You are matching a function with signature "void test(int param1, int param2)", and you are matching the first argument. The model should then return: Prefix: "void test(" Name: "int param1" Suffix: ", int param2)" If you don't use the highlighting, matching, etc. you can also return the columns in the usual way. |
BestMatchesCount |
This will be requested for each item to ask whether it should be included in computing a best-matches list. If you return a valid positive integer n here, the n best matches will be listed at top of the completion-list separately. This is expensive because all items of the whole completion-list will be tested for their matching-quality, with each of the level 1 argument-hints. For that reason the end-user should be able to disable this feature. |
AccessibilityNext |
The following three enumeration-values are only used on expanded completion-list items that contain an expanding-widget(.
You can use them to allow the user to interact with the widget by keyboard. AccessibilityNext will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special navigation short-cut to go to navigate to the next position within the expanding-widget(if applicable). Return QVariant(true) if the input was used. |
AccessibilityPrevious |
AccessibilityPrevious will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special navigation short-cut to go to navigate to the previous position within the expanding-widget(if applicable). Return QVariant(true) if the input was used. |
AccessibilityAccept |
AccessibilityAccept will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special shortcut to trigger the action associated with the position within the expanding-widget the user has navigated to using AccessibilityNext and AccessibilityPrevious. This should return QVariant(true) if an action was triggered, else QVariant(false) or QVariant(). |
GroupRole |
Using this Role, it is possible to greatly optimize the time needed to process very long completion-lists. In the completion-list, the items are usually ordered by some properties like argument-hint depth, inheritance-depth and attributes. Kate usually has to query the completion-models for these values for each item in the completion-list in order to extract the argument-hints and correctly sort the completion-list. However, with a very long completion-list, only a very small fraction of the items is actually visible. By using a tree structure you can give the items in a grouped order to kate, so it does not need to look at each item and query data in order to initially show the completion-list. This is how it works:
The recommended grouping order is: Argument-hint depth, inheritance depth, attributes. This role can also be used to define completely custom groups, bypassing the editors builtin grouping:
|
UnimportantItemRole |
Return a nonzero value here to enforce sorting the item at the end of the list. |
LastExtraItemDataRole |
Definition at line 149 of file codecompletionmodel.h.
Enumerator | |
---|---|
NoHighlighting | |
InternalHighlighting | |
CustomHighlighting |
Definition at line 140 of file codecompletionmodel.h.
Enumerator | |
---|---|
AutomaticInvocation | |
UserInvocation | |
ManualInvocation |
Definition at line 367 of file codecompletionmodel.h.
Constructor & Destructor Documentation
CodeCompletionModel::CodeCompletionModel | ( | QObject * | parent | ) |
Definition at line 36 of file codecompletionmodel.cpp.
|
virtual |
Definition at line 42 of file codecompletionmodel.cpp.
Member Function Documentation
|
virtual |
Reimplemented from QAbstractItemModel::columnCount().
The default implementation returns ColumnCount for all indices.
Definition at line 47 of file codecompletionmodel.cpp.
|
virtual |
This function is responsible to generating / updating the list of current completions.
The default implementation does nothing.
When implementing this function, remember to call setRowCount() (or implement rowCount()), and to generate the appropriate change notifications (for instance by calling QAbstractItemModel::reset()).
- Parameters
-
view The view to generate completions for range The range of text to generate completions for invocationType How the code completion was triggered
Definition at line 91 of file codecompletionmodel.cpp.
|
virtual |
- Deprecated:
- This does not work if your model is hierarchical(
- See also
- GroupRole). Use CodeCompletionModel2::executeCompletionItem2 instead.
This function is responsible for inserting a selected completion into the document. The default implementation replaces the text that the completions were based on with the Qt::DisplayRole of the Name column of the given match.
- Parameters
-
document The document to insert the completion into word The Range that the completions are based on (what the user entered so far) row The row of the completion match to insert
Definition at line 98 of file codecompletionmodel.cpp.
bool CodeCompletionModel::hasGroups | ( | ) | const |
This function returns true if the model needs grouping, otherwise false in KDE 4 default value is true, in KDE 5 the default will be false TODO KDE 5.
Definition at line 103 of file codecompletionmodel.cpp.
|
signal |
Internal.
|
virtual |
Reimplemented from QAbstractItemModel::index().
The default implementation returns a standard QModelIndex as long as the row and column are valid.
Definition at line 52 of file codecompletionmodel.cpp.
Reimplemented from QAbstractItemModel::itemData().
The default implementation returns a map with the QAbstractItemModel::data() for all roles that are used by the CodeCompletionInterface. You will need to reimplement either this function or QAbstractItemModel::data() in your CodeCompletionModel.
Definition at line 60 of file codecompletionmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::parent().
The default implementation returns an invalid QModelIndex for all items. This is appropriate for non-hierarchical / flat lists of completions.
Definition at line 73 of file codecompletionmodel.cpp.
|
virtual |
Reimplemented from QAbstractItemModel::rowCount().
The default implementation returns the value set by setRowCount() for invalid (toplevel) indices, and 0 for all other indices. This is appropriate for non-hierarchical / flat lists of completions
Definition at line 83 of file codecompletionmodel.cpp.
|
protected |
Definition at line 107 of file codecompletionmodel.cpp.
void CodeCompletionModel::setRowCount | ( | int | rowCount | ) |
Definition at line 78 of file codecompletionmodel.cpp.
|
signal |
Emit this if the code-completion for this model was invoked, some time is needed in order to get the data, and the model is reset once the data is available.
This only has an effect if emitted from within completionInvoked(..).
This prevents the code-completion list from showing until this model is reset, so there is no annoying flashing in the user-interface resulting from other models supplying their data earlier.
- Note
- The implementation may choose to show the completion-list anyway after some timeout
- Warning
- If you emit this, you must also reset the model at some point, else the code-completion will be completely broken to the user. Consider that there may always be additional completion-models apart from yours.
- Since
- KDE 4.3
Member Data Documentation
|
static |
Definition at line 96 of file codecompletionmodel.h.
|
static |
Definition at line 363 of file codecompletionmodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.