KTextEditor
#include <range.h>
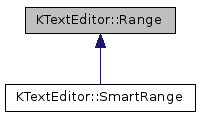
Public Member Functions | |
Range () | |
Range (const Cursor &start, const Cursor &end) | |
Range (const Cursor &start, int width) | |
Range (const Cursor &start, int endLine, int endColumn) | |
Range (int startLine, int startColumn, int endLine, int endColumn) | |
Range (const Range ©) | |
virtual | ~Range () |
Range | encompass (const Range &range) const |
Range | intersect (const Range &range) const |
virtual bool | isSmartRange () const |
virtual bool | isValid () const |
Range & | operator= (const Range &rhs) |
virtual SmartRange * | toSmartRange () const |
Position | |
The following functions provide access to, and manipulation of, the range's position. | |
Cursor & | start () |
const Cursor & | start () const |
Cursor & | end () |
const Cursor & | end () const |
void | setBothLines (int line) |
void | setBothColumns (int column) |
virtual void | setRange (const Range &range) |
void | setRange (const Cursor &start, const Cursor &end) |
virtual bool | expandToRange (const Range &range) |
virtual bool | confineToRange (const Range &range) |
bool | onSingleLine () const |
int | numberOfLines () const |
int | columnWidth () const |
bool | isEmpty () const |
Comparison | |
The following functions perform checks against this range in comparison to other lines, columns, cursors, and ranges. | |
bool | contains (const Range &range) const |
bool | contains (const Cursor &cursor) const |
bool | containsLine (int line) const |
bool | containsColumn (int column) const |
bool | overlaps (const Range &range) const |
bool | overlapsLine (int line) const |
bool | overlapsColumn (int column) const |
int | positionRelativeToCursor (const Cursor &cursor) const |
int | positionRelativeToLine (int line) const |
bool | boundaryAtCursor (const Cursor &cursor) const |
bool | boundaryOnLine (int line) const |
bool | boundaryOnColumn (int column) const |
Static Public Member Functions | |
static Range | invalid () |
Protected Member Functions | |
Range (Cursor *start, Cursor *end) | |
virtual void | rangeChanged (Cursor *cursor, const Range &from) |
Protected Attributes | |
Cursor * | m_end |
Cursor * | m_start |
Friends | |
bool | operator!= (const Range &r1, const Range &r2) |
Range | operator& (const Range &r1, const Range &r2) |
Range & | operator&= (Range &r1, const Range &r2) |
Range | operator+ (const Range &r1, const Range &r2) |
Range & | operator+= (Range &r1, const Range &r2) |
Range | operator- (const Range &r1, const Range &r2) |
Range & | operator-= (Range &r1, const Range &r2) |
bool | operator< (const Range &r1, const Range &r2) |
QDebug | operator<< (QDebug s, const Range &range) |
bool | operator== (const Range &r1, const Range &r2) |
bool | operator> (const Range &r1, const Range &r2) |
Detailed Description
An object representing a section of text, from one Cursor to another.
A Range is a basic class which represents a range of text with two Cursors, from a start() position to an end() position.
For simplicity and convenience, ranges always maintain their start position to be before or equal to their end position. Attempting to set either the start or end of the range beyond the respective end or start will result in both values being set to the specified position. In the constructor, the start and end will be swapped if necessary.
If you want additional functionality such as the ability to maintain position in a document, see SmartRange.
- See also
- SmartRange
Constructor & Destructor Documentation
Range::Range | ( | ) |
Range::Range | ( | const Cursor & | start, |
int | width | ||
) |
Range::Range | ( | const Cursor & | start, |
int | endLine, | ||
int | endColumn | ||
) |
Range::Range | ( | int | startLine, |
int | startColumn, | ||
int | endLine, | ||
int | endColumn | ||
) |
Range::Range | ( | const Range & | copy | ) |
Member Function Documentation
bool KTextEditor::Range::boundaryOnColumn | ( | int | column | ) | const |
bool Range::boundaryOnLine | ( | int | line | ) | const |
int KTextEditor::Range::columnWidth | ( | ) | const |
Confine this range if necessary to fit within range
.
- Parameters
-
range range which should contain this range
- Returns
- true if confinement occurred, false otherwise
Reimplemented in KTextEditor::SmartRange.
bool Range::containsColumn | ( | int | column | ) | const |
bool Range::containsLine | ( | int | line | ) | const |
Cursor & KTextEditor::Range::end | ( | ) |
Get the end position of this range.
This will always be >= start().
This non-const function allows direct manipulation of the end position, while still retaining notification support.
If end is set to a position before start, start will be moved to the same position as end, as ranges are not allowed to have start() > end().
- Note
- If you want to change both start() and end() simultaneously, you should use setRange(), for several reasons:
- Returns
- a reference to the end position of this range.
this function is virtual to allow for covariant return of SmartCursors.
const Cursor & KTextEditor::Range::end | ( | ) | const |
Get the end position of this range.
This will always be >= start().
- Returns
- a const reference to the end position of this range.
this function is virtual to allow for covariant return of SmartCursors.
Expand this range if necessary to contain range
.
- Parameters
-
range range which this range should contain
- Returns
- true if expansion occurred, false otherwise
Reimplemented in KTextEditor::SmartRange.
bool KTextEditor::Range::isEmpty | ( | ) | const |
|
virtual |
Returns whether this range is a SmartRange.
Reimplemented in KTextEditor::SmartRange.
|
virtual |
int KTextEditor::Range::numberOfLines | ( | ) | const |
bool KTextEditor::Range::onSingleLine | ( | ) | const |
Assignment operator.
Same as setRange().
- Parameters
-
rhs range to assign to this range.
- Returns
- a reference to this range, after assignment has occurred.
- See also
- setRange()
bool Range::overlapsColumn | ( | int | column | ) | const |
Check to see if this range overlaps column
; that is, if column
is between start().column() and end().column().
This function is most likely to be useful in relation to block text editing.
- Parameters
-
column the column to test
- Returns
- true if the column is between the range's starting and ending columns, otherwise false.
bool Range::overlapsLine | ( | int | line | ) | const |
int Range::positionRelativeToCursor | ( | const Cursor & | cursor | ) | const |
Determine where cursor
is positioned in relationship to this range.
Equivalency (a return value of 0) is returned when cursor
is contained within the range, not when overlapped - i.e., cursor
may be on a line which is also partially occupied by this range, but the position may not be eqivalent. For overlap checking, use positionRelativeToLine().
- Parameters
-
cursor position to check
- Returns
- -1 if before, +1 if after, and 0 if
cursor
is contained within the range.
- See also
- positionRelativeToLine()
int Range::positionRelativeToLine | ( | int | line | ) | const |
Determine where line
is positioned in relationship to this range.
Equivalency (a return value of 0) is returned when line
is overlapped within the range, not when contained - i.e., this range may not cover an entire line, but line's
position will still be eqivalent. For containment checking, use positionRelativeToCursor().
- Parameters
-
line line to check
- Returns
- -1 if before, +1 if after, and 0 if
line
is overlapped by this range.
- See also
- positionRelativeToCursor()
Notify this range that one or both of the cursors' position has changed directly.
- Parameters
-
cursor the cursor that changed. If 0L, both cursors have changed. from the previous position of this range
Reimplemented in KTextEditor::SmartRange.
void KTextEditor::Range::setBothColumns | ( | int | column | ) |
void Range::setBothLines | ( | int | line | ) |
|
virtual |
Set the start and end cursors to range.start() and range.end() respectively.
- Parameters
-
range range to assign to this range
Reimplemented in KTextEditor::SmartRange.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Set the start and end cursors to start and end respectively.
- Note
- If start is after end, they will be reversed.
- Parameters
-
start start cursor end end cursor
Cursor & KTextEditor::Range::start | ( | ) |
Get the start position of this range.
This will always be <= end().
This non-const function allows direct manipulation of the start position, while still retaining notification support.
If start is set to a position after end, end will be moved to the same position as start, as ranges are not allowed to have start() > end().
- Note
- If you want to change both start() and end() simultaneously, you should use setRange(), for several reasons:
- Returns
- a reference to the start position of this range.
const Cursor & KTextEditor::Range::start | ( | ) | const |
Get the start position of this range.
This will always be <= end().
- Returns
- a const reference to the start position of this range.
this function is virtual to allow for covariant return of SmartCursors.
|
virtual |
Returns this range as a SmartRange, if it is one.
Reimplemented in KTextEditor::SmartRange.
Friends And Related Function Documentation
|
friend |
Member Data Documentation
|
protected |
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.