KTextEditor
#include <cursor.h>
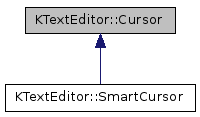
Public Member Functions | |
Cursor () | |
Cursor (int line, int column) | |
Cursor (const Cursor ©) | |
virtual | ~Cursor () |
virtual bool | isSmartCursor () const |
virtual bool | isValid () const |
Cursor & | operator= (const Cursor &cursor) |
Range * | range () const |
virtual SmartCursor * | toSmartCursor () const |
Position | |
The following functions provide access to, and manipulation of, the cursor's position. | |
virtual void | setPosition (const Cursor &position) |
void | setPosition (int line, int column) |
virtual int | line () const |
virtual void | setLine (int line) |
int | column () const |
virtual void | setColumn (int column) |
bool | atStartOfLine () const |
bool | atStartOfDocument () const |
void | position (int &line, int &column) const |
Static Public Member Functions | |
static Cursor | invalid () |
static Cursor | start () |
Protected Member Functions | |
void | cursorChangedDirectly (const Cursor &from) |
virtual void | setRange (Range *range) |
Protected Attributes | |
int | m_column |
int | m_line |
Range * | m_range |
Friends | |
bool | operator!= (const Cursor &c1, const Cursor &c2) |
Cursor | operator+ (const Cursor &c1, const Cursor &c2) |
Cursor & | operator+= (Cursor &c1, const Cursor &c2) |
Cursor | operator- (const Cursor &c1, const Cursor &c2) |
Cursor & | operator-= (Cursor &c1, const Cursor &c2) |
bool | operator< (const Cursor &c1, const Cursor &c2) |
QDebug | operator<< (QDebug s, const Cursor &cursor) |
bool | operator<= (const Cursor &c1, const Cursor &c2) |
bool | operator== (const Cursor &c1, const Cursor &c2) |
bool | operator> (const Cursor &c1, const Cursor &c2) |
bool | operator>= (const Cursor &c1, const Cursor &c2) |
Detailed Description
An object which represents a position in a Document.
A Cursor is a basic class which contains the line() and column() a position in a Document. It is very lightweight and maintains no affiliation with a particular Document.
If you want additional functionality such as the ability to maintain position in a document, see SmartCursor.
- Note
- The Cursor class is designed to be passed via value, while SmartCursor and derivatives must be passed via pointer or reference as they maintain a connection with their document internally and cannot be copied.
- Lines and columns start at 0.
- Think of cursors as having their position at the start of a character, not in the middle of one.
- If a Cursor is associated with a Range the Range will be notified whenever the cursor (i.e. start or end position) changes its position. Read the class documentation about Ranges for further details.
- See also
- SmartCursor
Constructor & Destructor Documentation
Cursor::Cursor | ( | ) |
The default constructor creates a cursor at position (0,0).
Definition at line 26 of file cursor.cpp.
Cursor::Cursor | ( | int | line, |
int | column | ||
) |
This constructor creates a cursor initialized with line
and column
.
- Parameters
-
line line for cursor column column for cursor
Definition at line 33 of file cursor.cpp.
Cursor::Cursor | ( | const Cursor & | copy | ) |
Copy constructor.
Does not copy the owning range, as a range does not have any association with copies of its cursors.
- Parameters
-
copy the cursor to copy.
Definition at line 40 of file cursor.cpp.
|
virtual |
Virtual destructor.
Definition at line 129 of file cursor.cpp.
Member Function Documentation
bool KTextEditor::Cursor::atStartOfDocument | ( | ) | const |
Determine if this cursor is located at the start of a document.
- Returns
- true if the cursor is situated at the start of the document, false if it isn't.
Definition at line 153 of file cursor.cpp.
bool KTextEditor::Cursor::atStartOfLine | ( | ) | const |
Determine if this cursor is located at the start of a line.
- Returns
- true if the cursor is situated at the start of the line, false if it isn't.
Definition at line 148 of file cursor.cpp.
int Cursor::column | ( | ) | const |
Retrieve the column on which this cursor is situated.
- Returns
- column number, where 0 is the first column.
Definition at line 79 of file cursor.cpp.
|
protected |
Notify the owning range, if any, that this cursor has changed directly.
Definition at line 138 of file cursor.cpp.
|
static |
Returns an invalid cursor.
Definition at line 52 of file cursor.cpp.
|
virtual |
Returns whether this cursor is a SmartCursor.
Reimplemented in KTextEditor::SmartCursor.
Definition at line 109 of file cursor.cpp.
|
virtual |
Returns whether the current position of this cursor is a valid position (line + column must both be >= 0).
Smart cursors should override this to return whether the cursor is valid within the linked document.
Reimplemented in KTextEditor::SmartCursor.
Definition at line 47 of file cursor.cpp.
|
virtual |
Retrieve the line on which this cursor is situated.
- Returns
- line number, where 0 is the first line.
Definition at line 62 of file cursor.cpp.
Assignment operator.
Same as setPosition().
- Parameters
-
cursor the position to assign.
- Returns
- a reference to this cursor
- See also
- setPosition()
void Cursor::position | ( | int & | line, |
int & | column | ||
) | const |
Get both the line and column of the cursor position.
- Parameters
-
line will be filled with current cursor line column will be filled with current cursor column
Definition at line 119 of file cursor.cpp.
Range * Cursor::range | ( | ) | const |
Returns the range that this cursor belongs to, if any.
Definition at line 124 of file cursor.cpp.
|
virtual |
Set the cursor column to column.
- Parameters
-
column new cursor column
Definition at line 84 of file cursor.cpp.
|
virtual |
Set the cursor line to line.
- Parameters
-
line new cursor line
Definition at line 67 of file cursor.cpp.
|
virtual |
Set the current cursor position to position.
- Parameters
-
position new cursor position
- Todo:
- add bool to indicate success or not, for smart cursors?
Definition at line 96 of file cursor.cpp.
void Cursor::setPosition | ( | int | line, |
int | column | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Set the cursor position to line and column.
- Parameters
-
line new cursor line column new cursor column
Definition at line 114 of file cursor.cpp.
|
protectedvirtual |
Sets the range that this cursor belongs to.
- Parameters
-
range the range that this cursor is referenced from.
Definition at line 133 of file cursor.cpp.
|
static |
Returns a cursor representing the start of any document - i.e., line 0, column 0.
Definition at line 57 of file cursor.cpp.
|
virtual |
Returns this cursor as a SmartCursor, if it is one.
Reimplemented in KTextEditor::SmartCursor.
Definition at line 158 of file cursor.cpp.
Friends And Related Function Documentation
|
friend |
Equality operator.
- Note
- comparison between two invalid cursors is undefined. comparison between and invalid and a valid cursor will always be false.
- Parameters
-
c1 first cursor to compare c2 second cursor to compare
- Returns
- true, if c1's and c2's line and column are equal.
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.