KTextEditor
#include <smartcursor.h>
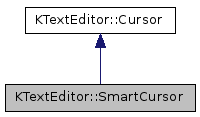
Public Member Functions | |
virtual | ~SmartCursor () |
virtual bool | isSmartCursor () const |
SmartCursor & | operator= (const SmartCursor &cursor) |
SmartRange * | smartRange () const |
virtual SmartCursor * | toSmartCursor () const |
Notification | |
The following functions allow for changes related to this cursor to be notified to 3rd party programs. | |
virtual bool | hasNotifier () const =0 |
virtual SmartCursorNotifier * | notifier ()=0 |
virtual void | deleteNotifier ()=0 |
virtual SmartCursorWatcher * | watcher () const =0 |
virtual void | setWatcher (SmartCursorWatcher *watcher=0L)=0 |
![]() | |
Cursor () | |
Cursor (int line, int column) | |
Cursor (const Cursor ©) | |
virtual | ~Cursor () |
Cursor & | operator= (const Cursor &cursor) |
Range * | range () const |
virtual void | setPosition (const Cursor &position) |
void | setPosition (int line, int column) |
virtual int | line () const |
virtual void | setLine (int line) |
int | column () const |
virtual void | setColumn (int column) |
bool | atStartOfLine () const |
bool | atStartOfDocument () const |
void | position (int &line, int &column) const |
Protected Member Functions | |
SmartCursor (const Cursor &position, Document *doc, InsertBehavior insertBehavior) | |
![]() | |
void | cursorChangedDirectly (const Cursor &from) |
virtual void | setRange (Range *range) |
Document-related functions | |
The following functions are provided for convenient access to the associated Document. | |
enum | AdvanceMode { ByCharacter, ByCursorPosition } |
Document * | document () const |
virtual bool | atEndOfLine () const |
virtual bool | atEndOfDocument () const |
virtual bool | isValid () const |
QChar | character () const |
virtual bool | insertText (const QStringList &text, bool block=false) |
virtual bool | advance (int distance, AdvanceMode mode=ByCharacter) |
Behavior | |
The following functions relate to the behavior of this SmartCursor. | |
enum | InsertBehavior { StayOnInsert = 0, MoveOnInsert } |
InsertBehavior | insertBehavior () const |
void | setInsertBehavior (InsertBehavior insertBehavior) |
Additional Inherited Members | |
![]() | |
static Cursor | invalid () |
static Cursor | start () |
![]() | |
int | m_column |
int | m_line |
Range * | m_range |
Detailed Description
A Cursor which is bound to a specific Document, and maintains its position.
A SmartCursor is an extension of the basic Cursor class. It maintains its position in the document and provides a number of convenience methods, including those for accessing and manipulating the content of the associated Document. As a result of this, SmartCursors may not be copied, as they need to maintain a connection to the associated Document.
To receive notifications when the position of the cursor changes, or other similar notifications, see either SmartCursorNotifier for QObject signal notification via notifier(), or SmartCursorWatcher for virtual inheritance notification via setWatcher().
Create a new SmartCursor like this:
When finished with a SmartCursor, simply delete it.
- See also
- Cursor, SmartCursorNotifier, SmartCursorWatcher, and SmartInterface.
Definition at line 65 of file smartcursor.h.
Member Enumeration Documentation
Defines the ways in which the cursor can be advanced.
Important for languages where multiple characters are required to form one letter.
Definition at line 149 of file smartcursor.h.
Enumerator | |
---|---|
StayOnInsert | |
MoveOnInsert |
Definition at line 187 of file smartcursor.h.
Constructor & Destructor Documentation
|
virtual |
Virtual destructor.
Subclasses should call SmartCursorNotifier::deleted() and SmartCursorWatcher::deleted() methods before cleaning themselves up.
Definition at line 34 of file smartcursor.cpp.
|
protected |
Constructor for subclasses to utilise. Protected to prevent direct instantiation.
- Note
- 3rd party developers: you do not (and should not) need to subclass the Smart* classes; instead, use the SmartInterface to create instances.
- Parameters
-
position the cursor position to assign doc the Document this cursor is associated with insertBehavior the behavior of this cursor when on the position of an insert.
Definition at line 26 of file smartcursor.cpp.
Member Function Documentation
|
virtual |
Move cursor by specified distance along the document buffer.
E.g.:
will move the cursor forward by one character, or, if the cursor is already on the end of the line, will move it to the start of the next line.
- Note
- Negative numbers should be accepted, and move backwards.
- Not all modes are required to be supported.
- Parameters
-
distance distance to advance (or go back if distance is negative) mode whether to move by character, or by number of valid cursor positions
- Returns
- true if the position could be reached within the document, otherwise false (the cursor should not move if distance is beyond the end of the document).
Definition at line 93 of file smartcursor.cpp.
|
virtual |
Determine if this cursor is located at the end of the document.
- Returns
- true if the cursor is situated at the end of the document, otherwise false.
Definition at line 43 of file smartcursor.cpp.
|
virtual |
Determine if this cursor is located at the end of the current line.
- Returns
- true if the cursor is situated at the end of the line, otherwise false.
Definition at line 73 of file smartcursor.cpp.
QChar SmartCursor::character | ( | ) | const |
Returns the character in the document immediately after this position, ie.
from this position to this position plus Cursor(0,1).
Definition at line 58 of file smartcursor.cpp.
|
pure virtual |
Deletes the current SmartCursorNotifier.
When finished with a notifier, call this method to save memory, and potentially editor logic processing time, by having the SmartCursorNotifier deleted.
Document * SmartCursor::document | ( | ) | const |
Returns the document to which this cursor is attached.
Definition at line 68 of file smartcursor.cpp.
|
pure virtual |
Determine if a notifier already exists for this smart cursor.
- Returns
- true if a notifier already exists, otherwise false
SmartCursor::InsertBehavior SmartCursor::insertBehavior | ( | ) | const |
Returns how this cursor behaves when text is inserted at the cursor.
Defaults to moving on insert.
Definition at line 78 of file smartcursor.cpp.
|
virtual |
Insert text
into the associated Document.
- Parameters
-
text text to insert block insert this text as a visual block of text rather than a linear sequence
- Returns
- true on success, otherwise false
Definition at line 53 of file smartcursor.cpp.
|
virtual |
Returns that this cursor is a SmartCursor.
Reimplemented from KTextEditor::Cursor.
Definition at line 48 of file smartcursor.cpp.
|
virtual |
- Reimplemented from superclass.
- See also
- Document::cursorInText()
Reimplemented from KTextEditor::Cursor.
Definition at line 38 of file smartcursor.cpp.
|
pure virtual |
Returns the current SmartCursorNotifier.
If one does not already exist, it will be created.
Connect to the notifier to receive signals indicating change of state of this cursor. The notifier is created at the time it is first requested. If you have finished with notifications for a reasonable period of time you can save memory, and potentially editor logic processing time, by calling deleteNotifier().
- Returns
- a pointer to the current SmartCursorNotifier for this SmartCursor. If one does not already exist, it will be created.
|
inline |
Assignment operator.
Assigns the current position of the provided cursor, c
, only; does not assign watchers, notifiers, behavior, etc.
- Note
- The assignment will be performed even if the provided cursor belongs to another Document.
- Parameters
-
cursor the position to assign.
- Returns
- a reference to this cursor, after assignment has occurred.
- See also
- setPosition()
Definition at line 274 of file smartcursor.h.
void SmartCursor::setInsertBehavior | ( | InsertBehavior | insertBehavior | ) |
Change the behavior of the cursor when text is inserted at the cursor.
If moveOnInsert
is true, the cursor will end up at the end of the insert.
Definition at line 83 of file smartcursor.cpp.
|
pure virtual |
Provide a SmartCursorWatcher to receive calls indicating change of state of this cursor.
To finish receiving notifications, call this function with watcher
set to 0L.
- Parameters
-
watcher the class which will receive notifications about changes to this cursor.
SmartRange * SmartCursor::smartRange | ( | ) | const |
Returns the range that this cursor belongs to, if any.
- See also
- Cursor::range()
Definition at line 63 of file smartcursor.cpp.
|
virtual |
Returns this cursor as a SmartCursor.
Reimplemented from KTextEditor::Cursor.
Definition at line 88 of file smartcursor.cpp.
|
pure virtual |
Returns a pointer to the current SmartCursorWatcher, if one has been set.
- Returns
- the current SmartCursorWatcher pointer if one exists, otherwise null.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.