KTextEditor
#include <view.h>
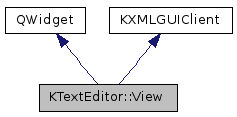
Public Types | |
enum | EditMode { EditInsert = 0, EditOverwrite = 1, EditViMode = 2 } |
![]() | |
enum | ReverseStateChange |
Signals | |
void | contextMenuAboutToShow (KTextEditor::View *view, QMenu *menu) |
void | cursorPositionChanged (KTextEditor::View *view, const KTextEditor::Cursor &newPosition) |
void | focusIn (KTextEditor::View *view) |
void | focusOut (KTextEditor::View *view) |
void | horizontalScrollPositionChanged (KTextEditor::View *view) |
void | informationMessage (KTextEditor::View *view, const QString &message) |
void | mousePositionChanged (KTextEditor::View *view, const KTextEditor::Cursor &newPosition) |
void | selectionChanged (KTextEditor::View *view) |
void | textInserted (KTextEditor::View *view, const KTextEditor::Cursor &position, const QString &text) |
void | verticalScrollPositionChanged (KTextEditor::View *view, const KTextEditor::Cursor &newPos) |
void | viewEditModeChanged (KTextEditor::View *view, enum KTextEditor::View::EditMode mode) |
void | viewModeChanged (KTextEditor::View *view) |
Additional Inherited Members | |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
A text widget with KXMLGUIClient that represents a Document.
Topics:
- Introduction
- Merging the View's GUI
- Text Selection
- Cursor Positions
- Mouse Tracking
- Edit Modes
- View Extension Interfaces
Introduction
The View class represents a single view of a KTextEditor::Document, get the document on which the view operates with document(). A view provides both the graphical representation of the text and the KXMLGUIClient for the actions. The view itself does not provide text manipulation, use the methods from the Document instead. The only method to insert text is insertText(), which inserts the given text at the current cursor position and emits the signal textInserted().
Usually a view is created by using Document::createView(). Furthermore a view can have a context menu. Set it with setContextMenu() and get it with contextMenu().
Merging the View's GUI
A View is derived from the class KXMLGUIClient, so its GUI elements (like menu entries and toolbar items) can be merged into the application's GUI (or into a KXMLGUIFactory) by calling
You can add only one view as client, so if you have several views, you first have to remove the current view, and then add the new one, like this
Text Selection
As the view is a graphical text editor it provides normal and block text selection. You can check with selection() whether a selection exists. removeSelection() will remove the selection without removing the text, whereas removeSelectionText() also removes both, the selection and the selected text. Use selectionText() to get the selected text and setSelection() to specify the selected textrange. The signal selectionChanged() is emitted whenever the selecteion changed.
Cursor Positions
A view has one Cursor which represents a line/column tuple. Two different kinds of cursor positions are supported: first is the real cursor position where a tab character only counts one character. Second is the virtual cursor position, where a tab character counts as many spaces as defined. Get the real position with cursorPosition() and the virtual position with cursorPositionVirtual(). Set the real cursor position with setCursorPosition(). You can even get the screen coordinates of the current cursor position in pixel by using cursorPositionCoordinates(). The signal cursorPositionChanged() is emitted whenever the cursor position changed.
Mouse Tracking
It is possible to get notified via the signal mousePositionChanged() for mouse move events, if mouseTrackingEnabled() returns true. Mouse tracking can be turned on/off by calling setMouseTrackingEnabled(). If an editor implementation does not support mouse tracking, mouseTrackingEnabled() will always return false.
Edit Modes
A view supports several edit modes (EditMode). Common edit modes are insert-mode (INS) and overwrite-mode (OVR). Which edit modes the editor supports depends on the implementation, another well-known mode is the command-mode for example in vim and yzis. The getter viewMode() returns a string like INS
or OVR
and is represented in the user interface for example in the status bar. Further you can get the edit mode as enum by using viewEditMode(). Whenever the edit mode changed the signals viewModeChanged() and viewEditModeChanged() are emitted.
View Extension Interfaces
A simple view represents the text of a Document and provides a text cursor, text selection, edit modes etc. Advanced concepts like code completion and text hints are defined in the extension interfaces. An KTextEditor implementation does not need to support all the extensions. To implement the interfaces multiple inheritance is used.
More information about interfaces for the view can be found in View Extension Interfaces.
Member Enumeration Documentation
Constructor & Destructor Documentation
View::View | ( | QWidget * | parent | ) |
Constructor.
Create a view attached to the widget parent
.
- Parameters
-
parent parent widget
- See also
- Document::createView()
Definition at line 197 of file ktexteditor.cpp.
|
virtual |
Virtual destructor.
Definition at line 202 of file ktexteditor.cpp.
Member Function Documentation
|
pure virtual |
Get the status of the selection mode.
true indicates that block selection mode is on. If this is true, selections applied via the SelectionInterface are handled as block selections and the Copy&Paste functions work on rectangular blocks of text rather than normal.
- Returns
- true, if block selection mode is enabled, otherwise false
- See also
- setBlockSelection()
|
pure virtual |
Get the context menu for this view.
The return value can be NULL if no context menu object was set and kxmlgui is not initialized yet. If there is no user set menu, the kxmlgui menu is returned. Do not delete this menu, if if it is the xmlgui menu.
- Returns
- context menu object
- See also
- setContextMenu()
|
signal |
Signal which is emitted immediately prior to showing the current context menu.
|
pure virtual |
Get the view's current cursor position.
A TAB character is handeled as only one character.
- Returns
- current cursor position
- See also
- setCursorPosition()
|
signal |
This signal is emitted whenever the view's
cursor position changed.
- Parameters
-
view view which emitted the signal newPosition new position of the cursor (Kate will pass the real cursor potition, not the virtual)
- See also
- cursorPosition(), cursorPositionVirtual()
|
pure virtual |
Get the screen coordinates (x/y) of the cursor position in pixels.
- Returns
- cursor screen coordinates
|
pure virtual |
Get the current virtual cursor position, virtual means the tabulator character (TAB) counts multiple characters, as configured by the user (e.g.
one TAB is 8 spaces). The virtual cursor position provides access to the user visible values of the current cursor position.
- Returns
- virtual cursor position
- See also
- cursorPosition()
|
pure virtual |
Get the screen coordinates (x, y) of the supplied cursor relative to the view widget in pixels.
Thus, 0,0 represents the top left hand of the view widget.
- Parameters
-
cursor cursor to determine coordinate for.
- Returns
- cursor screen coordinates relative to the view widget
Populate menu with default text editor actions.
If menu is null, a menu will be created with the view as its parent.
- Note
- to use this menu, you will next need to call setContextMenu(), as this does not assign the new context menu.
- Warning
- This contains only basic options from the editor component (katepart). Plugins are
not
merged/integrated into it! If you want to be a better citizen and take full advantage of KTextEditor plugins do something like:KXMLGUIClient* client = view;// search parent XmlGuiClientwhile (client->parentClient()) {client = client->parentClient();}if (client->factory()) {foreach (QWidget *w, conts) {if (w->objectName() == "ktexteditor_popup") {// do something with the menu (ie adding an onshow handler)break;}}} - or simply use the aboutToShow, aboutToHide signals !!!!!
- Parameters
-
menu the menu to be populated, or null to create a new menu.
- Returns
- the menu, whether created or passed initially
|
pure virtual |
Get the view's document, that means the view is a view of the returned document.
- Returns
- the view's document
|
signal |
This signal is emitted whenever the view
gets the focus.
- Parameters
-
view view which gets focus
- See also
- focusOut()
|
signal |
This signal is emitted whenever the view
loses the focus.
- Parameters
-
view view which lost focus
- See also
- focusIn()
|
signal |
This signal should be emitted whenever the view
is scrolled horizontally.
- Parameters
-
view view which emitted the signal
|
signal |
This signal is emitted whenever the view
wants to display a information message
.
The message
can be displayed in the status bar for example.
- Parameters
-
view view which sends out information message information message
This is a convenience function which inserts text
at the view's current cursor position.
You do not necessarily need to reimplement it, except you want to do some special things.
- Parameters
-
text Text to be inserted
- Returns
- true on success of insertion, otherwise false
- See also
- textInserted()
Definition at line 139 of file ktexteditor.cpp.
bool View::isActiveView | ( | ) | const |
Check whether this view is the document's active view.
This is equal to the code:
Definition at line 116 of file ktexteditor.cpp.
|
signal |
This signal is emitted whenever the position of the mouse changes over this view.
If the mouse moves off the view, an invalid cursor position should be emitted, i.e. Cursor::invalid().
- Note
- If mouseTrackingEnabled() returns false, this signal is never emitted.
- Parameters
-
view view which emitted the signal newPosition new position of the mouse or Cursor::invalid(), if the mouse moved out of the view
.
- See also
- mouseTrackingEnabled()
|
pure virtual |
Check, whether mouse tracking is enabled.
Mouse tracking is required to have the signal mousePositionChanged() emitted.
- Returns
- true, if mouse tracking is enabled, otherwise false
|
pure virtual |
Remove the view's current selection, without deleting the selected text.
- Returns
- true on success, otherwise false
- See also
- removeSelectionText()
|
pure virtual |
Remove the view's current selection including the selected text.
- Returns
- true on success, otherwise false
- See also
- removeSelection()
|
pure virtual |
Query the view whether it has selected text, i.e.
whether a selection exists.
- Returns
- true if a text selection exists, otherwise false
- See also
- setSelection(), selectionRange()
|
signal |
This signal is emitted whenever the view's
selection changes.
- Note
- If the mode switches from block selection to normal selection or vice versa this signal should also be emitted.
- Parameters
-
view view in which the selection changed
- See also
- selection(), selectionRange(), selectionText()
|
pure virtual |
Get the range occupied by the current selection.
- Returns
- selection range, valid only if a selection currently exists.
- See also
- setSelection()
|
pure virtual |
Set block selection mode to state on
.
- Parameters
-
on if true, block selection mode is turned on, otherwise off
- Returns
- true on success, otherwise false
- See also
- blockSelection()
|
pure virtual |
Set a context menu for this view to menu
.
- Note
- any previously assigned menu is not deleted. If you are finished with the previous menu, you may delete it.
- Warning
- Use this with care! Plugin xml gui clients are not merged into this menu!
- !!!!!! DON'T USE THIS FUNCTION, UNLESS YOU ARE SURE YOU DON'T WANT PLUGINS TO WORK !!!!!!
- Parameters
-
menu new context menu object for this view
- See also
- contextMenu()
Set the view's new cursor to position
.
A TAB character is handeled as only on character.
- Parameters
-
position new cursor position
- Returns
- true on success, otherwise false
- See also
- cursorPosition()
Try to enable or disable mouse tracking according to enable
.
The return value contains the state of mouse tracking after the request. Mouse tracking is required to have the mousePositionChanged() signal emitted.
- Note
- Implementation Notes: An implementation is not forced to support this, and should always return false if it does not have support.
- Parameters
-
enable if true, try to enable mouse tracking, otherwise disable it.
- Returns
- the current state of mouse tracking
Set the view's selection to the range selection
.
The old selection will be discarded.
- Parameters
-
range the range of the new selection
- Returns
- true on success, otherwise false (e.g. when the cursor range is invalid)
- See also
- selectionRange(), selection()
This is an overloaded member function, provided for convenience, it differs from the above function only in what argument(s) it accepts.
An existing old selection will be discarded. If possible you should reimplement the default implementation with a more efficient one.
- Parameters
-
position start or end position of the selection, depending on the length
parameterlength if >0 position
defines the start of the selection, if <0position
specifies the endwrap if false the selection does not wrap lines and reaches only to start/end of the cursors line. Default: true
- See also
- selectionRange(), selection()
- Todo:
- rodda - is this really needed? it can now be accomplished with SmartCursor::advance()
Definition at line 121 of file ktexteditor.cpp.
|
signal |
This signal is emitted from view
whenever the users inserts text
at position
, that means the user typed/pasted text.
- Parameters
-
view view in which the text was inserted position position where the text was inserted text the text the user has typed into the editor
- See also
- insertText()
|
signal |
This signal should be emitted whenever the view
is scrolled vertically.
- Parameters
-
view view which emitted the signal newPos the new scroll position
|
pure virtual |
Get the view's current edit mode.
The current mode can be insert mode, replace mode or any other the editor supports, e.g. a vim like command mode. If in doubt return EditInsert.
- Returns
- the current edit mode of this view
- See also
- viewEditModeChanged()
|
signal |
This signal is emitted whenever the view's
edit mode
changed from either EditInsert to EditOverwrite or vice versa.
- Parameters
-
view view which changed its edit mode mode new edit mode
- See also
- viewEditMode()
|
pure virtual |
Get the current view mode/state.
This can be used to visually indicate the view's current mode, for example INSERT mode, OVERWRITE mode or COMMAND mode - or whatever other edit modes are supported. The string should be translated (i18n), as this is a user aimed representation of the view state, which should be shown in the GUI, for example in the status bar. This string may be rich-text.
- Returns
- See also
- viewModeChanged()
|
signal |
This signal is emitted whenever the view mode of view
changes.
- Parameters
-
view the view which changed its mode
- See also
- viewMode()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.