Konsole
#include <ScreenWindow.h>
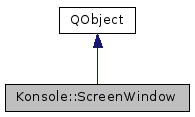
Public Types | |
enum | RelativeScrollMode { ScrollLines, ScrollPages } |
Public Slots | |
void | notifyOutputChanged () |
Signals | |
void | currentResultLineChanged () |
void | outputChanged () |
void | scrolled (int line) |
void | selectionChanged () |
Public Member Functions | |
ScreenWindow (QObject *parent=0) | |
virtual | ~ScreenWindow () |
bool | atEndOfOutput () const |
void | clearSelection () |
int | columnCount () const |
int | currentLine () const |
int | currentResultLine () const |
QPoint | cursorPosition () const |
Character * | getImage () |
QVector< LineProperty > | getLineProperties () |
void | getSelectionEnd (int &column, int &line) |
void | getSelectionStart (int &column, int &line) |
bool | isSelected (int column, int line) |
int | lineCount () const |
void | resetScrollCount () |
Screen * | screen () const |
void | scrollBy (RelativeScrollMode mode, int amount, bool fullPage) |
int | scrollCount () const |
QRect | scrollRegion () const |
void | scrollTo (int line) |
QString | selectedText (bool preserveLineBreaks, bool trimTrailingSpaces=false) const |
void | setCurrentResultLine (int line) |
void | setScreen (Screen *screen) |
void | setSelectionByLineRange (int start, int end) |
void | setSelectionEnd (int column, int line) |
void | setSelectionStart (int column, int line, bool columnMode) |
void | setTrackOutput (bool trackOutput) |
void | setWindowLines (int lines) |
bool | trackOutput () const |
int | windowColumns () const |
int | windowLines () const |
Detailed Description
Provides a window onto a section of a terminal screen.
A terminal widget can then render the contents of the window and use the window to change the terminal screen's selection in response to mouse or keyboard input.
A new ScreenWindow for a terminal session can be created by calling Emulation::createWindow()
Use the scrollTo() method to scroll the window up and down on the screen. Use the getImage() method to retrieve the character image which is currently visible in the window.
setTrackOutput() controls whether the window moves to the bottom of the associated screen when new lines are added to it.
Whenever the output from the underlying screen is changed, the notifyOutputChanged() slot should be called. This in turn will update the window's position and emit the outputChanged() signal if necessary.
Definition at line 52 of file ScreenWindow.h.
Member Enumeration Documentation
Describes the units which scrollBy() moves the window by.
Enumerator | |
---|---|
ScrollLines |
Scroll the window down by a given number of lines. |
ScrollPages |
Scroll the window down by a given number of pages, where one page is windowLines() lines. |
Definition at line 193 of file ScreenWindow.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a new screen window with the given parent.
A screen must be specified by calling setScreen() before calling getImage() or getLineProperties().
You should not call this constructor directly, instead use the Emulation::createWindow() method to create a window on the emulation which you wish to view. This allows the emulation to notify the window when the associated screen has changed and synchronize selection updates between all views on a session.
Definition at line 28 of file ScreenWindow.cpp.
|
virtual |
Definition at line 41 of file ScreenWindow.cpp.
Member Function Documentation
bool ScreenWindow::atEndOfOutput | ( | ) | const |
Convenience method.
Returns true if the window is currently at the bottom of the screen.
Definition at line 243 of file ScreenWindow.cpp.
void ScreenWindow::clearSelection | ( | ) |
Clears the current selection.
Definition at line 169 of file ScreenWindow.cpp.
int ScreenWindow::columnCount | ( | ) | const |
Returns the total number of columns in the screen.
Definition at line 196 of file ScreenWindow.cpp.
int ScreenWindow::currentLine | ( | ) | const |
Returns the index of the line which is currently at the top of this window.
Definition at line 211 of file ScreenWindow.cpp.
int ScreenWindow::currentResultLine | ( | ) | const |
Definition at line 216 of file ScreenWindow.cpp.
|
signal |
QPoint ScreenWindow::cursorPosition | ( | ) | const |
Returns the position of the cursor within the window.
Definition at line 201 of file ScreenWindow.cpp.
Character * ScreenWindow::getImage | ( | ) |
Returns the image of characters which are currently visible through this window onto the screen.
The returned buffer is managed by the ScreenWindow instance and does not need to be deleted by the caller.
Definition at line 57 of file ScreenWindow.cpp.
QVector< LineProperty > ScreenWindow::getLineProperties | ( | ) |
Returns the line attributes associated with the lines of characters which are currently visible through this window.
Definition at line 112 of file ScreenWindow.cpp.
void ScreenWindow::getSelectionEnd | ( | int & | column, |
int & | line | ||
) |
Retrieves the end of the selection within the window.
Definition at line 132 of file ScreenWindow.cpp.
void ScreenWindow::getSelectionStart | ( | int & | column, |
int & | line | ||
) |
Retrieves the start of the selection within the window.
Definition at line 127 of file ScreenWindow.cpp.
bool ScreenWindow::isSelected | ( | int | column, |
int | line | ||
) |
Returns true if the character at line
, column
is part of the selection.
Definition at line 164 of file ScreenWindow.cpp.
int ScreenWindow::lineCount | ( | ) | const |
Returns the total number of lines in the screen.
Definition at line 191 of file ScreenWindow.cpp.
|
slot |
Notifies the window that the contents of the associated terminal screen have changed.
This moves the window to the bottom of the screen if trackOutput() is true and causes the outputChanged() signal to be emitted.
Definition at line 295 of file ScreenWindow.cpp.
|
signal |
Emitted when the contents of the associated terminal screen (see screen()) changes.
void ScreenWindow::resetScrollCount | ( | ) |
Resets the count of scrolled lines returned by scrollCount()
Definition at line 280 of file ScreenWindow.cpp.
Screen * ScreenWindow::screen | ( | ) | const |
Returns the screen which this window looks onto.
Definition at line 52 of file ScreenWindow.cpp.
void ScreenWindow::scrollBy | ( | RelativeScrollMode | mode, |
int | amount, | ||
bool | fullPage | ||
) |
Scrolls the window relative to its current position on the screen.
- Parameters
-
mode Specifies whether amount
refers to the number of lines or the number of pages to scroll.amount The number of lines or pages ( depending on mode
) to scroll by. If this number is positive, the view is scrolled down. If this number is negative, the view is scrolled up.fullPage Specifies whether to scroll by full page or half page.
Definition at line 231 of file ScreenWindow.cpp.
int ScreenWindow::scrollCount | ( | ) | const |
Returns the number of lines which the region of the window specified by scrollRegion() has been scrolled by since the last call to resetScrollCount().
scrollRegion() is in most cases the whole window, but will be a smaller area in, for example, applications which provide split-screen facilities.
This is not guaranteed to be accurate, but allows views to optimize rendering by reducing the amount of costly text rendering that needs to be done when the output is scrolled.
Definition at line 275 of file ScreenWindow.cpp.
|
signal |
Emitted when the screen window is scrolled to a different position.
- Parameters
-
line The line which is now at the top of the window.
QRect ScreenWindow::scrollRegion | ( | ) | const |
Returns the area of the window which was last scrolled, this is usually the whole window area.
Like scrollCount(), this is not guaranteed to be accurate, but allows views to optimize rendering.
Definition at line 285 of file ScreenWindow.cpp.
void ScreenWindow::scrollTo | ( | int | line | ) |
Scrolls the window so that line
is at the top of the window.
Definition at line 248 of file ScreenWindow.cpp.
QString ScreenWindow::selectedText | ( | bool | preserveLineBreaks, |
bool | trimTrailingSpaces = false |
||
) | const |
Returns the text which is currently selected.
- Parameters
-
preserveLineBreaks See Screen::selectedText() trimTrailingSpaces See Screen::selectedText()
Definition at line 122 of file ScreenWindow.cpp.
|
signal |
Emitted when the selection is changed.
void ScreenWindow::setCurrentResultLine | ( | int | line | ) |
What line the next search will start from.
Definition at line 221 of file ScreenWindow.cpp.
void ScreenWindow::setScreen | ( | Screen * | screen | ) |
Sets the screen which this window looks onto.
Definition at line 45 of file ScreenWindow.cpp.
void ScreenWindow::setSelectionByLineRange | ( | int | start, |
int | end | ||
) |
Sets the selection as the range specified by line start
and line end
in the whole history.
Both start
and end
are absolute line number in the whole history, not relative line number in the window. This make it possible to select range larger than the window . A good use case is selecting the whole history.
Definition at line 153 of file ScreenWindow.cpp.
void ScreenWindow::setSelectionEnd | ( | int | column, |
int | line | ||
) |
Sets the end of the selection to the given line
and column
within the window.
Definition at line 145 of file ScreenWindow.cpp.
void ScreenWindow::setSelectionStart | ( | int | column, |
int | line, | ||
bool | columnMode | ||
) |
Sets the start of the selection to the given line
and column
within the window.
Definition at line 137 of file ScreenWindow.cpp.
void ScreenWindow::setTrackOutput | ( | bool | trackOutput | ) |
Specifies whether the window should automatically move to the bottom of the screen when new output is added.
If this is set to true, the window will be moved to the bottom of the associated screen ( see screen() ) when the notifyOutputChanged() method is called.
Definition at line 265 of file ScreenWindow.cpp.
void ScreenWindow::setWindowLines | ( | int | lines | ) |
Sets the number of lines in the window.
Definition at line 176 of file ScreenWindow.cpp.
bool ScreenWindow::trackOutput | ( | ) | const |
Returns whether the window automatically moves to the bottom of the screen as new output is added.
See setTrackOutput()
Definition at line 270 of file ScreenWindow.cpp.
int ScreenWindow::windowColumns | ( | ) | const |
Returns the number of columns in the window.
Definition at line 186 of file ScreenWindow.cpp.
int ScreenWindow::windowLines | ( | ) | const |
Returns the number of lines in the window.
Definition at line 181 of file ScreenWindow.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.