Konsole
#include <Emulation.h>
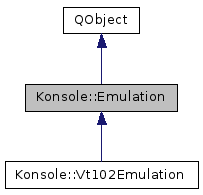
Public Slots | |
void | receiveData (const char *buffer, int len) |
virtual void | sendKeyEvent (QKeyEvent *) |
virtual void | sendMouseEvent (int buttons, int column, int line, int eventType) |
virtual void | sendString (const char *string, int length=-1)=0 |
virtual void | sendText (const QString &text)=0 |
virtual void | setImageSize (int lines, int columns) |
Signals | |
void | changeTabTextColorRequest (int color) |
void | flowControlKeyPressed (bool suspendKeyPressed) |
void | imageResizeRequest (const QSize &sizz) |
void | imageSizeChanged (int lineCount, int columnCount) |
void | imageSizeInitialized () |
void | outputChanged () |
void | primaryScreenInUse (bool use) |
void | profileChangeCommandReceived (const QString &text) |
void | programUsesMouseChanged (bool usesMouse) |
void | selectionChanged (const QString &text) |
void | sendData (const char *data, int len) |
void | stateSet (int state) |
void | titleChanged (int title, const QString &newTitle) |
void | useUtf8Request (bool) |
void | zmodemDetected () |
Public Member Functions | |
Emulation () | |
~Emulation () | |
virtual void | clearEntireScreen ()=0 |
void | clearHistory () |
const QTextCodec * | codec () const |
ScreenWindow * | createWindow () |
virtual char | eraseChar () const |
const HistoryType & | history () const |
QSize | imageSize () const |
QString | keyBindings () const |
int | lineCount () const |
bool | programUsesMouse () const |
virtual void | reset ()=0 |
void | setCodec (const QTextCodec *) |
void | setHistory (const HistoryType &) |
void | setKeyBindings (const QString &name) |
bool | utf8 () const |
virtual void | writeToStream (TerminalCharacterDecoder *decoder, int startLine, int endLine) |
Protected Types | |
enum | EmulationCodec { LocaleCodec = 0, Utf8Codec = 1 } |
Protected Slots | |
void | bufferedUpdate () |
void | checkScreenInUse () |
void | checkSelectedText () |
Protected Member Functions | |
virtual void | receiveChar (int ch) |
virtual void | resetMode (int mode)=0 |
void | setCodec (EmulationCodec codec) |
virtual void | setMode (int mode)=0 |
void | setScreen (int index) |
Protected Attributes | |
const QTextCodec * | _codec |
Screen * | _currentScreen |
QTextDecoder * | _decoder |
const KeyboardTranslator * | _keyTranslator |
Screen * | _screen [2] |
QList< ScreenWindow * > | _windows |
Detailed Description
Base class for terminal emulation back-ends.
The back-end is responsible for decoding an incoming character stream and producing an output image of characters.
When input from the terminal is received, the receiveData() slot should be called with the data which has arrived. The emulation will process the data and update the screen image accordingly. The codec used to decode the incoming character stream into the unicode characters used internally can be specified using setCodec()
The size of the screen image can be specified by calling setImageSize() with the desired number of lines and columns. When new lines are added, old content is moved into a history store, which can be set by calling setHistory().
The screen image can be accessed by creating a ScreenWindow onto this emulation by calling createWindow(). Screen windows provide access to a section of the output. Each screen window covers the same number of lines and columns as the image size returned by imageSize(). The screen window can be moved up and down and provides transparent access to both the current on-screen image and the previous output. The screen windows emit an outputChanged signal when the section of the image they are looking at changes. Graphical views can then render the contents of a screen window, listening for notifications of output changes from the screen window which they are associated with and updating accordingly.
The emulation also is also responsible for converting input from the connected views such as keypresses and mouse activity into a character string which can be sent to the terminal program. Key presses can be processed by calling the sendKeyEvent() slot, while mouse events can be processed using the sendMouseEvent() slot. When the character stream has been produced, the emulation will emit a sendData() signal with a pointer to the character buffer. This data should be fed to the standard input of the terminal process. The translation of key presses into an output character stream is performed using a lookup in a set of key bindings which map key sequences to output character sequences. The name of the key bindings set used can be specified using setKeyBindings()
The emulation maintains certain state information which changes depending on the input received. The emulation can be reset back to its starting state by calling reset().
The emulation also maintains an activity state, which specifies whether terminal is currently active ( when data is received ), normal ( when the terminal is idle or receiving user input ) or trying to alert the user ( also known as a "Bell" event ). The stateSet() signal is emitted whenever the activity state is set. This can be used to determine how long the emulation has been active/idle for and also respond to a 'bell' event in different ways.
Definition at line 117 of file Emulation.h.
Member Enumeration Documentation
|
protected |
Enumerator | |
---|---|
LocaleCodec | |
Utf8Codec |
Definition at line 432 of file Emulation.h.
Constructor & Destructor Documentation
Emulation::Emulation | ( | ) |
Constructs a new terminal emulation.
Definition at line 36 of file Emulation.cpp.
Emulation::~Emulation | ( | ) |
Definition at line 95 of file Emulation.cpp.
Member Function Documentation
|
protectedslot |
Schedules an update of attached views.
Repeated calls to bufferedUpdate() in close succession will result in only a single update, much like the Qt buffered update of widgets.
Definition at line 308 of file Emulation.cpp.
|
signal |
Requests that the color of the text used to represent the tabs associated with this emulation be changed.
This is a Konsole-specific extension from pre-KDE 4 times.
TODO: Document how the parameter works.
|
protectedslot |
Definition at line 84 of file Emulation.cpp.
|
protectedslot |
Definition at line 89 of file Emulation.cpp.
|
pure virtual |
Copies the current image into the history and clears the screen.
Implemented in Konsole::Vt102Emulation.
void Emulation::clearHistory | ( | ) |
Clears the history scroll.
Definition at line 121 of file Emulation.cpp.
|
inline |
Returns the codec used to decode incoming characters.
See setCodec()
Definition at line 169 of file Emulation.h.
ScreenWindow * Emulation::createWindow | ( | ) |
Creates a new window onto the output from this emulation.
The contents of the window are then rendered by views which are set to use this window using the TerminalDisplay::setScreenWindow() method.
Definition at line 67 of file Emulation.cpp.
|
virtual |
Returns the special character used for erasing character.
Reimplemented in Konsole::Vt102Emulation.
Definition at line 321 of file Emulation.cpp.
|
signal |
Emitted when a flow control key combination ( Ctrl+S or Ctrl+Q ) is pressed.
- Parameters
-
suspendKeyPressed True if Ctrl+S was pressed to suspend output or Ctrl+Q to resume output.
const HistoryType & Emulation::history | ( | ) | const |
Returns the history store used by this emulation.
See setHistory()
Definition at line 132 of file Emulation.cpp.
|
signal |
Emitted after receiving the escape sequence which asks to change the terminal emulator's size.
QSize Emulation::imageSize | ( | ) | const |
Returns the size of the screen image which the emulation produces.
Definition at line 366 of file Emulation.cpp.
|
signal |
Emitted when the terminal emulator's size has changed.
|
signal |
Emitted when the setImageSize() is called on this emulation for the first time.
QString Emulation::keyBindings | ( | ) | const |
Returns the name of the emulation's current key bindings.
See setKeyBindings()
Definition at line 167 of file Emulation.cpp.
int Emulation::lineCount | ( | ) | const |
Returns the total number of lines, including those stored in the history.
Definition at line 291 of file Emulation.cpp.
|
signal |
Emitted when the contents of the screen image change.
The emulation buffers the updates from successive image changes, and only emits outputChanged() at sensible intervals when there is a lot of terminal activity.
Normally there is no need for objects other than the screen windows created with createWindow() to listen for this signal.
ScreenWindow objects created using createWindow() will emit their own outputChanged() signal in response to this signal.
|
signal |
Emitted when the active screen is switched, to indicate whether the primary screen is in use.
|
signal |
Emitted when the terminal program requests to change various properties of the terminal display.
A profile change command occurs when a special escape sequence, followed by a string containing a series of name and value pairs is received. This string can be parsed using a ProfileCommandParser instance.
- Parameters
-
text A string expected to contain a series of key and value pairs in the form: name=value;name2=value2 ...
bool Emulation::programUsesMouse | ( | ) | const |
Returns true if the active terminal program wants mouse input events.
The programUsesMouseChanged() signal is emitted when this changes.
Definition at line 57 of file Emulation.cpp.
|
signal |
This is emitted when the program running in the shell indicates whether or not it is interested in mouse events.
- Parameters
-
usesMouse This will be true if the program wants to be informed about mouse events or false otherwise.
|
protectedvirtual |
Processes an incoming character.
See receiveData() ch
A unicode character code.
Reimplemented in Konsole::Vt102Emulation.
Definition at line 174 of file Emulation.cpp.
|
slot |
Processes an incoming stream of characters.
receiveData() decodes the incoming character buffer using the current codec(), and then calls receiveChar() for each unicode character in the resulting buffer.
receiveData() also starts a timer which causes the outputChanged() signal to be emitted when it expires. The timer allows multiple updates in quick succession to be buffered into a single outputChanged() signal emission.
- Parameters
-
buffer A string of characters received from the terminal program. len The length of buffer
Definition at line 213 of file Emulation.cpp.
|
pure virtual |
Resets the state of the terminal.
Implemented in Konsole::Vt102Emulation.
|
protectedpure virtual |
Implemented in Konsole::Vt102Emulation.
|
signal |
Emitted when the text selection is changed.
|
signal |
Emitted when a buffer of data is ready to send to the standard input of the terminal.
- Parameters
-
data The buffer of data ready to be sent len The length of data
in bytes
|
virtualslot |
Interprets a key press event and emits the sendData() signal with the resulting character stream.
Definition at line 187 of file Emulation.cpp.
|
virtualslot |
Converts information about a mouse event into an xterm-compatible escape sequence and emits the character sequence via sendData()
Definition at line 204 of file Emulation.cpp.
|
pure virtualslot |
Sends a string of characters to the foreground terminal process.
- Parameters
-
string The characters to send. length Length of string
or if set to a negative value,string
will be treated as a null-terminated string and its length will be determined automatically.
Definition at line 199 of file Emulation.cpp.
|
pure virtualslot |
Interprets a sequence of characters and sends the result to the terminal.
This is equivalent to calling sendKeyEvent() for each character in text
in succession.
void Emulation::setCodec | ( | const QTextCodec * | codec | ) |
Sets the codec used to decode incoming characters.
Definition at line 137 of file Emulation.cpp.
|
protected |
Definition at line 151 of file Emulation.cpp.
void Emulation::setHistory | ( | const HistoryType & | history | ) |
Sets the history store used by this emulation.
When new lines are added to the output, older lines at the top of the screen are transferred to a history store.
The number of lines which are kept and the storage location depend on the type of store.
Definition at line 125 of file Emulation.cpp.
|
virtualslot |
Change the size of the emulation's image.
Definition at line 326 of file Emulation.cpp.
void Emulation::setKeyBindings | ( | const QString & | name | ) |
Sets the key bindings used to key events ( received through sendKeyEvent() ) into character streams to send to the terminal.
Definition at line 159 of file Emulation.cpp.
|
protectedpure virtual |
Implemented in Konsole::Vt102Emulation.
|
protected |
Sets the active screen.
The terminal has two screens, primary and alternate. The primary screen is used by default. When certain interactive programs such as Vim are run, they trigger a switch to the alternate screen.
- Parameters
-
index 0 to switch to the primary screen, or 1 to switch to the alternate screen
Definition at line 106 of file Emulation.cpp.
|
signal |
Emitted when the activity state of the emulation is set.
- Parameters
-
state The new activity state, one of NOTIFYNORMAL, NOTIFYACTIVITY or NOTIFYBELL
|
signal |
Emitted when the program running in the terminal wishes to update the session's title.
This also allows terminal programs to customize other aspects of the terminal emulation display.
This signal is emitted when the escape sequence "\033]ARG;VALUE\007" is received in the input string, where ARG is a number specifying what should change and VALUE is a string specifying the new value.
TODO: The name of this method is not very accurate since this method is used to perform a whole range of tasks besides just setting the user-title of the session.
- Parameters
-
title Specifies what to change. -
0 - Set window icon text and session title to
newTitle
-
1 - Set window icon text to
newTitle
-
2 - Set session title to
newTitle
-
11 - Set the session's default background color to
newTitle
, wherenewTitle
can be an HTML-style string ("#RRGGBB") or a named color (eg 'red', 'blue'). See http://doc.trolltech.com/4.2/qcolor.html#setNamedColor for more details. -
31 - Supposedly treats
newTitle
as a URL and opens it (NOT IMPLEMENTED) -
32 - Sets the icon associated with the session.
newTitle
is the name of the icon to use, which can be the name of any icon in the current KDE icon theme (eg: 'konsole', 'kate', 'folder_home')
newTitle Specifies the new title -
0 - Set window icon text and session title to
|
signal |
Requests that the pty used by the terminal process be set to UTF 8 mode.
Refer to the IUTF8 entry in termios(3) for more information.
|
inline |
Convenience method.
Returns true if the current codec used to decode incoming characters is UTF-8
Definition at line 180 of file Emulation.h.
|
virtual |
Copies the output history from startLine
to endLine
into stream
, using decoder
to convert the terminal characters into text.
- Parameters
-
decoder A decoder which converts lines of terminal characters with appearance attributes into output text. PlainTextDecoder is the most commonly used decoder. startLine Index of first line to copy endLine Index of last line to copy
Definition at line 284 of file Emulation.cpp.
|
signal |
Emitted when the special sequence indicating the request for data transmission through ZModem protocol is detected.
Member Data Documentation
|
protected |
Definition at line 452 of file Emulation.h.
|
protected |
Definition at line 441 of file Emulation.h.
|
protected |
Definition at line 453 of file Emulation.h.
|
protected |
Definition at line 454 of file Emulation.h.
|
protected |
Definition at line 444 of file Emulation.h.
|
protected |
Definition at line 439 of file Emulation.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.