Konsole
#include <TerminalDisplay.h>
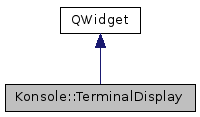
Classes | |
struct | DragInfo |
Public Slots | |
void | bell (const QString &message) |
void | copyToClipboard () |
void | copyToX11Selection () |
bool | flowControlWarningEnabled () const |
QColor | getBackgroundColor () const |
void | outputSuspended (bool suspended) |
void | pasteFromClipboard (bool appendEnter=false) |
void | pasteFromX11Selection (bool appendEnter=false) |
void | scrollScreenWindow (enum ScreenWindow::RelativeScrollMode mode, int amount) |
void | setAutoCopySelectedText (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setCenterContents (bool enable) |
void | setFlowControlWarningEnabled (bool enabled) |
void | setForegroundColor (const QColor &color) |
void | setMargin (int margin) |
void | setMiddleClickPasteMode (Enum::MiddleClickPasteModeEnum mode) |
void | setUsesMouse (bool usesMouse) |
void | updateImage () |
void | updateLineProperties () |
bool | usesMouse () const |
Signals | |
void | changedContentSizeSignal (int height, int width) |
void | changedFontMetricSignal (int height, int width) |
void | configureRequest (const QPoint &position) |
void | keyPressedSignal (QKeyEvent *event) |
void | mouseSignal (int button, int column, int line, int eventType) |
void | overrideShortcutCheck (QKeyEvent *keyEvent, bool &override) |
void | sendStringToEmu (const char *) |
Protected Types | |
enum | DragState { diNone, diPending, diDragging } |
Protected Slots | |
void | blinkCursorEvent () |
void | blinkTextEvent () |
void | scrollBarPositionChanged (int value) |
Protected Member Functions | |
QChar | charClass (const Character &ch) const |
void | clearImage () |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | doDrag () |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual bool | event (QEvent *event) |
virtual void | extendSelection (const QPoint &pos) |
virtual void | focusInEvent (QFocusEvent *event) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
virtual void | fontChange (const QFont &) |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
void | mouseTripleClickEvent (QMouseEvent *event) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | wheelEvent (QWheelEvent *event) |
Protected Attributes | |
struct Konsole::TerminalDisplay::DragInfo | _dragInfo |
Detailed Description
A widget which displays output from a terminal emulation and sends input keypresses and mouse activity to the terminal.
When the terminal emulation receives new output from the program running in the terminal, it will update the display by calling updateImage().
TODO More documentation
Definition at line 63 of file TerminalDisplay.h.
Member Enumeration Documentation
|
protected |
Enumerator | |
---|---|
diNone | |
diPending | |
diDragging |
Definition at line 639 of file TerminalDisplay.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a new terminal display widget with the specified parent.
Definition at line 294 of file TerminalDisplay.cpp.
|
virtual |
Definition at line 409 of file TerminalDisplay.cpp.
Member Function Documentation
|
inline |
Returns true if anti-aliasing of text in the terminal is enabled.
Definition at line 392 of file TerminalDisplay.h.
|
slot |
Shows a notification that a bell event has occurred in the terminal.
TODO: More documentation here
Definition at line 3028 of file TerminalDisplay.cpp.
int TerminalDisplay::bellMode | ( | ) | const |
Returns the type of effect used to alert the user when a 'bell' occurs in the terminal session.
See setBellMode()
Definition at line 3018 of file TerminalDisplay.cpp.
|
protectedslot |
Definition at line 1584 of file TerminalDisplay.cpp.
|
inline |
Returns true if the cursor is allowed to blink or false otherwise.
Definition at line 151 of file TerminalDisplay.h.
|
protectedslot |
Definition at line 1573 of file TerminalDisplay.cpp.
|
signal |
|
signal |
|
protected |
Definition at line 2632 of file TerminalDisplay.cpp.
|
protected |
Definition at line 1675 of file TerminalDisplay.cpp.
const ColorEntry * TerminalDisplay::colorTable | ( | ) | const |
Returns the terminal color palette used by the display.
Definition at line 124 of file TerminalDisplay.cpp.
|
inline |
Returns the number of characters of text which can be displayed on each line in the widget.
This will depend upon the width of the widget and the current font. See fontWidth()
Definition at line 278 of file TerminalDisplay.h.
|
signal |
Emitted when the user right clicks on the display, or right-clicks with the Shift key held down if usesMouse() is true.
This can be used to display a context menu.
|
protectedvirtual |
Definition at line 2999 of file TerminalDisplay.cpp.
|
slot |
Copies the selected text to the system clipboard.
Definition at line 2735 of file TerminalDisplay.cpp.
|
slot |
Copies the selected text to the X11 Selection.
Definition at line 2720 of file TerminalDisplay.cpp.
|
inline |
Definition at line 161 of file TerminalDisplay.h.
void TerminalDisplay::decreaseFontSize | ( | ) |
Decreases the font size.
Definition at line 246 of file TerminalDisplay.cpp.
|
protected |
Definition at line 3186 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 3081 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 3093 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2982 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2057 of file TerminalDisplay.cpp.
QList< QAction * > TerminalDisplay::filterActions | ( | const QPoint & | position | ) |
Returns a list of menu actions created by the filters for the content at the given position
.
Definition at line 1934 of file TerminalDisplay.cpp.
FilterChain * TerminalDisplay::filterChain | ( | ) | const |
Returns the display's filter chain.
When the image for the display is updated, the text is passed through each filter in the chain. Each filter can define hotspots which correspond to certain strings (such as URLs or particular words). Depending on the type of the hotspots created by the filter ( returned by Filter::Hotspot::type() ) the view will draw visual cues such as underlines on mouse-over for links or translucent rectangles for markers.
To add a new filter to the view, call: viewWidget->filterChain()->addFilter( filterObject );
Definition at line 1209 of file TerminalDisplay.cpp.
|
inlineslot |
Returns true if the flow control warning box is enabled.
See outputSuspended() and setFlowControlWarningEnabled()
Definition at line 504 of file TerminalDisplay.h.
|
protectedvirtual |
Definition at line 1562 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2622 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 1540 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 174 of file TerminalDisplay.cpp.
|
inline |
Returns the height of the characters in the font used to draw the text in the display.
Definition at line 285 of file TerminalDisplay.h.
|
inline |
Returns the width of the characters in the display.
This assumes the use of a fixed-width font.
Definition at line 292 of file TerminalDisplay.h.
|
slot |
Gets the background of the display.
Definition at line 141 of file TerminalDisplay.cpp.
|
inline |
Returns true if characters with intense colors are rendered in bold.
Definition at line 406 of file TerminalDisplay.h.
|
inline |
Returns true if links and email addresses should be opened when clicked with the mouse.
Definition at line 200 of file TerminalDisplay.h.
|
inline |
Returns true if links and email addresses should be underlined when hovered by the mouse.
Definition at line 185 of file TerminalDisplay.h.
|
inline |
Returns the font used to draw characters in the display.
Definition at line 366 of file TerminalDisplay.h.
|
protectedvirtual |
Definition at line 1763 of file TerminalDisplay.cpp.
void TerminalDisplay::increaseFontSize | ( | ) |
Increases the font size.
Definition at line 239 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2765 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2778 of file TerminalDisplay.cpp.
|
inline |
Returns the status of the BiDi rendering in this widget.
Definition at line 437 of file TerminalDisplay.h.
QColor TerminalDisplay::keyboardCursorColor | ( | ) | const |
Returns the color of the keyboard cursor, or an invalid color if the keyboard cursor color is set to change according to the foreground color of the character underneath it.
Definition at line 570 of file TerminalDisplay.cpp.
Enum::CursorShapeEnum TerminalDisplay::keyboardCursorShape | ( | ) | const |
Returns the shape of the keyboard cursor.
Definition at line 562 of file TerminalDisplay.cpp.
|
signal |
Emitted when the user presses a key whilst the terminal widget has focus.
|
protectedvirtual |
Definition at line 2908 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2048 of file TerminalDisplay.cpp.
|
inline |
Returns the number of lines of text which can be displayed in the widget.
This will depend upon the height of the widget and the current font. See fontHeight()
Definition at line 268 of file TerminalDisplay.h.
uint TerminalDisplay::lineSpacing | ( | ) | const |
Definition at line 255 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2341 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 1944 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 1856 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2248 of file TerminalDisplay.cpp.
|
signal |
A mouse event occurred.
- Parameters
-
button The mouse button (0 for left button, 1 for middle button, 2 for right button, 3 for release) column The character column where the event occurred line The character row where the event occurred eventType The type of event. 0 for a mouse press / release or 1 for mouse motion
|
protected |
Definition at line 2567 of file TerminalDisplay.cpp.
|
inline |
Returns the whether zoom terminal on Ctrl+mousewheel is enabled.
Definition at line 355 of file TerminalDisplay.h.
|
slot |
Causes the widget to display or hide a message informing the user that terminal output has been suspended (by using the flow control key combination Ctrl+S)
- Parameters
-
suspended True if terminal output has been suspended and the warning message should be shown or false to indicate that terminal output has been resumed and that the warning message should disappear.
Definition at line 2861 of file TerminalDisplay.cpp.
|
signal |
When a shortcut which is also a valid terminal key sequence is pressed while the terminal widget has focus, this signal is emitted to allow the host to decide whether the shortcut should be overridden.
When the shortcut is overridden, the key sequence will be sent to the terminal emulation instead and the action associated with the shortcut will not be triggered.
override
is set to false by default and the shortcut will be triggered as normal.
|
protectedvirtual |
Definition at line 1166 of file TerminalDisplay.cpp.
|
slot |
Pastes the content of the clipboard into the display.
Definition at line 2747 of file TerminalDisplay.cpp.
|
slot |
Pastes the content of the X11 selection into the display.
Definition at line 2753 of file TerminalDisplay.cpp.
void TerminalDisplay::printContent | ( | QPainter & | painter, |
bool | friendly | ||
) |
Definition at line 1180 of file TerminalDisplay.cpp.
void TerminalDisplay::processFilters | ( | ) |
Updates the filters in the display's filter chain.
This will cause the hotspots to be updated to match the current image.
WARNING: This function can be expensive depending on the image size and number of filters in the filterChain()
TODO - This API does not really allow efficient usage. Revise it so that the processing can be done in a better way.
eg:
- Area of interest may be known ( eg. mouse cursor hovering over an area )
Definition at line 939 of file TerminalDisplay.cpp.
uint TerminalDisplay::randomSeed | ( | ) | const |
Returns the seed used to generate random colors for the display (in color schemes that support them).
Definition at line 804 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 1604 of file TerminalDisplay.cpp.
ScreenWindow * TerminalDisplay::screenWindow | ( | ) | const |
Returns the terminal screen section which is displayed in this widget.
Definition at line 103 of file TerminalDisplay.cpp.
|
inline |
Definition at line 98 of file TerminalDisplay.h.
|
protectedslot |
Definition at line 1803 of file TerminalDisplay.cpp.
bool TerminalDisplay::scrollFullPage | ( | ) | const |
Definition at line 1846 of file TerminalDisplay.cpp.
|
slot |
Scrolls current ScreenWindow.
it's needed for proper handling scroll commands in the Vt102Emulation class
Definition at line 2899 of file TerminalDisplay.cpp.
|
signal |
SessionController * TerminalDisplay::sessionController | ( | ) |
Definition at line 3201 of file TerminalDisplay.cpp.
|
inline |
Specified whether anti-aliasing of text in the terminal display is enabled or not.
Defaults to enabled.
Definition at line 386 of file TerminalDisplay.h.
|
slot |
Definition at line 2710 of file TerminalDisplay.cpp.
|
slot |
Sets the background of the display to the specified color.
Definition at line 128 of file TerminalDisplay.cpp.
void TerminalDisplay::setBellMode | ( | int | mode | ) |
Sets the type of effect used to alert the user when a 'bell' occurs in the terminal session.
The terminal session can trigger the bell effect by calling bell() with the alert message.
Definition at line 3013 of file TerminalDisplay.cpp.
|
inline |
Sets the status of the BiDi rendering inside the terminal display.
Defaults to disabled.
Definition at line 431 of file TerminalDisplay.h.
void TerminalDisplay::setBlinkingCursorEnabled | ( | bool | blink | ) |
Specifies whether or not the cursor can blink.
Definition at line 1510 of file TerminalDisplay.cpp.
void TerminalDisplay::setBlinkingTextEnabled | ( | bool | blink | ) |
Specifies whether or not text can blink.
Definition at line 1527 of file TerminalDisplay.cpp.
|
inline |
Specifies whether characters with intense colors should be rendered as bold.
Defaults to true.
Definition at line 400 of file TerminalDisplay.h.
|
slot |
Sets whether the contents are centered between the margins.
Definition at line 1774 of file TerminalDisplay.cpp.
void TerminalDisplay::setColorTable | ( | const ColorEntry | table[] | ) |
Sets the terminal color palette used by the display.
Definition at line 152 of file TerminalDisplay.cpp.
|
inline |
Definition at line 158 of file TerminalDisplay.h.
void TerminalDisplay::setFixedSize | ( | int | columns, |
int | lines | ||
) |
Definition at line 1731 of file TerminalDisplay.cpp.
|
slot |
Changes whether the flow control warning box should be shown when the flow control stop key (Ctrl+S) are pressed.
Definition at line 2851 of file TerminalDisplay.cpp.
|
virtual |
Reimplemented.
Has no effect. Use setVTFont() to change the font used to draw characters in the display.
Definition at line 234 of file TerminalDisplay.cpp.
|
slot |
Sets the text of the display to the specified color.
Definition at line 146 of file TerminalDisplay.cpp.
void TerminalDisplay::setKeyboardCursorColor | ( | const QColor & | color | ) |
Sets the color used to draw the keyboard cursor.
The keyboard cursor defaults to using the foreground color of the character underneath it.
- Parameters
-
color By default, the widget uses the color of the character under the cursor to draw the cursor, and inverts the color of that character to make sure it is still readable. If color
is a valid QColor, the widget uses that color to draw the cursor. Ifcolor
is not an valid QColor, the widget falls back to the default behavior.
Definition at line 566 of file TerminalDisplay.cpp.
void TerminalDisplay::setKeyboardCursorShape | ( | Enum::CursorShapeEnum | shape | ) |
Sets the shape of the keyboard cursor.
This is the cursor drawn at the position in the terminal where keyboard input will appear.
In addition the terminal display widget also has a cursor for the mouse pointer, which can be set using the QWidget::setCursor() method.
Defaults to BlockCursor
Definition at line 558 of file TerminalDisplay.cpp.
void TerminalDisplay::setLineSpacing | ( | uint | i | ) |
Definition at line 260 of file TerminalDisplay.cpp.
|
slot |
Sets the display's contents margins.
Definition at line 1768 of file TerminalDisplay.cpp.
|
slot |
Definition at line 2715 of file TerminalDisplay.cpp.
|
inline |
Specified whether zoom terminal on Ctrl+mousewheel is enabled or not.
Defaults to enabled.
Definition at line 347 of file TerminalDisplay.h.
void TerminalDisplay::setOpacity | ( | qreal | opacity | ) |
Sets the opacity of the terminal display.
Definition at line 575 of file TerminalDisplay.cpp.
|
inline |
Specifies whether links and email addresses should be opened when clicked with the mouse.
Defaults to false.
Definition at line 193 of file TerminalDisplay.h.
void TerminalDisplay::setRandomSeed | ( | uint | seed | ) |
Sets the seed used to generate random colors for the display (in color schemes that support them).
Definition at line 800 of file TerminalDisplay.cpp.
void TerminalDisplay::setScreenWindow | ( | ScreenWindow * | window | ) |
Sets the terminal screen section which is displayed in this widget.
When updateImage() is called, the display fetches the latest character image from the the associated terminal screen window.
In terms of the model-view paradigm, the ScreenWindow is the model which is rendered by the TerminalDisplay.
Definition at line 107 of file TerminalDisplay.cpp.
void TerminalDisplay::setScroll | ( | int | cursor, |
int | lines | ||
) |
Sets the current position and range of the display's scroll bar.
- Parameters
-
cursor The position of the scroll bar's thumb. lines The maximum value of the scroll bar.
Definition at line 1820 of file TerminalDisplay.cpp.
void TerminalDisplay::setScrollBarPosition | ( | Enum::ScrollBarPositionEnum | position | ) |
Specifies whether the terminal display has a vertical scroll bar, and if so whether it is shown on the left or right side of the display.
Definition at line 1787 of file TerminalDisplay.cpp.
void TerminalDisplay::setScrollFullPage | ( | bool | fullPage | ) |
Definition at line 1841 of file TerminalDisplay.cpp.
void TerminalDisplay::setSessionController | ( | SessionController * | controller | ) |
Definition at line 3196 of file TerminalDisplay.cpp.
|
inline |
Sets whether or not the current height and width of the terminal in lines and columns is displayed whilst the widget is being resized.
Definition at line 415 of file TerminalDisplay.h.
void TerminalDisplay::setSize | ( | int | columns, |
int | lines | ||
) |
Definition at line 1716 of file TerminalDisplay.cpp.
|
inline |
Sets whether trailing spaces should be trimmed in selected text.
Definition at line 207 of file TerminalDisplay.h.
|
inline |
Sets how the text is selected when the user triple clicks within the display.
Definition at line 166 of file TerminalDisplay.h.
|
inline |
Specifies whether links and email addresses should be underlined when hovered by the mouse.
Defaults to true.
Definition at line 178 of file TerminalDisplay.h.
|
slot |
Sets whether the program whose output is being displayed in the view is interested in mouse events.
If this is set to true, mouse signals will be emitted by the view when the user clicks, drags or otherwise moves the mouse inside the view. The user interaction needed to create selections will also change, and the user will be required to hold down the shift key to create a selection or perform other mouse activities inside the view area - since the program running in the terminal is being allowed to handle normal mouse events itself.
- Parameters
-
usesMouse Set to true if the program running in the terminal is interested in mouse events or false otherwise.
Definition at line 2666 of file TerminalDisplay.cpp.
void TerminalDisplay::setVTFont | ( | const QFont & | font | ) |
Sets the font used to draw the display.
Has no effect if font
is larger than the size of the display itself.
Definition at line 205 of file TerminalDisplay.cpp.
void TerminalDisplay::setWallpaper | ( | ColorSchemeWallpaper::Ptr | p | ) |
Sets the background picture.
Definition at line 594 of file TerminalDisplay.cpp.
void TerminalDisplay::setWordCharacters | ( | const QString & | wc | ) |
Sets which characters, in addition to letters and numbers, are regarded as being part of a word for the purposes of selecting words in the display by double clicking on them.
The word boundaries occur at the first and last characters which are either a letter, number, or a character in wc
- Parameters
-
wc An array of characters which are to be considered parts of a word ( in addition to letters and numbers ).
Definition at line 2658 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 1759 of file TerminalDisplay.cpp.
|
inline |
Returns whether or not the current height and width of the terminal in lines and columns is displayed whilst the widget is being resized.
Definition at line 423 of file TerminalDisplay.h.
QSize TerminalDisplay::sizeHint | ( | ) | const |
Definition at line 1749 of file TerminalDisplay.cpp.
|
inline |
Returns true if trailing spaces should be trimmed in selected text.
Definition at line 214 of file TerminalDisplay.h.
|
inline |
See setTripleClickSelectionMode()
Definition at line 170 of file TerminalDisplay.h.
|
slot |
Causes the terminal display to fetch the latest character image from the associated terminal screen ( see setScreenWindow() ) and redraw the display.
Definition at line 962 of file TerminalDisplay.cpp.
|
slot |
Causes the terminal display to fetch the latest line status flags from the associated terminal screen ( see setScreenWindow() ).
Definition at line 2312 of file TerminalDisplay.cpp.
|
slot |
See setUsesMouse()
Definition at line 2671 of file TerminalDisplay.cpp.
void TerminalDisplay::visualBell | ( | ) |
Play a visual bell for prompt or warning.
Definition at line 3059 of file TerminalDisplay.cpp.
|
protectedvirtual |
Definition at line 2434 of file TerminalDisplay.cpp.
|
inline |
Returns the characters which are considered part of a word for the purpose of selecting words in the display with the mouse.
- See also
- setWordCharacters()
Definition at line 320 of file TerminalDisplay.h.
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.