Konsole
#include <SessionController.h>
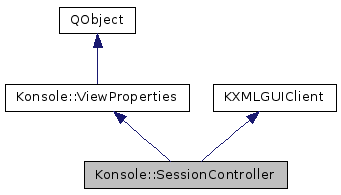
Public Types | |
enum | CopyInputToEnum { CopyInputToAllTabsMode = 0, CopyInputToSelectedTabsMode = 1, CopyInputToNoneMode = 2 } |
Public Slots | |
void | closeSession () |
void | decreaseFontSize () |
void | increaseFontSize () |
void | openUrl (const KUrl &url) |
void | selectionChanged (const QString &selectedText) |
void | setupPrimaryScreenSpecificActions (bool use) |
![]() |
Signals | |
void | currentDirectoryChanged (const QString &dir) |
void | focused (SessionController *controller) |
void | rawTitleChanged () |
![]() | |
void | activity (ViewProperties *item) |
void | iconChanged (ViewProperties *properties) |
void | titleChanged (ViewProperties *properties) |
Public Member Functions | |
SessionController (Session *session, TerminalDisplay *view, QObject *parent) | |
~SessionController () | |
virtual bool | confirmClose () const |
virtual bool | confirmForceClose () const |
virtual QString | currentDir () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
bool | isValid () const |
EditProfileDialog * | profileDialogPointer () |
virtual void | rename () |
IncrementalSearchBar * | searchBar () const |
QPointer< Session > | session () |
void | setSearchBar (IncrementalSearchBar *searchBar) |
void | setSearchStartTo (int line) |
void | setSearchStartToWindowCurrentLine () |
void | setShowMenuAction (QAction *action) |
virtual KUrl | url () const |
QString | userTitle () const |
QPointer< TerminalDisplay > | view () |
![]() | |
ViewProperties (QObject *parent) | |
virtual | ~ViewProperties () |
QIcon | icon () const |
int | identifier () const |
QString | title () const |
Static Public Member Functions | |
static QSet< SessionController * > | allControllers () |
![]() | |
static QMimeData * | createMimeData (int id) |
static int | decodeMimeData (const QMimeData *mimeData) |
static QString | mimeType () |
static ViewProperties * | propertiesById (int id) |
Additional Inherited Members | |
![]() | |
void | fireActivity () |
![]() | |
void | setIcon (const QIcon &icon) |
void | setIdentifier (int id) |
void | setTitle (const QString &title) |
Detailed Description
Provides the menu actions to manipulate a single terminal session and view pair.
The actions provided by this class are defined in the sessionui.rc XML file.
SessionController monitors the session and provides access to basic information about the session such as title(), icon() and currentDir(). SessionController provides notifications of activity in the session via the activity() signal.
When the controlled view receives the focus, the focused() signal is emitted with a pointer to the controller. This can be used by main application window which contains the view to plug the controller's actions into the menu when the view is focused.
Definition at line 85 of file SessionController.h.
Member Enumeration Documentation
Definition at line 90 of file SessionController.h.
Constructor & Destructor Documentation
SessionController::SessionController | ( | Session * | session, |
TerminalDisplay * | view, | ||
QObject * | parent | ||
) |
Constructs a new SessionController which operates on session
and view
.
Definition at line 100 of file SessionController.cpp.
SessionController::~SessionController | ( | ) |
Definition at line 217 of file SessionController.cpp.
Member Function Documentation
|
inlinestatic |
Returns the set of all controllers that exist.
Definition at line 168 of file SessionController.h.
|
slot |
close the associated session.
This might involve user interaction for confirmation.
Definition at line 900 of file SessionController.cpp.
|
virtual |
Sub-classes may re-implement this method to display a message to the user to allow them to confirm whether to close a view.
The default implementation always returns true
Reimplemented from Konsole::ViewProperties.
Definition at line 850 of file SessionController.cpp.
|
virtual |
Definition at line 875 of file SessionController.cpp.
|
virtual |
Returns the current directory associated with a view.
This may be the same as url() The default implementation returns an empty string.
Reimplemented from Konsole::ViewProperties.
Definition at line 285 of file SessionController.cpp.
|
signal |
Emitted when the current working directory of the session associated with the controller is changed.
|
slot |
Decrease font size.
Definition at line 1437 of file SessionController.cpp.
|
virtual |
Definition at line 458 of file SessionController.cpp.
|
signal |
Emitted when the view associated with the controller is focused.
This can be used by other classes to plug the controller's actions into a window's menus.
|
slot |
Increase font size.
Definition at line 1432 of file SessionController.cpp.
|
inline |
Returns true if the controller is valid.
A valid controller is one which has a non-null session() and view().
Equivalent to "!session().isNull() && !view().isNull()"
Definition at line 363 of file SessionController.h.
|
slot |
Issues a command to the session to navigate to the specified URL.
This may not succeed if the foreground program does not understand the command sent to it ( 'cd path' for local URLs ) or is not responding to input.
openUrl() currently supports urls for local paths and those using the 'ssh' protocol ( eg. "ssh://joebloggs@hostname" )
Definition at line 300 of file SessionController.cpp.
EditProfileDialog * SessionController::profileDialogPointer | ( | ) |
Definition at line 794 of file SessionController.cpp.
|
signal |
|
virtual |
Requests the renaming of this view.
The default implementation does nothing.
Reimplemented from Konsole::ViewProperties.
Definition at line 295 of file SessionController.cpp.
IncrementalSearchBar * SessionController::searchBar | ( | ) | const |
see setSearchBar()
Definition at line 543 of file SessionController.cpp.
|
slot |
update actions which are closely related with the selected text.
Definition at line 374 of file SessionController.cpp.
|
inline |
Returns the session associated with this controller.
Definition at line 108 of file SessionController.h.
void SessionController::setSearchBar | ( | IncrementalSearchBar * | searchBar | ) |
Sets the widget used for searches through the session's output.
When the user clicks on the "Search Output" menu action the searchBar
's show() method will be called. The SessionController will then connect to the search bar's signals to update the search when the widget's controls are pressed.
Definition at line 519 of file SessionController.cpp.
void SessionController::setSearchStartTo | ( | int | line | ) |
Set the start line from which the next search will be done.
Definition at line 1096 of file SessionController.cpp.
void SessionController::setSearchStartToWindowCurrentLine | ( | ) |
set start line to the first or last line (depending on the reverse search setting) in the terminal display
Definition at line 1091 of file SessionController.cpp.
void SessionController::setShowMenuAction | ( | QAction * | action | ) |
Sets the action displayed in the session's context menu to hide or show the menu bar.
Definition at line 548 of file SessionController.cpp.
|
slot |
update actions which are meaningful only when primary screen is in use.
Definition at line 361 of file SessionController.cpp.
|
virtual |
Returns the URL current associated with a view.
The default implementation returns an empty URL.
Reimplemented from Konsole::ViewProperties.
Definition at line 290 of file SessionController.cpp.
QString SessionController::userTitle | ( | ) | const |
Returns the "window title" of the associated session.
Definition at line 1975 of file SessionController.cpp.
|
inline |
Returns the view associated with this controller.
Definition at line 112 of file SessionController.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.