Konsole
#include <Session.h>
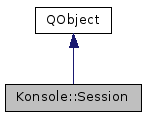
Public Types | |
enum | TabTitleContext { LocalTabTitle, RemoteTabTitle } |
enum | TitleRole { NameRole, DisplayedTitleRole } |
enum | UserTitleChange { IconNameAndWindowTitle = 0, IconName = 1, WindowTitle = 2, TextColor = 10, BackgroundColor = 11, SessionName = 30, SessionIcon = 32, ProfileChange = 50 } |
Public Slots | |
void | addEnvironmentEntry (const QString &entry) |
void | close () |
bool | closeInForceWay () |
bool | closeInNormalWay () |
Q_SCRIPTABLE QByteArray | codec () |
Q_SCRIPTABLE QStringList | environment () const |
Q_SCRIPTABLE bool | flowControlEnabled () const |
Q_SCRIPTABLE int | foregroundProcessId () |
Q_SCRIPTABLE int | historySize () const |
Q_SCRIPTABLE bool | isMonitorActivity () const |
Q_SCRIPTABLE bool | isMonitorSilence () const |
Q_SCRIPTABLE int | processId () const |
void | run () |
Q_SCRIPTABLE void | runCommand (const QString &command) const |
Q_SCRIPTABLE void | sendMouseEvent (int buttons, int column, int line, int eventType) |
Q_SCRIPTABLE void | sendText (const QString &text) const |
Q_SCRIPTABLE bool | setCodec (QByteArray codec) |
Q_SCRIPTABLE void | setEnvironment (const QStringList &environment) |
Q_SCRIPTABLE void | setFlowControlEnabled (bool enabled) |
Q_SCRIPTABLE void | setHistorySize (int lines) |
Q_SCRIPTABLE void | setMonitorActivity (bool) |
Q_SCRIPTABLE void | setMonitorSilence (bool) |
Q_SCRIPTABLE void | setMonitorSilenceSeconds (int seconds) |
Q_SCRIPTABLE void | setTabTitleFormat (int context, const QString &format) |
Q_SCRIPTABLE void | setTitle (int role, const QString &title) |
void | setUserTitle (int what, const QString &caption) |
Q_SCRIPTABLE QString | shellSessionId () const |
Q_SCRIPTABLE QString | tabTitleFormat (int context) const |
Q_SCRIPTABLE QString | title (int role) const |
Signals | |
void | bellRequest (const QString &message) |
void | changeBackgroundColorRequest (const QColor &) |
void | changeForegroundColorRequest (const QColor &) |
void | changeTabTextColorRequest (int) |
void | currentDirectoryChanged (const QString &dir) |
void | finished () |
void | flowControlEnabledChanged (bool enabled) |
void | openUrlRequest (const QString &url) |
void | primaryScreenInUse (bool use) |
void | profileChangeCommandReceived (const QString &text) |
void | resizeRequest (const QSize &size) |
void | selectionChanged (const QString &text) |
void | started () |
void | stateChanged (int state) |
void | titleChanged () |
void | zmodemDetected () |
Public Member Functions | |
Session (QObject *parent=0) | |
~Session () | |
void | addView (TerminalDisplay *widget) |
QStringList | arguments () const |
bool | autoClose () const |
void | cancelZModem () |
void | clearHistory () |
QString | currentWorkingDirectory () |
Emulation * | emulation () const |
QString | foregroundProcessName () |
QString | getDynamicTitle () |
KUrl | getUrl () |
const HistoryType & | historyType () const |
QString | iconName () const |
QString | iconText () const |
QString | initialWorkingDirectory () |
bool | isForegroundProcessActive () |
bool | isRemote () |
bool | isRunning () const |
bool | isZModemBusy () |
QString | keyBindings () const |
QString | nameTitle () const |
void | openTeletype (int masterFd) |
QSize | preferredSize () const |
QString | program () const |
void | refresh () |
void | removeView (TerminalDisplay *widget) |
void | restoreSession (KConfigGroup &group) |
void | saveSession (KConfigGroup &group) |
void | sendSignal (int signal) |
int | sessionId () const |
void | setAddToUtmp (bool) |
void | setArguments (const QStringList &arguments) |
void | setAutoClose (bool close) |
void | setCodec (QTextCodec *codec) |
void | setDarkBackground (bool darkBackground) |
void | setHistoryType (const HistoryType &type) |
void | setIconName (const QString &iconName) |
void | setIconText (const QString &iconText) |
void | setInitialWorkingDirectory (const QString &dir) |
void | setKeyBindings (const QString &name) |
void | setPreferredSize (const QSize &size) |
void | setProgram (const QString &program) |
void | setSize (const QSize &size) |
void | setTabTitleFormat (TabTitleContext context, const QString &format) |
void | setTitle (TitleRole role, const QString &title) |
QSize | size () |
void | startZModem (const QString &rz, const QString &dir, const QStringList &list) |
QString | tabTitleFormat (TabTitleContext context) const |
QString | title (TitleRole role) const |
QString | userTitle () const |
QList< TerminalDisplay * > | views () const |
Properties | |
QString | keyBindings |
QString | name |
int | processId |
QSize | size |
Detailed Description
Represents a terminal session consisting of a pseudo-teletype and a terminal emulation.
The pseudo-teletype (or PTY) handles I/O between the terminal process and Konsole. The terminal emulation ( Emulation and subclasses ) processes the output stream from the PTY and produces a character image which is then shown on views connected to the session.
Each Session can be connected to one or more views by using the addView() method. The attached views can then display output from the program running in the terminal or send input to the program in the terminal in the form of keypresses and mouse activity.
Member Enumeration Documentation
Possible values of the what
parameter for setUserTitle() See "Operating System Controls" section on http://rtfm.etla.org/xterm/ctlseq.html.
Enumerator | |
---|---|
IconNameAndWindowTitle | |
IconName | |
WindowTitle | |
TextColor | |
BackgroundColor | |
SessionName | |
SessionIcon | |
ProfileChange |
Constructor & Destructor Documentation
|
explicit |
Constructs a new session.
To start the terminal process, call the run() method, after specifying the program and arguments using setProgram() and setArguments()
If no program or arguments are specified explicitly, the Session falls back to using the program specified in the SHELL environment variable.
Definition at line 98 of file Session.cpp.
Session::~Session | ( | ) |
Definition at line 161 of file Session.cpp.
Member Function Documentation
|
slot |
Adds one entry for the environment of this session entry
should be like VARIABLE=VALUE.
Definition at line 888 of file Session.cpp.
void Session::addView | ( | TerminalDisplay * | widget | ) |
Adds a new view for this session.
The viewing widget will display the output from the terminal and input from the viewing widget (key presses, mouse activity etc.) will be sent to the terminal.
Views can be removed using removeView(). The session is automatically closed when the last view is removed.
Definition at line 308 of file Session.cpp.
QStringList Session::arguments | ( | ) | const |
Returns the arguments passed to the shell process when run() is called.
Definition at line 1075 of file Session.cpp.
bool Session::autoClose | ( | ) | const |
See setAutoClose()
Definition at line 1141 of file Session.cpp.
|
signal |
Emitted when a bell event occurs in the session.
void Session::cancelZModem | ( | ) |
Definition at line 1170 of file Session.cpp.
|
signal |
Requests that the background color of views on this session should be changed.
|
signal |
Requests that the text color of views on this session should be changed to color
.
|
signal |
Requests that the color the text for any tabs associated with this session should be changed;.
TODO: Document what the parameter does
void Session::clearHistory | ( | ) |
Clears the history store used by this session.
Definition at line 1070 of file Session.cpp.
|
slot |
Closes the terminal session.
It kills the terminal process by calling closeInNormalWay() and, optionally, closeInForceWay().
Definition at line 764 of file Session.cpp.
|
slot |
kill terminal process in force way.
This send a SIGKILL signal to the terminal process. It should be called only after closeInNormalWay() has failed. Take it as last resort.
Definition at line 800 of file Session.cpp.
|
slot |
Kill the terminal process in normal way.
This sends a hangup signal (SIGHUP) to the terminal process and causes the finished() signal to be emitted. If the process does not respond to the SIGHUP signal then the terminal connection (the pty) is closed and Konsole waits for the process to exit. This method works most of the time, but fails with some programs which respond to SIGHUP signal in special way, such as autossh and irssi.
Definition at line 775 of file Session.cpp.
|
slot |
Returns the codec used to decode incoming characters in this terminal emulation.
Definition at line 262 of file Session.cpp.
|
signal |
Emitted when the current working directory of this session changes.
- Parameters
-
dir The new current working directory of the session.
QString Session::currentWorkingDirectory | ( | ) |
Returns the current directory of the foreground process in the session.
Definition at line 282 of file Session.cpp.
Emulation * Session::emulation | ( | ) | const |
Returns the terminal emulation instance being used to encode / decode characters to / from the process.
Definition at line 868 of file Session.cpp.
|
slot |
Returns the environment of this session as a list of strings like VARIABLE=VALUE.
Definition at line 878 of file Session.cpp.
|
signal |
Emitted when the terminal process exits.
|
slot |
Returns whether flow control is enabled for this terminal session.
Definition at line 1155 of file Session.cpp.
|
signal |
Emitted when the flow control state changes.
- Parameters
-
enabled True if flow control is enabled or false otherwise.
|
slot |
Returns the process id of the terminal's foreground process.
This is initially the same as processId() but can change as the user starts other programs inside the terminal.
Definition at line 1386 of file Session.cpp.
QString Session::foregroundProcessName | ( | ) |
Returns the name of the current foreground process.
Definition at line 1404 of file Session.cpp.
QString Session::getDynamicTitle | ( | ) |
Returns a title generated from tab format and process information.
Definition at line 983 of file Session.cpp.
KUrl Session::getUrl | ( | ) |
Return URL for the session.
Definition at line 1001 of file Session.cpp.
|
slot |
Returns the history capacity of this session.
Definition at line 1371 of file Session.cpp.
const HistoryType & Session::historyType | ( | ) | const |
Returns the type of history store used by this session.
Definition at line 1065 of file Session.cpp.
QString Session::iconName | ( | ) | const |
Returns the name of the icon associated with this session.
Definition at line 1050 of file Session.cpp.
QString Session::iconText | ( | ) | const |
Returns the text of the icon associated with this session.
Definition at line 1055 of file Session.cpp.
|
inline |
bool Session::isForegroundProcessActive | ( | ) |
Returns true if the user has started a program in the session.
Definition at line 1398 of file Session.cpp.
|
slot |
Returns true if monitoring for activity is enabled.
Definition at line 1085 of file Session.cpp.
|
slot |
Returns true if monitoring for inactivity (silence) in the session is enabled.
Definition at line 1089 of file Session.cpp.
bool Session::isRemote | ( | ) |
Returns true if the session currently contains a connection to a remote computer.
It currently supports ssh.
Definition at line 975 of file Session.cpp.
bool Session::isRunning | ( | ) | const |
Returns true if the session is currently running.
This will be true after run() has been called successfully.
Definition at line 240 of file Session.cpp.
QString Konsole::Session::keyBindings | ( | ) | const |
Returns the name of the key bindings used by this session.
|
inline |
void Session::openTeletype | ( | int | masterFd | ) |
Connect to an existing terminal.
When a new Session() is constructed it automatically searches for and opens a new teletype. If you want to use an existing teletype (given its file descriptor) call this after constructing the session.
Calling openTeletype() while a session is running has no effect.
- Parameters
-
masterFd The file descriptor of the pseudo-teletype master (See KPtyProcess::KPtyProcess())
Definition at line 170 of file Session.cpp.
|
signal |
TODO: Document me.
QSize Session::preferredSize | ( | ) | const |
Definition at line 1291 of file Session.cpp.
|
signal |
Emitted when the active screen is switched, to indicate whether the primary screen is in use.
This signal serves as a relayer of Emulation::priamyScreenInUse(bool), making it usable for higher level component.
|
slot |
Returns the process id of the terminal process.
This is the id used by the system API to refer to the process.
|
signal |
Emitted when a profile change command is received from the terminal.
- Parameters
-
text The text of the command. This is a string of the form "PropertyName=Value;PropertyName=Value ..."
QString Session::program | ( | ) | const |
Returns the program name of the shell process started when run() is called.
Definition at line 1080 of file Session.cpp.
void Session::refresh | ( | ) |
Attempts to get the shell program to redraw the current display area.
This can be used after clearing the screen, for example, to get the shell to redraw the prompt line.
Definition at line 713 of file Session.cpp.
void Session::removeView | ( | TerminalDisplay * | widget | ) |
Removes a view from this session.
When the last view is removed, the session will be closed automatically.
widget
will no longer display output from or send input to the terminal
Definition at line 350 of file Session.cpp.
|
signal |
Emitted when the terminal process requests a change in the size of the terminal window.
- Parameters
-
size The requested window size in terms of lines and columns.
void Session::restoreSession | ( | KConfigGroup & | group | ) |
Definition at line 1427 of file Session.cpp.
|
slot |
Starts the terminal session.
This creates the terminal process and connects the teletype to it.
Definition at line 422 of file Session.cpp.
|
slot |
Sends command
to the current foreground terminal program.
Definition at line 818 of file Session.cpp.
void Session::saveSession | ( | KConfigGroup & | group | ) |
Definition at line 1418 of file Session.cpp.
|
signal |
Emitted when the text selection is changed.
This signal serves as a relayer of Emulation::selectedText(QString), making it usable for higher level component.
|
slot |
Sends a mouse event of type eventType
emitted by button buttons
on column/
the current foreground terminal program. line
to
Definition at line 823 of file Session.cpp.
void Session::sendSignal | ( | int | signal | ) |
Definition at line 735 of file Session.cpp.
|
slot |
Sends text
to the current foreground terminal program.
Definition at line 813 of file Session.cpp.
int Session::sessionId | ( | ) | const |
Returns the unique ID for this session.
Definition at line 893 of file Session.cpp.
void Session::setAddToUtmp | ( | bool | add | ) |
Specifies whether a utmp entry should be created for the pty used by this session.
If true, KPty::login() is called when the session is started.
Definition at line 1131 of file Session.cpp.
void Session::setArguments | ( | const QStringList & | arguments | ) |
Sets the command line arguments which the session's program will be passed when run() is called.
Definition at line 272 of file Session.cpp.
void Session::setAutoClose | ( | bool | close | ) |
Specifies whether to close the session automatically when the terminal process terminates.
Definition at line 1136 of file Session.cpp.
void Session::setCodec | ( | QTextCodec * | codec | ) |
Definition at line 245 of file Session.cpp.
|
slot |
Sets the text codec used by this sessions terminal emulation.
Overloaded to accept a QByteArray for convenience since DBus does not accept QTextCodec directly.
Definition at line 250 of file Session.cpp.
void Session::setDarkBackground | ( | bool | darkBackground | ) |
Sets whether the session has a dark background or not.
The session uses this information to set the COLORFGBG variable in the process's environment, which allows the programs running in the terminal to determine whether the background is light or dark and use appropriate colors by default.
This has no effect once the session is running.
Definition at line 235 of file Session.cpp.
|
slot |
Sets the environment for this session.
environment
should be a list of strings like VARIABLE=VALUE
Definition at line 883 of file Session.cpp.
|
slot |
Sets whether flow control is enabled for this terminal session.
Definition at line 1146 of file Session.cpp.
|
slot |
Sets the history capacity of this session.
- Parameters
-
lines The history capacity in unit of lines. Its value can be: - positive integer - fixed size history
- 0 - no history
- negative integer - unlimited history
Definition at line 1360 of file Session.cpp.
void Session::setHistoryType | ( | const HistoryType & | type | ) |
Sets the type of history store used by this session.
Lines of output produced by the terminal are added to the history store. The type of history store used affects the number of lines which can be remembered before they are lost and the storage (in memory, on-disk etc.) used.
Definition at line 1060 of file Session.cpp.
void Session::setIconName | ( | const QString & | iconName | ) |
Sets the name of the icon associated with this session.
Definition at line 1037 of file Session.cpp.
void Session::setIconText | ( | const QString & | iconText | ) |
Sets the text of the icon associated with this session.
Definition at line 1045 of file Session.cpp.
void Session::setInitialWorkingDirectory | ( | const QString & | dir | ) |
Sets the initial working directory for the session when it is run This has no effect once the session has been started.
Definition at line 277 of file Session.cpp.
void Session::setKeyBindings | ( | const QString & | name | ) |
Sets the key bindings used by this session.
The bindings specify how input key sequences are translated into the character stream which is sent to the terminal.
- Parameters
-
name The name of the key bindings to use. The names of available key bindings can be determined using the KeyboardTranslatorManager class.
Definition at line 898 of file Session.cpp.
|
slot |
Enables monitoring for activity in the session.
This will cause notifySessionState() to be emitted with the NOTIFYACTIVITY state flag when output is received from the terminal.
Definition at line 1094 of file Session.cpp.
|
slot |
Enables monitoring for silence in the session.
This will cause notifySessionState() to be emitted with the NOTIFYSILENCE state flag when output is not received from the terminal for a certain period of time, specified with setMonitorSilenceSeconds()
Definition at line 1108 of file Session.cpp.
|
slot |
Definition at line 1123 of file Session.cpp.
void Session::setPreferredSize | ( | const QSize & | size | ) |
Definition at line 1296 of file Session.cpp.
void Session::setProgram | ( | const QString & | program | ) |
Sets the program to be executed when run() is called.
Definition at line 267 of file Session.cpp.
void Session::setSize | ( | const QSize & | size | ) |
Emits a request to resize the session to accommodate the specified window size.
- Parameters
-
size The size in lines and columns to request.
Definition at line 1283 of file Session.cpp.
void Session::setTabTitleFormat | ( | TabTitleContext | context, |
const QString & | format | ||
) |
Sets the format used by this session for tab titles.
- Parameters
-
context The context whose format should be set. format The tab title format. This may be a mixture of plain text and dynamic elements denoted by a '' character followed by a letter. (eg. d for directory). The dynamic elements available depend on the context
Definition at line 582 of file Session.cpp.
|
slot |
Sets the session's tab title format for the specified context
to format
.
This is an overloaded member function for setTabTitleFormat(TabTitleContext, QString) provided for convenience since enum data types may not be exported directly through DBus
Definition at line 1336 of file Session.cpp.
void Session::setTitle | ( | TitleRole | role, |
const QString & | title | ||
) |
Sets the session's title for the specified role
to title
.
Definition at line 903 of file Session.cpp.
|
slot |
Sets the session's title for the specified role
to title
.
This is an overloaded member function for setTitle(TitleRole, QString) provided for convenience since enum data types may not be exported directly through DBus
Definition at line 1306 of file Session.cpp.
|
slot |
Changes the session title or other customizable aspects of the terminal emulation display.
For a list of what may be changed see the Emulation::titleChanged() signal.
- Parameters
-
what The feature being changed. Value is one of UserTitleChange caption The text part of the terminal command
Definition at line 512 of file Session.cpp.
|
slot |
Returns the "friendly" version of the QUuid of this session.
This is a QUuid with the braces and dashes removed, so it cannot be used to construct a new QUuid. The same text appears in the SHELL_SESSION_ID environment variable.
Definition at line 414 of file Session.cpp.
QSize Konsole::Session::size | ( | ) |
Returns the terminal session's window size in lines and columns.
|
signal |
Emitted when the terminal process starts.
void Session::startZModem | ( | const QString & | rz, |
const QString & | dir, | ||
const QStringList & | list | ||
) |
Definition at line 1176 of file Session.cpp.
|
signal |
Emitted when the activity state of this session changes.
- Parameters
-
state The new state of the session. This may be one of NOTIFYNORMAL, NOTIFYSILENCE or NOTIFYACTIVITY
QString Session::tabTitleFormat | ( | TabTitleContext | context | ) | const |
Returns the format used by this session for tab titles.
Definition at line 589 of file Session.cpp.
|
slot |
Returns the session's tab title format for the specified context
.
This is an overloaded member function for tabTitleFormat(TitleRole) provided for convenience since enum data types may not be exported directly through DBus
Definition at line 1348 of file Session.cpp.
QString Session::title | ( | TitleRole | role | ) | const |
Returns the session's title for the specified role
.
Definition at line 915 of file Session.cpp.
|
slot |
Returns the session's title for the specified role
.
This is an overloaded member function for setTitle(TitleRole) provided for convenience since enum data types may not be exported directly through DBus
Definition at line 1324 of file Session.cpp.
|
signal |
Emitted when the session's title has changed.
QString Session::userTitle | ( | ) | const |
Return the session title set by the user (ie.
the program running in the terminal), or an empty string if the user has not set a custom title
Definition at line 578 of file Session.cpp.
QList< TerminalDisplay * > Session::views | ( | ) | const |
Returns the views connected to this session.
Definition at line 303 of file Session.cpp.
|
signal |
Emitted when the request for data transmission through ZModem protocol is detected.
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.